Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Markup / XamlWriter.cs / 1 / XamlWriter.cs
//---------------------------------------------------------------------------- // // File: XamlWriter.cs // // Description: // base Parser class that parses XML markup into an Avalon Element Tree // // // History: // 6/06/01: rogerg Created as Parser.cs // 5/29/03: peterost Ported to wcp as Parser.cs // 8/04/05: a-neabbu Split Parser into XamlReader and XamlWriter // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.IO.Packaging; using System.Windows; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Windows.Markup.Primitives; using MS.Internal.IO.Packaging; using MS.Internal.PresentationFramework; namespace System.Windows.Markup { ////// Parsing class used to create an Windows Presentation Platform Tree /// public static class XamlWriter { #region Public Methods ////// Save gets the xml respresentation /// for the given object instance /// /// /// Object instance /// ////// XAML string representing object instance /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static string Save(object obj) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } // Create TextWriter StringBuilder sb = new StringBuilder(); TextWriter writer = new StringWriter(sb, XamlSerializerUtil.EnglishUSCulture); try { Save(obj, writer); } finally { // Close writer writer.Close(); } return sb.ToString(); } /// /// Save writes the xml respresentation /// for the given object instance using the given writer /// /// /// Object instance /// /// /// Text Writer /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, TextWriter writer) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (writer == null) { throw new ArgumentNullException("writer"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(writer); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance to the given stream /// /// /// Object instance /// /// /// Stream /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, Stream stream) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (stream == null) { throw new ArgumentNullException("stream"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(stream, null); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance using the given /// writer. In addition it also allows the designer /// to participate in this conversion. /// /// /// Object instance /// /// /// XmlWriter /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XmlWriter xmlWriter) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (xmlWriter == null) { throw new ArgumentNullException("xmlWriter"); } try { MarkupWriter.SaveAsXml(xmlWriter, obj); } finally { xmlWriter.Flush(); } } /// /// Save writes the xml respresentation /// for the given object instance using the /// given XmlTextWriter embedded in the manager. /// /// /// Object instance /// /// /// Serialization Manager /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XamlDesignerSerializationManager manager) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (manager == null) { throw new ArgumentNullException("manager"); } MarkupWriter.SaveAsXml(manager.XmlWriter, obj, manager); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // // File: XamlWriter.cs // // Description: // base Parser class that parses XML markup into an Avalon Element Tree // // // History: // 6/06/01: rogerg Created as Parser.cs // 5/29/03: peterost Ported to wcp as Parser.cs // 8/04/05: a-neabbu Split Parser into XamlReader and XamlWriter // // Copyright (C) 2003 by Microsoft Corporation. All rights reserved. // //--------------------------------------------------------------------------- using System; using System.Xml; using System.IO; using System.IO.Packaging; using System.Windows; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Windows.Threading; using MS.Utility; using System.Security; using System.Security.Permissions; using System.Security.Policy; using System.Text; using System.ComponentModel.Design.Serialization; using System.Globalization; using System.Windows.Markup.Primitives; using MS.Internal.IO.Packaging; using MS.Internal.PresentationFramework; namespace System.Windows.Markup { /// /// Parsing class used to create an Windows Presentation Platform Tree /// public static class XamlWriter { #region Public Methods ////// Save gets the xml respresentation /// for the given object instance /// /// /// Object instance /// ////// XAML string representing object instance /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static string Save(object obj) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } // Create TextWriter StringBuilder sb = new StringBuilder(); TextWriter writer = new StringWriter(sb, XamlSerializerUtil.EnglishUSCulture); try { Save(obj, writer); } finally { // Close writer writer.Close(); } return sb.ToString(); } /// /// Save writes the xml respresentation /// for the given object instance using the given writer /// /// /// Object instance /// /// /// Text Writer /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, TextWriter writer) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (writer == null) { throw new ArgumentNullException("writer"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(writer); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance to the given stream /// /// /// Object instance /// /// /// Stream /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, Stream stream) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (stream == null) { throw new ArgumentNullException("stream"); } // Create XmlTextWriter XmlTextWriter xmlWriter = new XmlTextWriter(stream, null); MarkupWriter.SaveAsXml(xmlWriter, obj); } /// /// Save writes the xml respresentation /// for the given object instance using the given /// writer. In addition it also allows the designer /// to participate in this conversion. /// /// /// Object instance /// /// /// XmlWriter /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XmlWriter xmlWriter) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (xmlWriter == null) { throw new ArgumentNullException("xmlWriter"); } try { MarkupWriter.SaveAsXml(xmlWriter, obj); } finally { xmlWriter.Flush(); } } /// /// Save writes the xml respresentation /// for the given object instance using the /// given XmlTextWriter embedded in the manager. /// /// /// Object instance /// /// /// Serialization Manager /// ////// We only allow Serialization in partial trust. Although we would throw an exception later anyways, /// we throw one here so we know where to expect the exception. ( public static void Save(object obj, XamlDesignerSerializationManager manager) { // Must be in full trust SecurityHelper.DemandUnmanagedCode(); // Validate input arguments if (obj == null) { throw new ArgumentNullException("obj"); } if (manager == null) { throw new ArgumentNullException("manager"); } MarkupWriter.SaveAsXml(manager.XmlWriter, obj, manager); } #endregion Public Methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
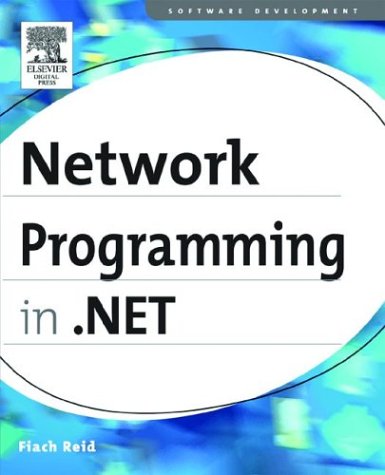
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextOnlyOutput.cs
- XmlSchemaDocumentation.cs
- SingleAnimationBase.cs
- SafeLocalMemHandle.cs
- ListViewAutomationPeer.cs
- DataGridTablesFactory.cs
- LongAverageAggregationOperator.cs
- AutomationPeer.cs
- ListViewHitTestInfo.cs
- LineSegment.cs
- TraceUtils.cs
- StorageEntityTypeMapping.cs
- IndependentAnimationStorage.cs
- XamlTypeMapperSchemaContext.cs
- ErrorStyle.cs
- LineGeometry.cs
- OutputChannel.cs
- WindowsGraphicsCacheManager.cs
- ThreadPool.cs
- SerializationAttributes.cs
- InteropExecutor.cs
- BitStream.cs
- DataSet.cs
- TableLayoutStyleCollection.cs
- ExpressionBuilderCollection.cs
- Point3DCollectionConverter.cs
- PtsHelper.cs
- InvalidCastException.cs
- DataContract.cs
- dataobject.cs
- InstanceNotReadyException.cs
- StrongNameIdentityPermission.cs
- CultureInfo.cs
- Asn1IntegerConverter.cs
- TreeNodeEventArgs.cs
- SelectionChangedEventArgs.cs
- ScopeElementCollection.cs
- ReadOnlyObservableCollection.cs
- ActivityStateRecord.cs
- KeyMatchBuilder.cs
- DocumentReferenceCollection.cs
- DataGridViewComponentPropertyGridSite.cs
- XmlQueryStaticData.cs
- LinkedResourceCollection.cs
- AliasedExpr.cs
- CollectionBuilder.cs
- Collection.cs
- XmlEncoding.cs
- ContentControl.cs
- Figure.cs
- Application.cs
- StringPropertyBuilder.cs
- _SingleItemRequestCache.cs
- ListViewSelectEventArgs.cs
- HttpResponseInternalWrapper.cs
- AlphaSortedEnumConverter.cs
- GifBitmapEncoder.cs
- X509RawDataKeyIdentifierClause.cs
- SessionParameter.cs
- WindowVisualStateTracker.cs
- TCEAdapterGenerator.cs
- HttpTransportSecurity.cs
- TextEvent.cs
- WebPartVerbsEventArgs.cs
- XmlSequenceWriter.cs
- JournalEntry.cs
- sortedlist.cs
- XmlCharCheckingWriter.cs
- ActivationArguments.cs
- MatrixAnimationUsingPath.cs
- FilterableAttribute.cs
- Parser.cs
- ResourcesGenerator.cs
- CngKeyCreationParameters.cs
- HtmlInputImage.cs
- DbConnectionOptions.cs
- PrintingPermission.cs
- UnmanagedHandle.cs
- UncommonField.cs
- GridViewRow.cs
- XmlNamespaceMappingCollection.cs
- SingleKeyFrameCollection.cs
- ObjectStateManagerMetadata.cs
- ConfigurationStrings.cs
- SqlUdtInfo.cs
- ImageCollectionCodeDomSerializer.cs
- HotCommands.cs
- LockedHandleGlyph.cs
- EventLogPermissionHolder.cs
- TextTreeText.cs
- EndOfStreamException.cs
- ControlBuilder.cs
- HtmlTableRow.cs
- TypefaceMap.cs
- InfoCardKeyedHashAlgorithm.cs
- TableItemProviderWrapper.cs
- mediaeventshelper.cs
- WebColorConverter.cs
- GroupPartitionExpr.cs
- SqlError.cs