Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntity / System / Data / Query / InternalTrees / RelOps.cs / 2 / RelOps.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Text; namespace System.Data.Query.InternalTrees { internal abstract class ScanTableBaseOp : RelOp { #region private state private Table m_table; #endregion #region constructors protected ScanTableBaseOp(OpType opType, Table table) : base(opType) { m_table = table; } protected ScanTableBaseOp(OpType opType) : base(opType) { } #endregion #region public methods ////// Get the table instance produced by this Op /// internal Table Table { get { return m_table; } } #endregion } ////// Scans a table /// internal sealed class ScanTableOp : ScanTableBaseOp { #region constructors ////// Scan constructor /// /// internal ScanTableOp(Table table) : base(OpType.ScanTable, table) { } private ScanTableOp() : base(OpType.ScanTable) { } #endregion #region public methods ////// Only to be used for pattern matches /// internal static readonly ScanTableOp Pattern = new ScanTableOp(); ////// No children /// internal override int Arity {get {return 0;} } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Scans a view - very similar to a ScanTable /// internal sealed class ScanViewOp : ScanTableBaseOp { #region constructors ////// Scan constructor /// /// internal ScanViewOp(Table table) : base(OpType.ScanView, table) { } private ScanViewOp() : base(OpType.ScanView) { } #endregion #region public methods ////// Only to be used for pattern matches /// internal static readonly ScanViewOp Pattern = new ScanViewOp(); ////// Exactly 1 child /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Scans a virtual extent (ie) a transient collection /// internal sealed class UnnestOp : RelOp { #region private state private Table m_table; private Var m_var; #endregion #region constructors internal UnnestOp(Var v, Table t) : this() { m_var = v; m_table = t; } private UnnestOp() : base(OpType.Unnest) { } #endregion #region publics internal static readonly UnnestOp Pattern = new UnnestOp(); ////// The (collection-typed) Var that's being unnested /// internal Var Var { get { return m_var; } } ////// The table instance produced by this Op /// internal Table Table { get { return m_table; } } ////// Exactly 1 child /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Base class for all Join operations /// internal abstract class JoinBaseOp : RelOp { #region constructors internal JoinBaseOp(OpType opType) : base(opType) { } #endregion #region public surface ////// 3 children - left, right, pred /// internal override int Arity { get { return 3; } } #endregion } ////// A CrossJoin (n-way) /// internal sealed class CrossJoinOp : JoinBaseOp { #region constructors private CrossJoinOp() : base(OpType.CrossJoin) { } #endregion #region public methods ////// Singleton instance /// internal static readonly CrossJoinOp Instance = new CrossJoinOp(); internal static readonly CrossJoinOp Pattern = CrossJoinOp.Instance; ////// varying number of children (but usually greater than 1) /// internal override int Arity { get { return ArityVarying; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// An InnerJoin /// internal sealed class InnerJoinOp : JoinBaseOp { #region constructors private InnerJoinOp() : base(OpType.InnerJoin) { } #endregion #region public methods internal static readonly InnerJoinOp Instance = new InnerJoinOp(); internal static readonly InnerJoinOp Pattern = InnerJoinOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A LeftOuterJoin /// internal sealed class LeftOuterJoinOp : JoinBaseOp { #region constructors private LeftOuterJoinOp() : base(OpType.LeftOuterJoin) { } #endregion #region public methods internal static readonly LeftOuterJoinOp Instance = new LeftOuterJoinOp(); internal static readonly LeftOuterJoinOp Pattern = LeftOuterJoinOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A FullOuterJoin /// internal sealed class FullOuterJoinOp : JoinBaseOp { #region private constructors private FullOuterJoinOp() : base(OpType.FullOuterJoin) { } #endregion #region public methods internal static readonly FullOuterJoinOp Instance = new FullOuterJoinOp(); internal static readonly FullOuterJoinOp Pattern = FullOuterJoinOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Base class for all Apply Ops /// internal abstract class ApplyBaseOp : RelOp { #region constructors internal ApplyBaseOp(OpType opType) : base(opType) { } #endregion #region public surface ////// 2 children - left, right /// internal override int Arity { get { return 2; } } #endregion } ////// CrossApply /// internal sealed class CrossApplyOp : ApplyBaseOp { #region constructors private CrossApplyOp() : base(OpType.CrossApply) { } #endregion #region public methods internal static readonly CrossApplyOp Instance = new CrossApplyOp(); internal static readonly CrossApplyOp Pattern = CrossApplyOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// OuterApply /// internal sealed class OuterApplyOp : ApplyBaseOp { #region constructors private OuterApplyOp() : base(OpType.OuterApply) { } #endregion #region public methods internal static readonly OuterApplyOp Instance = new OuterApplyOp(); internal static readonly OuterApplyOp Pattern = OuterApplyOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// FilterOp /// internal sealed class FilterOp : RelOp { #region constructors private FilterOp() : base(OpType.Filter) { } #endregion #region public methods internal static readonly FilterOp Instance = new FilterOp(); internal static readonly FilterOp Pattern = FilterOp.Instance; ////// 2 children - input, pred /// internal override int Arity { get { return 2; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// ProjectOp /// internal sealed class ProjectOp : RelOp { #region private state private VarVec m_vars; #endregion #region constructors private ProjectOp() : base(OpType.Project) { } internal ProjectOp(VarVec vars) : this() { Debug.Assert(null != vars, "null vars?"); Debug.Assert(!vars.IsEmpty, "empty varlist?"); m_vars = vars; } #endregion #region public methods internal static readonly ProjectOp Pattern = new ProjectOp(); ////// 2 children - input, projections (VarDefList) /// internal override int Arity { get { return 2; } } ////// The Vars projected by this Op /// internal VarVec Outputs { get { return m_vars; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A Sortkey /// internal class SortKey { #region private state private Var m_var; private bool m_asc; private string m_collation; #endregion #region constructors internal SortKey(Var v, bool asc, string collation) { m_var = v; m_asc = asc; m_collation = collation; } #endregion #region public methods ////// The Var being sorted /// internal Var Var { get { return m_var; } set { m_var = value; } } ////// Is this a sort asc, or a sort desc /// internal bool AscendingSort { get { return m_asc; } } ////// An optional collation (only for string types) /// internal string Collation { get { return m_collation; } } #endregion } ////// Base type for SortOp and ConstrainedSortOp /// internal abstract class SortBaseOp : RelOp { #region private state private Listm_keys; #endregion #region Constructors // Pattern constructor internal SortBaseOp(OpType opType) : base(opType) { Debug.Assert(opType == OpType.Sort || opType == OpType.ConstrainedSort, "SortBaseOp OpType must be Sort or ConstrainedSort"); } internal SortBaseOp(OpType opType, List sortKeys) : this(opType) { m_keys = sortKeys; } #endregion /// /// Sort keys /// internal ListKeys { get { return m_keys; } } } /// /// A SortOp /// internal sealed class SortOp : SortBaseOp { #region constructors private SortOp() : base(OpType.Sort) { } internal SortOp(ListsortKeys) : base(OpType.Sort, sortKeys) {} #endregion #region public methods internal static readonly SortOp Pattern = new SortOp(); /// /// 1 child - the input, SortOp must not contain local VarDefs /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A Constrained SortOp. Used to represent physical paging (skip, limit, skip + limit) operations. /// internal sealed class ConstrainedSortOp : SortBaseOp { #region private state private bool _withTies; #endregion #region constructors // Pattern constructor private ConstrainedSortOp() : base(OpType.ConstrainedSort) { } internal ConstrainedSortOp(ListsortKeys, bool withTies) : base(OpType.ConstrainedSort, sortKeys) { _withTies = withTies; } #endregion #region public methods internal bool WithTies { get { return _withTies; } set { _withTies = value; } } internal static readonly ConstrainedSortOp Pattern = new ConstrainedSortOp(); /// /// 3 children - the input, a possibly NullOp limit and a possibly NullOp skip count. /// internal override int Arity { get { return 3; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// GroupByOp /// internal sealed class GroupByOp : RelOp { #region private state private VarVec m_keys; private VarVec m_outputs; #endregion #region constructors private GroupByOp() : base(OpType.GroupBy) { } internal GroupByOp(VarVec keys, VarVec outputs) : this() { m_keys = keys; m_outputs = outputs; } #endregion #region public methods internal static readonly GroupByOp Pattern = new GroupByOp(); ////// 3 children - input, keys (vardeflist), aggregates (vardeflist) /// internal override int Arity { get { return 3; } } ////// GroupBy keys /// internal VarVec Keys { get { return m_keys; } } ////// All outputs of this Op - includes keys and aggregates /// internal VarVec Outputs { get { return m_outputs; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Base class for set operations - union, intersect, except /// internal abstract class SetOp : RelOp { #region private state private VarMap[] m_varMap; private VarVec m_outputVars; #endregion #region constructors internal SetOp(OpType opType, VarVec outputs, VarMap left, VarMap right) : this(opType) { m_varMap = new VarMap[2]; m_varMap[0] = left; m_varMap[1] = right; m_outputVars = outputs; } protected SetOp(OpType opType) : base(opType) { } #endregion #region public methods ////// 2 children - left, right /// internal override int Arity { get { return 2; } } ////// Map of result vars to the vars of each branch of the setOp /// internal VarMap[] VarMap { get { return m_varMap; } } ////// Get the set of output vars produced /// internal VarVec Outputs { get { return m_outputVars; } } #endregion } ////// UnionAll (ie) no duplicate elimination /// internal sealed class UnionAllOp : SetOp { #region private state private Var m_branchDiscriminator; #endregion #region constructors private UnionAllOp() : base(OpType.UnionAll) { } internal UnionAllOp(VarVec outputs, VarMap left, VarMap right, Var branchDiscriminator) : base(OpType.UnionAll, outputs, left, right) { m_branchDiscriminator = branchDiscriminator; } #endregion #region public methods internal static readonly UnionAllOp Pattern = new UnionAllOp(); ////// Returns the branch discriminator var for this op. It may be null, if /// we haven't been through key pullup yet. /// internal Var BranchDiscriminator { get { return m_branchDiscriminator; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// An IntersectOp /// internal sealed class IntersectOp : SetOp { #region constructors private IntersectOp() : base(OpType.Intersect) { } internal IntersectOp(VarVec outputs, VarMap left, VarMap right) : base(OpType.Intersect, outputs, left,right) { } #endregion #region public methods internal static readonly IntersectOp Pattern = new IntersectOp(); ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// ExceptOp (Minus) /// internal sealed class ExceptOp : SetOp { #region constructors private ExceptOp() : base(OpType.Except) { } internal ExceptOp(VarVec outputs, VarMap left, VarMap right) : base(OpType.Except, outputs, left, right) { } #endregion #region public methods internal static readonly ExceptOp Pattern = new ExceptOp(); ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// DistinctOp /// internal sealed class DistinctOp : RelOp { #region private state private VarVec m_keys; #endregion #region constructors private DistinctOp() : base(OpType.Distinct) { } internal DistinctOp(VarVec keyVars) : this() { Debug.Assert(keyVars != null); Debug.Assert(!keyVars.IsEmpty); m_keys = keyVars; } #endregion #region public methods internal static readonly DistinctOp Pattern = new DistinctOp(); ////// 1 child - input /// internal override int Arity { get { return 1; } } ////// Get "key" vars for the distinct /// internal VarVec Keys { get { return m_keys; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Selects out a single row from a underlying subquery. Two flavors of this Op exist. /// The first flavor enforces the single-row-ness (ie) an error is raised if the /// underlying subquery produces more than one row. /// The other flavor simply choses any row from the input /// internal sealed class SingleRowOp : RelOp { #region constructors private SingleRowOp() : base(OpType.SingleRow) { } #endregion #region public methods ////// Singleton instance /// internal static readonly SingleRowOp Instance = new SingleRowOp(); ////// Pattern for transformation rules /// internal static readonly SingleRowOp Pattern = Instance; ////// 1 child - input /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Represents a table with a single row /// internal sealed class SingleRowTableOp : RelOp { #region constructors private SingleRowTableOp() : base(OpType.SingleRowTable) { } #endregion #region public methods ////// Singleton instance /// internal static readonly SingleRowTableOp Instance = new SingleRowTableOp(); ////// Pattern for transformation rules /// internal static readonly SingleRowTableOp Pattern = Instance; ////// 0 children /// internal override int Arity { get { return 0; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....], [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Diagnostics; using System.Globalization; using System.Text; namespace System.Data.Query.InternalTrees { internal abstract class ScanTableBaseOp : RelOp { #region private state private Table m_table; #endregion #region constructors protected ScanTableBaseOp(OpType opType, Table table) : base(opType) { m_table = table; } protected ScanTableBaseOp(OpType opType) : base(opType) { } #endregion #region public methods ////// Get the table instance produced by this Op /// internal Table Table { get { return m_table; } } #endregion } ////// Scans a table /// internal sealed class ScanTableOp : ScanTableBaseOp { #region constructors ////// Scan constructor /// /// internal ScanTableOp(Table table) : base(OpType.ScanTable, table) { } private ScanTableOp() : base(OpType.ScanTable) { } #endregion #region public methods ////// Only to be used for pattern matches /// internal static readonly ScanTableOp Pattern = new ScanTableOp(); ////// No children /// internal override int Arity {get {return 0;} } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Scans a view - very similar to a ScanTable /// internal sealed class ScanViewOp : ScanTableBaseOp { #region constructors ////// Scan constructor /// /// internal ScanViewOp(Table table) : base(OpType.ScanView, table) { } private ScanViewOp() : base(OpType.ScanView) { } #endregion #region public methods ////// Only to be used for pattern matches /// internal static readonly ScanViewOp Pattern = new ScanViewOp(); ////// Exactly 1 child /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Scans a virtual extent (ie) a transient collection /// internal sealed class UnnestOp : RelOp { #region private state private Table m_table; private Var m_var; #endregion #region constructors internal UnnestOp(Var v, Table t) : this() { m_var = v; m_table = t; } private UnnestOp() : base(OpType.Unnest) { } #endregion #region publics internal static readonly UnnestOp Pattern = new UnnestOp(); ////// The (collection-typed) Var that's being unnested /// internal Var Var { get { return m_var; } } ////// The table instance produced by this Op /// internal Table Table { get { return m_table; } } ////// Exactly 1 child /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Base class for all Join operations /// internal abstract class JoinBaseOp : RelOp { #region constructors internal JoinBaseOp(OpType opType) : base(opType) { } #endregion #region public surface ////// 3 children - left, right, pred /// internal override int Arity { get { return 3; } } #endregion } ////// A CrossJoin (n-way) /// internal sealed class CrossJoinOp : JoinBaseOp { #region constructors private CrossJoinOp() : base(OpType.CrossJoin) { } #endregion #region public methods ////// Singleton instance /// internal static readonly CrossJoinOp Instance = new CrossJoinOp(); internal static readonly CrossJoinOp Pattern = CrossJoinOp.Instance; ////// varying number of children (but usually greater than 1) /// internal override int Arity { get { return ArityVarying; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// An InnerJoin /// internal sealed class InnerJoinOp : JoinBaseOp { #region constructors private InnerJoinOp() : base(OpType.InnerJoin) { } #endregion #region public methods internal static readonly InnerJoinOp Instance = new InnerJoinOp(); internal static readonly InnerJoinOp Pattern = InnerJoinOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A LeftOuterJoin /// internal sealed class LeftOuterJoinOp : JoinBaseOp { #region constructors private LeftOuterJoinOp() : base(OpType.LeftOuterJoin) { } #endregion #region public methods internal static readonly LeftOuterJoinOp Instance = new LeftOuterJoinOp(); internal static readonly LeftOuterJoinOp Pattern = LeftOuterJoinOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A FullOuterJoin /// internal sealed class FullOuterJoinOp : JoinBaseOp { #region private constructors private FullOuterJoinOp() : base(OpType.FullOuterJoin) { } #endregion #region public methods internal static readonly FullOuterJoinOp Instance = new FullOuterJoinOp(); internal static readonly FullOuterJoinOp Pattern = FullOuterJoinOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Base class for all Apply Ops /// internal abstract class ApplyBaseOp : RelOp { #region constructors internal ApplyBaseOp(OpType opType) : base(opType) { } #endregion #region public surface ////// 2 children - left, right /// internal override int Arity { get { return 2; } } #endregion } ////// CrossApply /// internal sealed class CrossApplyOp : ApplyBaseOp { #region constructors private CrossApplyOp() : base(OpType.CrossApply) { } #endregion #region public methods internal static readonly CrossApplyOp Instance = new CrossApplyOp(); internal static readonly CrossApplyOp Pattern = CrossApplyOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// OuterApply /// internal sealed class OuterApplyOp : ApplyBaseOp { #region constructors private OuterApplyOp() : base(OpType.OuterApply) { } #endregion #region public methods internal static readonly OuterApplyOp Instance = new OuterApplyOp(); internal static readonly OuterApplyOp Pattern = OuterApplyOp.Instance; ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// FilterOp /// internal sealed class FilterOp : RelOp { #region constructors private FilterOp() : base(OpType.Filter) { } #endregion #region public methods internal static readonly FilterOp Instance = new FilterOp(); internal static readonly FilterOp Pattern = FilterOp.Instance; ////// 2 children - input, pred /// internal override int Arity { get { return 2; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// ProjectOp /// internal sealed class ProjectOp : RelOp { #region private state private VarVec m_vars; #endregion #region constructors private ProjectOp() : base(OpType.Project) { } internal ProjectOp(VarVec vars) : this() { Debug.Assert(null != vars, "null vars?"); Debug.Assert(!vars.IsEmpty, "empty varlist?"); m_vars = vars; } #endregion #region public methods internal static readonly ProjectOp Pattern = new ProjectOp(); ////// 2 children - input, projections (VarDefList) /// internal override int Arity { get { return 2; } } ////// The Vars projected by this Op /// internal VarVec Outputs { get { return m_vars; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A Sortkey /// internal class SortKey { #region private state private Var m_var; private bool m_asc; private string m_collation; #endregion #region constructors internal SortKey(Var v, bool asc, string collation) { m_var = v; m_asc = asc; m_collation = collation; } #endregion #region public methods ////// The Var being sorted /// internal Var Var { get { return m_var; } set { m_var = value; } } ////// Is this a sort asc, or a sort desc /// internal bool AscendingSort { get { return m_asc; } } ////// An optional collation (only for string types) /// internal string Collation { get { return m_collation; } } #endregion } ////// Base type for SortOp and ConstrainedSortOp /// internal abstract class SortBaseOp : RelOp { #region private state private Listm_keys; #endregion #region Constructors // Pattern constructor internal SortBaseOp(OpType opType) : base(opType) { Debug.Assert(opType == OpType.Sort || opType == OpType.ConstrainedSort, "SortBaseOp OpType must be Sort or ConstrainedSort"); } internal SortBaseOp(OpType opType, List sortKeys) : this(opType) { m_keys = sortKeys; } #endregion /// /// Sort keys /// internal ListKeys { get { return m_keys; } } } /// /// A SortOp /// internal sealed class SortOp : SortBaseOp { #region constructors private SortOp() : base(OpType.Sort) { } internal SortOp(ListsortKeys) : base(OpType.Sort, sortKeys) {} #endregion #region public methods internal static readonly SortOp Pattern = new SortOp(); /// /// 1 child - the input, SortOp must not contain local VarDefs /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// A Constrained SortOp. Used to represent physical paging (skip, limit, skip + limit) operations. /// internal sealed class ConstrainedSortOp : SortBaseOp { #region private state private bool _withTies; #endregion #region constructors // Pattern constructor private ConstrainedSortOp() : base(OpType.ConstrainedSort) { } internal ConstrainedSortOp(ListsortKeys, bool withTies) : base(OpType.ConstrainedSort, sortKeys) { _withTies = withTies; } #endregion #region public methods internal bool WithTies { get { return _withTies; } set { _withTies = value; } } internal static readonly ConstrainedSortOp Pattern = new ConstrainedSortOp(); /// /// 3 children - the input, a possibly NullOp limit and a possibly NullOp skip count. /// internal override int Arity { get { return 3; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// GroupByOp /// internal sealed class GroupByOp : RelOp { #region private state private VarVec m_keys; private VarVec m_outputs; #endregion #region constructors private GroupByOp() : base(OpType.GroupBy) { } internal GroupByOp(VarVec keys, VarVec outputs) : this() { m_keys = keys; m_outputs = outputs; } #endregion #region public methods internal static readonly GroupByOp Pattern = new GroupByOp(); ////// 3 children - input, keys (vardeflist), aggregates (vardeflist) /// internal override int Arity { get { return 3; } } ////// GroupBy keys /// internal VarVec Keys { get { return m_keys; } } ////// All outputs of this Op - includes keys and aggregates /// internal VarVec Outputs { get { return m_outputs; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Base class for set operations - union, intersect, except /// internal abstract class SetOp : RelOp { #region private state private VarMap[] m_varMap; private VarVec m_outputVars; #endregion #region constructors internal SetOp(OpType opType, VarVec outputs, VarMap left, VarMap right) : this(opType) { m_varMap = new VarMap[2]; m_varMap[0] = left; m_varMap[1] = right; m_outputVars = outputs; } protected SetOp(OpType opType) : base(opType) { } #endregion #region public methods ////// 2 children - left, right /// internal override int Arity { get { return 2; } } ////// Map of result vars to the vars of each branch of the setOp /// internal VarMap[] VarMap { get { return m_varMap; } } ////// Get the set of output vars produced /// internal VarVec Outputs { get { return m_outputVars; } } #endregion } ////// UnionAll (ie) no duplicate elimination /// internal sealed class UnionAllOp : SetOp { #region private state private Var m_branchDiscriminator; #endregion #region constructors private UnionAllOp() : base(OpType.UnionAll) { } internal UnionAllOp(VarVec outputs, VarMap left, VarMap right, Var branchDiscriminator) : base(OpType.UnionAll, outputs, left, right) { m_branchDiscriminator = branchDiscriminator; } #endregion #region public methods internal static readonly UnionAllOp Pattern = new UnionAllOp(); ////// Returns the branch discriminator var for this op. It may be null, if /// we haven't been through key pullup yet. /// internal Var BranchDiscriminator { get { return m_branchDiscriminator; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// An IntersectOp /// internal sealed class IntersectOp : SetOp { #region constructors private IntersectOp() : base(OpType.Intersect) { } internal IntersectOp(VarVec outputs, VarMap left, VarMap right) : base(OpType.Intersect, outputs, left,right) { } #endregion #region public methods internal static readonly IntersectOp Pattern = new IntersectOp(); ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// ExceptOp (Minus) /// internal sealed class ExceptOp : SetOp { #region constructors private ExceptOp() : base(OpType.Except) { } internal ExceptOp(VarVec outputs, VarMap left, VarMap right) : base(OpType.Except, outputs, left, right) { } #endregion #region public methods internal static readonly ExceptOp Pattern = new ExceptOp(); ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// DistinctOp /// internal sealed class DistinctOp : RelOp { #region private state private VarVec m_keys; #endregion #region constructors private DistinctOp() : base(OpType.Distinct) { } internal DistinctOp(VarVec keyVars) : this() { Debug.Assert(keyVars != null); Debug.Assert(!keyVars.IsEmpty); m_keys = keyVars; } #endregion #region public methods internal static readonly DistinctOp Pattern = new DistinctOp(); ////// 1 child - input /// internal override int Arity { get { return 1; } } ////// Get "key" vars for the distinct /// internal VarVec Keys { get { return m_keys; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Selects out a single row from a underlying subquery. Two flavors of this Op exist. /// The first flavor enforces the single-row-ness (ie) an error is raised if the /// underlying subquery produces more than one row. /// The other flavor simply choses any row from the input /// internal sealed class SingleRowOp : RelOp { #region constructors private SingleRowOp() : base(OpType.SingleRow) { } #endregion #region public methods ////// Singleton instance /// internal static readonly SingleRowOp Instance = new SingleRowOp(); ////// Pattern for transformation rules /// internal static readonly SingleRowOp Pattern = Instance; ////// 1 child - input /// internal override int Arity { get { return 1; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } /// /// Represents a table with a single row /// internal sealed class SingleRowTableOp : RelOp { #region constructors private SingleRowTableOp() : base(OpType.SingleRowTable) { } #endregion #region public methods ////// Singleton instance /// internal static readonly SingleRowTableOp Instance = new SingleRowTableOp(); ////// Pattern for transformation rules /// internal static readonly SingleRowTableOp Pattern = Instance; ////// 0 children /// internal override int Arity { get { return 0; } } ////// Visitor pattern method /// /// The BasicOpVisitor that is visiting this Op /// The Node that references this Op [DebuggerNonUserCode] internal override void Accept(BasicOpVisitor v, Node n) { v.Visit(this, n); } ////// Visitor pattern method for visitors with a return value /// /// The visitor /// The node in question ///An instance of TResultType [DebuggerNonUserCode] internal override TResultType Accept(BasicOpVisitorOfT v, Node n) { return v.Visit(this, n); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
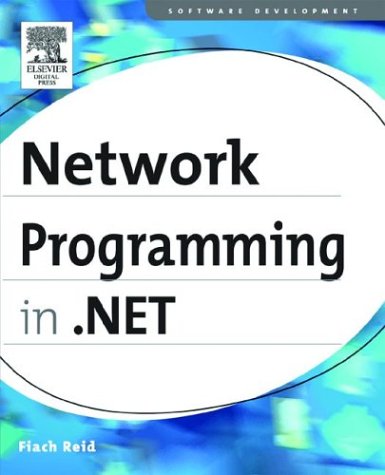
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- HtmlTextArea.cs
- MappingModelBuildProvider.cs
- FlowDocumentPaginator.cs
- _IPv6Address.cs
- WebBrowserNavigatedEventHandler.cs
- GroupPartitionExpr.cs
- SqlCommandBuilder.cs
- WorkflowLayouts.cs
- StandardOleMarshalObject.cs
- RepeaterCommandEventArgs.cs
- PermissionRequestEvidence.cs
- XsdDataContractExporter.cs
- SiteMapNodeItemEventArgs.cs
- FullTrustAssembliesSection.cs
- loginstatus.cs
- ListChangedEventArgs.cs
- TokenDescriptor.cs
- WindowsGrip.cs
- PersonalizationStateInfo.cs
- WindowsSolidBrush.cs
- Pair.cs
- AlphaSortedEnumConverter.cs
- SspiWrapper.cs
- ReadOnlyPermissionSet.cs
- Profiler.cs
- XmlNamespaceManager.cs
- RoleBoolean.cs
- CustomBindingElement.cs
- TreeViewHitTestInfo.cs
- AppDomainUnloadedException.cs
- RowCache.cs
- XmlSerializerFactory.cs
- ControlDesigner.cs
- ping.cs
- CalendarDay.cs
- DoubleConverter.cs
- SyndicationSerializer.cs
- TextCompositionEventArgs.cs
- DragDrop.cs
- EndEvent.cs
- DnsCache.cs
- AmbientLight.cs
- TextFormattingConverter.cs
- EntityDataSourceDataSelection.cs
- PropertyInfo.cs
- ProjectionCamera.cs
- PageRequestManager.cs
- RenderDataDrawingContext.cs
- MSHTMLHost.cs
- DataGridViewIntLinkedList.cs
- ClientEndpointLoader.cs
- DictionaryGlobals.cs
- ConfigXmlSignificantWhitespace.cs
- CodeAttachEventStatement.cs
- TypeConverterValueSerializer.cs
- FileDialogPermission.cs
- MetadataArtifactLoaderCompositeResource.cs
- SettingsPropertyValue.cs
- WindowsBrush.cs
- ResourcePermissionBase.cs
- DataServiceConfiguration.cs
- rsa.cs
- CollectionView.cs
- __Error.cs
- SerializerProvider.cs
- ProviderUtil.cs
- XmlImplementation.cs
- Signature.cs
- SQLBinaryStorage.cs
- RepeatButton.cs
- CalendarDateRangeChangingEventArgs.cs
- Imaging.cs
- TcpActivation.cs
- FieldAccessException.cs
- CellIdBoolean.cs
- ResolveNameEventArgs.cs
- WindowsGrip.cs
- ProcessManager.cs
- ReliableMessagingHelpers.cs
- Win32.cs
- ByeMessageApril2005.cs
- ProviderIncompatibleException.cs
- RadialGradientBrush.cs
- ClrPerspective.cs
- SrgsGrammar.cs
- BlurBitmapEffect.cs
- TextElementAutomationPeer.cs
- UIElementAutomationPeer.cs
- UInt16.cs
- CodeMemberProperty.cs
- XmlLoader.cs
- PropertyItem.cs
- TrackingConditionCollection.cs
- DiagnosticsConfiguration.cs
- DataGridViewUtilities.cs
- NotifyCollectionChangedEventArgs.cs
- TemplatedMailWebEventProvider.cs
- CombinedGeometry.cs
- _FixedSizeReader.cs
- TraceFilter.cs