Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / ModelPropertyCollectionImpl.cs / 1305376 / ModelPropertyCollectionImpl.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections.Generic; using System.Activities.Presentation.View; using System.ComponentModel; using System.IO; using System.Linq; // This provides a container for model properties of a modelItem. // This uses the TypeDescriptor.GetProperties() instead of using reflection // to get the properties of a model item. So any model instance implementing ICustomTypeProvider // is automatically taken care of. class ModelPropertyCollectionImpl : ModelPropertyCollection { ModelItem parent; bool createFakeModelProperties; public ModelPropertyCollectionImpl(ModelItem parent) { this.parent = parent; createFakeModelProperties = this.parent is FakeModelItemImpl; } public override IEnumeratorGetEnumerator() { foreach (PropertyDescriptor propertyDescriptor in GetPropertyDescriptors()) { yield return CreateProperty(parent, propertyDescriptor); } } protected override ModelProperty Find(System.Windows.DependencyProperty value, bool throwOnError ) { // We dont support dependency properties. if (throwOnError) { throw FxTrace.Exception.AsError(new NotSupportedException()); } else { return null; } } protected override ModelProperty Find(string name, bool throwOnError) { PropertyDescriptor propertyDescriptor = GetPropertyDescriptors()[name]; if (propertyDescriptor != null) { return CreateProperty(parent, propertyDescriptor); } return null; } ModelProperty CreateProperty(ModelItem parent, PropertyDescriptor propertyDescriptor) { bool isAttached = propertyDescriptor is AttachedPropertyDescriptor; return this.createFakeModelProperties ? (ModelProperty)(new FakeModelPropertyImpl((FakeModelItemImpl)parent, propertyDescriptor)) : (ModelProperty)(new ModelPropertyImpl(parent, propertyDescriptor,isAttached)); } PropertyDescriptorCollection GetPropertyDescriptors() { PropertyDescriptorCollection propertyDescriptors = PropertyDescriptorCollection.Empty; try { object instance = parent.GetCurrentValue(); if (instance != null) { if (!(instance is ICustomTypeDescriptor)) { Type instanceType = instance.GetType(); if (instanceType.IsValueType) { propertyDescriptors = TypeDescriptor.GetProvider(instanceType).GetTypeDescriptor(instanceType).GetProperties(); } else { propertyDescriptors = TypeDescriptor.GetProvider(instance).GetTypeDescriptor(instance).GetProperties(); } } else { propertyDescriptors = TypeDescriptor.GetProperties(instance); } } // Add browsable attached properties AttachedPropertiesService AttachedPropertiesService = this.parent.GetEditingContext().Services.GetService (); if (AttachedPropertiesService != null) { var browsableAttachedProperties = from attachedProperty in AttachedPropertiesService.GetAttachedProperties(this.parent.ItemType) where attachedProperty.IsBrowsable select new AttachedPropertyDescriptor(attachedProperty, this.parent); List mergedProperties = new List (); foreach (PropertyDescriptor propertyDescriptor in propertyDescriptors) { mergedProperties.Add(propertyDescriptor); } propertyDescriptors = new PropertyDescriptorCollection(mergedProperties.Concat(browsableAttachedProperties).ToArray(), true); } } catch (FileNotFoundException e) { EditingContext context = parent.GetEditingContext(); if (context.Items.GetValue () == null) { context.Items.SetValue(new ErrorItem { Message = e.Message, Details = e.ToString() }); } } return propertyDescriptors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections.Generic; using System.Activities.Presentation.View; using System.ComponentModel; using System.IO; using System.Linq; // This provides a container for model properties of a modelItem. // This uses the TypeDescriptor.GetProperties() instead of using reflection // to get the properties of a model item. So any model instance implementing ICustomTypeProvider // is automatically taken care of. class ModelPropertyCollectionImpl : ModelPropertyCollection { ModelItem parent; bool createFakeModelProperties; public ModelPropertyCollectionImpl(ModelItem parent) { this.parent = parent; createFakeModelProperties = this.parent is FakeModelItemImpl; } public override IEnumerator GetEnumerator() { foreach (PropertyDescriptor propertyDescriptor in GetPropertyDescriptors()) { yield return CreateProperty(parent, propertyDescriptor); } } protected override ModelProperty Find(System.Windows.DependencyProperty value, bool throwOnError ) { // We dont support dependency properties. if (throwOnError) { throw FxTrace.Exception.AsError(new NotSupportedException()); } else { return null; } } protected override ModelProperty Find(string name, bool throwOnError) { PropertyDescriptor propertyDescriptor = GetPropertyDescriptors()[name]; if (propertyDescriptor != null) { return CreateProperty(parent, propertyDescriptor); } return null; } ModelProperty CreateProperty(ModelItem parent, PropertyDescriptor propertyDescriptor) { bool isAttached = propertyDescriptor is AttachedPropertyDescriptor; return this.createFakeModelProperties ? (ModelProperty)(new FakeModelPropertyImpl((FakeModelItemImpl)parent, propertyDescriptor)) : (ModelProperty)(new ModelPropertyImpl(parent, propertyDescriptor,isAttached)); } PropertyDescriptorCollection GetPropertyDescriptors() { PropertyDescriptorCollection propertyDescriptors = PropertyDescriptorCollection.Empty; try { object instance = parent.GetCurrentValue(); if (instance != null) { if (!(instance is ICustomTypeDescriptor)) { Type instanceType = instance.GetType(); if (instanceType.IsValueType) { propertyDescriptors = TypeDescriptor.GetProvider(instanceType).GetTypeDescriptor(instanceType).GetProperties(); } else { propertyDescriptors = TypeDescriptor.GetProvider(instance).GetTypeDescriptor(instance).GetProperties(); } } else { propertyDescriptors = TypeDescriptor.GetProperties(instance); } } // Add browsable attached properties AttachedPropertiesService AttachedPropertiesService = this.parent.GetEditingContext().Services.GetService (); if (AttachedPropertiesService != null) { var browsableAttachedProperties = from attachedProperty in AttachedPropertiesService.GetAttachedProperties(this.parent.ItemType) where attachedProperty.IsBrowsable select new AttachedPropertyDescriptor(attachedProperty, this.parent); List mergedProperties = new List (); foreach (PropertyDescriptor propertyDescriptor in propertyDescriptors) { mergedProperties.Add(propertyDescriptor); } propertyDescriptors = new PropertyDescriptorCollection(mergedProperties.Concat(browsableAttachedProperties).ToArray(), true); } } catch (FileNotFoundException e) { EditingContext context = parent.GetEditingContext(); if (context.Items.GetValue () == null) { context.Items.SetValue(new ErrorItem { Message = e.Message, Details = e.ToString() }); } } return propertyDescriptors; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
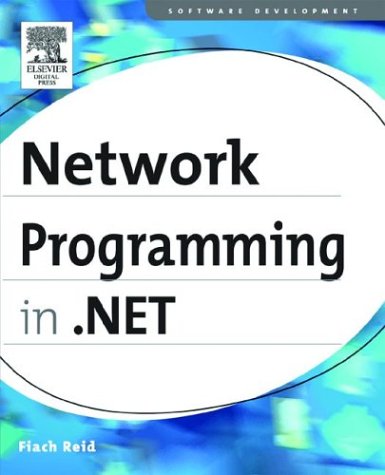
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataBindingHandlerAttribute.cs
- Menu.cs
- FixedPosition.cs
- StatusBarPanelClickEvent.cs
- BufferedStream.cs
- Content.cs
- Color.cs
- MailAddress.cs
- ChineseLunisolarCalendar.cs
- AccessControlEntry.cs
- StaticExtensionConverter.cs
- PrintController.cs
- EnterpriseServicesHelper.cs
- StylusOverProperty.cs
- AssemblyName.cs
- IProvider.cs
- LayoutUtils.cs
- HostExecutionContextManager.cs
- XmlSchemaSimpleContentRestriction.cs
- CompiledIdentityConstraint.cs
- SoapTypeAttribute.cs
- DesignerTransaction.cs
- PerfCounterSection.cs
- TemplateControl.cs
- SqlTransaction.cs
- EventRecord.cs
- Triangle.cs
- DeviceSpecificDesigner.cs
- SymLanguageVendor.cs
- StylusPointPropertyInfoDefaults.cs
- DataGridCellInfo.cs
- HttpClientProtocol.cs
- IArgumentProvider.cs
- ElementFactory.cs
- SamlAuthenticationClaimResource.cs
- QuestionEventArgs.cs
- EqualityComparer.cs
- RadioButtonBaseAdapter.cs
- _CacheStreams.cs
- FixedSOMTable.cs
- FlowDocumentReaderAutomationPeer.cs
- PersonalizableTypeEntry.cs
- SizeAnimationClockResource.cs
- CommonGetThemePartSize.cs
- NavigationCommands.cs
- SqlExpressionNullability.cs
- KeyEventArgs.cs
- DataGridViewBand.cs
- StringValidator.cs
- CollectionBuilder.cs
- EdmProperty.cs
- AmbiguousMatchException.cs
- MetadataItemEmitter.cs
- CodeComment.cs
- CodeArgumentReferenceExpression.cs
- UIElementParagraph.cs
- X509SecurityTokenParameters.cs
- DataSourceProvider.cs
- ExpressionVisitor.cs
- DecoderFallback.cs
- _Win32.cs
- XpsFilter.cs
- Win32MouseDevice.cs
- ValueUtilsSmi.cs
- EditableTreeList.cs
- SapiAttributeParser.cs
- FilteredReadOnlyMetadataCollection.cs
- UshortList2.cs
- PathFigureCollection.cs
- AutomationElement.cs
- OciLobLocator.cs
- AssemblyNameUtility.cs
- DesignerForm.cs
- DragDeltaEventArgs.cs
- CriticalHandle.cs
- ToolStripItemImageRenderEventArgs.cs
- BindingValueChangedEventArgs.cs
- InvalidFilterCriteriaException.cs
- RangeValuePatternIdentifiers.cs
- SizeFConverter.cs
- TextEditor.cs
- IdnElement.cs
- RedistVersionInfo.cs
- CodeChecksumPragma.cs
- Timer.cs
- OleStrCAMarshaler.cs
- TearOffProxy.cs
- BuildProvider.cs
- TableCellCollection.cs
- CommonXSendMessage.cs
- TopClause.cs
- Vector3DAnimationBase.cs
- ManifestSignatureInformation.cs
- XamlPointCollectionSerializer.cs
- SwitchLevelAttribute.cs
- DataGridViewRow.cs
- DescendentsWalker.cs
- WebPartConnectionsConfigureVerb.cs
- ImageClickEventArgs.cs
- KeyValuePairs.cs