Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / MultidimensionalArrayItemReference.cs / 1305376 / MultidimensionalArrayItemReference.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Expressions { using System.Collections.ObjectModel; using System.ComponentModel; using System.Runtime; using System.Runtime.Collections; using System.Runtime.Serialization; using System.Windows.Markup; [ContentProperty("Indices")] public sealed class MultidimensionalArrayItemReference: CodeActivity > { Collection > indices; [RequiredArgument] [DefaultValue(null)] public InArgument Array { get; set; } [DefaultValue(null)] public Collection > Indices { get { if (this.indices == null) { this.indices = new ValidatingCollection > { // disallow null values OnAddValidationCallback = item => { if (item == null) { throw FxTrace.Exception.ArgumentNull("item"); } }, }; } return this.indices; } } protected override void CacheMetadata(CodeActivityMetadata metadata) { if (this.Indices.Count == 0) { metadata.AddValidationError(SR.IndicesAreNeeded(this.GetType().Name, this.DisplayName)); } RuntimeArgument arrayArgument = new RuntimeArgument("Array", typeof(Array), ArgumentDirection.In, true); metadata.Bind(this.Array, arrayArgument); metadata.AddArgument(arrayArgument); for (int i = 0; i < this.Indices.Count; i++) { RuntimeArgument indexArgument = new RuntimeArgument("Index_" + i, typeof(int), ArgumentDirection.In, true); metadata.Bind(this.Indices[i], indexArgument); metadata.AddArgument(indexArgument); } RuntimeArgument resultArgument = new RuntimeArgument("Result", typeof(Location ), ArgumentDirection.Out); metadata.Bind(this.Result, resultArgument); metadata.AddArgument(resultArgument); } protected override Location Execute(CodeActivityContext context) { Array items = this.Array.Get(context); if (items == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.MemberCannotBeNull("Array", this.GetType().Name, this.DisplayName))); } Type realItemType = items.GetType().GetElementType(); if (!TypeHelper.AreTypesCompatible(typeof(TItem), realItemType)) { throw FxTrace.Exception.AsError(new InvalidCastException(SR.IncompatibleTypeForMultidimensionalArrayItemReference(typeof(TItem).Name, realItemType.Name))); } int[] itemIndex = new int[this.Indices.Count]; for (int i = 0; i < this.Indices.Count; i++) { itemIndex[i] = this.Indices[i].Get(context); } return new MultidimensionArrayLocation(items, itemIndex); } [DataContract] class MultidimensionArrayLocation : Location { [DataMember] Array array; [DataMember(EmitDefaultValue = false)] int[] indices; public MultidimensionArrayLocation(Array array, int[] indices) : base() { this.array = array; this.indices = indices; } public override TItem Value { get { return (TItem)this.array.GetValue(indices); } set { this.array.SetValue(value, indices); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
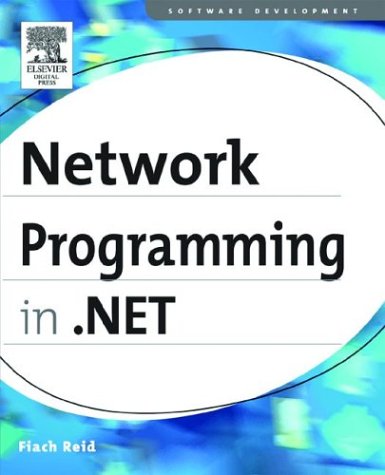
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SBCSCodePageEncoding.cs
- MailAddress.cs
- KnownBoxes.cs
- PolyBezierSegment.cs
- PaintValueEventArgs.cs
- BinaryKeyIdentifierClause.cs
- ListViewGroup.cs
- listitem.cs
- Logging.cs
- BmpBitmapEncoder.cs
- MulticastIPAddressInformationCollection.cs
- MultilineStringConverter.cs
- UrlEncodedParameterWriter.cs
- SelectingProviderEventArgs.cs
- QuarticEase.cs
- Misc.cs
- Line.cs
- MimeTypePropertyAttribute.cs
- ResumeStoryboard.cs
- PnrpPermission.cs
- XmlLanguage.cs
- CreationContext.cs
- DataGridViewSortCompareEventArgs.cs
- SerializationHelper.cs
- Lookup.cs
- TextTreeRootTextBlock.cs
- NavigationHelper.cs
- TraceProvider.cs
- WindowsListView.cs
- MdImport.cs
- TextServicesManager.cs
- SchemaDeclBase.cs
- DataGridViewEditingControlShowingEventArgs.cs
- EventMap.cs
- AlphabetConverter.cs
- List.cs
- xsdvalidator.cs
- WebPartsPersonalization.cs
- EditorServiceContext.cs
- WinFormsSecurity.cs
- UnaryExpression.cs
- SharedStatics.cs
- BaseTemplateCodeDomTreeGenerator.cs
- MsmqIntegrationElement.cs
- Paragraph.cs
- EntityDataSourceWizardForm.cs
- Control.cs
- Window.cs
- RemotingException.cs
- LingerOption.cs
- ToolStripItemImageRenderEventArgs.cs
- GraphicsContainer.cs
- translator.cs
- HScrollBar.cs
- ManagementPath.cs
- TimelineGroup.cs
- SqlInfoMessageEvent.cs
- IssuedTokenParametersElement.cs
- UTF8Encoding.cs
- AggregationMinMaxHelpers.cs
- PropertyFilterAttribute.cs
- SoapAttributeOverrides.cs
- PointLight.cs
- InkCanvasSelection.cs
- MappedMetaModel.cs
- RectKeyFrameCollection.cs
- SqlTransaction.cs
- BindToObject.cs
- FunctionMappingTranslator.cs
- ToolBarDesigner.cs
- ContainerUtilities.cs
- RegisteredScript.cs
- CellTreeNodeVisitors.cs
- PrintingPermission.cs
- Misc.cs
- Lasso.cs
- Utils.cs
- CookielessData.cs
- DataKeyArray.cs
- RepeaterCommandEventArgs.cs
- FontCacheLogic.cs
- RemoveStoryboard.cs
- XmlSchemaGroupRef.cs
- ExtendedPropertyCollection.cs
- CharStorage.cs
- DecimalConverter.cs
- EncodingDataItem.cs
- ClientRuntimeConfig.cs
- HMAC.cs
- TracePayload.cs
- DispatcherOperation.cs
- ClientUtils.cs
- ConstrainedDataObject.cs
- PersonalizationStateQuery.cs
- NeedSkipTokenVisitor.cs
- ControlParameter.cs
- NotifyParentPropertyAttribute.cs
- InputLanguageCollection.cs
- DomNameTable.cs
- ConnectionProviderAttribute.cs