Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Web / Configuration / TransformerInfoCollection.cs / 1305376 / TransformerInfoCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; [ConfigurationCollection(typeof(TransformerInfo))] public sealed class TransformerInfoCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _transformerEntries; static TransformerInfoCollection() { _properties = new ConfigurationPropertyCollection(); } ///protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TransformerInfo this[int index] { get { return (TransformerInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TransformerInfo transformerInfo) { BaseAdd(transformerInfo); } public void Clear() { BaseClear(); } /// protected override ConfigurationElement CreateNewElement() { return new TransformerInfo(); } public void Remove(string s) { BaseRemove(s); } public void RemoveAt(int index) { BaseRemoveAt(index); } /// protected override object GetElementKey(ConfigurationElement element) { return ((TransformerInfo)element).Name; } internal Hashtable GetTransformerEntries() { if (_transformerEntries == null) { lock (this) { if (_transformerEntries == null) { _transformerEntries = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TransformerInfo ti in this) { Type transformerType = ConfigUtil.GetType(ti.Type, "type", ti); if (transformerType.IsSubclassOf(typeof(WebPartTransformer)) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Type_doesnt_inherit_from_type, ti.Type, typeof(WebPartTransformer).FullName), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } Type consumerType; Type providerType; try { consumerType = WebPartTransformerAttribute.GetConsumerType(transformerType); providerType = WebPartTransformerAttribute.GetProviderType(transformerType); } catch (Exception e) { throw new ConfigurationErrorsException( SR.GetString(SR.Transformer_attribute_error, e.Message), e, ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } if (_transformerEntries.Count != 0) { foreach (DictionaryEntry entry in _transformerEntries) { Type existingTransformerType = (Type)entry.Value; // We know these methods will not throw, because for the type to be in the transformers // collection, we must have successfully gotten the types previously without an exception. Type existingConsumerType = WebPartTransformerAttribute.GetConsumerType(existingTransformerType); Type existingProviderType = WebPartTransformerAttribute.GetProviderType(existingTransformerType); if ((consumerType == existingConsumerType) && (providerType == existingProviderType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Transformer_types_already_added, (string)entry.Key, ti.Name), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } } } _transformerEntries[ti.Name] = transformerType; } } } } // return _transformerEntries; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Configuration; using System.Collections; using System.Collections.Specialized; using System.Security.Principal; using System.Web; using System.Web.Compilation; using System.Web.Configuration; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.WebControls.WebParts; using System.Web.Util; using System.Xml; using System.Security.Permissions; [ConfigurationCollection(typeof(TransformerInfo))] public sealed class TransformerInfoCollection : ConfigurationElementCollection { private static ConfigurationPropertyCollection _properties; private Hashtable _transformerEntries; static TransformerInfoCollection() { _properties = new ConfigurationPropertyCollection(); } ///protected override ConfigurationPropertyCollection Properties { get { return _properties; } } public TransformerInfo this[int index] { get { return (TransformerInfo)BaseGet(index); } set { if (BaseGet(index) != null) { BaseRemoveAt(index); } BaseAdd(index, value); } } public void Add(TransformerInfo transformerInfo) { BaseAdd(transformerInfo); } public void Clear() { BaseClear(); } /// protected override ConfigurationElement CreateNewElement() { return new TransformerInfo(); } public void Remove(string s) { BaseRemove(s); } public void RemoveAt(int index) { BaseRemoveAt(index); } /// protected override object GetElementKey(ConfigurationElement element) { return ((TransformerInfo)element).Name; } internal Hashtable GetTransformerEntries() { if (_transformerEntries == null) { lock (this) { if (_transformerEntries == null) { _transformerEntries = new Hashtable(StringComparer.OrdinalIgnoreCase); foreach (TransformerInfo ti in this) { Type transformerType = ConfigUtil.GetType(ti.Type, "type", ti); if (transformerType.IsSubclassOf(typeof(WebPartTransformer)) == false) { throw new ConfigurationErrorsException( SR.GetString( SR.Type_doesnt_inherit_from_type, ti.Type, typeof(WebPartTransformer).FullName), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } Type consumerType; Type providerType; try { consumerType = WebPartTransformerAttribute.GetConsumerType(transformerType); providerType = WebPartTransformerAttribute.GetProviderType(transformerType); } catch (Exception e) { throw new ConfigurationErrorsException( SR.GetString(SR.Transformer_attribute_error, e.Message), e, ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } if (_transformerEntries.Count != 0) { foreach (DictionaryEntry entry in _transformerEntries) { Type existingTransformerType = (Type)entry.Value; // We know these methods will not throw, because for the type to be in the transformers // collection, we must have successfully gotten the types previously without an exception. Type existingConsumerType = WebPartTransformerAttribute.GetConsumerType(existingTransformerType); Type existingProviderType = WebPartTransformerAttribute.GetProviderType(existingTransformerType); if ((consumerType == existingConsumerType) && (providerType == existingProviderType)) { throw new ConfigurationErrorsException( SR.GetString( SR.Transformer_types_already_added, (string)entry.Key, ti.Name), ti.ElementInformation.Properties["type"].Source, ti.ElementInformation.Properties["type"].LineNumber); } } } _transformerEntries[ti.Name] = transformerType; } } } } // return _transformerEntries; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
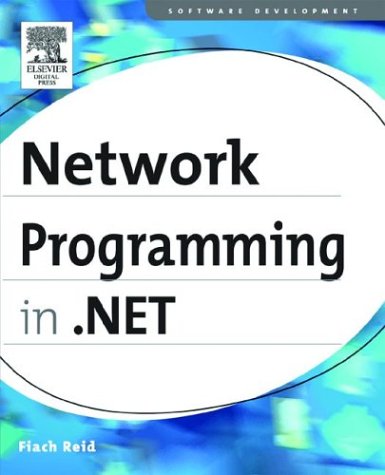
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PropertyTabAttribute.cs
- SystemInfo.cs
- TargetControlTypeCache.cs
- AbsoluteQuery.cs
- SystemNetworkInterface.cs
- TableRowGroup.cs
- PromptEventArgs.cs
- webclient.cs
- ZipIORawDataFileBlock.cs
- RtfToXamlReader.cs
- XPathPatternParser.cs
- RemoteWebConfigurationHostStream.cs
- StorageInfo.cs
- TextFormatterHost.cs
- Trace.cs
- SystemWebExtensionsSectionGroup.cs
- PtsHelper.cs
- WebPartEditorCancelVerb.cs
- UICuesEvent.cs
- ThicknessAnimationBase.cs
- WindowsSpinner.cs
- DecimalAnimation.cs
- SerialPinChanges.cs
- RectConverter.cs
- DefaultEvaluationContext.cs
- LexicalChunk.cs
- ResXFileRef.cs
- NamespaceEmitter.cs
- Mapping.cs
- InfoCardRSAOAEPKeyExchangeFormatter.cs
- SessionEndingCancelEventArgs.cs
- AmbiguousMatchException.cs
- BulletedListDesigner.cs
- DataViewManagerListItemTypeDescriptor.cs
- PersonalizableAttribute.cs
- ComponentConverter.cs
- TableLayoutPanel.cs
- wgx_sdk_version.cs
- DocumentReferenceCollection.cs
- ToolStripSeparatorRenderEventArgs.cs
- IntPtr.cs
- PartitionResolver.cs
- Stroke.cs
- ReliableSessionElement.cs
- DesignerWidgets.cs
- rsa.cs
- FormatConvertedBitmap.cs
- Win32Native.cs
- PointCollection.cs
- ZipIOExtraFieldElement.cs
- MatrixStack.cs
- HttpListenerResponse.cs
- MatrixTransform.cs
- HttpHandlerActionCollection.cs
- CapacityStreamGeometryContext.cs
- BitFlagsGenerator.cs
- BuildDependencySet.cs
- TextDecorationCollection.cs
- SimpleType.cs
- PriorityQueue.cs
- COM2DataTypeToManagedDataTypeConverter.cs
- UserNameSecurityTokenAuthenticator.cs
- VerticalAlignConverter.cs
- WizardSideBarListControlItemEventArgs.cs
- PerformanceCounterManager.cs
- DragCompletedEventArgs.cs
- SimpleWorkerRequest.cs
- UrlParameterWriter.cs
- ClientRuntimeConfig.cs
- LinqDataSourceDisposeEventArgs.cs
- OpenTypeLayout.cs
- CacheHelper.cs
- EventRecordWrittenEventArgs.cs
- RSAOAEPKeyExchangeDeformatter.cs
- OdbcCommandBuilder.cs
- DataBoundLiteralControl.cs
- FilterQueryOptionExpression.cs
- ProjectionPathBuilder.cs
- SynchronizationHandlesCodeDomSerializer.cs
- Point3DAnimationUsingKeyFrames.cs
- CommandHelpers.cs
- TextServicesHost.cs
- TextEditorParagraphs.cs
- CellConstantDomain.cs
- ModelPerspective.cs
- DiffuseMaterial.cs
- LightweightCodeGenerator.cs
- ScrollBar.cs
- SoapExtensionReflector.cs
- ChineseLunisolarCalendar.cs
- SHA256.cs
- UnsafeNativeMethods.cs
- ParserStreamGeometryContext.cs
- DataGridViewRowEventArgs.cs
- XmlAtomicValue.cs
- ConnectionProviderAttribute.cs
- WebServiceHandlerFactory.cs
- ManagementClass.cs
- Cursor.cs
- TextPatternIdentifiers.cs