Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Printing / Margins.cs / 1407647 / Margins.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Printing { using System.Runtime.Serialization.Formatters; using System.Runtime.Serialization; using System.Diagnostics; using System; using System.Runtime.InteropServices; using System.Drawing; using Microsoft.Win32; using System.ComponentModel; using System.Globalization; ////// /// [ Serializable, TypeConverterAttribute(typeof(MarginsConverter)) ] public class Margins : ICloneable { private int left; private int right; private int top; private int bottom; [OptionalField] private double doubleLeft; [OptionalField] private double doubleRight; [OptionalField] private double doubleTop; [OptionalField] private double doubleBottom; ////// Specifies the margins of a printed page. /// ////// /// public Margins() : this(100, 100, 100, 100) { } ////// Initializes a new instance of a the ///class with one-inch margins. /// /// /// public Margins(int left, int right, int top, int bottom) { CheckMargin(left, "left"); CheckMargin(right, "right"); CheckMargin(top, "top"); CheckMargin(bottom, "bottom"); this.left = left; this.right = right; this.top = top; this.bottom = bottom; this.doubleLeft = (double)left; this.doubleRight = (double)right; this.doubleTop = (double)top; this.doubleBottom = (double)bottom; } ////// Initializes a new instance of a the ///class with the specified left, right, top, and bottom /// margins. /// /// /// public int Left { get { return left;} set { CheckMargin(value, "Left"); left = value; this.doubleLeft = (double)value; } } ////// Gets or sets the left margin, in hundredths of an inch. /// ////// /// public int Right { get { return right;} set { CheckMargin(value, "Right"); right = value; this.doubleRight = (double)value; } } ////// Gets or sets the right margin, in hundredths of an inch. /// ////// /// public int Top { get { return top;} set { CheckMargin(value, "Top"); top = value; this.doubleTop = (double)value; } } ////// Gets or sets the top margin, in hundredths of an inch. /// ////// /// public int Bottom { get { return bottom;} set { CheckMargin(value, "Bottom"); bottom = value; this.doubleBottom = (double)value; } } ////// Gets or sets the bottom margin, in hundredths of an inch. /// ////// /// internal double DoubleLeft { get { return doubleLeft; } set { this.Left = (int)Math.Round(value); doubleLeft = value; } } ////// Gets or sets the left margin with double value, in hundredths of an inch. /// When use the setter, the ranger of setting double value should between /// 0 to Int.MaxValue; /// ////// /// internal double DoubleRight { get { return doubleRight; } set { this.Right = (int)Math.Round(value); doubleRight = value; } } ////// Gets or sets the right margin with double value, in hundredths of an inch. /// When use the setter, the ranger of setting double value should between /// 0 to Int.MaxValue; /// ////// /// internal double DoubleTop { get { return doubleTop; } set { this.Top = (int)Math.Round(value); doubleTop = value; } } ////// Gets or sets the top margin with double value, in hundredths of an inch. /// When use the setter, the ranger of setting double value should between /// 0 to Int.MaxValue; /// ////// /// internal double DoubleBottom { get { return doubleBottom; } set { this.Bottom = (int)Math.Round(value); doubleBottom = value; } } [OnDeserialized()] private void OnDeserializedMethod(StreamingContext context) { if (doubleLeft == 0 && left != 0) { doubleLeft = (double)left; } if (doubleRight == 0 && right != 0) { doubleRight = (double)right; } if (doubleTop == 0 && top != 0) { doubleTop = (double)top; } if (doubleBottom == 0 && bottom != 0) { doubleBottom = (double)bottom; } } private void CheckMargin(int margin, string name) { if (margin < 0) throw new ArgumentException(SR.GetString(SR.InvalidLowBoundArgumentEx, name, margin, "0")); } ////// Gets or sets the bottom margin with double value, in hundredths of an inch. /// When use the setter, the ranger of setting double value should between /// 0 to Int.MaxValue; /// ////// /// public object Clone() { return MemberwiseClone(); } ////// Retrieves a duplicate of this object, member by member. /// ////// /// public override bool Equals(object obj) { Margins margins = obj as Margins; if (margins == this) return true; if (margins == null) return false; return margins.Left == this.Left && margins.Right == this.Right && margins.Top == this.Top && margins.Bottom == this.Bottom; } ////// Compares this ///to a specified to see whether they /// are equal. /// /// /// public override int GetHashCode() { // return HashCodes.Combine(left, right, top, bottom); uint left = (uint) this.Left; uint right = (uint) this.Right; uint top = (uint) this.Top; uint bottom = (uint) this.Bottom; uint result = left ^ ((right << 13) | (right >> 19)) ^ ((top << 26) | (top >> 6)) ^ ((bottom << 7) | (bottom >> 25)); return(int) result; } ////// Calculates and retrieves a hash code based on the left, right, top, and bottom /// margins. /// ////// /// Tests whether two public static bool operator ==(Margins m1, Margins m2) { if (object.ReferenceEquals(m1, null) != object.ReferenceEquals(m2, null)) { return false; } if (!object.ReferenceEquals(m1, null)) { return m1.Left == m2.Left && m1.Top == m2.Top && m1.Right == m2.Right && m1.Bottom == m2.Bottom; } return true; } ///objects /// are identical. /// /// /// public static bool operator !=(Margins m1, Margins m2) { return !(m1 == m2); } ////// Tests whether two ///objects are different. /// /// /// /// public override string ToString() { return "[Margins" + " Left=" + Left.ToString(CultureInfo.InvariantCulture) + " Right=" + Right.ToString(CultureInfo.InvariantCulture) + " Top=" + Top.ToString(CultureInfo.InvariantCulture) + " Bottom=" + Bottom.ToString(CultureInfo.InvariantCulture) + "]"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Provides some interesting information for the Margins in /// String form. /// ///
Link Menu
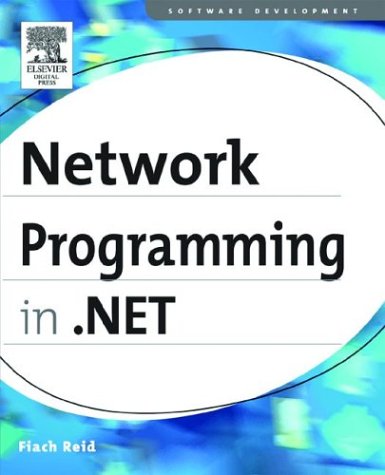
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OperatingSystem.cs
- HierarchicalDataBoundControlAdapter.cs
- DecimalAnimationBase.cs
- loginstatus.cs
- ResourceDictionary.cs
- TdsParameterSetter.cs
- NativeObjectSecurity.cs
- HtmlInputHidden.cs
- EventOpcode.cs
- CellCreator.cs
- GridViewUpdatedEventArgs.cs
- WpfKnownMemberInvoker.cs
- Header.cs
- AutomationPropertyInfo.cs
- Renderer.cs
- TemplateBuilder.cs
- EditorOptionAttribute.cs
- XmlNamespaceMapping.cs
- RegistrationContext.cs
- XmlTextReaderImplHelpers.cs
- UInt32Converter.cs
- IListConverters.cs
- ObjectToIdCache.cs
- Point.cs
- TreeIterator.cs
- FixedStringLookup.cs
- MimeFormReflector.cs
- X509ChainPolicy.cs
- EventLogPermissionAttribute.cs
- BackgroundFormatInfo.cs
- Border.cs
- ToolStripContextMenu.cs
- ComplexPropertyEntry.cs
- x509store.cs
- FileDataSource.cs
- VirtualPathProvider.cs
- ApplicationInfo.cs
- BaseCollection.cs
- DataGridViewColumnTypeEditor.cs
- ProfileService.cs
- SortDescriptionCollection.cs
- VariableElement.cs
- ExecutionScope.cs
- TdsParserSessionPool.cs
- FieldInfo.cs
- GacUtil.cs
- Comparer.cs
- SelectionItemProviderWrapper.cs
- Convert.cs
- Vertex.cs
- UTF8Encoding.cs
- ClientFormsIdentity.cs
- CellPartitioner.cs
- RowUpdatedEventArgs.cs
- DocumentSequenceHighlightLayer.cs
- SourceItem.cs
- FileEnumerator.cs
- XmlSchemaAppInfo.cs
- KeyInfo.cs
- _ProxyChain.cs
- CacheVirtualItemsEvent.cs
- InternalResources.cs
- _ListenerRequestStream.cs
- _SingleItemRequestCache.cs
- XmlSortKeyAccumulator.cs
- AsynchronousChannel.cs
- MachineSettingsSection.cs
- MergeLocalizationDirectives.cs
- WebPartVerbCollection.cs
- MemoryPressure.cs
- ObservableCollection.cs
- KeyToListMap.cs
- XmlIgnoreAttribute.cs
- HandleRef.cs
- CompoundFileIOPermission.cs
- FixedTextBuilder.cs
- ImpersonationContext.cs
- InstanceDataCollection.cs
- TrackingProfileDeserializationException.cs
- XPathAxisIterator.cs
- MultiByteCodec.cs
- IndicShape.cs
- AutomationInteropProvider.cs
- ClientTarget.cs
- HuffmanTree.cs
- ResourcesBuildProvider.cs
- OpenTypeMethods.cs
- X509UI.cs
- SelectionWordBreaker.cs
- WebPart.cs
- regiisutil.cs
- FixedNode.cs
- SubMenuStyleCollection.cs
- Content.cs
- FixedDSBuilder.cs
- ellipse.cs
- ThicknessAnimation.cs
- RepeatButtonAutomationPeer.cs
- CodeTypeDelegate.cs
- FormsIdentity.cs