Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Core / CSharp / System / Windows / Media / textformatting / TextRunTypographyProperties.cs / 1 / TextRunTypographyProperties.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextRunTypographyProperties.cs // // Contents: Text run Typography properties // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-7-2005 Sergey Malkin (SergeyM) // //----------------------------------------------------------------------- using System; using System.Windows; using MS.Internal.Shaping; namespace System.Windows.Media.TextFormatting { ////// Text run typography properties /// /// Client set properties to generate set of features /// that will be processed by OpenType layout engine. /// /// For details see OpenType font specification at /// http://www.microsoft.com/typography/ /// /// public abstract class TextRunTypographyProperties { #region Public typography properties ////// Common ligatures assisting with text readability. /// Examples: fi, fl ligatures /// public abstract bool StandardLigatures { get; } ////// Ligature forms depending on surrounding context /// public abstract bool ContextualLigatures { get; } ////// Additional ligatures to assist with text readability /// Examples: Qu, Th /// public abstract bool DiscretionaryLigatures { get; } ////// Ligatures used in historical typography /// Examples: ct, st /// public abstract bool HistoricalLigatures { get; } ////// Custom forms defined by surrounding context /// Examples: multiple medial forms in Urdu Nastaliq fonts /// public abstract bool ContextualAlternates { get; } ////// Forms commonly used in the past /// Examples: long s, old Fraktur k /// public abstract bool HistoricalForms { get; } ////// Feature adjusting spacing between charactersto enchance word shape /// public abstract bool Kerning { get; } ////// Feature adjusting inter-glyph spacing to provide better readability for all-`capital text /// public abstract bool CapitalSpacing { get; } ////// Feature adjusting punctuation types of characters to the case of the surrounding glyphs /// public abstract bool CaseSensitiveForms { get; } ////// Font specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet1 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet2 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet3 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet4 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet5 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet6 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet7 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet8 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet9 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet10 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet11 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet12 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet13 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet14 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet15 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet16 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet17 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet18 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet19 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet20 { get; } ////// Substitute nominal zero with slashed zero. /// public abstract bool SlashedZero { get; } ////// Substitute regular greek forms with forms used in mathematical notation. /// public abstract bool MathematicalGreek { get; } ////// Replace standard forms in Japaneese fonts with preferred typographic forms /// public abstract bool EastAsianExpertForms { get; } ////// Render different types of typographic variations. Include Normal, Subscript, Superscript, /// Inferior, Ordinal and Ruby. /// public abstract FontVariants Variants { get; } ////// Select set of `capital forms from Normal, SmallCaps, AllSmallCaps, /// PetiteCaps, AllPetiteCaps, Unicase and Titling. /// public abstract FontCapitals ----s { get; } ////// Feature selecting special fractional forms of nominator, denominator and slash. /// public abstract FontFraction Fraction { get; } ////// Select set of glyphs to render alternate numeral forms from Normal, OldStyle and Lining /// public abstract FontNumeralStyle NumeralStyle { get; } ////// Select glyph set for different numeral aligning options from Default, Proportional and Tabular /// public abstract FontNumeralAlignment NumeralAlignment { get; } ////// Select from different width styles for Latin characters in East Asian fonts. /// public abstract FontEastAsianWidths EastAsianWidths { get; } ////// Select glyphs forms specific for particular writing system and language. /// public abstract FontEastAsianLanguage EastAsianLanguage { get; } ////// Select glyph forms having swashes by specified index. /// Examples: Q with tail extended under following letter /// public abstract int StandardSwashes { get; } ////// Select swash forms of glyphs by specified index and based on surrounding characters. /// Examples: d with flourish occupying space above following ea. /// public abstract int ContextualSwashes { get; } ////// Select alternate form of glyphs by specified index. /// Examples: multiple forms of ampersand. /// public abstract int StylisticAlternates { get; } ////// Forms commonly used in notation. /// Examples: characters placed in circles, parentheses /// public abstract int AnnotationAlternates { get; } #endregion Public typography properties ////// Should be called every time any property changes it's value /// protected void OnPropertiesChanged() { _features = null; } ////// Returns cached feature array. /// internal void GetCachedFeatureSet(out Feature[] features, out int featureCount) { features = _features; featureCount = _featureCount; } ////// Set cached feature array. /// internal void SetCachedFeatureSet(Feature[] features, int featureCount) { _features = features; _featureCount = featureCount; } private Feature[] _features; private int _featureCount; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation // // File: TextRunTypographyProperties.cs // // Contents: Text run Typography properties // // Spec: http://team/sites/Avalon/Specs/Text%20Formatting%20API.doc // // Created: 1-7-2005 Sergey Malkin (SergeyM) // //----------------------------------------------------------------------- using System; using System.Windows; using MS.Internal.Shaping; namespace System.Windows.Media.TextFormatting { ////// Text run typography properties /// /// Client set properties to generate set of features /// that will be processed by OpenType layout engine. /// /// For details see OpenType font specification at /// http://www.microsoft.com/typography/ /// /// public abstract class TextRunTypographyProperties { #region Public typography properties ////// Common ligatures assisting with text readability. /// Examples: fi, fl ligatures /// public abstract bool StandardLigatures { get; } ////// Ligature forms depending on surrounding context /// public abstract bool ContextualLigatures { get; } ////// Additional ligatures to assist with text readability /// Examples: Qu, Th /// public abstract bool DiscretionaryLigatures { get; } ////// Ligatures used in historical typography /// Examples: ct, st /// public abstract bool HistoricalLigatures { get; } ////// Custom forms defined by surrounding context /// Examples: multiple medial forms in Urdu Nastaliq fonts /// public abstract bool ContextualAlternates { get; } ////// Forms commonly used in the past /// Examples: long s, old Fraktur k /// public abstract bool HistoricalForms { get; } ////// Feature adjusting spacing between charactersto enchance word shape /// public abstract bool Kerning { get; } ////// Feature adjusting inter-glyph spacing to provide better readability for all-`capital text /// public abstract bool CapitalSpacing { get; } ////// Feature adjusting punctuation types of characters to the case of the surrounding glyphs /// public abstract bool CaseSensitiveForms { get; } ////// Font specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet1 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet2 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet3 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet4 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet5 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet6 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet7 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet8 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet9 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet10 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet11 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet12 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet13 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet14 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet15 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet16 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet17 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet18 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet19 { get; } ////// Font-specific set of glyph forms designed to work with each other. /// public abstract bool StylisticSet20 { get; } ////// Substitute nominal zero with slashed zero. /// public abstract bool SlashedZero { get; } ////// Substitute regular greek forms with forms used in mathematical notation. /// public abstract bool MathematicalGreek { get; } ////// Replace standard forms in Japaneese fonts with preferred typographic forms /// public abstract bool EastAsianExpertForms { get; } ////// Render different types of typographic variations. Include Normal, Subscript, Superscript, /// Inferior, Ordinal and Ruby. /// public abstract FontVariants Variants { get; } ////// Select set of `capital forms from Normal, SmallCaps, AllSmallCaps, /// PetiteCaps, AllPetiteCaps, Unicase and Titling. /// public abstract FontCapitals ----s { get; } ////// Feature selecting special fractional forms of nominator, denominator and slash. /// public abstract FontFraction Fraction { get; } ////// Select set of glyphs to render alternate numeral forms from Normal, OldStyle and Lining /// public abstract FontNumeralStyle NumeralStyle { get; } ////// Select glyph set for different numeral aligning options from Default, Proportional and Tabular /// public abstract FontNumeralAlignment NumeralAlignment { get; } ////// Select from different width styles for Latin characters in East Asian fonts. /// public abstract FontEastAsianWidths EastAsianWidths { get; } ////// Select glyphs forms specific for particular writing system and language. /// public abstract FontEastAsianLanguage EastAsianLanguage { get; } ////// Select glyph forms having swashes by specified index. /// Examples: Q with tail extended under following letter /// public abstract int StandardSwashes { get; } ////// Select swash forms of glyphs by specified index and based on surrounding characters. /// Examples: d with flourish occupying space above following ea. /// public abstract int ContextualSwashes { get; } ////// Select alternate form of glyphs by specified index. /// Examples: multiple forms of ampersand. /// public abstract int StylisticAlternates { get; } ////// Forms commonly used in notation. /// Examples: characters placed in circles, parentheses /// public abstract int AnnotationAlternates { get; } #endregion Public typography properties ////// Should be called every time any property changes it's value /// protected void OnPropertiesChanged() { _features = null; } ////// Returns cached feature array. /// internal void GetCachedFeatureSet(out Feature[] features, out int featureCount) { features = _features; featureCount = _featureCount; } ////// Set cached feature array. /// internal void SetCachedFeatureSet(Feature[] features, int featureCount) { _features = features; _featureCount = featureCount; } private Feature[] _features; private int _featureCount; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
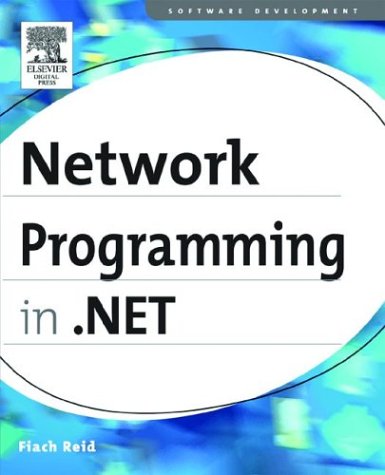
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ImpersonationContext.cs
- TabItemAutomationPeer.cs
- PeerApplicationLaunchInfo.cs
- SynchronizedInputProviderWrapper.cs
- TimeSpanParse.cs
- SplineKeyFrames.cs
- XmlCharCheckingReader.cs
- BasicHttpMessageCredentialType.cs
- NameValuePair.cs
- PageAdapter.cs
- PersonalizationDictionary.cs
- SelectedDatesCollection.cs
- ResourceProviderFactory.cs
- MemberDomainMap.cs
- XmlTextWriter.cs
- RectangleGeometry.cs
- _Semaphore.cs
- TTSEngineTypes.cs
- WebPartDisplayModeCollection.cs
- ComponentCommands.cs
- documentation.cs
- KeyFrames.cs
- DataRelationPropertyDescriptor.cs
- OleDbException.cs
- EndPoint.cs
- Polyline.cs
- MethodToken.cs
- StylusPointDescription.cs
- PageSetupDialog.cs
- RequestCache.cs
- PointAnimationUsingKeyFrames.cs
- JsonGlobals.cs
- CipherData.cs
- SharedUtils.cs
- ObjectSet.cs
- Timeline.cs
- GridPattern.cs
- ToolStripCodeDomSerializer.cs
- QueryOpeningEnumerator.cs
- ScriptBehaviorDescriptor.cs
- GridItemCollection.cs
- DisableDpiAwarenessAttribute.cs
- DirectoryNotFoundException.cs
- DataSetFieldSchema.cs
- TextEditorThreadLocalStore.cs
- DataTableTypeConverter.cs
- ViewStateException.cs
- EditorZone.cs
- ColumnHeaderConverter.cs
- ThreadPool.cs
- AssemblyFilter.cs
- MyContact.cs
- ServiceReference.cs
- EncoderReplacementFallback.cs
- WebAdminConfigurationHelper.cs
- SQLSingle.cs
- ControlTemplate.cs
- TrackingConditionCollection.cs
- SortedList.cs
- ConsoleCancelEventArgs.cs
- AppDomainAttributes.cs
- TypeDescriptionProvider.cs
- NestedContainer.cs
- BitmapEffectState.cs
- WebPartsSection.cs
- DataComponentNameHandler.cs
- SubMenuStyle.cs
- _NetRes.cs
- HttpDebugHandler.cs
- EntityClientCacheKey.cs
- ControlIdConverter.cs
- ShaderRenderModeValidation.cs
- BaseParaClient.cs
- DecoderFallbackWithFailureFlag.cs
- EdmTypeAttribute.cs
- FormsAuthenticationConfiguration.cs
- CalendarAutoFormatDialog.cs
- EncoderExceptionFallback.cs
- RoutingSection.cs
- BindingList.cs
- DigitalSignature.cs
- HtmlEmptyTagControlBuilder.cs
- BitmapEditor.cs
- EditorPart.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- SelectedDatesCollection.cs
- GridItemProviderWrapper.cs
- BamlLocalizableResource.cs
- Publisher.cs
- UnsafeNativeMethods.cs
- TextTreeText.cs
- ObjectToIdCache.cs
- XmlQueryRuntime.cs
- DefaultEventAttribute.cs
- SoapException.cs
- BitmapCodecInfo.cs
- FlowPosition.cs
- GridView.cs
- CheckedListBox.cs
- DesignParameter.cs