Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / IO / compression / GZipStream.cs / 1305376 / GZipStream.cs
namespace System.IO.Compression { using System.IO; using System.Diagnostics; using System.Security.Permissions; public class GZipStream : Stream { private DeflateStream deflateStream; public GZipStream(Stream stream, CompressionMode mode) : this( stream, mode, false) { } public GZipStream(Stream stream, CompressionMode mode, bool leaveOpen) { deflateStream = new DeflateStream(stream, mode, leaveOpen); if (mode == CompressionMode.Compress) { IFileFormatWriter writeCommand = new GZipFormatter(); deflateStream.SetFileFormatWriter(writeCommand); } else { IFileFormatReader readCommand = new GZipDecoder(); deflateStream.SetFileFormatReader(readCommand); } } public override bool CanRead { get { if( deflateStream == null) { return false; } return deflateStream.CanRead; } } public override bool CanWrite { get { if( deflateStream == null) { return false; } return deflateStream.CanWrite; } } public override bool CanSeek { get { if( deflateStream == null) { return false; } return deflateStream.CanSeek; } } public override long Length { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override long Position { get { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } set { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } } public override void Flush() { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Flush(); return; } public override long Seek(long offset, SeekOrigin origin) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } public override void SetLength(long value) { throw new NotSupportedException(SR.GetString(SR.NotSupported)); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginRead(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginRead(array, offset, count, asyncCallback, asyncState); } public override int EndRead(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.EndRead(asyncResult); } [HostProtection(ExternalThreading=true)] public override IAsyncResult BeginWrite(byte[] array, int offset, int count, AsyncCallback asyncCallback, object asyncState) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.BeginWrite(array, offset, count, asyncCallback, asyncState); } public override void EndWrite(IAsyncResult asyncResult) { if( deflateStream == null) { throw new InvalidOperationException(SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.EndWrite(asyncResult); } public override int Read(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } return deflateStream.Read(array, offset, count); } public override void Write(byte[] array, int offset, int count) { if( deflateStream == null) { throw new ObjectDisposedException(null, SR.GetString(SR.ObjectDisposed_StreamClosed)); } deflateStream.Write(array, offset, count); } protected override void Dispose(bool disposing) { try { if (disposing && deflateStream != null) { deflateStream.Close(); } deflateStream = null; } finally { base.Dispose(disposing); } } public Stream BaseStream { get { if( deflateStream != null) { return deflateStream.BaseStream; } else { return null; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
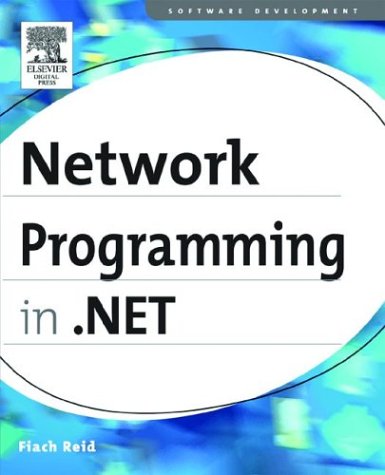
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContextMenu.cs
- IgnoreDataMemberAttribute.cs
- InOutArgument.cs
- OdbcDataReader.cs
- QilXmlReader.cs
- GridViewItemAutomationPeer.cs
- SplitterCancelEvent.cs
- CheckPair.cs
- NumberSubstitution.cs
- WebException.cs
- ProviderCollection.cs
- StreamHelper.cs
- DictionaryContent.cs
- DisplayInformation.cs
- _ChunkParse.cs
- XamlTypeMapper.cs
- MasterPageCodeDomTreeGenerator.cs
- NumericUpDown.cs
- GeometryHitTestResult.cs
- TemplateBindingExpressionConverter.cs
- DataTemplateKey.cs
- XmlSignatureProperties.cs
- GeometryValueSerializer.cs
- StatusBarAutomationPeer.cs
- TemplateColumn.cs
- RewritingPass.cs
- SafeMILHandle.cs
- XPathSingletonIterator.cs
- Certificate.cs
- StringValidator.cs
- MulticastNotSupportedException.cs
- BridgeDataRecord.cs
- XmlSchemaAnyAttribute.cs
- ImageDrawing.cs
- KeyPressEvent.cs
- CharAnimationUsingKeyFrames.cs
- CommunicationException.cs
- SqlDataSourceQueryEditorForm.cs
- SafeTimerHandle.cs
- DataGrid.cs
- ColorTranslator.cs
- PopupControlService.cs
- XmlDataSource.cs
- GetPageCompletedEventArgs.cs
- HighlightVisual.cs
- SystemResourceHost.cs
- StringUtil.cs
- BrowserPolicyValidator.cs
- RemotingConfiguration.cs
- Attachment.cs
- IisTraceListener.cs
- PixelFormats.cs
- XamlReader.cs
- XXXInfos.cs
- DbConnectionPool.cs
- BinaryCommonClasses.cs
- WebPartConnectionsEventArgs.cs
- CacheVirtualItemsEvent.cs
- DbConnectionPoolOptions.cs
- XPathNodeInfoAtom.cs
- ParenthesizePropertyNameAttribute.cs
- TraceContextRecord.cs
- connectionpool.cs
- CqlQuery.cs
- OutKeywords.cs
- LogicalTreeHelper.cs
- ListBindableAttribute.cs
- Repeater.cs
- ByteAnimationUsingKeyFrames.cs
- ScriptModule.cs
- CompositeCollection.cs
- BulletChrome.cs
- ResourceAssociationSetEnd.cs
- SqlProvider.cs
- Screen.cs
- EUCJPEncoding.cs
- OleCmdHelper.cs
- ComponentEditorPage.cs
- BaseTemplateBuildProvider.cs
- Config.cs
- QueryAccessibilityHelpEvent.cs
- TimeEnumHelper.cs
- FileRegion.cs
- ValidatingReaderNodeData.cs
- ApplicationSettingsBase.cs
- CompressStream.cs
- SchemaImporterExtensionElementCollection.cs
- ImportCatalogPart.cs
- CollectionChangedEventManager.cs
- AdditionalEntityFunctions.cs
- ApplicationContext.cs
- JsonServiceDocumentSerializer.cs
- IntSecurity.cs
- DrawTreeNodeEventArgs.cs
- XPathDocumentNavigator.cs
- ConfigurationErrorsException.cs
- RecognizedWordUnit.cs
- PipelineComponent.cs
- MULTI_QI.cs
- SizeChangedInfo.cs