Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaAnyAttribute.cs / 1 / XmlSchemaAnyAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.Schema { using System.Collections; using System.ComponentModel; using System.Xml.Serialization; ////// /// public class XmlSchemaAnyAttribute : XmlSchemaAnnotated { string ns; XmlSchemaContentProcessing processContents = XmlSchemaContentProcessing.None; NamespaceList namespaceList; ///[To be supplied.] ////// /// [XmlAttribute("namespace")] public string Namespace { get { return ns; } set { ns = value; } } ///[To be supplied.] ////// /// [XmlAttribute("processContents"), DefaultValue(XmlSchemaContentProcessing.None)] public XmlSchemaContentProcessing ProcessContents { get { return processContents; } set { processContents = value; } } [XmlIgnore] internal NamespaceList NamespaceList { get { return namespaceList; } } [XmlIgnore] internal XmlSchemaContentProcessing ProcessContentsCorrect { get { return processContents == XmlSchemaContentProcessing.None ? XmlSchemaContentProcessing.Strict : processContents; } } internal void BuildNamespaceList(string targetNamespace) { if (ns != null) { namespaceList = new NamespaceList(ns, targetNamespace); } else { namespaceList = new NamespaceList(); } } internal void BuildNamespaceListV1Compat(string targetNamespace) { if (ns != null) { namespaceList = new NamespaceListV1Compat(ns, targetNamespace); } else { namespaceList = new NamespaceList(); //This is only ##any, hence base class is sufficient } } internal bool Allows(XmlQualifiedName qname) { return namespaceList.Allows(qname.Namespace); } internal static bool IsSubset(XmlSchemaAnyAttribute sub, XmlSchemaAnyAttribute super) { return NamespaceList.IsSubset(sub.NamespaceList, super.NamespaceList); } internal static XmlSchemaAnyAttribute Intersection(XmlSchemaAnyAttribute o1, XmlSchemaAnyAttribute o2, bool v1Compat) { NamespaceList nsl = NamespaceList.Intersection(o1.NamespaceList, o2.NamespaceList, v1Compat); if (nsl != null) { XmlSchemaAnyAttribute anyAttribute = new XmlSchemaAnyAttribute(); anyAttribute.namespaceList = nsl; anyAttribute.ProcessContents = o1.ProcessContents; anyAttribute.Annotation = o1.Annotation; return anyAttribute; } else { // not expressible return null; } } internal static XmlSchemaAnyAttribute Union(XmlSchemaAnyAttribute o1, XmlSchemaAnyAttribute o2, bool v1Compat) { NamespaceList nsl = NamespaceList.Union(o1.NamespaceList, o2.NamespaceList, v1Compat); if (nsl != null) { XmlSchemaAnyAttribute anyAttribute = new XmlSchemaAnyAttribute(); anyAttribute.namespaceList = nsl; anyAttribute.processContents = o1.processContents; anyAttribute.Annotation = o1.Annotation; return anyAttribute; } else { // not expressible return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
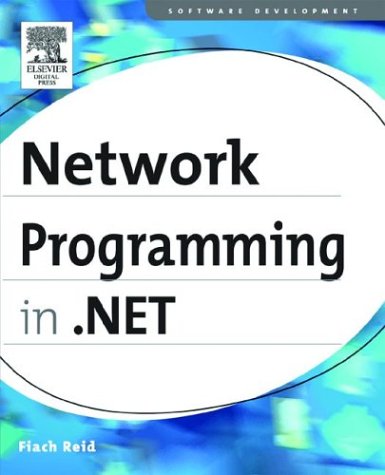
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlRewriteScalarSubqueries.cs
- MultilineStringEditor.cs
- MatrixTransform3D.cs
- GatewayDefinition.cs
- SubstitutionDesigner.cs
- ZipIOCentralDirectoryBlock.cs
- ItemsPanelTemplate.cs
- DataGridViewSelectedCellCollection.cs
- ArrangedElement.cs
- ModulesEntry.cs
- CustomCategoryAttribute.cs
- CultureMapper.cs
- ScriptResourceHandler.cs
- BitmapEffectState.cs
- ProfileGroupSettings.cs
- EncoderFallback.cs
- ConfigurationStrings.cs
- SafeFileHandle.cs
- MexNamedPipeBindingCollectionElement.cs
- Normalizer.cs
- MasterPageParser.cs
- PresentationTraceSources.cs
- DateTimeOffsetAdapter.cs
- SessionPageStatePersister.cs
- Attributes.cs
- EditingMode.cs
- ResourceProviderFactory.cs
- ProcessInfo.cs
- ModelItemImpl.cs
- DateTimeConstantAttribute.cs
- GeneralTransformGroup.cs
- ActivationServices.cs
- Pen.cs
- DesignerActionPropertyItem.cs
- CompositeControl.cs
- SortDescriptionCollection.cs
- ConnectionStringsSection.cs
- TypedTableBase.cs
- UpdatableWrapper.cs
- SizeF.cs
- DecoderExceptionFallback.cs
- SR.cs
- TableLayoutStyleCollection.cs
- DomainUpDown.cs
- ConditionCollection.cs
- MemoryPressure.cs
- ObjectConverter.cs
- TemplateControl.cs
- IisTraceListener.cs
- FillErrorEventArgs.cs
- SqlClientWrapperSmiStreamChars.cs
- EventProviderBase.cs
- DefaultBinder.cs
- OleDbConnectionInternal.cs
- Matrix.cs
- MonitorWrapper.cs
- DataObjectMethodAttribute.cs
- XmlChildNodes.cs
- TextBox.cs
- AsymmetricCryptoHandle.cs
- XPathDescendantIterator.cs
- OutOfMemoryException.cs
- VisualStateGroup.cs
- HandlerWithFactory.cs
- ApplicationContext.cs
- BaseCollection.cs
- GetPageNumberCompletedEventArgs.cs
- UriSectionData.cs
- ServerValidateEventArgs.cs
- SiteMapProvider.cs
- StagingAreaInputItem.cs
- WebPartDescriptionCollection.cs
- CodeIndexerExpression.cs
- CodeCatchClauseCollection.cs
- Connector.xaml.cs
- EnumType.cs
- TypeCollectionDesigner.xaml.cs
- InstanceNormalEvent.cs
- BeginStoryboard.cs
- EntityContainerEntitySet.cs
- ContractNamespaceAttribute.cs
- bidPrivateBase.cs
- FlowLayoutSettings.cs
- UnionExpr.cs
- XmlElementList.cs
- InstanceData.cs
- DPTypeDescriptorContext.cs
- ObjectListTitleAttribute.cs
- XComponentModel.cs
- PolyBezierSegmentFigureLogic.cs
- URLAttribute.cs
- PermissionListSet.cs
- XPathNodeIterator.cs
- RIPEMD160Managed.cs
- KeyManager.cs
- RpcCryptoRequest.cs
- Base64Decoder.cs
- SqlIdentifier.cs
- DrawingBrush.cs
- LocalTransaction.cs