Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / DataOracleClient / System / Data / OracleClient / OracleDataAdapter.cs / 1 / OracleDataAdapter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Diagnostics; //--------------------------------------------------------------------- // OracleDataAdapter // // Implements the Oracle Connection object, which connects // to the Oracle server // [ DefaultEvent("RowUpdated"), ToolboxItem("Microsoft.VSDesigner.Data.VS.OracleDataAdapterToolboxItem, " + AssemblyRef.MicrosoftVSDesigner), Designer("Microsoft.VSDesigner.Data.VS.OracleDataAdapterDesigner, " + AssemblyRef.MicrosoftVSDesigner) ] sealed public class OracleDataAdapter : DbDataAdapter, IDbDataAdapter, ICloneable { static internal readonly object EventRowUpdated = new object(); static internal readonly object EventRowUpdating = new object(); private OracleCommand _deleteCommand, _insertCommand, _selectCommand, _updateCommand; private OracleCommandSet _commandSet; private int _updateBatchSize = 1; // Construct an "empty" data adapter public OracleDataAdapter () { GC.SuppressFinalize(this); } // Construct an adapter from a command public OracleDataAdapter (OracleCommand selectCommand) : this() { SelectCommand = selectCommand; } // Construct an adapter from a command text and a connection string public OracleDataAdapter (string selectCommandText, string selectConnectionString) : this() { OracleConnection connection = new OracleConnection(selectConnectionString); SelectCommand = new OracleCommand(); SelectCommand.Connection = connection; SelectCommand.CommandText = selectCommandText; } // Construct an adapter from a command text and a connection public OracleDataAdapter (string selectCommandText, OracleConnection selectConnection) : this() { SelectCommand = new OracleCommand(); SelectCommand.Connection = selectConnection; SelectCommand.CommandText = selectCommandText; } private OracleDataAdapter(OracleDataAdapter from) : base(from) { // Clone GC.SuppressFinalize(this); } [ ResCategoryAttribute(Res.OracleCategory_Update), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_DeleteCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand DeleteCommand { get { return _deleteCommand; } set { _deleteCommand = value; } } IDbCommand IDbDataAdapter.DeleteCommand { get { return _deleteCommand; } set { _deleteCommand = (OracleCommand)value; } } [ ResCategoryAttribute(Res.OracleCategory_Update), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_InsertCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand InsertCommand { get { return _insertCommand; } set { _insertCommand = value; } } IDbCommand IDbDataAdapter.InsertCommand { get { return _insertCommand; } set { _insertCommand = (OracleCommand)value; } } [ ResCategoryAttribute(Res.OracleCategory_Fill), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_SelectCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand SelectCommand { get { return _selectCommand; } set { _selectCommand = value; } } IDbCommand IDbDataAdapter.SelectCommand { get { return _selectCommand; } set { _selectCommand = (OracleCommand)value; } } override public int UpdateBatchSize { get { //Bid.Trace("%d#\n", ObjectID); return _updateBatchSize; } set { if (0 > value) { // WebData 98157 throw ADP.MustBePositive("UpdateBatchSize"); } _updateBatchSize = value; //Bid.Trace(" %d#, %d\n", ObjectID, value); } } [ ResCategoryAttribute(Res.OracleCategory_Update), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_UpdateCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand UpdateCommand { get { return _updateCommand; } set { _updateCommand = value; } } IDbCommand IDbDataAdapter.UpdateCommand { get { return _updateCommand; } set { _updateCommand = (OracleCommand)value; } } override protected int AddToBatch(IDbCommand command) { int commandIdentifier = _commandSet.CommandCount; _commandSet.Append((OracleCommand)command); return commandIdentifier; } override protected void ClearBatch() { _commandSet.Clear(); } object ICloneable.Clone() { return new OracleDataAdapter(this); } override protected RowUpdatedEventArgs CreateRowUpdatedEvent( DataRow dataRow, IDbCommand command, StatementType statementType, DataTableMapping tableMapping ) { return new OracleRowUpdatedEventArgs(dataRow, command, statementType, tableMapping); } override protected RowUpdatingEventArgs CreateRowUpdatingEvent( DataRow dataRow, IDbCommand command, StatementType statementType, DataTableMapping tableMapping ) { return new OracleRowUpdatingEventArgs(dataRow, command, statementType, tableMapping); } override protected int ExecuteBatch() { Debug.Assert(null != _commandSet && (0 < _commandSet.CommandCount), "no commands"); return _commandSet.ExecuteNonQuery(); } override protected IDataParameter GetBatchedParameter(int commandIdentifier, int parameterIndex) { Debug.Assert(commandIdentifier < _commandSet.CommandCount, "commandIdentifier out of range"); Debug.Assert(parameterIndex < _commandSet.GetParameterCount(commandIdentifier), "parameter out of range"); IDataParameter parameter = _commandSet.GetParameter(commandIdentifier, parameterIndex); return parameter; } override protected bool GetBatchedRecordsAffected(int commandIdentifier, out int recordsAffected, out Exception error) { Debug.Assert(commandIdentifier < _commandSet.CommandCount, "commandIdentifier out of range"); error = null; return _commandSet.GetBatchedRecordsAffected(commandIdentifier, out recordsAffected); } override protected void InitializeBatching() { _commandSet = new OracleCommandSet(); OracleCommand command = SelectCommand; if (null == command) { command = InsertCommand; if (null == command) { command = UpdateCommand; if (null == command) { command = DeleteCommand; } } } if (null != command) { _commandSet.Connection = command.Connection; _commandSet.Transaction = command.Transaction; _commandSet.CommandTimeout = command.CommandTimeout; } } override protected void OnRowUpdated(RowUpdatedEventArgs value) { OracleRowUpdatedEventHandler handler = (OracleRowUpdatedEventHandler) Events[EventRowUpdated]; if ((null != handler) && (value is OracleRowUpdatedEventArgs)) { handler(this, (OracleRowUpdatedEventArgs) value); } } override protected void OnRowUpdating(RowUpdatingEventArgs value) { OracleRowUpdatingEventHandler handler = (OracleRowUpdatingEventHandler) Events[EventRowUpdating]; if ((null != handler) && (value is OracleRowUpdatingEventArgs)) { handler(this, (OracleRowUpdatingEventArgs) value); } } override protected void TerminateBatching() { if (null != _commandSet) { _commandSet.Dispose(); _commandSet = null; } } [ ResCategoryAttribute(Res.OracleCategory_Update), ResDescriptionAttribute(Res.DbDataAdapter_RowUpdated) ] public event OracleRowUpdatedEventHandler RowUpdated { add { Events.AddHandler( EventRowUpdated, value); } remove { Events.RemoveHandler(EventRowUpdated, value); } } [ ResCategoryAttribute(Res.OracleCategory_Update), ResDescriptionAttribute(Res.DbDataAdapter_RowUpdating) ] public event OracleRowUpdatingEventHandler RowUpdating { add { OracleRowUpdatingEventHandler handler = (OracleRowUpdatingEventHandler) Events[EventRowUpdating]; // MDAC 58177, 64513 // prevent someone from registering two different command builders on the adapter by // silently removing the old one if ((null != handler) && (value.Target is OracleCommandBuilder)) { OracleRowUpdatingEventHandler d = (OracleRowUpdatingEventHandler) ADP.FindBuilder(handler); if (null != d) { Events.RemoveHandler(EventRowUpdating, d); } } Events.AddHandler(EventRowUpdating, value); } remove { Events.RemoveHandler(EventRowUpdating, value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Data.OracleClient { using System; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Diagnostics; //--------------------------------------------------------------------- // OracleDataAdapter // // Implements the Oracle Connection object, which connects // to the Oracle server // [ DefaultEvent("RowUpdated"), ToolboxItem("Microsoft.VSDesigner.Data.VS.OracleDataAdapterToolboxItem, " + AssemblyRef.MicrosoftVSDesigner), Designer("Microsoft.VSDesigner.Data.VS.OracleDataAdapterDesigner, " + AssemblyRef.MicrosoftVSDesigner) ] sealed public class OracleDataAdapter : DbDataAdapter, IDbDataAdapter, ICloneable { static internal readonly object EventRowUpdated = new object(); static internal readonly object EventRowUpdating = new object(); private OracleCommand _deleteCommand, _insertCommand, _selectCommand, _updateCommand; private OracleCommandSet _commandSet; private int _updateBatchSize = 1; // Construct an "empty" data adapter public OracleDataAdapter () { GC.SuppressFinalize(this); } // Construct an adapter from a command public OracleDataAdapter (OracleCommand selectCommand) : this() { SelectCommand = selectCommand; } // Construct an adapter from a command text and a connection string public OracleDataAdapter (string selectCommandText, string selectConnectionString) : this() { OracleConnection connection = new OracleConnection(selectConnectionString); SelectCommand = new OracleCommand(); SelectCommand.Connection = connection; SelectCommand.CommandText = selectCommandText; } // Construct an adapter from a command text and a connection public OracleDataAdapter (string selectCommandText, OracleConnection selectConnection) : this() { SelectCommand = new OracleCommand(); SelectCommand.Connection = selectConnection; SelectCommand.CommandText = selectCommandText; } private OracleDataAdapter(OracleDataAdapter from) : base(from) { // Clone GC.SuppressFinalize(this); } [ ResCategoryAttribute(Res.OracleCategory_Update), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_DeleteCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand DeleteCommand { get { return _deleteCommand; } set { _deleteCommand = value; } } IDbCommand IDbDataAdapter.DeleteCommand { get { return _deleteCommand; } set { _deleteCommand = (OracleCommand)value; } } [ ResCategoryAttribute(Res.OracleCategory_Update), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_InsertCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand InsertCommand { get { return _insertCommand; } set { _insertCommand = value; } } IDbCommand IDbDataAdapter.InsertCommand { get { return _insertCommand; } set { _insertCommand = (OracleCommand)value; } } [ ResCategoryAttribute(Res.OracleCategory_Fill), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_SelectCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand SelectCommand { get { return _selectCommand; } set { _selectCommand = value; } } IDbCommand IDbDataAdapter.SelectCommand { get { return _selectCommand; } set { _selectCommand = (OracleCommand)value; } } override public int UpdateBatchSize { get { //Bid.Trace("%d#\n", ObjectID); return _updateBatchSize; } set { if (0 > value) { // WebData 98157 throw ADP.MustBePositive("UpdateBatchSize"); } _updateBatchSize = value; //Bid.Trace(" %d#, %d\n", ObjectID, value); } } [ ResCategoryAttribute(Res.OracleCategory_Update), DefaultValue(null), ResDescriptionAttribute(Res.DbDataAdapter_UpdateCommand), Editor("Microsoft.VSDesigner.Data.Design.DBCommandEditor, " + AssemblyRef.MicrosoftVSDesigner, "System.Drawing.Design.UITypeEditor, " + AssemblyRef.SystemDrawing) ] new public OracleCommand UpdateCommand { get { return _updateCommand; } set { _updateCommand = value; } } IDbCommand IDbDataAdapter.UpdateCommand { get { return _updateCommand; } set { _updateCommand = (OracleCommand)value; } } override protected int AddToBatch(IDbCommand command) { int commandIdentifier = _commandSet.CommandCount; _commandSet.Append((OracleCommand)command); return commandIdentifier; } override protected void ClearBatch() { _commandSet.Clear(); } object ICloneable.Clone() { return new OracleDataAdapter(this); } override protected RowUpdatedEventArgs CreateRowUpdatedEvent( DataRow dataRow, IDbCommand command, StatementType statementType, DataTableMapping tableMapping ) { return new OracleRowUpdatedEventArgs(dataRow, command, statementType, tableMapping); } override protected RowUpdatingEventArgs CreateRowUpdatingEvent( DataRow dataRow, IDbCommand command, StatementType statementType, DataTableMapping tableMapping ) { return new OracleRowUpdatingEventArgs(dataRow, command, statementType, tableMapping); } override protected int ExecuteBatch() { Debug.Assert(null != _commandSet && (0 < _commandSet.CommandCount), "no commands"); return _commandSet.ExecuteNonQuery(); } override protected IDataParameter GetBatchedParameter(int commandIdentifier, int parameterIndex) { Debug.Assert(commandIdentifier < _commandSet.CommandCount, "commandIdentifier out of range"); Debug.Assert(parameterIndex < _commandSet.GetParameterCount(commandIdentifier), "parameter out of range"); IDataParameter parameter = _commandSet.GetParameter(commandIdentifier, parameterIndex); return parameter; } override protected bool GetBatchedRecordsAffected(int commandIdentifier, out int recordsAffected, out Exception error) { Debug.Assert(commandIdentifier < _commandSet.CommandCount, "commandIdentifier out of range"); error = null; return _commandSet.GetBatchedRecordsAffected(commandIdentifier, out recordsAffected); } override protected void InitializeBatching() { _commandSet = new OracleCommandSet(); OracleCommand command = SelectCommand; if (null == command) { command = InsertCommand; if (null == command) { command = UpdateCommand; if (null == command) { command = DeleteCommand; } } } if (null != command) { _commandSet.Connection = command.Connection; _commandSet.Transaction = command.Transaction; _commandSet.CommandTimeout = command.CommandTimeout; } } override protected void OnRowUpdated(RowUpdatedEventArgs value) { OracleRowUpdatedEventHandler handler = (OracleRowUpdatedEventHandler) Events[EventRowUpdated]; if ((null != handler) && (value is OracleRowUpdatedEventArgs)) { handler(this, (OracleRowUpdatedEventArgs) value); } } override protected void OnRowUpdating(RowUpdatingEventArgs value) { OracleRowUpdatingEventHandler handler = (OracleRowUpdatingEventHandler) Events[EventRowUpdating]; if ((null != handler) && (value is OracleRowUpdatingEventArgs)) { handler(this, (OracleRowUpdatingEventArgs) value); } } override protected void TerminateBatching() { if (null != _commandSet) { _commandSet.Dispose(); _commandSet = null; } } [ ResCategoryAttribute(Res.OracleCategory_Update), ResDescriptionAttribute(Res.DbDataAdapter_RowUpdated) ] public event OracleRowUpdatedEventHandler RowUpdated { add { Events.AddHandler( EventRowUpdated, value); } remove { Events.RemoveHandler(EventRowUpdated, value); } } [ ResCategoryAttribute(Res.OracleCategory_Update), ResDescriptionAttribute(Res.DbDataAdapter_RowUpdating) ] public event OracleRowUpdatingEventHandler RowUpdating { add { OracleRowUpdatingEventHandler handler = (OracleRowUpdatingEventHandler) Events[EventRowUpdating]; // MDAC 58177, 64513 // prevent someone from registering two different command builders on the adapter by // silently removing the old one if ((null != handler) && (value.Target is OracleCommandBuilder)) { OracleRowUpdatingEventHandler d = (OracleRowUpdatingEventHandler) ADP.FindBuilder(handler); if (null != d) { Events.RemoveHandler(EventRowUpdating, d); } } Events.AddHandler(EventRowUpdating, value); } remove { Events.RemoveHandler(EventRowUpdating, value); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
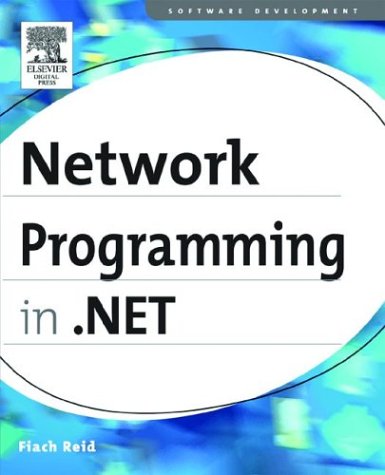
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MdiWindowListStrip.cs
- AssemblyCache.cs
- ContainerVisual.cs
- CmsInterop.cs
- SessionEndingEventArgs.cs
- HttpListenerRequestUriBuilder.cs
- AttachmentCollection.cs
- LexicalChunk.cs
- AsnEncodedData.cs
- ActivityCodeDomReferenceService.cs
- TextLineBreak.cs
- odbcmetadatacolumnnames.cs
- UpdatePanelTriggerCollection.cs
- Binding.cs
- FieldDescriptor.cs
- FSWPathEditor.cs
- ClientSettings.cs
- GiveFeedbackEvent.cs
- BaseValidator.cs
- Model3DGroup.cs
- RightsManagementLicense.cs
- SpnegoTokenProvider.cs
- CrossAppDomainChannel.cs
- AutomationPropertyInfo.cs
- _AuthenticationState.cs
- DuplicateWaitObjectException.cs
- _emptywebproxy.cs
- ExternalDataExchangeClient.cs
- DynamicContractTypeBuilder.cs
- MetadataSection.cs
- BezierSegment.cs
- WeakReferenceKey.cs
- mediaeventshelper.cs
- ZoneLinkButton.cs
- Point3D.cs
- CorePropertiesFilter.cs
- ObjRef.cs
- CollectionMarkupSerializer.cs
- SignatureToken.cs
- OlePropertyStructs.cs
- FixedDocumentPaginator.cs
- XPathPatternBuilder.cs
- PerformanceCounterCategory.cs
- TransactedBatchingElement.cs
- Expressions.cs
- RuleSetDialog.Designer.cs
- CatchBlock.cs
- HtmlTable.cs
- PkcsUtils.cs
- Configuration.cs
- StateItem.cs
- ActiveDesignSurfaceEvent.cs
- WebScriptClientGenerator.cs
- StructuralComparisons.cs
- SqlRecordBuffer.cs
- TypeUnloadedException.cs
- KeyEvent.cs
- XmlElement.cs
- ADMembershipUser.cs
- HostExecutionContextManager.cs
- SortedDictionary.cs
- IERequestCache.cs
- HttpCachePolicyWrapper.cs
- CipherData.cs
- XXXInfos.cs
- TextEffectResolver.cs
- ResourceSet.cs
- LayoutExceptionEventArgs.cs
- ReversePositionQuery.cs
- ConfigurationStrings.cs
- FusionWrap.cs
- ImageFormatConverter.cs
- GuidelineCollection.cs
- DeobfuscatingStream.cs
- SqlDataSourceCommandEventArgs.cs
- InheritedPropertyChangedEventArgs.cs
- RC2CryptoServiceProvider.cs
- XmlNamespaceMapping.cs
- ExpressionsCollectionEditor.cs
- UIntPtr.cs
- NGCPageContentCollectionSerializerAsync.cs
- RuntimeHelpers.cs
- UInt64Storage.cs
- ToolStripDropDownItem.cs
- RowType.cs
- StorageConditionPropertyMapping.cs
- WriteTimeStream.cs
- NameValueSectionHandler.cs
- FrameworkRichTextComposition.cs
- RequestCachePolicy.cs
- ManifestBasedResourceGroveler.cs
- StringExpressionSet.cs
- FocusManager.cs
- DayRenderEvent.cs
- UserControl.cs
- ExpressionConverter.cs
- DataListItemCollection.cs
- HttpRequestTraceRecord.cs
- DataRecord.cs
- UserValidatedEventArgs.cs