Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Actions / CallInfo.cs / 1305376 / CallInfo.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Dynamic.Utils; using System.Linq.Expressions; #if SILVERLIGHT using System.Core; #endif //SILVERLIGHT namespace System.Dynamic { ////// Describes arguments in the dynamic binding process. /// ////// ArgumentCount - all inclusive number of arguments. /// ArgumentNames - names for those arguments that are named. /// /// Argument names match to the argument values in left to right order /// and last name corresponds to the last argument. /// /// Example: /// Foo(arg1, arg2, arg3, name1 = arg4, name2 = arg5, name3 = arg6) /// /// will correspond to: /// ArgumentCount: 6 /// ArgumentNames: {"name1", "name2", "name3"} /// public sealed class CallInfo { private readonly int _argCount; private readonly ReadOnlyCollection_argNames; /// /// Creates a new PositionalArgumentInfo. /// /// The number of arguments. /// The argument names. ///The new CallInfo public CallInfo(int argCount, params string[] argNames) : this(argCount, (IEnumerable)argNames) { } /// /// Creates a new CallInfo that represents arguments in the dynamic binding process. /// /// The number of arguments. /// The argument names. ///The new CallInfo public CallInfo(int argCount, IEnumerableargNames) { ContractUtils.RequiresNotNull(argNames, "argNames"); var argNameCol = argNames.ToReadOnly(); if (argCount < argNameCol.Count) throw Error.ArgCntMustBeGreaterThanNameCnt(); ContractUtils.RequiresNotNullItems(argNameCol, "argNames"); _argCount = argCount; _argNames = argNameCol; } /// /// The number of arguments. /// public int ArgumentCount { get { return _argCount; } } ////// The argument names. /// public ReadOnlyCollectionArgumentNames { get { return _argNames; } } /// /// Serves as a hash function for the current CallInfo. /// ///A hash code for the current CallInfo. public override int GetHashCode() { return _argCount ^ _argNames.ListHashCode(); } ////// Determines whether the specified CallInfo instance is considered equal to the current. /// /// The instance of CallInfo to compare with the current instance. ///true if the specified instance is equal to the current one otherwise, false. public override bool Equals(object obj) { var other = obj as CallInfo; return _argCount == other._argCount && _argNames.ListEquals(other._argNames); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Collections.Generic; using System.Collections.ObjectModel; using System.Dynamic.Utils; using System.Linq.Expressions; #if SILVERLIGHT using System.Core; #endif //SILVERLIGHT namespace System.Dynamic { ////// Describes arguments in the dynamic binding process. /// ////// ArgumentCount - all inclusive number of arguments. /// ArgumentNames - names for those arguments that are named. /// /// Argument names match to the argument values in left to right order /// and last name corresponds to the last argument. /// /// Example: /// Foo(arg1, arg2, arg3, name1 = arg4, name2 = arg5, name3 = arg6) /// /// will correspond to: /// ArgumentCount: 6 /// ArgumentNames: {"name1", "name2", "name3"} /// public sealed class CallInfo { private readonly int _argCount; private readonly ReadOnlyCollection_argNames; /// /// Creates a new PositionalArgumentInfo. /// /// The number of arguments. /// The argument names. ///The new CallInfo public CallInfo(int argCount, params string[] argNames) : this(argCount, (IEnumerable)argNames) { } /// /// Creates a new CallInfo that represents arguments in the dynamic binding process. /// /// The number of arguments. /// The argument names. ///The new CallInfo public CallInfo(int argCount, IEnumerableargNames) { ContractUtils.RequiresNotNull(argNames, "argNames"); var argNameCol = argNames.ToReadOnly(); if (argCount < argNameCol.Count) throw Error.ArgCntMustBeGreaterThanNameCnt(); ContractUtils.RequiresNotNullItems(argNameCol, "argNames"); _argCount = argCount; _argNames = argNameCol; } /// /// The number of arguments. /// public int ArgumentCount { get { return _argCount; } } ////// The argument names. /// public ReadOnlyCollectionArgumentNames { get { return _argNames; } } /// /// Serves as a hash function for the current CallInfo. /// ///A hash code for the current CallInfo. public override int GetHashCode() { return _argCount ^ _argNames.ListHashCode(); } ////// Determines whether the specified CallInfo instance is considered equal to the current. /// /// The instance of CallInfo to compare with the current instance. ///true if the specified instance is equal to the current one otherwise, false. public override bool Equals(object obj) { var other = obj as CallInfo; return _argCount == other._argCount && _argNames.ListEquals(other._argNames); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
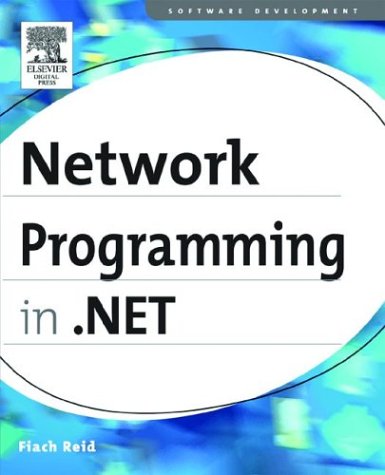
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PanelStyle.cs
- OSEnvironmentHelper.cs
- SqlCacheDependency.cs
- Image.cs
- StoreItemCollection.Loader.cs
- LocalsItemDescription.cs
- HitTestResult.cs
- ModelUIElement3D.cs
- WebPartAddingEventArgs.cs
- DataServiceCollectionOfT.cs
- SynchronizedCollection.cs
- ConsoleKeyInfo.cs
- FileRecordSequenceHelper.cs
- HttpDictionary.cs
- Misc.cs
- SamlDoNotCacheCondition.cs
- GregorianCalendarHelper.cs
- XPathParser.cs
- TypeGenericEnumerableViewSchema.cs
- DllNotFoundException.cs
- StylusPointProperties.cs
- PropertyDescriptorComparer.cs
- UserControl.cs
- LocalValueEnumerator.cs
- AssociationSet.cs
- FlagsAttribute.cs
- SmiMetaData.cs
- Grid.cs
- NullableFloatSumAggregationOperator.cs
- IconHelper.cs
- XamlWriter.cs
- DesignerSerializerAttribute.cs
- _IPv6Address.cs
- DesignSurfaceManager.cs
- ServiceParser.cs
- ExtensionFile.cs
- unsafeIndexingFilterStream.cs
- ForceCopyBuildProvider.cs
- EncryptedReference.cs
- OdbcConnectionOpen.cs
- XmlSchemaSimpleContentExtension.cs
- OneWayChannelFactory.cs
- StrokeSerializer.cs
- FrameSecurityDescriptor.cs
- WinInetCache.cs
- ToolStripOverflowButton.cs
- DeferredElementTreeState.cs
- QueryCursorEventArgs.cs
- Point3DValueSerializer.cs
- SplineKeyFrames.cs
- Clause.cs
- Rect3DValueSerializer.cs
- ReflectPropertyDescriptor.cs
- PageAsyncTaskManager.cs
- ListBindingHelper.cs
- LoginUtil.cs
- SqlParameterizer.cs
- DetailsViewPageEventArgs.cs
- DataGridViewCellEventArgs.cs
- WorkflowViewElement.cs
- KeyPressEvent.cs
- HttpCookieCollection.cs
- FileClassifier.cs
- InProcStateClientManager.cs
- UICuesEvent.cs
- OdbcHandle.cs
- TextBoxBase.cs
- DomainConstraint.cs
- ToolStripProfessionalLowResolutionRenderer.cs
- BypassElement.cs
- FileDialogCustomPlace.cs
- Model3DCollection.cs
- DocumentOrderComparer.cs
- SqlProfileProvider.cs
- ConstraintEnumerator.cs
- ParameterCollection.cs
- sqlstateclientmanager.cs
- DoubleAnimationUsingKeyFrames.cs
- GregorianCalendar.cs
- ErrorEventArgs.cs
- PrtTicket_Public_Simple.cs
- Directory.cs
- BitVec.cs
- SystemColors.cs
- DynamicResourceExtension.cs
- SpellerError.cs
- WebPartVerb.cs
- QueryableDataSourceEditData.cs
- DateTimeFormatInfo.cs
- StreamUpgradeAcceptor.cs
- LoginNameDesigner.cs
- LocationSectionRecord.cs
- MetadataImporter.cs
- WorkflowOperationBehavior.cs
- DesignerAutoFormatStyle.cs
- FormsAuthenticationModule.cs
- PageCache.cs
- FilterException.cs
- WebPart.cs
- Int32.cs