Code:
/ FX-1434 / FX-1434 / 1.0 / untmp / whidbey / REDBITS / ndp / clr / src / BCL / System / TypedReference.cs / 1 / TypedReference.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== namespace System { // TypedReference is basically only ever seen on the call stack, and in param arrays. // These are blob that must be dealt with by the compiler. using System; using System.Reflection; using System.Runtime.CompilerServices; using CultureInfo = System.Globalization.CultureInfo; using FieldInfo = System.Reflection.FieldInfo; using System.Security.Permissions; [CLSCompliant(false)] [System.Runtime.InteropServices.ComVisible(true)] public struct TypedReference { private IntPtr Value; private IntPtr Type; [CLSCompliant(false)] [ReflectionPermission(SecurityAction.LinkDemand, MemberAccess=true)] public static TypedReference MakeTypedReference(Object target, FieldInfo[] flds) { if (target == null) throw new ArgumentNullException("target"); if (flds == null) throw new ArgumentNullException("flds"); if (flds.Length == 0) throw new ArgumentException(Environment.GetResourceString("Arg_ArrayZeroError")); else { RuntimeFieldHandle[] fields = new RuntimeFieldHandle[flds.Length]; // For proper handling of Nullabledon't change GetType() to something like 'IsAssignableFrom' // Currently we can't make a TypedReference to fields of Nullable , which is fine. Type targetType = target.GetType(); for (int i = 0; i < flds.Length; i++) { FieldInfo field = flds[i]; if (!(field is RuntimeFieldInfo)) throw new ArgumentException(Environment.GetResourceString("Argument_MustBeRuntimeFieldInfo")); else if (field.IsInitOnly || field.IsStatic) throw new ArgumentException(Environment.GetResourceString("Argument_TypedReferenceInvalidField")); if (targetType != field.DeclaringType && !targetType.IsSubclassOf(field.DeclaringType)) throw new MissingMemberException(Environment.GetResourceString("MissingMemberTypeRef")); Type fieldType = field.FieldType; if (fieldType.IsPrimitive) throw new ArgumentException(Environment.GetResourceString("Arg_TypeRefPrimitve")); if (i < flds.Length - 1) if (!fieldType.IsValueType) throw new MissingMemberException(Environment.GetResourceString("MissingMemberNestErr")); fields[i] = field.FieldHandle; targetType = fieldType; } TypedReference result = new TypedReference (); // reference to TypedReference is banned, so have to pass result as pointer unsafe { InternalMakeTypedReference(&result, target, fields, targetType.TypeHandle); } return result; } } [MethodImplAttribute(MethodImplOptions.InternalCall)] // reference to TypedReference is banned, so have to pass result as pointer private unsafe static extern void InternalMakeTypedReference(void * result, Object target, RuntimeFieldHandle[] flds, RuntimeTypeHandle lastFieldType); public override int GetHashCode() { if (Type == IntPtr.Zero) return 0; else return __reftype(this).GetHashCode(); } public override bool Equals(Object o) { throw new NotSupportedException(Environment.GetResourceString("NotSupported_NYI")); } public unsafe static Object ToObject(TypedReference value) { return InternalToObject(&value); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal unsafe extern static Object InternalToObject(void * value); internal bool IsNull { get { return Value.IsNull() && Type.IsNull(); } } public static Type GetTargetType (TypedReference value) { return __reftype(value); } public static RuntimeTypeHandle TargetTypeToken (TypedReference value) { return __reftype(value).TypeHandle; } // This may cause the type to be changed. [CLSCompliant(false)] public unsafe static void SetTypedReference(TypedReference target, Object value) { InternalSetTypedReference(&target, value); } [MethodImplAttribute(MethodImplOptions.InternalCall)] internal unsafe extern static void InternalSetTypedReference(void * target, Object value); } }
Link Menu
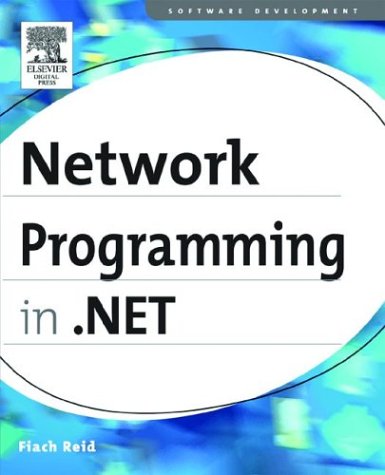
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DesignTimeResourceProviderFactoryAttribute.cs
- FloatMinMaxAggregationOperator.cs
- XamlFilter.cs
- GPPOINT.cs
- Sentence.cs
- AllMembershipCondition.cs
- XmlEntityReference.cs
- CellParaClient.cs
- Inflater.cs
- SqlXmlStorage.cs
- TraceListener.cs
- UpdatePanel.cs
- DataGridViewRowPrePaintEventArgs.cs
- StringUtil.cs
- PropertyDescriptorComparer.cs
- DetailsViewPageEventArgs.cs
- Decoder.cs
- DataGridViewRowStateChangedEventArgs.cs
- RoleManagerEventArgs.cs
- BufferedOutputAsyncStream.cs
- ExtensibleClassFactory.cs
- WindowsGraphics.cs
- CodePrimitiveExpression.cs
- SequentialWorkflowHeaderFooter.cs
- OutKeywords.cs
- IDictionary.cs
- XPathNodePointer.cs
- PlatformNotSupportedException.cs
- _FtpDataStream.cs
- SystemEvents.cs
- ClosureBinding.cs
- XPathPatternParser.cs
- Baml2006ReaderFrame.cs
- EntityDataSourceDesigner.cs
- StylusPointPropertyId.cs
- TransformGroup.cs
- WarningException.cs
- OleDbTransaction.cs
- FileChangeNotifier.cs
- DBBindings.cs
- PropertyCondition.cs
- XPathQilFactory.cs
- PageAsyncTask.cs
- WindowsRegion.cs
- WebBrowserSiteBase.cs
- Thread.cs
- MsmqMessageProperty.cs
- XmlSignatureProperties.cs
- HashSetDebugView.cs
- SharedPersonalizationStateInfo.cs
- DataService.cs
- NavigationService.cs
- TransactionScopeDesigner.cs
- SerializerWriterEventHandlers.cs
- RijndaelCryptoServiceProvider.cs
- UIElementAutomationPeer.cs
- xsdvalidator.cs
- ManagementOperationWatcher.cs
- LineInfo.cs
- DataGridHelper.cs
- ImageButton.cs
- x509store.cs
- SchemaMerger.cs
- CompressEmulationStream.cs
- ServiceObjectContainer.cs
- SchemaLookupTable.cs
- CodeCompileUnit.cs
- BindingFormattingDialog.cs
- BufferModesCollection.cs
- FontDialog.cs
- ProcessHostMapPath.cs
- Socket.cs
- DispatcherProcessingDisabled.cs
- TypeExtensionSerializer.cs
- RuleSettings.cs
- WrapperEqualityComparer.cs
- WebPartConnectionsDisconnectVerb.cs
- GlyphRunDrawing.cs
- UInt32Converter.cs
- ComponentSerializationService.cs
- SamlNameIdentifierClaimResource.cs
- BamlLocalizationDictionary.cs
- sqlnorm.cs
- ItemAutomationPeer.cs
- MbpInfo.cs
- ConfigurationErrorsException.cs
- CultureInfo.cs
- Solver.cs
- DataIdProcessor.cs
- HtmlInputCheckBox.cs
- ContentPosition.cs
- SoapParser.cs
- DataGridViewRowEventArgs.cs
- EntityDataSourceUtil.cs
- InputBuffer.cs
- EntityAdapter.cs
- ProcessModuleCollection.cs
- FilterableAttribute.cs
- ResolveDuplexAsyncResult.cs
- DoubleConverter.cs