Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / Internal / Synthesis / TTSVoice.cs / 1 / TTSVoice.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // TTS voice entry from the object token. The same TTS engine dll // can be used for multiple voice. So both an instance created and // the object token used must be preserved. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Speech.Synthesis; using System.Collections.Generic; using System.Speech.AudioFormat; using System.Speech.Internal.ObjectTokens; using System.Runtime.InteropServices; namespace System.Speech.Internal.Synthesis { internal class TTSVoice { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal TTSVoice (ITtsEngineProxy engine, VoiceInfo voiceId) { _engine = engine; _voiceId = voiceId; } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods ////// Tests whether two objects are equivalent /// public override bool Equals (object obj) { TTSVoice voice = obj as TTSVoice; return voice != null && (_voiceId.Equals (voice.VoiceInfo)); } ////// Overrides Object.GetHashCode() /// public override int GetHashCode () { return _voiceId.GetHashCode (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods internal void UpdateLexicons (Listlexicons) { // Remove the lexicons that are defined in this voice but are not in the list for (int i = _lexicons.Count - 1; i >= 0; i--) { LexiconEntry entry = _lexicons [i]; if (!lexicons.Contains (entry)) { // Remove the entry first, just in case the RemoveLexicon throws _lexicons.RemoveAt (i); TtsEngine.RemoveLexicon (entry._uri); } } // Addd the lexicons that are defined in this voice but are not in the list foreach (LexiconEntry entry in lexicons) { if (!_lexicons.Contains (entry)) { // Remove the entry first, just in case the RemoveLexicon throws TtsEngine.AddLexicon (entry._uri, entry._mediaType); _lexicons.Add (entry); } } } internal byte [] WaveFormat (byte [] targetWaveFormat) { #if !SPEECHSERVER // Get the Wave header if it has not been set by the user if (targetWaveFormat == null && _waveFormat == null) { // The registry values contains a default rate if (VoiceInfo.SupportedAudioFormats.Count > 0) { // Create the array of bytes containing the format targetWaveFormat = VoiceInfo.SupportedAudioFormats [0].WaveFormat; } } #endif // No input specified and we already got the default if (targetWaveFormat == null && _waveFormat != null) { return _waveFormat; } // New waveFormat provided? if (_waveFormat == null || !Array.Equals (targetWaveFormat, _waveFormat)) { IntPtr waveFormat = IntPtr.Zero; GCHandle targetFormat = new GCHandle (); if (targetWaveFormat != null) { targetFormat = GCHandle.Alloc (targetWaveFormat, GCHandleType.Pinned); } try { waveFormat = _engine.GetOutputFormat (targetWaveFormat != null ? targetFormat.AddrOfPinnedObject () : IntPtr.Zero); } finally { if (targetWaveFormat != null) { targetFormat.Free (); } } if (waveFormat != IntPtr.Zero) { _waveFormat = WAVEFORMATEX.ToBytes (waveFormat); // Free the buffer Marshal.FreeCoTaskMem (waveFormat); } else { _waveFormat = WAVEFORMATEX.Default.ToBytes (); } } return _waveFormat; } #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties internal ITtsEngineProxy TtsEngine { get { return _engine; } } internal VoiceInfo VoiceInfo { get { return _voiceId; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields private ITtsEngineProxy _engine; private VoiceInfo _voiceId; private List _lexicons = new List (); private byte [] _waveFormat; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // // // TTS voice entry from the object token. The same TTS engine dll // can be used for multiple voice. So both an instance created and // the object token used must be preserved. // // History: // 2/1/2005 jeanfp Created from the Sapi Managed code //----------------------------------------------------------------- using System; using System.Speech.Synthesis; using System.Collections.Generic; using System.Speech.AudioFormat; using System.Speech.Internal.ObjectTokens; using System.Runtime.InteropServices; namespace System.Speech.Internal.Synthesis { internal class TTSVoice { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors internal TTSVoice (ITtsEngineProxy engine, VoiceInfo voiceId) { _engine = engine; _voiceId = voiceId; } #endregion //******************************************************************** // // Public Methods // //******************************************************************* #region public Methods ////// Tests whether two objects are equivalent /// public override bool Equals (object obj) { TTSVoice voice = obj as TTSVoice; return voice != null && (_voiceId.Equals (voice.VoiceInfo)); } ////// Overrides Object.GetHashCode() /// public override int GetHashCode () { return _voiceId.GetHashCode (); } #endregion //******************************************************************** // // Internal Methods // //******************************************************************** #region Internal Methods internal void UpdateLexicons (Listlexicons) { // Remove the lexicons that are defined in this voice but are not in the list for (int i = _lexicons.Count - 1; i >= 0; i--) { LexiconEntry entry = _lexicons [i]; if (!lexicons.Contains (entry)) { // Remove the entry first, just in case the RemoveLexicon throws _lexicons.RemoveAt (i); TtsEngine.RemoveLexicon (entry._uri); } } // Addd the lexicons that are defined in this voice but are not in the list foreach (LexiconEntry entry in lexicons) { if (!_lexicons.Contains (entry)) { // Remove the entry first, just in case the RemoveLexicon throws TtsEngine.AddLexicon (entry._uri, entry._mediaType); _lexicons.Add (entry); } } } internal byte [] WaveFormat (byte [] targetWaveFormat) { #if !SPEECHSERVER // Get the Wave header if it has not been set by the user if (targetWaveFormat == null && _waveFormat == null) { // The registry values contains a default rate if (VoiceInfo.SupportedAudioFormats.Count > 0) { // Create the array of bytes containing the format targetWaveFormat = VoiceInfo.SupportedAudioFormats [0].WaveFormat; } } #endif // No input specified and we already got the default if (targetWaveFormat == null && _waveFormat != null) { return _waveFormat; } // New waveFormat provided? if (_waveFormat == null || !Array.Equals (targetWaveFormat, _waveFormat)) { IntPtr waveFormat = IntPtr.Zero; GCHandle targetFormat = new GCHandle (); if (targetWaveFormat != null) { targetFormat = GCHandle.Alloc (targetWaveFormat, GCHandleType.Pinned); } try { waveFormat = _engine.GetOutputFormat (targetWaveFormat != null ? targetFormat.AddrOfPinnedObject () : IntPtr.Zero); } finally { if (targetWaveFormat != null) { targetFormat.Free (); } } if (waveFormat != IntPtr.Zero) { _waveFormat = WAVEFORMATEX.ToBytes (waveFormat); // Free the buffer Marshal.FreeCoTaskMem (waveFormat); } else { _waveFormat = WAVEFORMATEX.Default.ToBytes (); } } return _waveFormat; } #endregion //******************************************************************* // // Internal Properties // //******************************************************************** #region Internal Properties internal ITtsEngineProxy TtsEngine { get { return _engine; } } internal VoiceInfo VoiceInfo { get { return _voiceId; } } #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region private Fields private ITtsEngineProxy _engine; private VoiceInfo _voiceId; private List _lexicons = new List (); private byte [] _waveFormat; #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
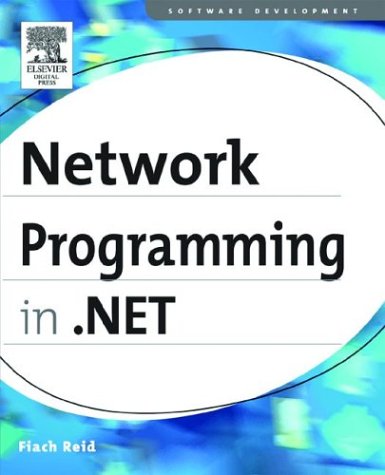
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CheckBoxBaseAdapter.cs
- HierarchicalDataSourceControl.cs
- MsmqInputChannel.cs
- ClearTypeHintValidation.cs
- Rectangle.cs
- Main.cs
- SoapTypeAttribute.cs
- parserscommon.cs
- DataProtection.cs
- TreeNodeCollection.cs
- HtmlInputFile.cs
- StrokeCollectionDefaultValueFactory.cs
- MenuCommandsChangedEventArgs.cs
- SignatureGenerator.cs
- DialogBaseForm.cs
- TypeValidationEventArgs.cs
- PageParserFilter.cs
- DataContractSerializer.cs
- TypeNameParser.cs
- FunctionDescription.cs
- MasterPage.cs
- FtpRequestCacheValidator.cs
- InputScopeAttribute.cs
- LassoHelper.cs
- TagNameToTypeMapper.cs
- StrokeCollection2.cs
- RemotingAttributes.cs
- PauseStoryboard.cs
- SecurityCriticalDataForSet.cs
- BrowserCapabilitiesFactory.cs
- Cursor.cs
- GeneralTransform3D.cs
- WorkflowServiceInstance.cs
- PixelFormatConverter.cs
- TableDetailsRow.cs
- FullTextBreakpoint.cs
- DESCryptoServiceProvider.cs
- TdsParserHelperClasses.cs
- AssemblyUtil.cs
- HttpHeaderCollection.cs
- ObjectList.cs
- ListViewItemMouseHoverEvent.cs
- LayoutInformation.cs
- SubpageParaClient.cs
- JsonReaderWriterFactory.cs
- DataSetUtil.cs
- MemberInfoSerializationHolder.cs
- TextEffectCollection.cs
- OrderPreservingSpoolingTask.cs
- ImmutableCollection.cs
- RoutingConfiguration.cs
- TemplateColumn.cs
- XPathSelfQuery.cs
- ReturnEventArgs.cs
- ExtendedProtectionPolicyTypeConverter.cs
- DataFormat.cs
- GeometryHitTestResult.cs
- AncestorChangedEventArgs.cs
- TrackingServices.cs
- adornercollection.cs
- XmlArrayAttribute.cs
- SessionParameter.cs
- FocusManager.cs
- JsonWriter.cs
- HttpMethodConstraint.cs
- StringUtil.cs
- FormsAuthenticationEventArgs.cs
- TableHeaderCell.cs
- HttpModule.cs
- WebBrowserNavigatingEventHandler.cs
- AnnotationDocumentPaginator.cs
- EntityDesignerBuildProvider.cs
- TableStyle.cs
- WebScriptMetadataMessage.cs
- ValidatorCollection.cs
- AttachInfo.cs
- ControlPersister.cs
- CodeChecksumPragma.cs
- AudioFormatConverter.cs
- RoleGroupCollection.cs
- IdnElement.cs
- ServiceRoute.cs
- PanelContainerDesigner.cs
- EnumerationRangeValidationUtil.cs
- AutomationEventArgs.cs
- HostProtectionPermission.cs
- Math.cs
- SizeAnimationBase.cs
- ToolBarPanel.cs
- ErrorRuntimeConfig.cs
- HtmlInputCheckBox.cs
- HttpCachePolicyElement.cs
- EntitySetBaseCollection.cs
- ColumnReorderedEventArgs.cs
- IntranetCredentialPolicy.cs
- EditCommandColumn.cs
- ClientTargetCollection.cs
- HttpCapabilitiesEvaluator.cs
- HMACSHA384.cs
- NavigationExpr.cs