Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activation / ServiceHostFactory.cs / 1305376 / ServiceHostFactory.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Activation { using System.Collections.ObjectModel; using System.Reflection; using System.Runtime.CompilerServices; [TypeForwardedFrom("System.ServiceModel, Version=3.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089")] public class ServiceHostFactory : ServiceHostFactoryBase { CollectionreferencedAssemblies; public ServiceHostFactory() { this.referencedAssemblies = new Collection (); } internal void AddAssemblyReference(string assemblyName) { this.referencedAssemblies.Add(assemblyName); } public override ServiceHostBase CreateServiceHost(string constructorString, Uri[] baseAddresses) { if (!AspNetEnvironment.Enabled) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.Hosting_ProcessNotExecutingUnderHostedContext("ServiceHostFactory.CreateServiceHost"))); } if (string.IsNullOrEmpty(constructorString)) { throw FxTrace.Exception.Argument("constructorString", SR.Hosting_ServiceTypeNotProvided); } Type type = Type.GetType(constructorString, false); if (type == null) { //config service activation scenario if (this.referencedAssemblies.Count == 0) { AspNetEnvironment.Current.EnsureAllReferencedAssemblyLoaded(); } foreach (string assemblyName in this.referencedAssemblies) { Assembly assembly = Assembly.Load(assemblyName); type = assembly.GetType(constructorString, false); if (type != null) { break; } } } if (type == null) { Assembly[] assemblies = AppDomain.CurrentDomain.GetAssemblies(); for (int i = 0; i < assemblies.Length; i++) { type = assemblies[i].GetType(constructorString, false); if (type != null) { break; } } } if (type == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.Hosting_ServiceTypeNotResolved(constructorString))); } return CreateServiceHost(type, baseAddresses); } protected virtual ServiceHost CreateServiceHost(Type serviceType, Uri[] baseAddresses) { return new ServiceHost(serviceType, baseAddresses); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
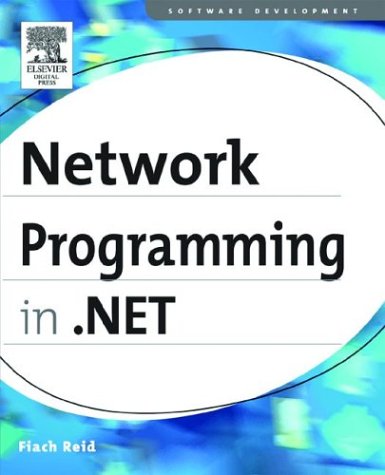
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextServicesLoader.cs
- GeneralTransform3DTo2DTo3D.cs
- AccessDataSource.cs
- GridPatternIdentifiers.cs
- ListViewInsertionMark.cs
- FactoryMaker.cs
- RowTypeElement.cs
- IPAddressCollection.cs
- ProtectedConfigurationProviderCollection.cs
- MiniMapControl.xaml.cs
- ReadWriteObjectLock.cs
- ErrorProvider.cs
- WebZoneDesigner.cs
- ObjectContextServiceProvider.cs
- SizeKeyFrameCollection.cs
- BufferedWebEventProvider.cs
- WsdlImporter.cs
- PinnedBufferMemoryStream.cs
- ServiceProviders.cs
- IdentitySection.cs
- WebScriptEnablingElement.cs
- unsafenativemethodsother.cs
- Control.cs
- FrameworkElementFactoryMarkupObject.cs
- RuleConditionDialog.Designer.cs
- SqlConnection.cs
- Separator.cs
- Point3DConverter.cs
- NestedContainer.cs
- TraceHandlerErrorFormatter.cs
- DSACryptoServiceProvider.cs
- DoubleStorage.cs
- SafeNativeMethods.cs
- QueryResponse.cs
- RequestCacheEntry.cs
- SchemaObjectWriter.cs
- DownloadProgressEventArgs.cs
- SHA384CryptoServiceProvider.cs
- QueryContinueDragEvent.cs
- XamlSerializerUtil.cs
- AspCompat.cs
- ResponseBodyWriter.cs
- CompModHelpers.cs
- ParameterToken.cs
- SortDescription.cs
- PageBreakRecord.cs
- CustomTypeDescriptor.cs
- ResolveNameEventArgs.cs
- OleDbConnectionFactory.cs
- ExpressionBuilder.cs
- RegexFCD.cs
- ISAPIRuntime.cs
- InternalControlCollection.cs
- CodeVariableReferenceExpression.cs
- TableLayoutSettingsTypeConverter.cs
- MetadataSource.cs
- XmlSerializerVersionAttribute.cs
- CatalogZone.cs
- PrimitiveCodeDomSerializer.cs
- GPPOINT.cs
- ProfileSettings.cs
- TypeConstant.cs
- ZipIOExtraFieldElement.cs
- EmptyStringExpandableObjectConverter.cs
- CalendarAutomationPeer.cs
- ExceptionValidationRule.cs
- LogicalExpr.cs
- XsltException.cs
- TreeBuilderBamlTranslator.cs
- Point.cs
- TimeEnumHelper.cs
- __ConsoleStream.cs
- LabelDesigner.cs
- DataGridViewColumnEventArgs.cs
- ComponentChangedEvent.cs
- VariableQuery.cs
- OpCopier.cs
- GAC.cs
- Messages.cs
- StopStoryboard.cs
- handlecollector.cs
- SubtreeProcessor.cs
- DataGridViewCellEventArgs.cs
- XmlChildEnumerator.cs
- DispatcherOperation.cs
- ConcurrentDictionary.cs
- MulticastIPAddressInformationCollection.cs
- TypeSchema.cs
- StringBlob.cs
- Matrix.cs
- DataGridViewSelectedRowCollection.cs
- PrivilegedConfigurationManager.cs
- ThreadAttributes.cs
- ExpressionUtilities.cs
- RandomNumberGenerator.cs
- Composition.cs
- xmlglyphRunInfo.cs
- CollectionChangeEventArgs.cs
- WebPartConnectionCollection.cs
- RSAPKCS1SignatureDeformatter.cs