Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Print / Reach / Serialization / manager / ReachNamespaceInfo.cs / 1 / ReachNamespaceInfo.cs
/*++ Copyright (C) 2004- 2005 Microsoft Corporation All rights reserved. Module Name: ReachNamespaceInfo.cs Abstract: Contains the class definition of some classes that maintain the NameSpaces specific information. Author: [....] ([....]) 1-December-2004 Revision History: --*/ using System; using System.Collections; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Reflection; using System.Xml; using System.IO; using System.Security; using System.Security.Permissions; using System.ComponentModel.Design.Serialization; using System.Windows.Xps.Packaging; using System.Windows.Documents; using System.Windows.Media; using System.Windows.Markup; namespace System.Windows.Xps.Serialization { internal class SerializableObjectNamespaceInfo { #region Constructor internal SerializableObjectNamespaceInfo( Type type, string prefix, string xmlNamespace ) : this(type.Namespace, prefix, xmlNamespace) { } internal SerializableObjectNamespaceInfo( string clrNamespace, string prefix, string xmlNamespace ) { this._xmlNamespace = xmlNamespace; this._clrNamespace = clrNamespace; this._prefix = prefix; } #endregion Constructor #region Internal Properties internal string Prefix { get { return _prefix; } } internal string XmlNamespace { get { return _xmlNamespace; } } internal string ClrNamespace { get { return _clrNamespace; } } #endregion Internal Properties #region Private Data private readonly string _prefix; private readonly string _xmlNamespace; private readonly string _clrNamespace; #endregion Private Data }; internal class MetroSerializationNamespaceTable { #region Constructor internal MetroSerializationNamespaceTable( MetroSerializationNamespaceTable parent ) { Initialize(parent); } #endregion Constructor #region Internal Properties internal SerializableObjectNamespaceInfo this[Type type] { get { return (SerializableObjectNamespaceInfo)_innerDictionary[type]; } set { _innerDictionary[type] = value; } } #endregion Internal Properties #region Internal Methods internal bool Contains( Type type ) { return _innerDictionary.Contains(type); } internal void Add( Type type, SerializableObjectNamespaceInfo namespaceInfo) { _innerDictionary.Add(type, namespaceInfo); } internal void Initialize( MetroSerializationNamespaceTable parent ) { _innerDictionary = new Hashtable(11); } #endregion Internal Methods #region Private Data private IDictionary _innerDictionary; #endregion Private Data }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
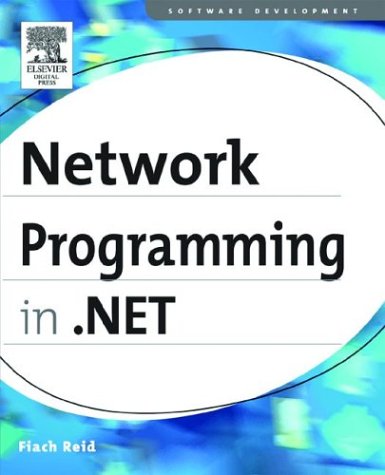
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Sql8ExpressionRewriter.cs
- relpropertyhelper.cs
- DocumentGridPage.cs
- ProxyAttribute.cs
- ThreadBehavior.cs
- WindowsTokenRoleProvider.cs
- DecimalStorage.cs
- OutOfMemoryException.cs
- SimpleType.cs
- ListViewSortEventArgs.cs
- PenThreadWorker.cs
- SiteMapDesignerDataSourceView.cs
- TCPClient.cs
- TemplateAction.cs
- GcHandle.cs
- DBSqlParserTableCollection.cs
- EventLogPermissionEntry.cs
- QuaternionKeyFrameCollection.cs
- ManagementClass.cs
- SchemaImporterExtensionElement.cs
- RootBrowserWindow.cs
- GenericsInstances.cs
- List.cs
- ColorDialog.cs
- ClientSponsor.cs
- PrintController.cs
- PaperSource.cs
- RichTextBoxConstants.cs
- PowerStatus.cs
- RelativeSource.cs
- XPathEmptyIterator.cs
- DispatcherObject.cs
- FieldInfo.cs
- EntityDataSource.cs
- TextBoxBase.cs
- InputLanguageEventArgs.cs
- PeerApplicationLaunchInfo.cs
- SimpleHandlerBuildProvider.cs
- BuildProviderCollection.cs
- Gdiplus.cs
- MouseButtonEventArgs.cs
- ConfigurationManager.cs
- SevenBitStream.cs
- basevalidator.cs
- BinaryConverter.cs
- Listbox.cs
- HtmlElementEventArgs.cs
- TextViewSelectionProcessor.cs
- RangeBaseAutomationPeer.cs
- StrongBox.cs
- HwndMouseInputProvider.cs
- DataGridRelationshipRow.cs
- XmlSchemaProviderAttribute.cs
- MemberHolder.cs
- ParameterModifier.cs
- DecimalConstantAttribute.cs
- StateChangeEvent.cs
- WebReferencesBuildProvider.cs
- JsonUriDataContract.cs
- SubstitutionResponseElement.cs
- ItemsPanelTemplate.cs
- NavigationService.cs
- Geometry3D.cs
- XmlSchemaSimpleContent.cs
- _AutoWebProxyScriptEngine.cs
- SparseMemoryStream.cs
- GridViewItemAutomationPeer.cs
- GeneratedContractType.cs
- XmlSchemaSimpleType.cs
- SmiEventStream.cs
- DesignerActionMethodItem.cs
- Function.cs
- HttpListenerRequestUriBuilder.cs
- Serializer.cs
- SqlConnectionHelper.cs
- SqlNotificationEventArgs.cs
- DependencyObjectValidator.cs
- BufferedGraphicsContext.cs
- TextSimpleMarkerProperties.cs
- EventWaitHandle.cs
- ToolStripPanelRenderEventArgs.cs
- RegularExpressionValidator.cs
- Compilation.cs
- XmlStringTable.cs
- System.Data_BID.cs
- ColumnWidthChangedEvent.cs
- TableParaClient.cs
- HotSpot.cs
- StrokeSerializer.cs
- ValidationErrorEventArgs.cs
- IRCollection.cs
- RtType.cs
- ExpressionPrefixAttribute.cs
- SqlMethods.cs
- Win32.cs
- KerberosRequestorSecurityToken.cs
- DifferencingCollection.cs
- Win32Exception.cs
- Win32SafeHandles.cs
- FontNamesConverter.cs