Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Map / ViewGeneration / Structures / TypeConstant.cs / 1305376 / TypeConstant.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Structures { // A constant for storing type values, e.g., a type constant is used to // denotes (say) a Person type, Address type, etc. It essentially // encapsulates a CDM Type internal class TypeConstant : Constant { #region Constructor // effects: Creates a type constant corresponding to "type" internal TypeConstant(EdmType type) { m_cdmType = type; } #endregion #region Fields // The CDM type denoted by this type constant private EdmType m_cdmType; #endregion #region Properties // effects: Returns the CDM type corresponding to this internal EdmType CdmType { get { return m_cdmType; } } #endregion #region Methods // effects: Given a projectedSlot that contributes to outputMember in // the output extent view, generates a constructor expression for // outputMember's type, i.e, an expression of the form "Type(....)" // If refscope is non-null, instead of constructing an Entity or // Complex type, constructs a reference internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Gather the fields for a constructor or a Ref expression Listfields = new List (); EntitySet refScope = outputMember.GetScopeOfRelationEnd(); EntityType refEntityType = null; if (refScope != null) { // Construct a scoped reference: CreateRef(CPerson1, row(pid1, pid2)) EntityType entityType = refScope.ElementType; refEntityType = (EntityType)(((RefType)outputMember.EdmType).ElementType); builder.Append("CreateRef("); CqlWriter.AppendEscapedQualifiedName(builder, refScope.EntityContainer.Name, refScope.Name); builder.Append(", row("); foreach (EdmMember keyMember in entityType.KeyMembers) { // Given the member, we need its aliased name MemberPath memberPath = new MemberPath(outputMember, keyMember); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } } else { // Construct an entity/complex/Association type in the Members order // for fields: CPerson(CPerson1_Pid, CPerson1_Name) // It better be a structural type StructuralType structuralType = (StructuralType)m_cdmType; foreach (EdmMember member in Helper.GetAllStructuralMembers(structuralType)) { // Given the member, we need its aliased name: CPerson1_Pid MemberPath memberPath = new MemberPath(outputMember, member); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } // Constructor call for the constructedType CqlWriter.AppendEscapedTypeName(builder, m_cdmType); builder.Append('('); } StringUtil.ToSeparatedString(builder, fields, ", ", null); builder.Append(')'); if (refScope != null) { Debug.Assert(refEntityType != null); builder.Append(","); CqlWriter.AppendEscapedTypeName(builder, refEntityType); builder.Append(')'); } return builder; } #endregion #region Public Override Methods for object and String methods protected override bool IsEqualTo(Constant right) { TypeConstant rightTypeConstant = right as TypeConstant; if (rightTypeConstant == null) { return false; } return m_cdmType == rightTypeConstant.m_cdmType; } protected override int GetHash() { if (m_cdmType == null) { // null type constant return 0; } else { return m_cdmType.GetHashCode(); } } private void InternalToString(StringBuilder builder, bool isInvariant) { if (m_cdmType == null) { // NULL type constant builder.Append(isInvariant ? "NULL" : System.Data.Entity.Strings.ViewGen_Null); } else { builder.Append(m_cdmType.Name); } } internal override string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System.Text; using System.Collections.Generic; using System.Data.Mapping.ViewGeneration.CqlGeneration; using System.Data.Common; using System.Data.Common.Utils; using System.Data.Metadata.Edm; using System.Diagnostics; namespace System.Data.Mapping.ViewGeneration.Structures { // A constant for storing type values, e.g., a type constant is used to // denotes (say) a Person type, Address type, etc. It essentially // encapsulates a CDM Type internal class TypeConstant : Constant { #region Constructor // effects: Creates a type constant corresponding to "type" internal TypeConstant(EdmType type) { m_cdmType = type; } #endregion #region Fields // The CDM type denoted by this type constant private EdmType m_cdmType; #endregion #region Properties // effects: Returns the CDM type corresponding to this internal EdmType CdmType { get { return m_cdmType; } } #endregion #region Methods // effects: Given a projectedSlot that contributes to outputMember in // the output extent view, generates a constructor expression for // outputMember's type, i.e, an expression of the form "Type(....)" // If refscope is non-null, instead of constructing an Entity or // Complex type, constructs a reference internal override StringBuilder AsCql(StringBuilder builder, MemberPath outputMember, string blockAlias) { // Gather the fields for a constructor or a Ref expression Listfields = new List (); EntitySet refScope = outputMember.GetScopeOfRelationEnd(); EntityType refEntityType = null; if (refScope != null) { // Construct a scoped reference: CreateRef(CPerson1, row(pid1, pid2)) EntityType entityType = refScope.ElementType; refEntityType = (EntityType)(((RefType)outputMember.EdmType).ElementType); builder.Append("CreateRef("); CqlWriter.AppendEscapedQualifiedName(builder, refScope.EntityContainer.Name, refScope.Name); builder.Append(", row("); foreach (EdmMember keyMember in entityType.KeyMembers) { // Given the member, we need its aliased name MemberPath memberPath = new MemberPath(outputMember, keyMember); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } } else { // Construct an entity/complex/Association type in the Members order // for fields: CPerson(CPerson1_Pid, CPerson1_Name) // It better be a structural type StructuralType structuralType = (StructuralType)m_cdmType; foreach (EdmMember member in Helper.GetAllStructuralMembers(structuralType)) { // Given the member, we need its aliased name: CPerson1_Pid MemberPath memberPath = new MemberPath(outputMember, member); string fullFieldAlias = CqlWriter.GetQualifiedName(blockAlias, memberPath.CqlFieldAlias); fields.Add(fullFieldAlias); } // Constructor call for the constructedType CqlWriter.AppendEscapedTypeName(builder, m_cdmType); builder.Append('('); } StringUtil.ToSeparatedString(builder, fields, ", ", null); builder.Append(')'); if (refScope != null) { Debug.Assert(refEntityType != null); builder.Append(","); CqlWriter.AppendEscapedTypeName(builder, refEntityType); builder.Append(')'); } return builder; } #endregion #region Public Override Methods for object and String methods protected override bool IsEqualTo(Constant right) { TypeConstant rightTypeConstant = right as TypeConstant; if (rightTypeConstant == null) { return false; } return m_cdmType == rightTypeConstant.m_cdmType; } protected override int GetHash() { if (m_cdmType == null) { // null type constant return 0; } else { return m_cdmType.GetHashCode(); } } private void InternalToString(StringBuilder builder, bool isInvariant) { if (m_cdmType == null) { // NULL type constant builder.Append(isInvariant ? "NULL" : System.Data.Entity.Strings.ViewGen_Null); } else { builder.Append(m_cdmType.Name); } } internal override string ToUserString() { StringBuilder builder = new StringBuilder(); InternalToString(builder, false); return builder.ToString(); } internal override void ToCompactString(StringBuilder builder) { InternalToString(builder, true); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
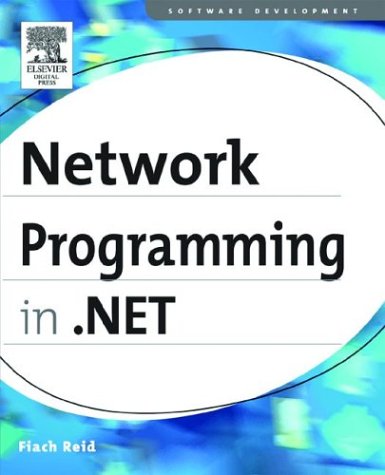
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MailDefinition.cs
- NullableBoolConverter.cs
- TextMetrics.cs
- AVElementHelper.cs
- HttpResponseHeader.cs
- WindowShowOrOpenTracker.cs
- PeerNodeTraceRecord.cs
- ClientConfigPaths.cs
- IPHostEntry.cs
- _NetworkingPerfCounters.cs
- ApplicationManager.cs
- ListBoxItemAutomationPeer.cs
- RegularExpressionValidator.cs
- CommonProperties.cs
- Empty.cs
- SessionStateModule.cs
- FileChangesMonitor.cs
- MsmqDiagnostics.cs
- TypeSystemHelpers.cs
- SecurityPolicySection.cs
- DataGridTextBoxColumn.cs
- CharacterMetricsDictionary.cs
- Rules.cs
- QfeChecker.cs
- ObjectListItem.cs
- CustomAttributeSerializer.cs
- WebFormsRootDesigner.cs
- StrictModeSecurityHeaderElementInferenceEngine.cs
- DataSourceCacheDurationConverter.cs
- VirtualStackFrame.cs
- ZipIOLocalFileDataDescriptor.cs
- EncoderNLS.cs
- DateTimeValueSerializerContext.cs
- SaveWorkflowCommand.cs
- SQLSingleStorage.cs
- Models.cs
- LogStore.cs
- DialogResultConverter.cs
- TemplateControlParser.cs
- ConstraintManager.cs
- ImpersonationContext.cs
- InputLangChangeEvent.cs
- BinaryReader.cs
- securitycriticaldataformultiplegetandset.cs
- ProcessThread.cs
- ModelUIElement3D.cs
- RestClientProxyHandler.cs
- TemplateKey.cs
- GeneratedContractType.cs
- SelectionRange.cs
- WebPartZoneBase.cs
- ComboBoxAutomationPeer.cs
- QuaternionConverter.cs
- ToolStripComboBox.cs
- WsdlWriter.cs
- DataTransferEventArgs.cs
- HttpRuntime.cs
- VerificationAttribute.cs
- MemberExpression.cs
- UnsafeNativeMethods.cs
- SingleStorage.cs
- DataList.cs
- Help.cs
- ModelChangedEventArgsImpl.cs
- MonthChangedEventArgs.cs
- Visitors.cs
- WorkItem.cs
- SlotInfo.cs
- Stopwatch.cs
- ExceptionUtil.cs
- QilScopedVisitor.cs
- SchemaCollectionCompiler.cs
- ContainerUIElement3D.cs
- DataGridViewTopRowAccessibleObject.cs
- Rotation3DAnimationBase.cs
- DataGridViewCellLinkedList.cs
- TrackingServices.cs
- InteropAutomationProvider.cs
- DebuggerAttributes.cs
- LoginName.cs
- SerializationIncompleteException.cs
- AssemblyBuilder.cs
- TypeNameConverter.cs
- TextTreeObjectNode.cs
- DataBoundLiteralControl.cs
- WebBrowserDocumentCompletedEventHandler.cs
- XmlCharCheckingWriter.cs
- panel.cs
- DataControlHelper.cs
- IDQuery.cs
- IISUnsafeMethods.cs
- ProxyGenerator.cs
- DataGridViewRowPostPaintEventArgs.cs
- RepeaterItemEventArgs.cs
- CustomPopupPlacement.cs
- FormViewDeletedEventArgs.cs
- CacheDependency.cs
- RecognizerBase.cs
- SingleConverter.cs
- MetafileHeader.cs