Code:
/ DotNET / DotNET / 8.0 / untmp / whidbey / REDBITS / ndp / fx / src / xsp / System / Web / UI / WebControls / XmlDataSourceView.cs / 1 / XmlDataSourceView.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.UI.WebControls { using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Diagnostics; using System.Drawing.Design; using System.Security.Permissions; using System.Text; using System.Web; using System.Web.UI; using System.Xml; ////// [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class XmlDataSourceView : DataSourceView { private XmlDataSource _owner; ////// Creates a new instance of XmlDataSourceView. /// public XmlDataSourceView(XmlDataSource owner, string name) : base(owner, name) { _owner = owner; } ////// Returns all the rows of the datasource. /// protected internal override IEnumerable ExecuteSelect(DataSourceSelectArguments arguments) { arguments.RaiseUnsupportedCapabilitiesError(this); XmlNode root = _owner.GetXmlDocument(); XmlNodeList nodes = null; if (_owner.XPath.Length != 0) { // If an XPath is specified on the control, use it nodes = root.SelectNodes(_owner.XPath); } else { // Otherwise, get all the children of the root nodes = root.SelectNodes("/node()/node()"); } return new XmlDataSourceNodeDescriptorEnumeration(nodes); } public IEnumerable Select(DataSourceSelectArguments arguments) { return ExecuteSelect(arguments); } private class XmlDataSourceNodeDescriptorEnumeration : ICollection { private XmlNodeList _nodes; private int _count = -1; // -1 indicates we have not yet calculated the count public XmlDataSourceNodeDescriptorEnumeration(XmlNodeList nodes) { Debug.Assert(nodes != null, "Did not expect null node list"); _nodes = nodes; } IEnumerator IEnumerable.GetEnumerator() { foreach (XmlNode node in _nodes) { if (node.NodeType == XmlNodeType.Element) { yield return new XmlDataSourceNodeDescriptor(node); } } } int ICollection.Count { get { if (_count == -1) { // If the count has not yet been set, calculate the element count _count = 0; foreach (XmlNode node in _nodes) { if (node.NodeType == XmlNodeType.Element) { _count++; } } } return _count; } } bool ICollection.IsSynchronized { get { return false; } } object ICollection.SyncRoot { get { return null; } } void ICollection.CopyTo(Array array, int index) { for (IEnumerator e = ((IEnumerable)this).GetEnumerator(); e.MoveNext(); ) { array.SetValue(e.Current, index++); } } } } }
Link Menu
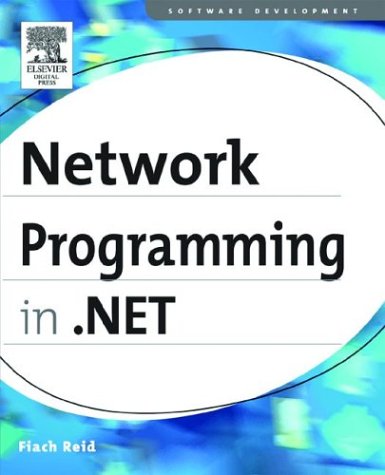
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MarkedHighlightComponent.cs
- XmlBaseReader.cs
- XmlSerializerAssemblyAttribute.cs
- ClientData.cs
- ActivityExecutorSurrogate.cs
- ThreadAbortException.cs
- SrgsOneOf.cs
- UpdatePanelTriggerCollection.cs
- Zone.cs
- IImplicitResourceProvider.cs
- TypeConverters.cs
- CompositeClientFormatter.cs
- HttpHostedTransportConfiguration.cs
- XsdBuilder.cs
- OutputCacheProfileCollection.cs
- SecuritySessionSecurityTokenProvider.cs
- Border.cs
- WindowsListViewItem.cs
- ReplyAdapterChannelListener.cs
- NameSpaceEvent.cs
- ZipIOCentralDirectoryBlock.cs
- MatchingStyle.cs
- WSTrust.cs
- X509SubjectKeyIdentifierClause.cs
- EmptyEnumerator.cs
- FilteredReadOnlyMetadataCollection.cs
- NotSupportedException.cs
- XmlNotation.cs
- GeneralTransform3DCollection.cs
- WebConfigurationHostFileChange.cs
- GenericWebPart.cs
- PointAnimationBase.cs
- Stacktrace.cs
- DataGridViewRowDividerDoubleClickEventArgs.cs
- DataBindingCollection.cs
- ToolStripSystemRenderer.cs
- DeclarativeCatalogPart.cs
- GetWinFXPath.cs
- ClientConfigurationSystem.cs
- CompensationHandlingFilter.cs
- _CommandStream.cs
- PaintEvent.cs
- TextPatternIdentifiers.cs
- XmlTypeAttribute.cs
- ConnectionManager.cs
- InternalControlCollection.cs
- ContentHostHelper.cs
- QilLiteral.cs
- FullTrustAssembliesSection.cs
- Part.cs
- ObjectToIdCache.cs
- FixedSOMTableCell.cs
- DataGridViewColumnEventArgs.cs
- OutgoingWebRequestContext.cs
- FastEncoderWindow.cs
- CodeMemberEvent.cs
- SetterTriggerConditionValueConverter.cs
- SafeProcessHandle.cs
- uribuilder.cs
- HwndSource.cs
- SetUserLanguageRequest.cs
- DataGridHyperlinkColumn.cs
- DataSourceXmlAttributeAttribute.cs
- PolicyReader.cs
- RuntimeConfigLKG.cs
- BoolExpressionVisitors.cs
- SqlNamer.cs
- FunctionDescription.cs
- CodeExpressionStatement.cs
- MetadataSerializer.cs
- WorkflowOperationFault.cs
- TextWriter.cs
- PrtTicket_Public_Simple.cs
- _ContextAwareResult.cs
- KeyedByTypeCollection.cs
- ReaderOutput.cs
- ProcessThreadCollection.cs
- UrlUtility.cs
- DocumentOrderQuery.cs
- SmtpReplyReader.cs
- TableHeaderCell.cs
- StrongTypingException.cs
- CacheSection.cs
- Blend.cs
- SoapAttributeOverrides.cs
- BrowserTree.cs
- SelectingProviderEventArgs.cs
- CorePropertiesFilter.cs
- ToolboxItemFilterAttribute.cs
- EntityFunctions.cs
- DesignerDataParameter.cs
- SmtpTransport.cs
- UnsafeNativeMethods.cs
- BooleanAnimationUsingKeyFrames.cs
- JsonFormatGeneratorStatics.cs
- BamlCollectionHolder.cs
- XmlComment.cs
- SafeMILHandle.cs
- loginstatus.cs
- Int32AnimationUsingKeyFrames.cs