Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / MS / Internal / AppModel / ProgressPage.cs / 1 / ProgressPage.cs
//+------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: // Deployment progress page. This is primarily a proxy to the native progress page, which supersedes // the managed one from up to v3.5. See Host\DLL\ProgressPage.hxx for details. // // History: // 2007/12/xx ChangoV Created // //----------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Interop; using System.Windows.Threading; using System.Security; namespace MS.Internal.AppModel { ////// Critical due to SUC. /// Even if a partilar method is considered safe, applying [SecurityTreatAsSafe] to it here won't help /// much, because the transparency model still requires SUC-d methods to be called only from /// SecurityCritical ones. /// [ComImport, Guid("1f681651-1024-4798-af36-119bbe5e5665")] [InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] [SecurityCritical, SuppressUnmanagedCodeSecurity] interface INativeProgressPage { void Show(); void Hide(); void ShowProgressMessage(string message); void SetApplicationName(string appName); void SetPublisherName(string publisherName); void OnDownloadProgress(ulong bytesDownloaded, ulong bytesTotal); }; ////// IProgressPage is public. It was introduced for the Media Center integration, which is now considered /// deprecated, but we have to support it at least for as long as we keep doing in-place upgrades. /// interface IProgressPage2 : IProgressPage { void ShowProgressMessage(string message); }; class NativeProgressPageProxy : IProgressPage2 { internal NativeProgressPageProxy(INativeProgressPage npp) { _npp = npp; } ////// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: No concern about "spoofing" progress messages. A web site could just render an HTML /// page that looks like our progress page. /// [SecurityCritical, SecurityTreatAsSafe] public void ShowProgressMessage(string message) { _npp.ShowProgressMessage(message); } public Uri DeploymentPath { set { } get { throw new System.NotImplementedException(); } } /// /// The native progress page sends a stop/cancel request to its host object, which then calls /// IBrowserHostServices.ExecCommand(OLECMDID_STOP). /// public DispatcherOperationCallback StopCallback { set { } get { throw new System.NotImplementedException(); } } ////// The native progress page sends a Refresh request to its host object, which then calls /// IBrowserHostServices.ExecCommand(OLECMDID_REFRESH). /// public System.Windows.Threading.DispatcherOperationCallback RefreshCallback { set { } get { return null; } } ////// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: 1) The application name is coming from the manifest, so it could be anything. /// This means the input doesn't need to be trusted. /// 2) Setting arbitrary application/publisher can be considered spoofing, but a malicious website /// could fake the whole progress page and still achieve the same. /// public string ApplicationName { [SecurityCritical, SecurityTreatAsSafe] set { _npp.SetApplicationName(value); } get { throw new System.NotImplementedException(); } } ////// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: 1) The publisher name is coming from the manifest, so it could be anything. /// This means the input doesn't need to be trusted. /// 2) Setting arbitrary application/publisher can be considered spoofing, but a malicious website /// could fake the whole progress page and still achieve the same. /// public string PublisherName { [SecurityCritical, SecurityTreatAsSafe] set { _npp.SetPublisherName(value); } get { throw new System.NotImplementedException(); } } /// /// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: Sending even arbitrary progress updates not considered harmful. /// [SecurityCritical, SecurityTreatAsSafe] public void UpdateProgress(long bytesDownloaded, long bytesTotal) { _npp.OnDownloadProgress((ulong)bytesDownloaded, (ulong)bytesTotal); } INativeProgressPage _npp; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //+------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: // Deployment progress page. This is primarily a proxy to the native progress page, which supersedes // the managed one from up to v3.5. See Host\DLL\ProgressPage.hxx for details. // // History: // 2007/12/xx ChangoV Created // //----------------------------------------------------------------------- using System; using System.Runtime.InteropServices; using System.Windows.Interop; using System.Windows.Threading; using System.Security; namespace MS.Internal.AppModel { /// /// Critical due to SUC. /// Even if a partilar method is considered safe, applying [SecurityTreatAsSafe] to it here won't help /// much, because the transparency model still requires SUC-d methods to be called only from /// SecurityCritical ones. /// [ComImport, Guid("1f681651-1024-4798-af36-119bbe5e5665")] [InterfaceType(ComInterfaceType.InterfaceIsIUnknown)] [SecurityCritical, SuppressUnmanagedCodeSecurity] interface INativeProgressPage { void Show(); void Hide(); void ShowProgressMessage(string message); void SetApplicationName(string appName); void SetPublisherName(string publisherName); void OnDownloadProgress(ulong bytesDownloaded, ulong bytesTotal); }; ////// IProgressPage is public. It was introduced for the Media Center integration, which is now considered /// deprecated, but we have to support it at least for as long as we keep doing in-place upgrades. /// interface IProgressPage2 : IProgressPage { void ShowProgressMessage(string message); }; class NativeProgressPageProxy : IProgressPage2 { internal NativeProgressPageProxy(INativeProgressPage npp) { _npp = npp; } ////// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: No concern about "spoofing" progress messages. A web site could just render an HTML /// page that looks like our progress page. /// [SecurityCritical, SecurityTreatAsSafe] public void ShowProgressMessage(string message) { _npp.ShowProgressMessage(message); } public Uri DeploymentPath { set { } get { throw new System.NotImplementedException(); } } /// /// The native progress page sends a stop/cancel request to its host object, which then calls /// IBrowserHostServices.ExecCommand(OLECMDID_STOP). /// public DispatcherOperationCallback StopCallback { set { } get { throw new System.NotImplementedException(); } } ////// The native progress page sends a Refresh request to its host object, which then calls /// IBrowserHostServices.ExecCommand(OLECMDID_REFRESH). /// public System.Windows.Threading.DispatcherOperationCallback RefreshCallback { set { } get { return null; } } ////// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: 1) The application name is coming from the manifest, so it could be anything. /// This means the input doesn't need to be trusted. /// 2) Setting arbitrary application/publisher can be considered spoofing, but a malicious website /// could fake the whole progress page and still achieve the same. /// public string ApplicationName { [SecurityCritical, SecurityTreatAsSafe] set { _npp.SetApplicationName(value); } get { throw new System.NotImplementedException(); } } ////// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: 1) The publisher name is coming from the manifest, so it could be anything. /// This means the input doesn't need to be trusted. /// 2) Setting arbitrary application/publisher can be considered spoofing, but a malicious website /// could fake the whole progress page and still achieve the same. /// public string PublisherName { [SecurityCritical, SecurityTreatAsSafe] set { _npp.SetPublisherName(value); } get { throw new System.NotImplementedException(); } } /// /// Critical: Calls a SUC'd COM interface method. /// TreatAsSafe: Sending even arbitrary progress updates not considered harmful. /// [SecurityCritical, SecurityTreatAsSafe] public void UpdateProgress(long bytesDownloaded, long bytesTotal) { _npp.OnDownloadProgress((ulong)bytesDownloaded, (ulong)bytesTotal); } INativeProgressPage _npp; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
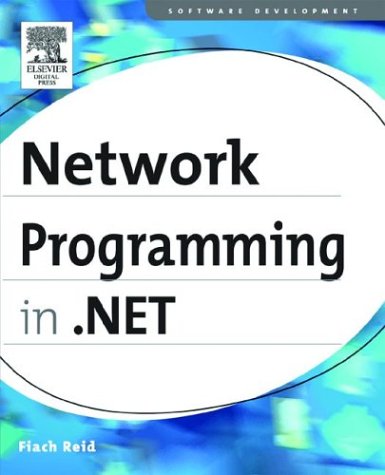
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseGestureConverter.cs
- SamlAuthorizationDecisionStatement.cs
- UserControlFileEditor.cs
- PolyQuadraticBezierSegment.cs
- ClientEventManager.cs
- XmlDigitalSignatureProcessor.cs
- SystemUnicastIPAddressInformation.cs
- CompilerInfo.cs
- HScrollBar.cs
- WsdlBuildProvider.cs
- Nodes.cs
- DataTablePropertyDescriptor.cs
- Comparer.cs
- XmlILCommand.cs
- ExtensibleClassFactory.cs
- MessageQueuePermissionEntryCollection.cs
- CodeFieldReferenceExpression.cs
- Identity.cs
- DesignerGenericWebPart.cs
- sapiproxy.cs
- TabletCollection.cs
- ChtmlTextWriter.cs
- HttpListener.cs
- ValidatingReaderNodeData.cs
- DescendantQuery.cs
- TheQuery.cs
- SingleObjectCollection.cs
- DownloadProgressEventArgs.cs
- BidOverLoads.cs
- MsmqTransportSecurityElement.cs
- VirtualDirectoryMapping.cs
- SchemaElementDecl.cs
- DataSourceXmlSubItemAttribute.cs
- Menu.cs
- clipboard.cs
- RuntimeConfig.cs
- AlternateView.cs
- xmlglyphRunInfo.cs
- Permission.cs
- EasingQuaternionKeyFrame.cs
- XPathSelectionIterator.cs
- DoWorkEventArgs.cs
- SdlChannelSink.cs
- FontFaceLayoutInfo.cs
- ListViewHitTestInfo.cs
- PolicyDesigner.cs
- SendingRequestEventArgs.cs
- Int32EqualityComparer.cs
- XPathException.cs
- SelectionRangeConverter.cs
- EdmToObjectNamespaceMap.cs
- NoPersistHandle.cs
- RequestSecurityToken.cs
- SqlDataSourceConnectionPanel.cs
- TraceEventCache.cs
- ConvertersCollection.cs
- ObjectAssociationEndMapping.cs
- SchemaCollectionCompiler.cs
- SliderAutomationPeer.cs
- EncodingTable.cs
- WebBrowser.cs
- GenericAuthenticationEventArgs.cs
- exports.cs
- WmlSelectionListAdapter.cs
- filewebresponse.cs
- MetabaseServerConfig.cs
- AttachedAnnotation.cs
- FrameworkRichTextComposition.cs
- TemplateLookupAction.cs
- AudioLevelUpdatedEventArgs.cs
- SQLBinaryStorage.cs
- Variant.cs
- ProtocolProfile.cs
- MouseEvent.cs
- SqlMethodCallConverter.cs
- ObjectParameter.cs
- SQLInt16Storage.cs
- SerializationFieldInfo.cs
- TripleDESCryptoServiceProvider.cs
- SignatureDescription.cs
- XmlSchemaAppInfo.cs
- Misc.cs
- TextParaLineResult.cs
- RequestCacheEntry.cs
- CustomErrorsSection.cs
- Lazy.cs
- HtmlToClrEventProxy.cs
- PagerSettings.cs
- LayoutUtils.cs
- ChannelManagerService.cs
- DescendantBaseQuery.cs
- BmpBitmapEncoder.cs
- StatusBarItem.cs
- XmlSchemaSimpleContentRestriction.cs
- Slider.cs
- Aes.cs
- CrossAppDomainChannel.cs
- SQLUtility.cs
- DelegateSerializationHolder.cs
- XmlEnumAttribute.cs