Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / XmlUtil.cs / 1 / XmlUtil.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static xml utility functions // //--------------------------------------------------------------------- #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Xml; ////// static uri utility functions /// internal static partial class UriUtil { ///Creates a URI suitable for host-agnostic comparison purposes. /// URI to compare. ///URI suitable for comparison. private static Uri CreateBaseComparableUri(Uri uri) { Debug.Assert(uri != null, "uri != null"); uri = new Uri(uri.OriginalString.ToUpper(CultureInfo.InvariantCulture), UriKind.RelativeOrAbsolute); UriBuilder builder = new UriBuilder(uri); builder.Host = "h"; builder.Port = 80; builder.Scheme = "http"; return builder.Uri; } ///Parse the atom link relation attribute value /// atom link relation attribute value ///the assocation name or null internal static string GetNameFromAtomLinkRelationAttribute(string value) { string name = null; if (!String.IsNullOrEmpty(value)) { Uri uri = null; try { uri = new Uri(value, UriKind.RelativeOrAbsolute); } catch (UriFormatException) { // ignore the exception, obviously we don't have our expected relation value } if ((null != uri) && uri.IsAbsoluteUri) { string unescaped = uri.GetComponents(UriComponents.AbsoluteUri, UriFormat.SafeUnescaped); if (unescaped.StartsWith(XmlConstants.DataWebRelatedNamespace, StringComparison.Ordinal)) { name = unescaped.Substring(XmlConstants.DataWebRelatedNamespace.Length); } } } return name; } ///is the serviceRoot the base of the request uri /// baseUriWithSlash /// requestUri ///true if the serviceRoot is the base of the request uri internal static bool IsBaseOf(Uri baseUriWithSlash, Uri requestUri) { #if !ASTORIA_LIGHT // Uri.IsBaseOf not available return baseUriWithSlash.IsBaseOf(requestUri); #else return requestUri.OriginalString.StartsWith(baseUriWithSlash.OriginalString, StringComparison.Ordinal); #endif } ////// Determines whether the /// Candidate base URI. /// The specified Uri instance to test. ///Uri instance is a /// base of the specified Uri instance. /// true if the current Uri instance is a base of uri; otherwise, false. internal static bool UriInvariantInsensitiveIsBaseOf(Uri current, Uri uri) { Debug.Assert(current != null, "current != null"); Debug.Assert(uri != null, "uri != null"); Uri upperCurrent = CreateBaseComparableUri(current); Uri upperUri = CreateBaseComparableUri(uri); return UriUtil.IsBaseOf(upperCurrent, upperUri); } } ////// static xml utility function /// internal static partial class XmlUtil { ////// An initial nametable so that string comparisons during /// deserialization become reference comparisions /// ///nametable with element names used in application/atom+xml payload private static NameTable CreateAtomNameTable() { NameTable table = new NameTable(); table.Add(XmlConstants.AtomNamespace); table.Add(XmlConstants.DataWebNamespace); table.Add(XmlConstants.DataWebMetadataNamespace); table.Add(XmlConstants.AtomContentElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomContentSrcAttributeName); #endif table.Add(XmlConstants.AtomEntryElementName); table.Add(XmlConstants.AtomETagAttributeName); table.Add(XmlConstants.AtomFeedElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomIdElementName); #endif table.Add(XmlConstants.AtomInlineElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomLinkElementName); table.Add(XmlConstants.AtomLinkRelationAttributeName); #endif table.Add(XmlConstants.AtomNullAttributeName); table.Add(XmlConstants.AtomPropertiesElementName); table.Add(XmlConstants.AtomTitleElementName); table.Add(XmlConstants.AtomTypeAttributeName); table.Add(XmlConstants.XmlErrorCodeElementName); table.Add(XmlConstants.XmlErrorElementName); table.Add(XmlConstants.XmlErrorInnerElementName); table.Add(XmlConstants.XmlErrorMessageElementName); table.Add(XmlConstants.XmlErrorTypeElementName); return table; } ////// Creates a new XmlReader instance using the specified stream reader /// /// The stream reader from which you want to read /// The encoding of the stream ///XmlReader with the appropriate xml settings internal static XmlReader CreateXmlReader(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null stream"); XmlReaderSettings settings = new XmlReaderSettings(); settings.CheckCharacters = false; settings.CloseInput = true; settings.IgnoreWhitespace = true; settings.NameTable = XmlUtil.CreateAtomNameTable(); if (null == encoding) { // auto-detect the encoding return XmlReader.Create(stream, settings); } return XmlReader.Create(new StreamReader(stream, encoding), settings); } ////// Creates a new XmlWriter instance using the specified stream and writers the processing instruction /// with the given encoding value /// /// The stream to which you want to write /// Encoding that you want to specify in the reader settings as well as the processing instruction ///mlWriter with the appropriate xml settings and processing instruction internal static XmlWriter CreateXmlWriterAndWriteProcessingInstruction(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null stream"); Debug.Assert(null != encoding, "null encoding"); XmlWriterSettings settings = new XmlWriterSettings(); settings.CheckCharacters = false; settings.ConformanceLevel = ConformanceLevel.Fragment; settings.Encoding = encoding; settings.Indent = true; settings.NewLineHandling = NewLineHandling.Entitize; XmlWriter writer = XmlWriter.Create(stream, settings); writer.WriteProcessingInstruction("xml", "version=\"1.0\" encoding=\"" + encoding.WebName + "\" standalone=\"yes\""); return writer; } #if ASTORIA_CLIENT ////// get attribute value from specified namespace or empty namespace /// /// reader /// attributeName /// namespaceUri ///attribute value internal static string GetAttributeEx(this XmlReader reader, string attributeName, string namespaceUri) { return reader.GetAttribute(attributeName, namespaceUri) ?? reader.GetAttribute(attributeName); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// static xml utility functions // //--------------------------------------------------------------------- #if ASTORIA_CLIENT namespace System.Data.Services.Client #else namespace System.Data.Services #endif { using System.Diagnostics; using System.Globalization; using System.IO; using System.Text; using System.Xml; ////// static uri utility functions /// internal static partial class UriUtil { ///Creates a URI suitable for host-agnostic comparison purposes. /// URI to compare. ///URI suitable for comparison. private static Uri CreateBaseComparableUri(Uri uri) { Debug.Assert(uri != null, "uri != null"); uri = new Uri(uri.OriginalString.ToUpper(CultureInfo.InvariantCulture), UriKind.RelativeOrAbsolute); UriBuilder builder = new UriBuilder(uri); builder.Host = "h"; builder.Port = 80; builder.Scheme = "http"; return builder.Uri; } ///Parse the atom link relation attribute value /// atom link relation attribute value ///the assocation name or null internal static string GetNameFromAtomLinkRelationAttribute(string value) { string name = null; if (!String.IsNullOrEmpty(value)) { Uri uri = null; try { uri = new Uri(value, UriKind.RelativeOrAbsolute); } catch (UriFormatException) { // ignore the exception, obviously we don't have our expected relation value } if ((null != uri) && uri.IsAbsoluteUri) { string unescaped = uri.GetComponents(UriComponents.AbsoluteUri, UriFormat.SafeUnescaped); if (unescaped.StartsWith(XmlConstants.DataWebRelatedNamespace, StringComparison.Ordinal)) { name = unescaped.Substring(XmlConstants.DataWebRelatedNamespace.Length); } } } return name; } ///is the serviceRoot the base of the request uri /// baseUriWithSlash /// requestUri ///true if the serviceRoot is the base of the request uri internal static bool IsBaseOf(Uri baseUriWithSlash, Uri requestUri) { #if !ASTORIA_LIGHT // Uri.IsBaseOf not available return baseUriWithSlash.IsBaseOf(requestUri); #else return requestUri.OriginalString.StartsWith(baseUriWithSlash.OriginalString, StringComparison.Ordinal); #endif } ////// Determines whether the /// Candidate base URI. /// The specified Uri instance to test. ///Uri instance is a /// base of the specified Uri instance. /// true if the current Uri instance is a base of uri; otherwise, false. internal static bool UriInvariantInsensitiveIsBaseOf(Uri current, Uri uri) { Debug.Assert(current != null, "current != null"); Debug.Assert(uri != null, "uri != null"); Uri upperCurrent = CreateBaseComparableUri(current); Uri upperUri = CreateBaseComparableUri(uri); return UriUtil.IsBaseOf(upperCurrent, upperUri); } } ////// static xml utility function /// internal static partial class XmlUtil { ////// An initial nametable so that string comparisons during /// deserialization become reference comparisions /// ///nametable with element names used in application/atom+xml payload private static NameTable CreateAtomNameTable() { NameTable table = new NameTable(); table.Add(XmlConstants.AtomNamespace); table.Add(XmlConstants.DataWebNamespace); table.Add(XmlConstants.DataWebMetadataNamespace); table.Add(XmlConstants.AtomContentElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomContentSrcAttributeName); #endif table.Add(XmlConstants.AtomEntryElementName); table.Add(XmlConstants.AtomETagAttributeName); table.Add(XmlConstants.AtomFeedElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomIdElementName); #endif table.Add(XmlConstants.AtomInlineElementName); #if ASTORIA_CLIENT table.Add(XmlConstants.AtomLinkElementName); table.Add(XmlConstants.AtomLinkRelationAttributeName); #endif table.Add(XmlConstants.AtomNullAttributeName); table.Add(XmlConstants.AtomPropertiesElementName); table.Add(XmlConstants.AtomTitleElementName); table.Add(XmlConstants.AtomTypeAttributeName); table.Add(XmlConstants.XmlErrorCodeElementName); table.Add(XmlConstants.XmlErrorElementName); table.Add(XmlConstants.XmlErrorInnerElementName); table.Add(XmlConstants.XmlErrorMessageElementName); table.Add(XmlConstants.XmlErrorTypeElementName); return table; } ////// Creates a new XmlReader instance using the specified stream reader /// /// The stream reader from which you want to read /// The encoding of the stream ///XmlReader with the appropriate xml settings internal static XmlReader CreateXmlReader(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null stream"); XmlReaderSettings settings = new XmlReaderSettings(); settings.CheckCharacters = false; settings.CloseInput = true; settings.IgnoreWhitespace = true; settings.NameTable = XmlUtil.CreateAtomNameTable(); if (null == encoding) { // auto-detect the encoding return XmlReader.Create(stream, settings); } return XmlReader.Create(new StreamReader(stream, encoding), settings); } ////// Creates a new XmlWriter instance using the specified stream and writers the processing instruction /// with the given encoding value /// /// The stream to which you want to write /// Encoding that you want to specify in the reader settings as well as the processing instruction ///mlWriter with the appropriate xml settings and processing instruction internal static XmlWriter CreateXmlWriterAndWriteProcessingInstruction(Stream stream, Encoding encoding) { Debug.Assert(null != stream, "null stream"); Debug.Assert(null != encoding, "null encoding"); XmlWriterSettings settings = new XmlWriterSettings(); settings.CheckCharacters = false; settings.ConformanceLevel = ConformanceLevel.Fragment; settings.Encoding = encoding; settings.Indent = true; settings.NewLineHandling = NewLineHandling.Entitize; XmlWriter writer = XmlWriter.Create(stream, settings); writer.WriteProcessingInstruction("xml", "version=\"1.0\" encoding=\"" + encoding.WebName + "\" standalone=\"yes\""); return writer; } #if ASTORIA_CLIENT ////// get attribute value from specified namespace or empty namespace /// /// reader /// attributeName /// namespaceUri ///attribute value internal static string GetAttributeEx(this XmlReader reader, string attributeName, string namespaceUri) { return reader.GetAttribute(attributeName, namespaceUri) ?? reader.GetAttribute(attributeName); } #endif } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
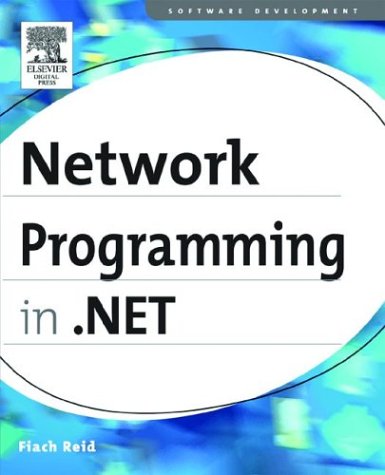
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PolicyManager.cs
- ISAPIApplicationHost.cs
- ProviderSettingsCollection.cs
- BooleanFunctions.cs
- TransformerInfoCollection.cs
- X509SubjectKeyIdentifierClause.cs
- IncrementalHitTester.cs
- MapPathBasedVirtualPathProvider.cs
- DataReaderContainer.cs
- XmlSchemaNotation.cs
- TrackingWorkflowEventArgs.cs
- ListViewHitTestInfo.cs
- XmlWhitespace.cs
- StateManagedCollection.cs
- TableItemStyle.cs
- HitTestFilterBehavior.cs
- UIElementCollection.cs
- KeyboardEventArgs.cs
- CacheAxisQuery.cs
- returneventsaver.cs
- DrawingContext.cs
- AuthenticationConfig.cs
- IgnorePropertiesAttribute.cs
- baseshape.cs
- SchemaNames.cs
- InternalDuplexBindingElement.cs
- SoapIgnoreAttribute.cs
- SHA256.cs
- SQLInt64.cs
- BatchStream.cs
- GenericAuthenticationEventArgs.cs
- Win32PrintDialog.cs
- SelectorAutomationPeer.cs
- XmlTextReaderImpl.cs
- ControlValuePropertyAttribute.cs
- LoadedOrUnloadedOperation.cs
- TraceSection.cs
- XmlSchemaAttribute.cs
- _UncName.cs
- StringToken.cs
- MyContact.cs
- BitArray.cs
- TraceLog.cs
- PropertyMapper.cs
- XComponentModel.cs
- SoapSchemaExporter.cs
- DiscoveryClientProtocol.cs
- FactorySettingsElement.cs
- BuilderPropertyEntry.cs
- ListViewItem.cs
- X509DefaultServiceCertificateElement.cs
- CodeTypeConstructor.cs
- FileLevelControlBuilderAttribute.cs
- LogLogRecord.cs
- SqlCommandSet.cs
- SimpleWebHandlerParser.cs
- SmuggledIUnknown.cs
- Base64Stream.cs
- Int64AnimationBase.cs
- AbsoluteQuery.cs
- HashAlgorithm.cs
- InvalidPropValue.cs
- GridViewCellAutomationPeer.cs
- HttpCapabilitiesEvaluator.cs
- HtmlImage.cs
- EntryPointNotFoundException.cs
- SmiContextFactory.cs
- CodeMemberMethod.cs
- SqlCommand.cs
- FrameworkPropertyMetadata.cs
- BuilderPropertyEntry.cs
- SignerInfo.cs
- XmlAttributeCache.cs
- DateBoldEvent.cs
- SharedPerformanceCounter.cs
- ListBindingHelper.cs
- SiteIdentityPermission.cs
- HeaderedContentControl.cs
- UInt64Converter.cs
- MonitoringDescriptionAttribute.cs
- ContentPropertyAttribute.cs
- CodeAttributeArgument.cs
- UiaCoreTypesApi.cs
- CursorConverter.cs
- EventLogRecord.cs
- HttpListenerRequest.cs
- RowVisual.cs
- WCFModelStrings.Designer.cs
- MappedMetaModel.cs
- XmlDataDocument.cs
- XmlNamespaceMapping.cs
- TextHidden.cs
- ContextStack.cs
- DashStyle.cs
- HttpException.cs
- XDeferredAxisSource.cs
- EntryPointNotFoundException.cs
- ControlType.cs
- DemultiplexingClientMessageFormatter.cs
- WindowsRichEdit.cs