Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / ndp / fx / src / DataEntityDesign / Design / System / Data / EntityModel / Emitters / NamespaceEmitter.cs / 2 / NamespaceEmitter.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.CodeDom; using System.Data; using Som = System.Data.EntityModel.SchemaObjectModel; using System.Collections.Generic; using System.Data.Entity.Design; using System.Data.Metadata.Edm; using System.Collections.ObjectModel; using System.Diagnostics; using System.Data.Entity.Design.SsdlGenerator; using System.Data.Entity.Design.Common; namespace System.Data.EntityModel.Emitters { ////// This class is responsible for Emitting the code to create the CLR namespace container and assembly level attributes /// internal sealed class NamespaceEmitter : Emitter { #region Static Fields private static Pair[] EmitterCreators = new Pair [] { new Pair (typeof(EntityType), delegate (ClientApiGenerator generator, GlobalItem element) { return new EntityTypeEmitter(generator,(EntityType)element); }), new Pair (typeof(ComplexType), delegate (ClientApiGenerator generator, GlobalItem element) { return new ComplexTypeEmitter(generator,(ComplexType)element); }), new Pair (typeof(EntityContainer), delegate (ClientApiGenerator generator, GlobalItem element) { return new EntityContainerEmitter(generator,(EntityContainer)element); }), new Pair (typeof(AssociationType), delegate (ClientApiGenerator generator, GlobalItem element) { return new AssociationTypeEmitter(generator,(AssociationType)element); }), }; #endregion #region Private Fields private string _codeNamespace = null; private string _targetFilePath = null; #endregion #region Public Methods /// /// /// /// public NamespaceEmitter(ClientApiGenerator generator, string codeNamespace, string targetFilePath) : base(generator) { _codeNamespace = codeNamespace; _targetFilePath = targetFilePath != null ? targetFilePath : string.Empty; } ////// Creates the CodeTypeDeclarations necessary to generate the code /// public void Emit() { // it is a valid scenario for namespaceName to be empty string namespaceName = Generator.SourceObjectNamespaceName; // emit the namespace definition CodeNamespace codeNamespace = new CodeNamespace( namespaceName ); // output some boiler plate comments string comments = Strings.NamespaceComments( System.IO.Path.GetFileName( _targetFilePath ), DateTime.Now.ToString( System.Globalization.CultureInfo.CurrentCulture )); CommentEmitter.EmitComments( CommentEmitter.GetFormattedLines( comments, false ), codeNamespace.Comments, false ); CompileUnit.Namespaces.Add( codeNamespace ); // Add the assembly attribute. CodeAttributeDeclaration assemblyAttribute; // SQLBUDT 505339: VB compiler fails if multiple assembly attributes exist in the same project. // This adds a GUID to the assembly attribute so that each generated file will have a unique EdmSchemaAttribute in VB. if (this.Generator.Language == System.Data.Entity.Design.LanguageOption.GenerateVBCode) //The GUID is only added in VB { assemblyAttribute = AttributeEmitter.EmitSimpleAttribute("System.Data.Objects.DataClasses.EdmSchemaAttribute", System.Guid.NewGuid().ToString()); } else { assemblyAttribute = AttributeEmitter.EmitSimpleAttribute("System.Data.Objects.DataClasses.EdmSchemaAttribute"); } CompileUnit.AssemblyCustomAttributes.Add(assemblyAttribute); DictionaryusedClassName = new Dictionary (StringComparer.Ordinal); // Emit the classes in the schema foreach (GlobalItem element in Generator.GetSourceTypes()) { if (AddElementNameToCache(element, usedClassName)) { SchemaTypeEmitter emitter = CreateElementEmitter(element); CodeTypeDeclarationCollection typeDeclaration = emitter.EmitApiClass(); if (typeDeclaration.Count > 0) { codeNamespace.Types.AddRange(typeDeclaration); } } } } #endregion #region Private Properties /// /// Gets the compile unit (top level codedom object) /// ///private CodeCompileUnit CompileUnit { get { return Generator.CompileUnit; } } #endregion private bool AddElementNameToCache(GlobalItem element, Dictionary cache) { if (element.BuiltInTypeKind == BuiltInTypeKind.EntityContainer) { return TryAddNameToCache((element as EntityContainer).Name, element.BuiltInTypeKind.ToString(), cache); } else if (element.BuiltInTypeKind == BuiltInTypeKind.EntityType || element.BuiltInTypeKind == BuiltInTypeKind.ComplexType || element.BuiltInTypeKind == BuiltInTypeKind.AssociationType) { return TryAddNameToCache((element as StructuralType).Name, element.BuiltInTypeKind.ToString(), cache); } return true; } private bool TryAddNameToCache(string name, string type, Dictionary cache) { if (!cache.ContainsKey(name)) { cache.Add(name, type); } else { this.Generator.AddError(Strings.DuplicateClassName(type, name, cache[name]), ModelBuilderErrorCode.DuplicateClassName, EdmSchemaErrorSeverity.Error, name); return false; } return true; } /// /// Create an Emitter for a schema type element /// /// ///private SchemaTypeEmitter CreateElementEmitter( GlobalItem element ) { Type typeOfElement = element.GetType(); foreach ( Pair pair in EmitterCreators ) { if ( pair.First.IsAssignableFrom( typeOfElement ) ) return pair.Second( Generator, element ); } return null; } private delegate SchemaTypeEmitter CreateEmitter( ClientApiGenerator generator, GlobalItem item ); /// /// Reponsible for relating two objects together into a pair /// ////// private class Pair { public T1 First; public T2 Second; internal Pair( T1 first, T2 second ) { First = first; Second = second; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.CodeDom; using System.Data; using Som = System.Data.EntityModel.SchemaObjectModel; using System.Collections.Generic; using System.Data.Entity.Design; using System.Data.Metadata.Edm; using System.Collections.ObjectModel; using System.Diagnostics; using System.Data.Entity.Design.SsdlGenerator; using System.Data.Entity.Design.Common; namespace System.Data.EntityModel.Emitters { ////// This class is responsible for Emitting the code to create the CLR namespace container and assembly level attributes /// internal sealed class NamespaceEmitter : Emitter { #region Static Fields private static Pair[] EmitterCreators = new Pair [] { new Pair (typeof(EntityType), delegate (ClientApiGenerator generator, GlobalItem element) { return new EntityTypeEmitter(generator,(EntityType)element); }), new Pair (typeof(ComplexType), delegate (ClientApiGenerator generator, GlobalItem element) { return new ComplexTypeEmitter(generator,(ComplexType)element); }), new Pair (typeof(EntityContainer), delegate (ClientApiGenerator generator, GlobalItem element) { return new EntityContainerEmitter(generator,(EntityContainer)element); }), new Pair (typeof(AssociationType), delegate (ClientApiGenerator generator, GlobalItem element) { return new AssociationTypeEmitter(generator,(AssociationType)element); }), }; #endregion #region Private Fields private string _codeNamespace = null; private string _targetFilePath = null; #endregion #region Public Methods /// /// /// /// public NamespaceEmitter(ClientApiGenerator generator, string codeNamespace, string targetFilePath) : base(generator) { _codeNamespace = codeNamespace; _targetFilePath = targetFilePath != null ? targetFilePath : string.Empty; } ////// Creates the CodeTypeDeclarations necessary to generate the code /// public void Emit() { // it is a valid scenario for namespaceName to be empty string namespaceName = Generator.SourceObjectNamespaceName; // emit the namespace definition CodeNamespace codeNamespace = new CodeNamespace( namespaceName ); // output some boiler plate comments string comments = Strings.NamespaceComments( System.IO.Path.GetFileName( _targetFilePath ), DateTime.Now.ToString( System.Globalization.CultureInfo.CurrentCulture )); CommentEmitter.EmitComments( CommentEmitter.GetFormattedLines( comments, false ), codeNamespace.Comments, false ); CompileUnit.Namespaces.Add( codeNamespace ); // Add the assembly attribute. CodeAttributeDeclaration assemblyAttribute; // SQLBUDT 505339: VB compiler fails if multiple assembly attributes exist in the same project. // This adds a GUID to the assembly attribute so that each generated file will have a unique EdmSchemaAttribute in VB. if (this.Generator.Language == System.Data.Entity.Design.LanguageOption.GenerateVBCode) //The GUID is only added in VB { assemblyAttribute = AttributeEmitter.EmitSimpleAttribute("System.Data.Objects.DataClasses.EdmSchemaAttribute", System.Guid.NewGuid().ToString()); } else { assemblyAttribute = AttributeEmitter.EmitSimpleAttribute("System.Data.Objects.DataClasses.EdmSchemaAttribute"); } CompileUnit.AssemblyCustomAttributes.Add(assemblyAttribute); DictionaryusedClassName = new Dictionary (StringComparer.Ordinal); // Emit the classes in the schema foreach (GlobalItem element in Generator.GetSourceTypes()) { if (AddElementNameToCache(element, usedClassName)) { SchemaTypeEmitter emitter = CreateElementEmitter(element); CodeTypeDeclarationCollection typeDeclaration = emitter.EmitApiClass(); if (typeDeclaration.Count > 0) { codeNamespace.Types.AddRange(typeDeclaration); } } } } #endregion #region Private Properties /// /// Gets the compile unit (top level codedom object) /// ///private CodeCompileUnit CompileUnit { get { return Generator.CompileUnit; } } #endregion private bool AddElementNameToCache(GlobalItem element, Dictionary cache) { if (element.BuiltInTypeKind == BuiltInTypeKind.EntityContainer) { return TryAddNameToCache((element as EntityContainer).Name, element.BuiltInTypeKind.ToString(), cache); } else if (element.BuiltInTypeKind == BuiltInTypeKind.EntityType || element.BuiltInTypeKind == BuiltInTypeKind.ComplexType || element.BuiltInTypeKind == BuiltInTypeKind.AssociationType) { return TryAddNameToCache((element as StructuralType).Name, element.BuiltInTypeKind.ToString(), cache); } return true; } private bool TryAddNameToCache(string name, string type, Dictionary cache) { if (!cache.ContainsKey(name)) { cache.Add(name, type); } else { this.Generator.AddError(Strings.DuplicateClassName(type, name, cache[name]), ModelBuilderErrorCode.DuplicateClassName, EdmSchemaErrorSeverity.Error, name); return false; } return true; } /// /// Create an Emitter for a schema type element /// /// ///private SchemaTypeEmitter CreateElementEmitter( GlobalItem element ) { Type typeOfElement = element.GetType(); foreach ( Pair pair in EmitterCreators ) { if ( pair.First.IsAssignableFrom( typeOfElement ) ) return pair.Second( Generator, element ); } return null; } private delegate SchemaTypeEmitter CreateEmitter( ClientApiGenerator generator, GlobalItem item ); /// /// Reponsible for relating two objects together into a pair /// ////// private class Pair { public T1 First; public T2 Second; internal Pair( T1 first, T2 second ) { First = first; Second = second; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
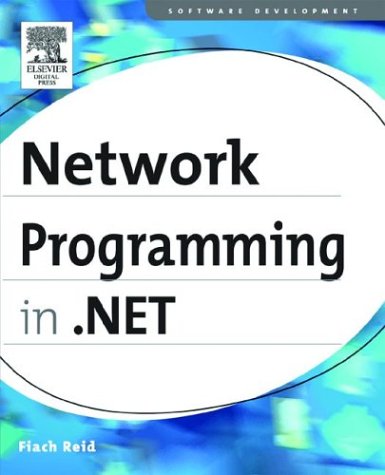
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackBarRenderer.cs
- ExtractorMetadata.cs
- TrackingDataItemValue.cs
- SQLDoubleStorage.cs
- SHA1.cs
- TogglePattern.cs
- PersonalizationProvider.cs
- RegexWriter.cs
- EventRouteFactory.cs
- TypeDescriptor.cs
- ImageField.cs
- WebPartHeaderCloseVerb.cs
- DataGridViewComboBoxCell.cs
- PriorityRange.cs
- X509PeerCertificateAuthentication.cs
- DesignerLabelAdapter.cs
- SwitchLevelAttribute.cs
- CompiledQueryCacheEntry.cs
- XslTransform.cs
- XmlQueryCardinality.cs
- OracleDataAdapter.cs
- HttpWebRequestElement.cs
- Parameter.cs
- _CommandStream.cs
- TableCellCollection.cs
- MetadataItem_Static.cs
- EntityReference.cs
- VisualTreeUtils.cs
- EncoderNLS.cs
- counter.cs
- EntryWrittenEventArgs.cs
- ConstraintConverter.cs
- XPathAxisIterator.cs
- Processor.cs
- SqlDataRecord.cs
- InstanceLockQueryResult.cs
- TableItemPattern.cs
- WebEvents.cs
- EntityParameterCollection.cs
- PointHitTestResult.cs
- CustomAssemblyResolver.cs
- InternalsVisibleToAttribute.cs
- x509utils.cs
- IisTraceWebEventProvider.cs
- RadialGradientBrush.cs
- CalendarDateRangeChangingEventArgs.cs
- Setter.cs
- MethodBuilderInstantiation.cs
- latinshape.cs
- DBPropSet.cs
- PasswordDeriveBytes.cs
- HttpDebugHandler.cs
- ProtocolsConfigurationEntry.cs
- ColorBlend.cs
- LinearQuaternionKeyFrame.cs
- AvTraceDetails.cs
- SafeCryptoKeyHandle.cs
- DataGridItemEventArgs.cs
- MasterPage.cs
- ContextProperty.cs
- Compensation.cs
- JsonCollectionDataContract.cs
- ColorBlend.cs
- AssertSection.cs
- Buffer.cs
- SiteMapDesignerDataSourceView.cs
- DataSysAttribute.cs
- TemplateControlCodeDomTreeGenerator.cs
- XmlSerializerNamespaces.cs
- ExpressionBinding.cs
- ComponentDispatcher.cs
- PeerPresenceInfo.cs
- WinOEToolBoxItem.cs
- TextEditor.cs
- HtmlInputFile.cs
- ClientFormsAuthenticationCredentials.cs
- MenuItem.cs
- HeaderCollection.cs
- Point3D.cs
- RootBrowserWindowAutomationPeer.cs
- EventLogStatus.cs
- StatusBarDesigner.cs
- TTSEngineProxy.cs
- Double.cs
- ProtectedConfiguration.cs
- ExecutionContext.cs
- DateTimeValueSerializerContext.cs
- ImageSource.cs
- Oid.cs
- XmlCharacterData.cs
- ListenerSingletonConnectionReader.cs
- DataGridViewButtonColumn.cs
- StickyNoteContentControl.cs
- ClientApiGenerator.cs
- TreeNodeMouseHoverEvent.cs
- XamlInterfaces.cs
- CustomValidator.cs
- InputScope.cs
- SerializationEventsCache.cs
- DoubleLink.cs