Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / DataServiceHostWrapper.cs / 1458001 / DataServiceHostWrapper.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides the wrapper class for DataService hosts. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Collections.Generic; using System.IO; using System.Net; using System.Diagnostics; #endregion Namespaces. ////// Enum to represent various http methods /// internal enum AstoriaVerbs { #region Values. ///Not Initialized. None, ///Represents the GET http method. GET, ///Represents the PUT http method. PUT, ///Represents the POST http method. POST, ///Represents the DELETE http method. DELETE, ///Represents the MERGE http method. MERGE #endregion Values. } ////// Provides access to the environment for a DataService, /// including information about the current request. /// internal class DataServiceHostWrapper { #region Private Fields ///Reference to the IDataServiceHost object we are wrapping private readonly IDataServiceHost host; ///Gets a comma-separated list of client-supported MIME Accept types. private readonly string requestAccept; ///Gets the string with the specification for the character set encoding that the client requested, possibly null. private readonly string requestAcceptCharSet; ///Gets the HTTP MIME type of the request stream. private readonly string requestContentType; ///Gets the value of the If-Match header from the request made private readonly string requestIfMatch; ///Gets the value of the If-None-Match header from the request made private readonly string requestIfNoneMatch; ///Gets the value for the MaxDataServiceVersion request header. private readonly string requestMaxVersion; ///Gets the value for the DataServiceVersion request header. private readonly string requestVersion; ////// Get the enum representing the http method name. /// We have this for perf reason since enum comparison is faster than string comparison. /// private AstoriaVerbs astoriaHttpVerb; ///Gets the HTTP data transfer method (such as GET, POST, or HEAD) used by the client. private string requestHttpMethod; ///Gets the absolute URI to the resource upon which to apply the request. private Uri absoluteRequestUri; ///Gets the absolute URI to the service. private Uri absoluteServiceUri; ///Gets the private Stream requestStream; ///from which the input must be read to the client. Gets the private Stream responseStream; ///to be written to send a response to the client. Request headers private WebHeaderCollection requestHeaders; ///Response headers private WebHeaderCollection responseHeaders; #endregion Private Fields #region Constructor ////// Constructs an instance of the DataServiceHostWrapper object. /// /// IDataServiceHost instance to wrap. internal DataServiceHostWrapper(IDataServiceHost host) { Debug.Assert(host != null, "host != null"); this.host = host; this.astoriaHttpVerb = AstoriaVerbs.None; this.requestAccept = host.RequestAccept; this.requestAcceptCharSet = host.RequestAcceptCharSet; this.requestContentType = host.RequestContentType; this.requestIfMatch = host.RequestIfMatch; this.requestIfNoneMatch = host.RequestIfNoneMatch; this.requestMaxVersion = host.RequestMaxVersion; this.requestVersion = host.RequestVersion; } #endregion Constructor #region Properties. ////// Get the enum representing the http method name. /// /// http method used for the request. ///enum representing the http method name. internal AstoriaVerbs AstoriaHttpVerb { get { if (this.astoriaHttpVerb == AstoriaVerbs.None) { string requestMethod = this.RequestHttpMethod; Debug.Assert(!string.IsNullOrEmpty(requestMethod), "!string.IsNullOrEmpty(requestMethod)"); switch (requestMethod) { case XmlConstants.HttpMethodGet: this.astoriaHttpVerb = AstoriaVerbs.GET; break; case XmlConstants.HttpMethodPost: this.astoriaHttpVerb = AstoriaVerbs.POST; break; case XmlConstants.HttpMethodPut: this.astoriaHttpVerb = AstoriaVerbs.PUT; break; case XmlConstants.HttpMethodMerge: this.astoriaHttpVerb = AstoriaVerbs.MERGE; break; case XmlConstants.HttpMethodDelete: this.astoriaHttpVerb = AstoriaVerbs.DELETE; break; default: // 501: Not Implemented (rather than 405 - Method Not Allowed, // which implies it was understood and rejected). throw DataServiceException.CreateMethodNotImplemented(Strings.DataService_NotImplementedException); } } return this.astoriaHttpVerb; } } ///Gets the absolute resource upon which to apply the request. internal Uri AbsoluteRequestUri { get { if (this.absoluteRequestUri == null) { this.absoluteRequestUri = this.host.AbsoluteRequestUri; if (this.absoluteRequestUri == null) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteRequestUriCannotBeNull); } else if (!this.absoluteRequestUri.IsAbsoluteUri) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteRequestUriMustBeAbsolute); } } return this.absoluteRequestUri; } } ///Gets the absolute URI to the service. internal Uri AbsoluteServiceUri { get { if (this.absoluteServiceUri == null) { this.absoluteServiceUri = this.host.AbsoluteServiceUri; if (this.absoluteServiceUri == null) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteServiceUriCannotBeNull); } else if (!this.absoluteServiceUri.IsAbsoluteUri) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteServiceUriMustBeAbsolute); } } return this.absoluteServiceUri; } } ///Gets a comma-separated list of client-supported MIME Accept types. internal string RequestAccept { get { return this.requestAccept; } } ///Gets the string with the specification for the character set encoding that the client requested, possibly null. internal string RequestAcceptCharSet { get { return this.requestAcceptCharSet; } } ///Gets the HTTP MIME type of the request stream. internal string RequestContentType { get { return this.requestContentType; } } ///Gets the HTTP data transfer method (such as GET, POST, or HEAD) used by the client. internal string RequestHttpMethod { get { if (string.IsNullOrEmpty(this.requestHttpMethod)) { this.requestHttpMethod = this.host.RequestHttpMethod; if (String.IsNullOrEmpty(this.requestHttpMethod)) { throw new InvalidOperationException(Strings.DataServiceHost_EmptyHttpMethod); } } return this.requestHttpMethod; } } ///Gets the value of the If-Match header from the request made internal string RequestIfMatch { get { return this.requestIfMatch; } } ///Gets the value of the If-None-Match header from the request made internal string RequestIfNoneMatch { get { return this.requestIfNoneMatch; } } ///Gets the value for the MaxDataServiceVersion request header. internal string RequestMaxVersion { get { return this.requestMaxVersion; } } ///Gets the internal Stream RequestStream { get { if (this.requestStream == null) { this.requestStream = this.host.RequestStream; if (this.requestStream == null) { throw DataServiceException.CreateBadRequestError(Strings.BadRequest_NullRequestStream); } } return this.requestStream; } } ///from which the input must be read to the client. Gets the value for the DataServiceVersion request header. internal string RequestVersion { get { return this.requestVersion; } } ///Request headers internal WebHeaderCollection RequestHeaders { get { if (this.requestHeaders == null) { IDataServiceHost2 host2 = DataServiceHostWrapper.ValidateAndCast(this.host); this.requestHeaders = host2.RequestHeaders; if (this.requestHeaders == null) { throw new InvalidOperationException(Strings.DataServiceHost_RequestHeadersCannotBeNull); } } return this.requestHeaders; } } /// Response headers internal WebHeaderCollection ResponseHeaders { get { if (this.responseHeaders == null) { IDataServiceHost2 host2 = DataServiceHostWrapper.ValidateAndCast(this.host); this.responseHeaders = host2.ResponseHeaders; if (this.responseHeaders == null) { throw new InvalidOperationException(Strings.DataServiceHost_ResponseHeadersCannotBeNull); } } return this.responseHeaders; } } /// Gets or sets the Cache-Control header on the response. internal string ResponseCacheControl { // No up stream caller // get { return this.host.ResponseCacheControl; } set { this.host.ResponseCacheControl = value; } } ///Gets or sets the HTTP MIME type of the output stream. internal string ResponseContentType { get { return this.host.ResponseContentType; } set { this.host.ResponseContentType = value; } } ///Gets/Sets the value of the ETag header on the response internal string ResponseETag { get { return this.host.ResponseETag; } set { this.host.ResponseETag = value; } } ///Gets or sets the Location header on the response. internal string ResponseLocation { // No up stream caller // get { return this.host.ResponseLocation; } set { this.host.ResponseLocation = value; } } ////// Returns the status code for the request made /// internal int ResponseStatusCode { get { return this.host.ResponseStatusCode; } set { this.host.ResponseStatusCode = value; } } ///Gets the internal Stream ResponseStream { get { if (this.responseStream == null) { this.responseStream = this.host.ResponseStream; if (this.responseStream == null) { throw DataServiceException.CreateBadRequestError(Strings.BadRequest_NullResponseStream); } } return this.responseStream; } } ///to be written to send a response to the client. Gets or sets the value for the DataServiceVersion response header. internal string ResponseVersion { set { this.host.ResponseVersion = value; } } ///If the wrapped host is a HttpContextServiceHost, returns the host internal HttpContextServiceHost HttpContextServiceHost { get { return this.host as HttpContextServiceHost; } } ///If the wrapped host is a BatchServiceHost, returns the batch host internal BatchServiceHost BatchServiceHost { get { Debug.Assert(this.host is BatchServiceHost, "We shouldn't be calling this outside of the batching code path."); return this.host as BatchServiceHost; } } #endregion Properties. #region Methods. ///Gets the value for the specified item in the request query string. /// Item to return. ////// The value for the specified item in the request query string; /// null if internal string GetQueryStringItem(string item) { return this.host.GetQueryStringItem(item); } ///is not found. /// Method to handle a data service exception during processing. /// Exception handling description. internal void ProcessException(HandleExceptionArgs args) { this.host.ProcessException(args); } ///Verifies that query parameters are valid. internal void VerifyQueryParameters() { if (this.host is HttpContextServiceHost) { ((HttpContextServiceHost)this.host).VerifyQueryParameters(); } } ///Checks whether the given object instance is of type T. /// object instance. ///type which we need to cast the given instance to. ///Returns strongly typed instance of T, if the given object is an instance of type T. ///If the given object is not of Type T. private static T ValidateAndCast(object instance) where T : class { T instanceAsT = instance as T; if (instanceAsT == null) { throw new InvalidOperationException(Strings.DataServiceException_GeneralError); } return instanceAsT; } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides the wrapper class for DataService hosts. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services { #region Namespaces. using System; using System.Collections.Generic; using System.IO; using System.Net; using System.Diagnostics; #endregion Namespaces. ////// Enum to represent various http methods /// internal enum AstoriaVerbs { #region Values. ///Not Initialized. None, ///Represents the GET http method. GET, ///Represents the PUT http method. PUT, ///Represents the POST http method. POST, ///Represents the DELETE http method. DELETE, ///Represents the MERGE http method. MERGE #endregion Values. } ////// Provides access to the environment for a DataService, /// including information about the current request. /// internal class DataServiceHostWrapper { #region Private Fields ///Reference to the IDataServiceHost object we are wrapping private readonly IDataServiceHost host; ///Gets a comma-separated list of client-supported MIME Accept types. private readonly string requestAccept; ///Gets the string with the specification for the character set encoding that the client requested, possibly null. private readonly string requestAcceptCharSet; ///Gets the HTTP MIME type of the request stream. private readonly string requestContentType; ///Gets the value of the If-Match header from the request made private readonly string requestIfMatch; ///Gets the value of the If-None-Match header from the request made private readonly string requestIfNoneMatch; ///Gets the value for the MaxDataServiceVersion request header. private readonly string requestMaxVersion; ///Gets the value for the DataServiceVersion request header. private readonly string requestVersion; ////// Get the enum representing the http method name. /// We have this for perf reason since enum comparison is faster than string comparison. /// private AstoriaVerbs astoriaHttpVerb; ///Gets the HTTP data transfer method (such as GET, POST, or HEAD) used by the client. private string requestHttpMethod; ///Gets the absolute URI to the resource upon which to apply the request. private Uri absoluteRequestUri; ///Gets the absolute URI to the service. private Uri absoluteServiceUri; ///Gets the private Stream requestStream; ///from which the input must be read to the client. Gets the private Stream responseStream; ///to be written to send a response to the client. Request headers private WebHeaderCollection requestHeaders; ///Response headers private WebHeaderCollection responseHeaders; #endregion Private Fields #region Constructor ////// Constructs an instance of the DataServiceHostWrapper object. /// /// IDataServiceHost instance to wrap. internal DataServiceHostWrapper(IDataServiceHost host) { Debug.Assert(host != null, "host != null"); this.host = host; this.astoriaHttpVerb = AstoriaVerbs.None; this.requestAccept = host.RequestAccept; this.requestAcceptCharSet = host.RequestAcceptCharSet; this.requestContentType = host.RequestContentType; this.requestIfMatch = host.RequestIfMatch; this.requestIfNoneMatch = host.RequestIfNoneMatch; this.requestMaxVersion = host.RequestMaxVersion; this.requestVersion = host.RequestVersion; } #endregion Constructor #region Properties. ////// Get the enum representing the http method name. /// /// http method used for the request. ///enum representing the http method name. internal AstoriaVerbs AstoriaHttpVerb { get { if (this.astoriaHttpVerb == AstoriaVerbs.None) { string requestMethod = this.RequestHttpMethod; Debug.Assert(!string.IsNullOrEmpty(requestMethod), "!string.IsNullOrEmpty(requestMethod)"); switch (requestMethod) { case XmlConstants.HttpMethodGet: this.astoriaHttpVerb = AstoriaVerbs.GET; break; case XmlConstants.HttpMethodPost: this.astoriaHttpVerb = AstoriaVerbs.POST; break; case XmlConstants.HttpMethodPut: this.astoriaHttpVerb = AstoriaVerbs.PUT; break; case XmlConstants.HttpMethodMerge: this.astoriaHttpVerb = AstoriaVerbs.MERGE; break; case XmlConstants.HttpMethodDelete: this.astoriaHttpVerb = AstoriaVerbs.DELETE; break; default: // 501: Not Implemented (rather than 405 - Method Not Allowed, // which implies it was understood and rejected). throw DataServiceException.CreateMethodNotImplemented(Strings.DataService_NotImplementedException); } } return this.astoriaHttpVerb; } } ///Gets the absolute resource upon which to apply the request. internal Uri AbsoluteRequestUri { get { if (this.absoluteRequestUri == null) { this.absoluteRequestUri = this.host.AbsoluteRequestUri; if (this.absoluteRequestUri == null) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteRequestUriCannotBeNull); } else if (!this.absoluteRequestUri.IsAbsoluteUri) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteRequestUriMustBeAbsolute); } } return this.absoluteRequestUri; } } ///Gets the absolute URI to the service. internal Uri AbsoluteServiceUri { get { if (this.absoluteServiceUri == null) { this.absoluteServiceUri = this.host.AbsoluteServiceUri; if (this.absoluteServiceUri == null) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteServiceUriCannotBeNull); } else if (!this.absoluteServiceUri.IsAbsoluteUri) { throw new InvalidOperationException(Strings.RequestUriProcessor_AbsoluteServiceUriMustBeAbsolute); } } return this.absoluteServiceUri; } } ///Gets a comma-separated list of client-supported MIME Accept types. internal string RequestAccept { get { return this.requestAccept; } } ///Gets the string with the specification for the character set encoding that the client requested, possibly null. internal string RequestAcceptCharSet { get { return this.requestAcceptCharSet; } } ///Gets the HTTP MIME type of the request stream. internal string RequestContentType { get { return this.requestContentType; } } ///Gets the HTTP data transfer method (such as GET, POST, or HEAD) used by the client. internal string RequestHttpMethod { get { if (string.IsNullOrEmpty(this.requestHttpMethod)) { this.requestHttpMethod = this.host.RequestHttpMethod; if (String.IsNullOrEmpty(this.requestHttpMethod)) { throw new InvalidOperationException(Strings.DataServiceHost_EmptyHttpMethod); } } return this.requestHttpMethod; } } ///Gets the value of the If-Match header from the request made internal string RequestIfMatch { get { return this.requestIfMatch; } } ///Gets the value of the If-None-Match header from the request made internal string RequestIfNoneMatch { get { return this.requestIfNoneMatch; } } ///Gets the value for the MaxDataServiceVersion request header. internal string RequestMaxVersion { get { return this.requestMaxVersion; } } ///Gets the internal Stream RequestStream { get { if (this.requestStream == null) { this.requestStream = this.host.RequestStream; if (this.requestStream == null) { throw DataServiceException.CreateBadRequestError(Strings.BadRequest_NullRequestStream); } } return this.requestStream; } } ///from which the input must be read to the client. Gets the value for the DataServiceVersion request header. internal string RequestVersion { get { return this.requestVersion; } } ///Request headers internal WebHeaderCollection RequestHeaders { get { if (this.requestHeaders == null) { IDataServiceHost2 host2 = DataServiceHostWrapper.ValidateAndCast(this.host); this.requestHeaders = host2.RequestHeaders; if (this.requestHeaders == null) { throw new InvalidOperationException(Strings.DataServiceHost_RequestHeadersCannotBeNull); } } return this.requestHeaders; } } /// Response headers internal WebHeaderCollection ResponseHeaders { get { if (this.responseHeaders == null) { IDataServiceHost2 host2 = DataServiceHostWrapper.ValidateAndCast(this.host); this.responseHeaders = host2.ResponseHeaders; if (this.responseHeaders == null) { throw new InvalidOperationException(Strings.DataServiceHost_ResponseHeadersCannotBeNull); } } return this.responseHeaders; } } /// Gets or sets the Cache-Control header on the response. internal string ResponseCacheControl { // No up stream caller // get { return this.host.ResponseCacheControl; } set { this.host.ResponseCacheControl = value; } } ///Gets or sets the HTTP MIME type of the output stream. internal string ResponseContentType { get { return this.host.ResponseContentType; } set { this.host.ResponseContentType = value; } } ///Gets/Sets the value of the ETag header on the response internal string ResponseETag { get { return this.host.ResponseETag; } set { this.host.ResponseETag = value; } } ///Gets or sets the Location header on the response. internal string ResponseLocation { // No up stream caller // get { return this.host.ResponseLocation; } set { this.host.ResponseLocation = value; } } ////// Returns the status code for the request made /// internal int ResponseStatusCode { get { return this.host.ResponseStatusCode; } set { this.host.ResponseStatusCode = value; } } ///Gets the internal Stream ResponseStream { get { if (this.responseStream == null) { this.responseStream = this.host.ResponseStream; if (this.responseStream == null) { throw DataServiceException.CreateBadRequestError(Strings.BadRequest_NullResponseStream); } } return this.responseStream; } } ///to be written to send a response to the client. Gets or sets the value for the DataServiceVersion response header. internal string ResponseVersion { set { this.host.ResponseVersion = value; } } ///If the wrapped host is a HttpContextServiceHost, returns the host internal HttpContextServiceHost HttpContextServiceHost { get { return this.host as HttpContextServiceHost; } } ///If the wrapped host is a BatchServiceHost, returns the batch host internal BatchServiceHost BatchServiceHost { get { Debug.Assert(this.host is BatchServiceHost, "We shouldn't be calling this outside of the batching code path."); return this.host as BatchServiceHost; } } #endregion Properties. #region Methods. ///Gets the value for the specified item in the request query string. /// Item to return. ////// The value for the specified item in the request query string; /// null if internal string GetQueryStringItem(string item) { return this.host.GetQueryStringItem(item); } ///is not found. /// Method to handle a data service exception during processing. /// Exception handling description. internal void ProcessException(HandleExceptionArgs args) { this.host.ProcessException(args); } ///Verifies that query parameters are valid. internal void VerifyQueryParameters() { if (this.host is HttpContextServiceHost) { ((HttpContextServiceHost)this.host).VerifyQueryParameters(); } } ///Checks whether the given object instance is of type T. /// object instance. ///type which we need to cast the given instance to. ///Returns strongly typed instance of T, if the given object is an instance of type T. ///If the given object is not of Type T. private static T ValidateAndCast(object instance) where T : class { T instanceAsT = instance as T; if (instanceAsT == null) { throw new InvalidOperationException(Strings.DataServiceException_GeneralError); } return instanceAsT; } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
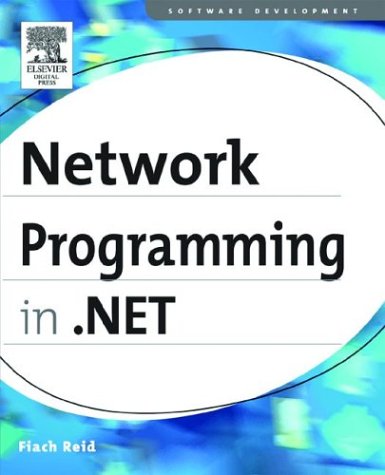
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ObjectListCommandCollection.cs
- ZoomingMessageFilter.cs
- ThreadSafeList.cs
- ExpressionConverter.cs
- ContainerControl.cs
- TextAutomationPeer.cs
- SoapSchemaMember.cs
- TreeNode.cs
- XsdDuration.cs
- CodeMemberField.cs
- UriTemplateEquivalenceComparer.cs
- Rect3D.cs
- TextChange.cs
- TextServicesCompartmentContext.cs
- PropertyPushdownHelper.cs
- SmtpReplyReaderFactory.cs
- CollectionMarkupSerializer.cs
- ReceiveDesigner.xaml.cs
- EmptyEnumerable.cs
- CallSiteOps.cs
- ComponentResourceManager.cs
- CancelEventArgs.cs
- ContainerFilterService.cs
- WebConfigurationFileMap.cs
- QilNode.cs
- VerticalAlignConverter.cs
- UIElementHelper.cs
- UDPClient.cs
- oledbmetadatacolumnnames.cs
- COM2Properties.cs
- HttpConfigurationContext.cs
- MasterPageCodeDomTreeGenerator.cs
- CancellableEnumerable.cs
- ThreadExceptionEvent.cs
- ContextStaticAttribute.cs
- DynamicValueConverter.cs
- MdImport.cs
- OdbcConnectionString.cs
- Inline.cs
- LinqDataSourceUpdateEventArgs.cs
- EventSinkHelperWriter.cs
- PaintEvent.cs
- StreamReader.cs
- GridSplitterAutomationPeer.cs
- NumericUpDown.cs
- DescendentsWalkerBase.cs
- FunctionQuery.cs
- EastAsianLunisolarCalendar.cs
- ConnectionStringEditor.cs
- SamlAttributeStatement.cs
- HostnameComparisonMode.cs
- EncoderFallback.cs
- Executor.cs
- ClientSession.cs
- RuntimeComponentFilter.cs
- CatalogZone.cs
- WebPartEditVerb.cs
- input.cs
- FloaterParaClient.cs
- ToolStripItem.cs
- XmlBoundElement.cs
- EventMappingSettings.cs
- KerberosSecurityTokenProvider.cs
- DiscoveryClientElement.cs
- ThreadStaticAttribute.cs
- PropertyRecord.cs
- StorageTypeMapping.cs
- SymDocumentType.cs
- SolidColorBrush.cs
- KerberosSecurityTokenParameters.cs
- Timeline.cs
- ComponentDispatcher.cs
- DataIdProcessor.cs
- ToolStripButton.cs
- LayoutExceptionEventArgs.cs
- GridErrorDlg.cs
- BamlLocalizableResource.cs
- ConfigurationLocationCollection.cs
- Msec.cs
- Matrix3D.cs
- DropTarget.cs
- Form.cs
- ParameterSubsegment.cs
- Line.cs
- ThreadStaticAttribute.cs
- StringWriter.cs
- PropertyTabAttribute.cs
- EntityContainerEmitter.cs
- mda.cs
- RadioButtonBaseAdapter.cs
- ScriptResourceAttribute.cs
- SplitterCancelEvent.cs
- HuffModule.cs
- ThrowHelper.cs
- ElementHostAutomationPeer.cs
- Task.cs
- SqlFunctionAttribute.cs
- ConstantSlot.cs
- DiagnosticTrace.cs
- SizeConverter.cs