Code:
/ DotNET / DotNET / 8.0 / untmp / WIN_WINDOWS / lh_tools_devdiv_wpf / Windows / wcp / Speech / Src / AudioFormat / SpeechAudioFormatInfo.cs / 1 / SpeechAudioFormatInfo.cs
//------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------- using System; using System.ComponentModel; using System.Speech.Internal.Synthesis; namespace System.Speech.AudioFormat { /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo"]/*' /> [Serializable] #if !SPEECHSERVER public #else internal #endif class SpeechAudioFormatInfo { //******************************************************************* // // Constructors // //******************************************************************* #region Constructors private SpeechAudioFormatInfo (EncodingFormat encodingFormat, int samplesPerSecond, short bitsPerSample, short channelCount, byte [] formatSpecificData) { if (encodingFormat == 0) { throw new ArgumentException (SR.Get (SRID.CannotUseCustomFormat), "encodingFormat"); } if (samplesPerSecond <= 0) { throw new ArgumentOutOfRangeException ("samplesPerSecond", SR.Get (SRID.MustBeGreaterThanZero)); } if (bitsPerSample <= 0) { throw new ArgumentOutOfRangeException ("bitsPerSample", SR.Get (SRID.MustBeGreaterThanZero)); } if (channelCount <= 0) { throw new ArgumentOutOfRangeException ("channelCount", SR.Get (SRID.MustBeGreaterThanZero)); } _encodingFormat = encodingFormat; _samplesPerSecond = samplesPerSecond; _bitsPerSample = bitsPerSample; _channelCount = channelCount; if (formatSpecificData == null) { _formatSpecificData = new byte [0]; } else { _formatSpecificData = (byte []) formatSpecificData.Clone (); } switch (encodingFormat) { case EncodingFormat.ALaw: case EncodingFormat.ULaw: if (bitsPerSample != 8) { throw new ArgumentOutOfRangeException ("bitsPerSample"); } if (formatSpecificData != null && formatSpecificData.Length != 0) { throw new ArgumentOutOfRangeException ("formatSpecificData"); } break; } } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.AudioFormatInfo1"]/*' /> [EditorBrowsable (EditorBrowsableState.Advanced)] public SpeechAudioFormatInfo (EncodingFormat encodingFormat, int samplesPerSecond, int bitsPerSample, int channelCount, int averageBytesPerSecond, int blockAlign, byte [] formatSpecificData) : this (encodingFormat, samplesPerSecond, (short) bitsPerSample, (short) channelCount, formatSpecificData) { // Don't explicitly check these are sensible values - allow flexibility here as some formats may do unexpected things here. if (averageBytesPerSecond <= 0) { throw new ArgumentOutOfRangeException ("averageBytesPerSecond", SR.Get (SRID.MustBeGreaterThanZero)); } if (blockAlign <= 0) { throw new ArgumentOutOfRangeException ("blockAlign", SR.Get (SRID.MustBeGreaterThanZero)); } _averageBytesPerSecond = averageBytesPerSecond; _blockAlign = (short) blockAlign; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.AudioFormatInfo2"]/*' /> public SpeechAudioFormatInfo (int samplesPerSecond, AudioBitsPerSample bitsPerSample, AudioChannel channel) : this (EncodingFormat.Pcm, samplesPerSecond, (short) bitsPerSample, (short) channel, null) { // Don't explicitly check these are sensible values - allow flexibility here as some formats may do unexpected things here. _blockAlign = (short) (_channelCount * (_bitsPerSample / 8)); _averageBytesPerSecond = _samplesPerSecond * _blockAlign; } #endregion //******************************************************************** // // Public Properties // //******************************************************************* #region Public Properties /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.AverageBytesPerSecond"]/*' /> [EditorBrowsable (EditorBrowsableState.Advanced)] public int AverageBytesPerSecond { get { return _averageBytesPerSecond; } } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.BitsPerSample"]/*' /> [EditorBrowsable (EditorBrowsableState.Advanced)] public int BitsPerSample { get { return _bitsPerSample; } } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.BlockAlign"]/*' /> [EditorBrowsable (EditorBrowsableState.Advanced)] public int BlockAlign { get { return _blockAlign; } } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.Format"]/*' /> public EncodingFormat EncodingFormat { get { return _encodingFormat; } } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.NumberOfChannels"]/*' /> public int ChannelCount { get { return _channelCount; } } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.SamplesPerSecond"]/*' /> public int SamplesPerSecond { get { return _samplesPerSecond; } } #endregion //******************************************************************** // // Public Methods // //******************************************************************** #region Public Methods /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.FormatSpecificData"]/*' /> public byte [] FormatSpecificData () { return (byte []) _formatSpecificData.Clone (); } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.Equals"]/*' /> public override bool Equals (object obj) { SpeechAudioFormatInfo refObj = obj as SpeechAudioFormatInfo; if (refObj == null) { return false; } if (!(_averageBytesPerSecond.Equals (refObj._averageBytesPerSecond) && _bitsPerSample.Equals (refObj._bitsPerSample) && _blockAlign.Equals (refObj._blockAlign) && _encodingFormat.Equals (refObj._encodingFormat) && _channelCount.Equals (refObj._channelCount) && _samplesPerSecond.Equals (refObj._samplesPerSecond))) { return false; } if (_formatSpecificData.Length != refObj._formatSpecificData.Length) { return false; } for (int i = 0; i < _formatSpecificData.Length; i++) { if (_formatSpecificData [i] != refObj._formatSpecificData [i]) { return false; } } return true; } /// TODOC <_include file='doc\SpeechAudioFormatInfo.uex' path='docs/doc[@for="SpeechAudioFormatInfo.GetHashCode"]/*' /> public override int GetHashCode () { return _averageBytesPerSecond.GetHashCode (); } #endregion //******************************************************************* // // Internal Methods // //******************************************************************** #region Internal Methods #if !SPEECHSERVER internal byte [] WaveFormat { get { WAVEFORMATEX wfx = new WAVEFORMATEX (); wfx.wFormatTag = (short) EncodingFormat; wfx.nChannels = (short) ChannelCount; wfx.nSamplesPerSec = SamplesPerSecond; wfx.nAvgBytesPerSec = AverageBytesPerSecond; wfx.nBlockAlign = (short) BlockAlign; wfx.wBitsPerSample = (short) BitsPerSample; wfx.cbSize = (short) FormatSpecificData ().Length; byte [] abWfx = wfx.ToBytes (); if (wfx.cbSize > 0) { byte [] wfxTemp = new byte [abWfx.Length + wfx.cbSize]; Array.Copy (abWfx, wfxTemp, abWfx.Length); Array.Copy (FormatSpecificData (), 0, wfxTemp, abWfx.Length, wfx.cbSize); abWfx = wfxTemp; } return abWfx; } } #endif #endregion //******************************************************************* // // Private Fields // //******************************************************************* #region Private Fields private int _averageBytesPerSecond; private short _bitsPerSample; private short _blockAlign; private EncodingFormat _encodingFormat; private short _channelCount; private int _samplesPerSecond; private byte [] _formatSpecificData; #endregion } //******************************************************************* // // Public Properties // //******************************************************************** #region Public Properties ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum AudioChannel { ////// TODOC /// Mono = 1, ////// TODOC /// Stereo = 2 } ////// TODOC /// #if !SPEECHSERVER public #else internal #endif enum AudioBitsPerSample { ////// TODOC /// Eight = 8, ////// TODOC /// Sixteen = 16 } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
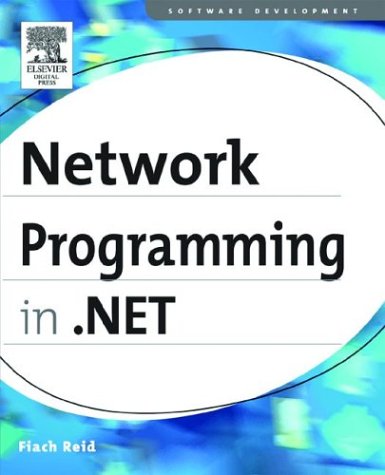
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CornerRadiusConverter.cs
- Stack.cs
- BrushMappingModeValidation.cs
- FullTextLine.cs
- ParseNumbers.cs
- StandardOleMarshalObject.cs
- TextWriterEngine.cs
- WeakReference.cs
- ListViewDesigner.cs
- Parameter.cs
- VoiceInfo.cs
- MouseWheelEventArgs.cs
- BaseInfoTable.cs
- XsdCachingReader.cs
- CacheHelper.cs
- MTConfigUtil.cs
- MsmqBindingBase.cs
- StoreItemCollection.cs
- ListBoxDesigner.cs
- TransactionFlowAttribute.cs
- AutomationPropertyInfo.cs
- OracleNumber.cs
- SchemaMapping.cs
- CoTaskMemSafeHandle.cs
- XPathDocument.cs
- ParameterModifier.cs
- DocumentSequenceHighlightLayer.cs
- Part.cs
- CompositeTypefaceMetrics.cs
- BaseResourcesBuildProvider.cs
- WebDisplayNameAttribute.cs
- SecurityTokenException.cs
- XsdCachingReader.cs
- XPathParser.cs
- DBSchemaRow.cs
- QuotedPairReader.cs
- FlowLayout.cs
- Cursor.cs
- BitmapPalettes.cs
- PanelDesigner.cs
- ReliabilityContractAttribute.cs
- InstanceData.cs
- uribuilder.cs
- PrintDialog.cs
- PropertyConverter.cs
- ServiceEndpointElement.cs
- ContextStaticAttribute.cs
- LazyTextWriterCreator.cs
- SqlWebEventProvider.cs
- FrameworkName.cs
- TemplatedWizardStep.cs
- TextWriter.cs
- ImmComposition.cs
- InstancePersistenceException.cs
- SecureStringHasher.cs
- XomlCompilerParameters.cs
- DefaultValueAttribute.cs
- ObjectManager.cs
- SamlEvidence.cs
- ImageButton.cs
- X509CertificateValidationMode.cs
- SamlSerializer.cs
- GlobalProxySelection.cs
- ToolStripItemCollection.cs
- DeferredElementTreeState.cs
- WebPartDisplayModeCancelEventArgs.cs
- BrowserInteropHelper.cs
- DataAdapter.cs
- UpdatePanelControlTrigger.cs
- __ConsoleStream.cs
- FontFamily.cs
- GridViewRowPresenterBase.cs
- Grid.cs
- updatecommandorderer.cs
- TdsParser.cs
- TableRow.cs
- PropertyToken.cs
- DesignerValidatorAdapter.cs
- AdPostCacheSubstitution.cs
- AppearanceEditorPart.cs
- SamlAction.cs
- ToolboxItemLoader.cs
- SpanIndex.cs
- InputMethodStateTypeInfo.cs
- InternalRelationshipCollection.cs
- EpmTargetTree.cs
- Solver.cs
- GregorianCalendarHelper.cs
- Asn1Utilities.cs
- _LocalDataStoreMgr.cs
- PointValueSerializer.cs
- DataGridViewButtonColumn.cs
- UrlMapping.cs
- PixelFormats.cs
- ManifestResourceInfo.cs
- IdnMapping.cs
- EntityRecordInfo.cs
- TreeNodeClickEventArgs.cs
- ScriptControlManager.cs
- PeerCollaborationPermission.cs