Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / Internal / PropertyEditing / Editors / FlagPanel.cs / 1305376 / FlagPanel.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Internal.PropertyEditing.Editors { using System.Windows.Controls; using System.Windows.Data; using System.Globalization; using System.Windows; using System.Windows.Automation; using System.Runtime; sealed class FlagPanel : StackPanel { public static readonly DependencyProperty FlagStringProperty = DependencyProperty.Register("FlagString", typeof(string), typeof(FlagPanel), new PropertyMetadata(string.Empty)); public static readonly DependencyProperty FlagTypeProperty = DependencyProperty.Register( "FlagType", typeof(Type), typeof(FlagPanel), new PropertyMetadata(null, new PropertyChangedCallback(OnFlagTypeChanged))); public Type FlagType { get { return (Type)GetValue(FlagTypeProperty); } set { SetValue(FlagTypeProperty, value); } } public string FlagString { get { return (string)GetValue(FlagStringProperty); } set { SetValue(FlagStringProperty, value); } } static void OnFlagTypeChanged(DependencyObject sender, DependencyPropertyChangedEventArgs args) { Type flagType = args.NewValue as Type; Fx.Assert(flagType != null && flagType.IsEnum, "FlagType should be enum"); Fx.Assert(Attribute.IsDefined(flagType, typeof(FlagsAttribute)), "FlagType should have flags attribute"); int index = 0; FlagPanel panel = sender as FlagPanel; string[] flagNames = flagType.GetEnumNames(); string zeroValueString = Enum.ToObject(flagType, 0).ToString(); foreach (string flagName in flagNames) { if (zeroValueString.Equals("0") || !flagName.Equals(zeroValueString)) { CheckBox checkBox = new CheckBox(); panel.Children.Add(checkBox); checkBox.Content = flagName; checkBox.DataContext = panel; checkBox.SetValue(AutomationProperties.AutomationIdProperty, flagName); Binding binding = new Binding("FlagString"); binding.Mode = BindingMode.TwoWay; binding.Converter = new CheckBoxStringConverter(index); binding.ConverterParameter = panel; checkBox.SetBinding(CheckBox.IsCheckedProperty, binding); index++; } } } sealed class CheckBoxStringConverter : IValueConverter { int index; public CheckBoxStringConverter(int index) { this.index = index; } public object Convert(object value, Type targetType, object parameter, CultureInfo culture) { string str = (value as string).ToUpperInvariant(); FlagPanel panel = parameter as FlagPanel; if (str.Contains((panel.Children[this.index] as CheckBox).Content.ToString().ToUpperInvariant())) { return true; } else { return false; } } public object ConvertBack(object value, Type targetType, object parameter, CultureInfo culture) { FlagPanel panel = parameter as FlagPanel; string str = string.Empty; for (int i = 0; i < panel.Children.Count; i++) { if ((i != this.index && ((bool)(panel.Children[i] as CheckBox).IsChecked)) || (i == this.index && (bool)value)) { if (!string.IsNullOrEmpty(str)) { str += ", "; } str += (panel.Children[i] as CheckBox).Content.ToString(); } } if (string.IsNullOrEmpty(str)) { Type flagType = panel.FlagType; Fx.Assert(flagType != null && flagType.IsEnum, "FlagType should be enum"); Fx.Assert(Attribute.IsDefined(flagType, typeof(FlagsAttribute)), "FlagType should have flags attribute"); return Enum.ToObject(flagType, 0).ToString(); } return str; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. 10821,/DEVDIV_TFS/Dev10/Releases/RTMRel/ndp/cdf/src/NetFx40/Tools/System.Activities.Presentation/System/Activities/Presentation/Base/Core/Internal/PropertyEditing/Editors/FlagEditor.xaml.cs/1458001/FlagEditor.xaml.cs,4/26/2010 8:54:39 AM,4967 //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Internal.PropertyEditing.Editors { using System.Windows.Controls; using System.Windows; using System.Windows.Data; using System.Windows.Input; using System.Activities.Presentation.Internal.PropertyEditing.FromExpression.Framework.ValueEditors; using System.Activities.Presentation.PropertyEditing; using System.Windows.Media; using System.Windows.Threading; partial class FlagEditor : ComboBox { bool isCommitting = false; public static readonly DependencyProperty FlagTypeProperty = DependencyProperty.Register("FlagType", typeof(Type), typeof(FlagEditor), new PropertyMetadata(null)); public Type FlagType { get { return (Type)GetValue(FlagTypeProperty); } set { SetValue(FlagTypeProperty, value); } } public FlagEditor() { InitializeComponent(); } void Cancel() { BindingExpression binding = this.GetBindingExpression(ComboBox.TextProperty); binding.UpdateTarget(); } void Commit() { // In case of error, the popup can make the control lose focus; we don't want to commit twice. if (!this.isCommitting) { this.isCommitting = true; BindingExpression binding = this.GetBindingExpression(ComboBox.TextProperty); try { binding.UpdateSource(); } catch (ArgumentException exception) { ErrorReporting.ShowErrorMessage(exception.Message); binding.UpdateTarget(); } this.isCommitting = false; } } void Finish() { ValueEditorUtils.ExecuteCommand(PropertyValueEditorCommands.FinishEditing, this, null); } protected override void OnPreviewKeyDown(KeyEventArgs e) { if (e.Key == Key.Down) { this.IsDropDownOpen = true; e.Handled = true; } if (e.Key == Key.Enter || e.Key == Key.Return) { if (this.IsDropDownOpen) { this.IsDropDownOpen = false; } this.Commit(); if ((e.KeyboardDevice.Modifiers & ModifierKeys.Shift) == 0) { this.Finish(); } //Handle this event so that the combo box item is not applied to the text box on "Enter". e.Handled = true; } else if (e.Key == Key.Escape) { this.Cancel(); } base.OnPreviewKeyDown(e); } protected override void OnDropDownOpened(EventArgs e) { base.OnDropDownOpened(e); this.Dispatcher.BeginInvoke(DispatcherPriority.ApplicationIdle, (Action)(() => { // The item should contains the panel we placed in the combobox, // and we should always have one and only one item. ComboBoxItem comboBoxItem = this.ItemContainerGenerator.ContainerFromIndex(0) as ComboBoxItem; if (comboBoxItem != null && VisualTreeHelper.GetChildrenCount(comboBoxItem) > 0) { comboBoxItem.Focusable = false; StackPanel panel = VisualTreeHelper.GetChild(comboBoxItem, 0) as StackPanel; if (panel != null && VisualTreeHelper.GetChildrenCount(panel) > 0) { // focus on the first UIElement on the panel. UIElement item = VisualTreeHelper.GetChild(panel, 0) as UIElement; if (item != null) { item.Focus(); } } } })); } protected override void OnLostKeyboardFocus(KeyboardFocusChangedEventArgs e) { if (!this.IsKeyboardFocusWithin) { this.Commit(); } base.OnLostKeyboardFocus(e); } protected override void OnDropDownClosed(EventArgs e) { this.Commit(); base.OnDropDownClosed(e); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. 10822,/DEVDIV_TFS/Dev10/Releases/RTMRel/ndp/cdf/src/NetFx40/Tools/System.Activities.Presentation/System/Activities/Presentation/Base/Core/Internal/PropertyEditing/Editors/EditorUtilities.cs/1305376/EditorUtilities.cs,4/26/2010 8:54:39 AM,4580 //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Internal.PropertyEditing.Editors { using System; using System.Collections; using System.Globalization; using System.Reflection; using System.Activities.Presentation.PropertyEditing; using System.Activities.Presentation.Internal.PropertyEditing.FromExpression.Framework.PropertyInspector; //// Collection of utilities used by the various value editors // internal static class EditorUtilities { // Key = Type, Value = bool private static Hashtable _cachedLookups = new Hashtable(); //// Checks to see whether the specified Type is concrete and has a default constructor. // That information if both returned and cached for future reference. // // NOTE: This method does not handle structs correctly because it will return FALSE // for struct types, which is incorrect. However, this bug has its counter-part in // System.Activities.Presentation.dll where the default NewItemFactory only instantiates // non-struct classes. Both of these need to be fixed at the same time because // they are used in conjunction. However, MWD is currently locked. // // // Type to verify //True if the specified type is concrete and has a default constructor, // false otherwise. public static bool IsConcreteWithDefaultCtor(Type type) { object returnValue = _cachedLookups[type]; if (returnValue == null) { if (type == null || type.IsAbstract) { returnValue = false; } else { ConstructorInfo defaultCtor = type.GetConstructor(Type.EmptyTypes); returnValue = (defaultCtor != null && defaultCtor.IsPublic); } _cachedLookups[type] = returnValue; } return (bool)returnValue; } //// Substitutes user-friendly display names for values of properties // // Item to attempt to identify //String value for the item (guaranteed to be non-null) public static string GetDisplayName(object item) { if (item == null) { return string.Empty; } // Display a user-friendly string for PropertyValues PropertyValue propertyValue = item as PropertyValue; if (propertyValue != null) { return PropertyValueToDisplayNameConverter.Instance.Convert( propertyValue, typeof(string), null, CultureInfo.CurrentCulture).ToString(); } // Display a user-friendly string for NewItemFactoryTypeModels NewItemFactoryTypeModel model = item as NewItemFactoryTypeModel; if (model != null) { return NewItemFactoryTypeModelToDisplayNameConverter.Instance.Convert( model, typeof(string), null, CultureInfo.CurrentCulture).ToString(); } // Otherwise, resort to ToString() implementation return item.ToString(); } //// Tests whether a type t is a nullable enum type // // The type object to be tested //A bool indicating the test result public static bool IsNullableEnumType(Type t) { if (t.IsGenericType && t.GetGenericTypeDefinition() == typeof(Nullable<>)) { Type[] genericArgs = t.GetGenericArguments(); if (genericArgs != null && genericArgs.Length == 1) { return genericArgs[0].IsEnum; } } return false; } public const string NullString = "(null)"; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. 10823,/DEVDIV_TFS/Dev10/Releases/RTMRel/ndp/cdf/src/NetFx40/Tools/System.Activities.Presentation/System/Activities/Presentation/Base/Core/Internal/PropertyEditing/Editors/BoolViewEditor.cs/1305376/BoolViewEditor.cs,4/26/2010 8:54:39 AM,885 //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Internal.PropertyEditing.Editors { using System.Windows; using System.Activities.Presentation.PropertyEditing; using System.Activities.Presentation.Internal.PropertyEditing.Resources; //// Simple PropertyValueEditor that uses the BoolViewTemplate (see StylesCore.Editors.xaml) // internal class BoolViewEditor : PropertyValueEditor { public BoolViewEditor() : base(PropertyInspectorResources.GetResources()["BoolViewTemplate"] as DataTemplate) { } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. 10824,/DEVDIV_TFS/Dev10/Releases/RTMRel/ndp/cdf/src/NetFx40/Tools/System.Activities.Presentation/System/Activities/Presentation/Base/Core/Internal/PropertyEditing/CiderCategoryContainer.cs/1305376/CiderCategoryContainer.cs,4/26/2010 8:54:39 AM,15268 //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Internal.PropertyEditing { using System; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Collections.Specialized; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Text; using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Controls; using System.Activities.Presentation.PropertyEditing; using Blend = System.Activities.Presentation.Internal.PropertyEditing.FromExpression.Framework.PropertyInspector; using System.Activities.Presentation.Internal.PropertyEditing.Automation; using System.Activities.Presentation.Internal.PropertyEditing.Model; using System.Activities.Presentation.Internal.PropertyEditing.Selection; //// HACK: wrapper around Sparkle's CategoryContainer that doesn't come with // any initial set of visuals, since we re-brand the control ourselves. // Sparkle doesn't plan to change this code before they ship, so we need // this work-around. // internal class CiderCategoryContainer : Blend.CategoryContainer { private static readonly DependencyPropertyKey IsEmptyPropertyKey = DependencyProperty.RegisterReadOnly( "IsEmpty", typeof(bool), typeof(CiderCategoryContainer), new PropertyMetadata(true, null, new CoerceValueCallback(CiderCategoryContainer.CoerceIsEmpty))); //// IsEmpty property indicates whether this CategoryContainer contains any editors or unconsumed properties. // If the value is true, UI for this container will hide itself // public static readonly DependencyProperty IsEmptyProperty = IsEmptyPropertyKey.DependencyProperty; //// Property indicating whether the header for the category should be rendered or not // public static DependencyProperty ShowCategoryHeaderProperty = DependencyProperty.Register( "ShowCategoryHeader", typeof(bool), typeof(CiderCategoryContainer), new PropertyMetadata(true)); // Exposed ItemsControls that know how to convert objects into their // visual counterparts private ItemsControl _basicCategoryEditorsContainer; private ItemsControl _advancedCategoryEditorsContainer; private ItemsControl _basicPropertyContainersContainer; private ItemsControl _advancedPropertyContainersContainer; private bool _UIHooksInitialized; // Keyboard navigation helpers private CategorySelectionStop _basicCategorySelectionStop; private CategorySelectionStop _advancedCategorySelectionStop; public CiderCategoryContainer() : base(false) { // Note: this logic, along with the IsEmpty DP should be pushed into the base class this.BasicCategoryEditors.CollectionChanged += new NotifyCollectionChangedEventHandler(OnContentChanged); this.AdvancedCategoryEditors.CollectionChanged += new NotifyCollectionChangedEventHandler(OnContentChanged); this.UnconsumedBasicProperties.CollectionChanged += new NotifyCollectionChangedEventHandler(OnContentChanged); this.UnconsumedAdvancedProperties.CollectionChanged += new NotifyCollectionChangedEventHandler(OnContentChanged); } // IsEmpty ReadOnly DP // Events that would have ideally been baked in the base class public event EventHandler ExpandedChanged; public event EventHandler AdvancedSectionPinnedChanged; //// Gets the value for IsEmpty DP // public bool IsEmpty { get { return (bool)this.GetValue(IsEmptyProperty); } } //// Gets or set the value of ShowCategoryHeaderProperty // public bool ShowCategoryHeader { get { return (bool)this.GetValue(ShowCategoryHeaderProperty); } set { this.SetValue(ShowCategoryHeaderProperty, value); } } //// Wrapper around the ExpandedProperty that keyboard navigation understands. Called from Xaml. // [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public ISelectionStop BasicCategorySelectionStop { get { if (_basicCategorySelectionStop == null) { _basicCategorySelectionStop = new CategorySelectionStop(this, false); } return _basicCategorySelectionStop; } } //// Wrapper around the AdvancedSectionPinnedProperty that keyboard navigation understands // [SuppressMessage("Microsoft.Performance", "CA1811:AvoidUncalledPrivateCode")] public ISelectionStop AdvancedCategorySelectionStop { get { if (_advancedCategorySelectionStop == null) { _advancedCategorySelectionStop = new CategorySelectionStop(this, true); } return _advancedCategorySelectionStop; } } private ItemsControl BasicCategoryEditorsContainer { get { // // If called before the UI itself is rendered, this property // will return null, which needs to be handled by the caller // EnsureUIHooksInitialized(); return _basicCategoryEditorsContainer; } } private ItemsControl AdvancedCategoryEditorsContainer { get { // // If called before the UI itself is rendered, this property // will return null, which needs to be handled by the caller // EnsureUIHooksInitialized(); return _advancedCategoryEditorsContainer; } } private ItemsControl BasicPropertyContainersContainer { get { // // If called before the UI itself is rendered, this property // will return null, which needs to be handled by the caller // EnsureUIHooksInitialized(); return _basicPropertyContainersContainer; } } private ItemsControl AdvancedPropertyContainersContainer { get { // // If called before the UI itself is rendered, this property // will return null, which needs to be handled by the caller // EnsureUIHooksInitialized(); return _advancedPropertyContainersContainer; } } private static object CoerceIsEmpty(DependencyObject obj, object value) { CiderCategoryContainer theThis = obj as CiderCategoryContainer; if (theThis == null) { return value; } return theThis.BasicCategoryEditors.Count == 0 && theThis.UnconsumedBasicProperties.Count == 0 && theThis.AdvancedCategoryEditors.Count == 0 && theThis.UnconsumedAdvancedProperties.Count == 0; } private void OnContentChanged(object sender, NotifyCollectionChangedEventArgs e) { this.CoerceValue(IsEmptyProperty); } // ShowCategoryHeader DP // In Cider and unlike in Blend, we expose all properties (browsable and non-browsable) through the // property editing object model. Hence, we need to make sure that the UI representing it (CategoryContainer) // does the filtering instead. This is by design and, for consistency, it should be pushed into Blend as well protected override void AddProperty(PropertyEntry property, ObservableCollectionunconsumedProperties, ObservableCollection referenceOrder, ObservableCollection categoryEditors) { // Is this property browsable? ModelPropertyEntry modelPropertyEntry = property as ModelPropertyEntry; if (modelPropertyEntry != null && !modelPropertyEntry.IsBrowsable) { return; } // Yes, so we can safely add it to the list base.AddProperty(property, unconsumedProperties, referenceOrder, categoryEditors); } // // Attempts to look up the corresponding PropertyContainer to the specified PropertyEntry // // Property to look up // Set to true if the specified property may have a container // but the visual does not exist yet and should be requested later. //Corresponding PropertyContainer, if found, null otherwise public PropertyContainer ContainerFromProperty(PropertyEntry property, out bool pendingGeneration) { pendingGeneration = false; if (property == null) { return null; } if (this.BasicPropertyContainersContainer != null) { PropertyContainer propertyContainer = this.BasicPropertyContainersContainer.ItemContainerGenerator.ContainerFromItem(property) as PropertyContainer; if (propertyContainer != null) { return propertyContainer; } } if (this.AdvancedPropertyContainersContainer != null) { PropertyContainer propertyContainer = this.AdvancedPropertyContainersContainer.ItemContainerGenerator.ContainerFromItem(property) as PropertyContainer; if (propertyContainer != null) { return propertyContainer; } } if (!_UIHooksInitialized) { pendingGeneration = true; } return null; } //// Attempts to look up the corresponding UI representation of the specified CategoryEditor // // Editor to look up // Set to true if the specified editor may have a container // but the visual does not exist yet and should be requested later. //UI representation of the specified CategoryEditor, if found, null otherwise. public UIElement ContainerFromEditor(CategoryEditor editor, out bool pendingGeneration) { pendingGeneration = false; if (editor == null) { return null; } if (this.BasicCategoryEditorsContainer != null) { UIElement categoryEditor = this.BasicCategoryEditorsContainer.ItemContainerGenerator.ContainerFromItem(editor) as UIElement; if (categoryEditor != null) { return categoryEditor; } } if (this.AdvancedCategoryEditorsContainer != null) { UIElement categoryEditor = this.AdvancedCategoryEditorsContainer.ItemContainerGenerator.ContainerFromItem(editor) as UIElement; if (categoryEditor != null) { return categoryEditor; } } if (!_UIHooksInitialized) { pendingGeneration = true; } return null; } protected override AutomationPeer OnCreateAutomationPeer() { return CategoryContainerAutomationPeer.CreateStandAloneAutomationPeer(this); } // This method fires ExpandedChanged and AdvancedSectionPinnedChanged events. Ideally we would include // these events in Blend.CategoryContainer, but the assembly containing that class has already been locked protected override void OnPropertyChanged(System.Windows.DependencyPropertyChangedEventArgs e) { base.OnPropertyChanged(e); if (e.Property == ExpandedProperty && ExpandedChanged != null) { ExpandedChanged(this, EventArgs.Empty); } if (e.Property == AdvancedSectionPinnedProperty && AdvancedSectionPinnedChanged != null) { AdvancedSectionPinnedChanged(this, EventArgs.Empty); } } // Returns true if the hooks should have already been generated // false otherwise. // private bool EnsureUIHooksInitialized() { if (_UIHooksInitialized) { return true; } _basicCategoryEditorsContainer = VisualTreeUtils.GetNamedChild(this, "PART_BasicCategoryEditors"); _advancedCategoryEditorsContainer = VisualTreeUtils.GetNamedChild (this, "PART_AdvancedCategoryEditors"); _basicPropertyContainersContainer = VisualTreeUtils.GetNamedChild (this, "PART_BasicPropertyList"); _advancedPropertyContainersContainer = VisualTreeUtils.GetNamedChild (this, "PART_AdvancedPropertyList"); if (_basicCategoryEditorsContainer == null && _advancedCategoryEditorsContainer == null && _basicPropertyContainersContainer == null && _advancedPropertyContainersContainer == null) { return false; } if (_basicCategoryEditorsContainer == null || _advancedCategoryEditorsContainer == null || _basicPropertyContainersContainer == null || _advancedPropertyContainersContainer == null) { Debug.Fail("UI for CategoryContainer changed. Need to update CiderCategoryContainer logic."); } _UIHooksInitialized = true; return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
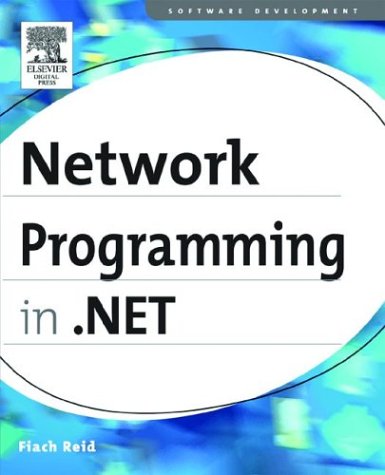
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScriptMethodAttribute.cs
- BamlStream.cs
- ChangeConflicts.cs
- XmlEncoding.cs
- MatrixUtil.cs
- ShaperBuffers.cs
- SessionStateContainer.cs
- Command.cs
- ColorConvertedBitmap.cs
- TypeExtensionSerializer.cs
- NonVisualControlAttribute.cs
- SymbolTable.cs
- UnionCqlBlock.cs
- DmlSqlGenerator.cs
- ConstrainedDataObject.cs
- ToolStripRenderEventArgs.cs
- CodeArgumentReferenceExpression.cs
- AppSettingsSection.cs
- MsmqEncryptionAlgorithm.cs
- TypeUnloadedException.cs
- ComponentEditorPage.cs
- JsonQNameDataContract.cs
- PropertyItemInternal.cs
- DocumentsTrace.cs
- BitHelper.cs
- PackageRelationship.cs
- StringDictionary.cs
- ScrollPattern.cs
- WinEventWrap.cs
- UnSafeCharBuffer.cs
- CryptoHandle.cs
- KoreanLunisolarCalendar.cs
- BitmapEffectDrawing.cs
- OrderByBuilder.cs
- AssemblyBuilder.cs
- HitTestWithPointDrawingContextWalker.cs
- WaitingCursor.cs
- CommandField.cs
- RSAOAEPKeyExchangeFormatter.cs
- DataKey.cs
- PromptBuilder.cs
- DirectionalLight.cs
- CacheMode.cs
- XmlValidatingReader.cs
- CustomAssemblyResolver.cs
- CryptoProvider.cs
- Int32Rect.cs
- AcceptorSessionSymmetricTransportSecurityProtocol.cs
- Visitor.cs
- RelatedImageListAttribute.cs
- DashStyle.cs
- X509RecipientCertificateClientElement.cs
- UrlPropertyAttribute.cs
- ListControlActionList.cs
- ConnectionPoolManager.cs
- ScriptResourceHandler.cs
- StyleSheetRefUrlEditor.cs
- ProfileSettingsCollection.cs
- ContextMenuService.cs
- BoolExpressionVisitors.cs
- DrawItemEvent.cs
- SyndicationDeserializer.cs
- TransformedBitmap.cs
- IPAddress.cs
- ProcessHostMapPath.cs
- ParamArrayAttribute.cs
- Image.cs
- HtmlInputHidden.cs
- PrintPreviewControl.cs
- OutputCacheModule.cs
- ComplexObject.cs
- PropertyInformation.cs
- PolicyException.cs
- TypeBuilder.cs
- StrongNameKeyPair.cs
- keycontainerpermission.cs
- RegisteredHiddenField.cs
- CellQuery.cs
- RunClient.cs
- DbTypeMap.cs
- ClientProtocol.cs
- TextTreeInsertElementUndoUnit.cs
- DashStyle.cs
- TemplateBindingExpression.cs
- BitmapEffectGeneralTransform.cs
- WindowsRichEditRange.cs
- DocumentViewerBase.cs
- WebSysDescriptionAttribute.cs
- WorkflowRuntimeSection.cs
- SparseMemoryStream.cs
- FlowDocumentPageViewerAutomationPeer.cs
- AffineTransform3D.cs
- XmlUtf8RawTextWriter.cs
- RowSpanVector.cs
- ApplicationServiceManager.cs
- ProcessInfo.cs
- lengthconverter.cs
- Parser.cs
- WebConfigurationHost.cs
- SendMailErrorEventArgs.cs