Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Framework / System / Windows / Markup / Localizer / BamlLocalizableResource.cs / 1 / BamlLocalizableResource.cs
//------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizableResource.cs // // Contents: BamlLocalizableResource class, part of Baml Localization API // // Created: 3/4/2004 garyyang // History: 8/3/2004 garyyang Move to System.Windows namespace // 11/29/2004 garyyang Move to System.Windows.Markup.Localization namespace // 03/24/2005 Garyyang Move to System.Windows.Markup.Localizer namespace // //----------------------------------------------------------------------- using System; using System.Windows; using MS.Internal; using System.Diagnostics; namespace System.Windows.Markup.Localizer { ////// Localization resource in Baml /// public class BamlLocalizableResource { //-------------------------------- // constructor //-------------------------------- ////// Constructor of LocalizableResource /// public BamlLocalizableResource() : this ( null, null, LocalizationCategory.None, true, true ) { } ////// Constructor of LocalizableResource /// public BamlLocalizableResource( string content, string comments, LocalizationCategory category, bool modifiable, bool readable ) { _content = content; _comments = comments; _category = category; Modifiable = modifiable; Readable = readable; } ////// constructor that creates a deep copy of the other localizable resource /// /// the other localizale resource internal BamlLocalizableResource(BamlLocalizableResource other) { Debug.Assert(other != null); _content = other._content; _comments = other._comments; _flags = other._flags; _category = other._category; } //--------------------------------- // public properties //--------------------------------- ////// The localizable value /// public string Content { get { return _content; } set { _content = value; } } ////// The localization comments /// public string Comments { get { return _comments; } set { _comments = value; } } ////// Localization Lock by developer /// public bool Modifiable { get { return (_flags & LocalizationFlags.Modifiable) > 0; } set { if (value) { _flags |= LocalizationFlags.Modifiable; } else { _flags &= (~LocalizationFlags.Modifiable); } } } ////// Visibility of the resource for translation /// public bool Readable { get { return (_flags & LocalizationFlags.Readable) > 0; } set { if (value) { _flags |= LocalizationFlags.Readable; } else { _flags &= (~LocalizationFlags.Readable); } } } ////// String category of the resource /// public LocalizationCategory Category { get { return _category; } set { _category = value; } } ////// compare equality /// public override bool Equals(object other) { BamlLocalizableResource otherResource = other as BamlLocalizableResource; if (otherResource == null) return false; return (_content == otherResource._content && _comments == otherResource._comments && _flags == otherResource._flags && _category == otherResource._category); } //////Return the hashcode. /// public override int GetHashCode() { return (_content == null ? 0 : _content.GetHashCode()) ^(_comments == null ? 0 : _comments.GetHashCode()) ^ (int) _flags ^ (int) _category; } //--------------------------------- // private members //--------------------------------- private string _content; private string _comments; private LocalizationFlags _flags; private LocalizationCategory _category; //--------------------------------- // Private type //--------------------------------- [Flags] private enum LocalizationFlags : byte { Readable = 1, Modifiable = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------ // // Microsoft Windows Client Platform // Copyright (C) Microsoft Corporation, 2001 // // File: BamlLocalizableResource.cs // // Contents: BamlLocalizableResource class, part of Baml Localization API // // Created: 3/4/2004 garyyang // History: 8/3/2004 garyyang Move to System.Windows namespace // 11/29/2004 garyyang Move to System.Windows.Markup.Localization namespace // 03/24/2005 Garyyang Move to System.Windows.Markup.Localizer namespace // //----------------------------------------------------------------------- using System; using System.Windows; using MS.Internal; using System.Diagnostics; namespace System.Windows.Markup.Localizer { ////// Localization resource in Baml /// public class BamlLocalizableResource { //-------------------------------- // constructor //-------------------------------- ////// Constructor of LocalizableResource /// public BamlLocalizableResource() : this ( null, null, LocalizationCategory.None, true, true ) { } ////// Constructor of LocalizableResource /// public BamlLocalizableResource( string content, string comments, LocalizationCategory category, bool modifiable, bool readable ) { _content = content; _comments = comments; _category = category; Modifiable = modifiable; Readable = readable; } ////// constructor that creates a deep copy of the other localizable resource /// /// the other localizale resource internal BamlLocalizableResource(BamlLocalizableResource other) { Debug.Assert(other != null); _content = other._content; _comments = other._comments; _flags = other._flags; _category = other._category; } //--------------------------------- // public properties //--------------------------------- ////// The localizable value /// public string Content { get { return _content; } set { _content = value; } } ////// The localization comments /// public string Comments { get { return _comments; } set { _comments = value; } } ////// Localization Lock by developer /// public bool Modifiable { get { return (_flags & LocalizationFlags.Modifiable) > 0; } set { if (value) { _flags |= LocalizationFlags.Modifiable; } else { _flags &= (~LocalizationFlags.Modifiable); } } } ////// Visibility of the resource for translation /// public bool Readable { get { return (_flags & LocalizationFlags.Readable) > 0; } set { if (value) { _flags |= LocalizationFlags.Readable; } else { _flags &= (~LocalizationFlags.Readable); } } } ////// String category of the resource /// public LocalizationCategory Category { get { return _category; } set { _category = value; } } ////// compare equality /// public override bool Equals(object other) { BamlLocalizableResource otherResource = other as BamlLocalizableResource; if (otherResource == null) return false; return (_content == otherResource._content && _comments == otherResource._comments && _flags == otherResource._flags && _category == otherResource._category); } //////Return the hashcode. /// public override int GetHashCode() { return (_content == null ? 0 : _content.GetHashCode()) ^(_comments == null ? 0 : _comments.GetHashCode()) ^ (int) _flags ^ (int) _category; } //--------------------------------- // private members //--------------------------------- private string _content; private string _comments; private LocalizationFlags _flags; private LocalizationCategory _category; //--------------------------------- // Private type //--------------------------------- [Flags] private enum LocalizationFlags : byte { Readable = 1, Modifiable = 2, } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
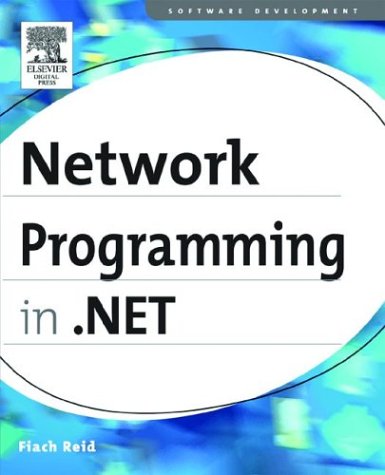
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DispatcherHooks.cs
- MessageQueue.cs
- Button.cs
- Literal.cs
- SplineQuaternionKeyFrame.cs
- MultipleViewPatternIdentifiers.cs
- Slider.cs
- FieldInfo.cs
- GeneralTransform3D.cs
- XpsDocumentEvent.cs
- ExpressionVisitor.cs
- SplayTreeNode.cs
- FormViewDesigner.cs
- DataGridCell.cs
- XmlEncodedRawTextWriter.cs
- TextFindEngine.cs
- TCPClient.cs
- QueryableDataSource.cs
- TextBox.cs
- TableCell.cs
- Utils.cs
- IPAddressCollection.cs
- InternalPermissions.cs
- IMembershipProvider.cs
- OdbcCommand.cs
- Tablet.cs
- SafeHandle.cs
- ValueOfAction.cs
- OleDbException.cs
- ListChangedEventArgs.cs
- GreaterThanOrEqual.cs
- NetWebProxyFinder.cs
- RightsManagementUser.cs
- NotificationContext.cs
- TableAutomationPeer.cs
- XmlWriter.cs
- CompilerGlobalScopeAttribute.cs
- PartManifestEntry.cs
- TraceContext.cs
- DataServiceRequestOfT.cs
- DataColumnMapping.cs
- IdentifierService.cs
- ResponseBodyWriter.cs
- ImageCodecInfoPrivate.cs
- DashStyle.cs
- WebPartsPersonalization.cs
- _BufferOffsetSize.cs
- DesignerResources.cs
- path.cs
- login.cs
- Canvas.cs
- SystemInformation.cs
- Part.cs
- EntityDataSourceDataSelection.cs
- ConnectionManagementElementCollection.cs
- XmlWriterSettings.cs
- ArithmeticException.cs
- PlaceHolder.cs
- ControlBuilderAttribute.cs
- GridViewHeaderRowPresenter.cs
- DataColumnMapping.cs
- ToolStripComboBox.cs
- BrowserCapabilitiesCompiler.cs
- InputMethodStateChangeEventArgs.cs
- AuthenticationModuleElement.cs
- NativeMethods.cs
- HttpResponse.cs
- COM2TypeInfoProcessor.cs
- Point3DIndependentAnimationStorage.cs
- DrawListViewSubItemEventArgs.cs
- MultiSelectRootGridEntry.cs
- UIElement.cs
- QueryAccessibilityHelpEvent.cs
- IntSecurity.cs
- DrawingAttributesDefaultValueFactory.cs
- ItemCheckedEvent.cs
- SpecialTypeDataContract.cs
- ConnectionStringsExpressionBuilder.cs
- CompoundFileDeflateTransform.cs
- XmlCountingReader.cs
- ExceptionUtil.cs
- ComplexPropertyEntry.cs
- Control.cs
- TableRowCollection.cs
- ICspAsymmetricAlgorithm.cs
- LambdaCompiler.Logical.cs
- DateTimeConstantAttribute.cs
- WebBaseEventKeyComparer.cs
- MessageSecurityOverTcp.cs
- TimeZone.cs
- InputReportEventArgs.cs
- BypassElement.cs
- SecurityProtocolFactory.cs
- HuffModule.cs
- CompletedAsyncResult.cs
- SizeAnimationClockResource.cs
- ContextMenuAutomationPeer.cs
- ArraySegment.cs
- AccessKeyManager.cs
- Bookmark.cs