Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Cryptography / SignatureDescription.cs / 1305376 / SignatureDescription.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // SignatureDescription.cs // namespace System.Security.Cryptography { using System.Security.Util; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public class SignatureDescription { private String _strKey; private String _strDigest; private String _strFormatter; private String _strDeformatter; // // public constructors // public SignatureDescription() { } public SignatureDescription(SecurityElement el) { if (el == null) throw new ArgumentNullException("el"); Contract.EndContractBlock(); _strKey = el.SearchForTextOfTag("Key"); _strDigest = el.SearchForTextOfTag("Digest"); _strFormatter = el.SearchForTextOfTag("Formatter"); _strDeformatter = el.SearchForTextOfTag("Deformatter"); } // // property methods // public String KeyAlgorithm { get { return _strKey; } set { _strKey = value; } } public String DigestAlgorithm { get { return _strDigest; } set { _strDigest = value; } } public String FormatterAlgorithm { get { return _strFormatter; } set { _strFormatter = value; } } public String DeformatterAlgorithm { get {return _strDeformatter; } set {_strDeformatter = value; } } // // public methods // [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item; item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(_strDeformatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureFormatter CreateFormatter(AsymmetricAlgorithm key) { AsymmetricSignatureFormatter item; item = (AsymmetricSignatureFormatter) CryptoConfig.CreateFromName(_strFormatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual HashAlgorithm CreateDigest() { return (HashAlgorithm) CryptoConfig.CreateFromName(_strDigest); } } internal class RSAPKCS1SHA1SignatureDescription : SignatureDescription { public RSAPKCS1SHA1SignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.RSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureDeformatter"; } public override AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(DeformatterAlgorithm); item.SetKey(key); item.SetHashAlgorithm("SHA1"); return item; } } internal class DSASignatureDescription : SignatureDescription { public DSASignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.DSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.DSASignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.DSASignatureDeformatter"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== //[....] // // // SignatureDescription.cs // namespace System.Security.Cryptography { using System.Security.Util; using System.Diagnostics.Contracts; [System.Runtime.InteropServices.ComVisible(true)] public class SignatureDescription { private String _strKey; private String _strDigest; private String _strFormatter; private String _strDeformatter; // // public constructors // public SignatureDescription() { } public SignatureDescription(SecurityElement el) { if (el == null) throw new ArgumentNullException("el"); Contract.EndContractBlock(); _strKey = el.SearchForTextOfTag("Key"); _strDigest = el.SearchForTextOfTag("Digest"); _strFormatter = el.SearchForTextOfTag("Formatter"); _strDeformatter = el.SearchForTextOfTag("Deformatter"); } // // property methods // public String KeyAlgorithm { get { return _strKey; } set { _strKey = value; } } public String DigestAlgorithm { get { return _strDigest; } set { _strDigest = value; } } public String FormatterAlgorithm { get { return _strFormatter; } set { _strFormatter = value; } } public String DeformatterAlgorithm { get {return _strDeformatter; } set {_strDeformatter = value; } } // // public methods // [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item; item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(_strDeformatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual AsymmetricSignatureFormatter CreateFormatter(AsymmetricAlgorithm key) { AsymmetricSignatureFormatter item; item = (AsymmetricSignatureFormatter) CryptoConfig.CreateFromName(_strFormatter); item.SetKey(key); return item; } [System.Security.SecuritySafeCritical] // auto-generated public virtual HashAlgorithm CreateDigest() { return (HashAlgorithm) CryptoConfig.CreateFromName(_strDigest); } } internal class RSAPKCS1SHA1SignatureDescription : SignatureDescription { public RSAPKCS1SHA1SignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.RSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.RSAPKCS1SignatureDeformatter"; } public override AsymmetricSignatureDeformatter CreateDeformatter(AsymmetricAlgorithm key) { AsymmetricSignatureDeformatter item = (AsymmetricSignatureDeformatter) CryptoConfig.CreateFromName(DeformatterAlgorithm); item.SetKey(key); item.SetHashAlgorithm("SHA1"); return item; } } internal class DSASignatureDescription : SignatureDescription { public DSASignatureDescription() { KeyAlgorithm = "System.Security.Cryptography.DSACryptoServiceProvider"; DigestAlgorithm = "System.Security.Cryptography.SHA1CryptoServiceProvider"; FormatterAlgorithm = "System.Security.Cryptography.DSASignatureFormatter"; DeformatterAlgorithm = "System.Security.Cryptography.DSASignatureDeformatter"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
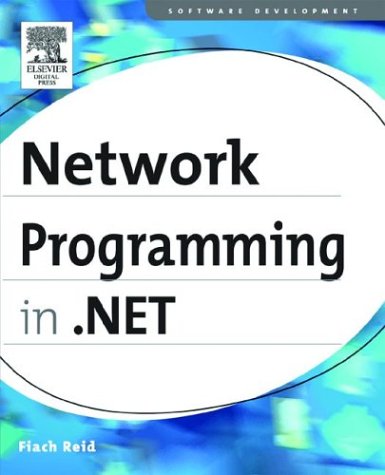
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlBulkCopyColumnMapping.cs
- CodeDelegateInvokeExpression.cs
- WebBrowserSiteBase.cs
- FontStretches.cs
- InvalidCastException.cs
- ContractHandle.cs
- FactoryMaker.cs
- QuadraticBezierSegment.cs
- UIPropertyMetadata.cs
- UInt64.cs
- SafeProcessHandle.cs
- DefaultValidator.cs
- RelAssertionDirectKeyIdentifierClause.cs
- NavigationPropertySingletonExpression.cs
- LogLogRecordHeader.cs
- ReadOnlyDictionary.cs
- DeploymentSection.cs
- CompositeDataBoundControl.cs
- ReadOnlyState.cs
- Utilities.cs
- SqlRetyper.cs
- ResourceContainer.cs
- PropertyMetadata.cs
- LinkDescriptor.cs
- Range.cs
- EntityContainerRelationshipSetEnd.cs
- ListViewCancelEventArgs.cs
- StorageEndPropertyMapping.cs
- ProfileSection.cs
- UnsafeNativeMethods.cs
- PasswordRecovery.cs
- _AutoWebProxyScriptEngine.cs
- ErrorTableItemStyle.cs
- Cursor.cs
- DataGridViewImageColumn.cs
- WebPartEditorApplyVerb.cs
- AddValidationError.cs
- TypeConverter.cs
- PrivilegeNotHeldException.cs
- IOException.cs
- SqlFormatter.cs
- OletxDependentTransaction.cs
- ProtocolsConfigurationHandler.cs
- LazyTextWriterCreator.cs
- ContextBase.cs
- DbException.cs
- SerialErrors.cs
- Matrix3DStack.cs
- UnsafeNativeMethodsCLR.cs
- BitmapMetadata.cs
- QueryCacheEntry.cs
- StandardBindingElementCollection.cs
- InputGestureCollection.cs
- ObservableDictionary.cs
- HttpProfileBase.cs
- BorderSidesEditor.cs
- BoundsDrawingContextWalker.cs
- EmbeddedMailObjectsCollection.cs
- SerializationObjectManager.cs
- ProcessThreadCollection.cs
- HttpListenerContext.cs
- HtmlInputHidden.cs
- CategoryValueConverter.cs
- NamespaceQuery.cs
- SerialStream.cs
- MatcherBuilder.cs
- ServiceOperationWrapper.cs
- DockingAttribute.cs
- HandleRef.cs
- InputLanguageEventArgs.cs
- ProxyFragment.cs
- GenerateScriptTypeAttribute.cs
- XmlUrlResolver.cs
- Drawing.cs
- CatalogZoneBase.cs
- TemplateManager.cs
- ADMembershipUser.cs
- StrongNamePublicKeyBlob.cs
- SoapIncludeAttribute.cs
- BitmapScalingModeValidation.cs
- DataGridViewRowHeaderCell.cs
- IncrementalCompileAnalyzer.cs
- TraceSwitch.cs
- HttpProfileGroupBase.cs
- StylusOverProperty.cs
- QilUnary.cs
- ToolStripSeparator.cs
- MapPathBasedVirtualPathProvider.cs
- InvalidProgramException.cs
- Listbox.cs
- HtmlFormWrapper.cs
- DebuggerAttributes.cs
- AssemblyBuilderData.cs
- AttachInfo.cs
- SchemaCreator.cs
- NavigatorOutput.cs
- BasicKeyConstraint.cs
- ModuleBuilder.cs
- DragDeltaEventArgs.cs
- DesignColumn.cs