Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / Orcas / QFE / wpf / src / Core / CSharp / System / Windows / Media / GlyphRunDrawing.cs / 1 / GlyphRunDrawing.cs
//---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: GlyphRunDrawing represents a drawing operation that renders // a GlyphRun. // // History: // // 2004/11/17 : timothyc - Created it. // //--------------------------------------------------------------------------- using System.Diagnostics; namespace System.Windows.Media { ////// GlyphRunDrawing represents a drawing operation that renders a GlyphRun. /// public sealed partial class GlyphRunDrawing : Drawing { #region Constructors ////// Default GlyphRunDrawing constructor. /// Constructs an object with all properties set to their default values /// public GlyphRunDrawing() { } ////// Two-argument GlyphRunDrawing constructor. /// Constructs an object with the GlyphRun and ForegroundBrush properties /// set to the value of their respective arguments. /// public GlyphRunDrawing(Brush foregroundBrush, GlyphRun glyphRun) { GlyphRun = glyphRun; ForegroundBrush = foregroundBrush; } #endregion #region Internal methods ////// Called when an object needs to Update it's realizations. /// Currently, GlyphRun is the only MIL object that needs realization /// updates. /// internal override void UpdateRealizations(RealizationContext realizationContext) { if (RequiresRealizationUpdates) { GlyphRun glyphRun = GlyphRun; Brush foregroundBrush = ForegroundBrush; Debug.Assert(glyphRun != null, "Because the RequriesRealizationUpdates flag is only set if GlyphRun != null."); Debug.Assert(foregroundBrush!= null, "Because the RequiresRealizationUpdates flag is only set if ForegroundBrush != null."); Rect bounds = glyphRun.ComputeInkBoundingBox(); if (!bounds.IsEmpty) { Point baselineOrigin = glyphRun.BaselineOrigin; bounds.X += baselineOrigin.X; bounds.Y += baselineOrigin.Y; foregroundBrush.UpdateRealizations(bounds, realizationContext); } } } ////// Calls methods on the DrawingContext that are equivalent to the /// Drawing with the Drawing's current value. /// internal override void WalkCurrentValue(DrawingContextWalker ctx) { // We avoid unneccessary ShouldStopWalking checks based on assumptions // about when ShouldStopWalking is set. Guard that assumption with an // assertion. See DrawingGroup.WalkCurrentValue comment for more details. Debug.Assert(!ctx.ShouldStopWalking); ctx.DrawGlyphRun( ForegroundBrush, GlyphRun ); } ////// PrecomputeCore for GlyphRuns. /// internal override void PrecomputeCore() { // Reset the flag. RequiresRealizationUpdates = false; // Check if we need to push realization updates into the foreground brush. if (GlyphRun != null) { Brush foregroundBrush = ForegroundBrush; if (foregroundBrush != null) { foregroundBrush.Precompute(); // We require realization no matter what because this is a glyph run // drawing. RequiresRealizationUpdates = true; } } } #endregion Internal methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // Description: GlyphRunDrawing represents a drawing operation that renders // a GlyphRun. // // History: // // 2004/11/17 : timothyc - Created it. // //--------------------------------------------------------------------------- using System.Diagnostics; namespace System.Windows.Media { ////// GlyphRunDrawing represents a drawing operation that renders a GlyphRun. /// public sealed partial class GlyphRunDrawing : Drawing { #region Constructors ////// Default GlyphRunDrawing constructor. /// Constructs an object with all properties set to their default values /// public GlyphRunDrawing() { } ////// Two-argument GlyphRunDrawing constructor. /// Constructs an object with the GlyphRun and ForegroundBrush properties /// set to the value of their respective arguments. /// public GlyphRunDrawing(Brush foregroundBrush, GlyphRun glyphRun) { GlyphRun = glyphRun; ForegroundBrush = foregroundBrush; } #endregion #region Internal methods ////// Called when an object needs to Update it's realizations. /// Currently, GlyphRun is the only MIL object that needs realization /// updates. /// internal override void UpdateRealizations(RealizationContext realizationContext) { if (RequiresRealizationUpdates) { GlyphRun glyphRun = GlyphRun; Brush foregroundBrush = ForegroundBrush; Debug.Assert(glyphRun != null, "Because the RequriesRealizationUpdates flag is only set if GlyphRun != null."); Debug.Assert(foregroundBrush!= null, "Because the RequiresRealizationUpdates flag is only set if ForegroundBrush != null."); Rect bounds = glyphRun.ComputeInkBoundingBox(); if (!bounds.IsEmpty) { Point baselineOrigin = glyphRun.BaselineOrigin; bounds.X += baselineOrigin.X; bounds.Y += baselineOrigin.Y; foregroundBrush.UpdateRealizations(bounds, realizationContext); } } } ////// Calls methods on the DrawingContext that are equivalent to the /// Drawing with the Drawing's current value. /// internal override void WalkCurrentValue(DrawingContextWalker ctx) { // We avoid unneccessary ShouldStopWalking checks based on assumptions // about when ShouldStopWalking is set. Guard that assumption with an // assertion. See DrawingGroup.WalkCurrentValue comment for more details. Debug.Assert(!ctx.ShouldStopWalking); ctx.DrawGlyphRun( ForegroundBrush, GlyphRun ); } ////// PrecomputeCore for GlyphRuns. /// internal override void PrecomputeCore() { // Reset the flag. RequiresRealizationUpdates = false; // Check if we need to push realization updates into the foreground brush. if (GlyphRun != null) { Brush foregroundBrush = ForegroundBrush; if (foregroundBrush != null) { foregroundBrush.Precompute(); // We require realization no matter what because this is a glyph run // drawing. RequiresRealizationUpdates = true; } } } #endregion Internal methods } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
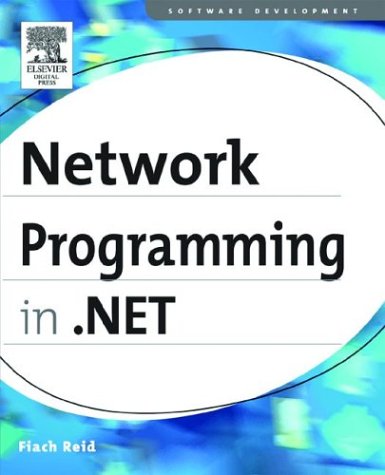
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CurrentChangedEventManager.cs
- PropertyEntry.cs
- MinMaxParagraphWidth.cs
- TextDecoration.cs
- AuthenticateEventArgs.cs
- Stroke.cs
- ProviderSettings.cs
- MaskedTextBox.cs
- RecordManager.cs
- ShapeTypeface.cs
- SocketElement.cs
- HandleCollector.cs
- AdapterUtil.cs
- DataGridViewCellFormattingEventArgs.cs
- WebAdminConfigurationHelper.cs
- SqlCommandBuilder.cs
- LogicalExpressionEditor.cs
- GlobalProxySelection.cs
- BaseValidator.cs
- RowToParametersTransformer.cs
- HybridObjectCache.cs
- MsmqUri.cs
- StorageEntityTypeMapping.cs
- XmlMapping.cs
- TheQuery.cs
- SqlOuterApplyReducer.cs
- GenericTypeParameterBuilder.cs
- Application.cs
- HttpResponseInternalWrapper.cs
- X509ThumbprintKeyIdentifierClause.cs
- FormatterConverter.cs
- BufferedGraphicsContext.cs
- PhysicalAddress.cs
- HttpValueCollection.cs
- WebPartConnectVerb.cs
- TraceContextRecord.cs
- AppDomainAttributes.cs
- InputBinding.cs
- XmlUtil.cs
- ISAPIApplicationHost.cs
- GroupStyle.cs
- MenuAutomationPeer.cs
- MenuTracker.cs
- Function.cs
- CustomErrorCollection.cs
- XmlNavigatorStack.cs
- ProfileEventArgs.cs
- ItemMap.cs
- DESCryptoServiceProvider.cs
- GatewayDefinition.cs
- MatchAttribute.cs
- OutputCacheSettings.cs
- UpdateTranslator.cs
- StackBuilderSink.cs
- HttpCookieCollection.cs
- CustomAssemblyResolver.cs
- DataBindingHandlerAttribute.cs
- BindingManagerDataErrorEventArgs.cs
- DeviceSpecific.cs
- Accessible.cs
- ProxyGenerator.cs
- Certificate.cs
- AdPostCacheSubstitution.cs
- TraceHwndHost.cs
- SQLGuid.cs
- QueryUtil.cs
- UserPreferenceChangingEventArgs.cs
- HtmlInputFile.cs
- TableProvider.cs
- HMAC.cs
- ManipulationCompletedEventArgs.cs
- CodeTypeParameter.cs
- DesignerDataStoredProcedure.cs
- StorageAssociationSetMapping.cs
- HtmlInputFile.cs
- SqlAliasesReferenced.cs
- ErrorLog.cs
- PreDigestedSignedInfo.cs
- ValidationSummary.cs
- OutgoingWebRequestContext.cs
- StylusPointPropertyInfo.cs
- FontDifferentiator.cs
- EditingMode.cs
- WSSecurityOneDotZeroReceiveSecurityHeader.cs
- httpstaticobjectscollection.cs
- BitmapPalettes.cs
- FlowLayoutSettings.cs
- DbDataSourceEnumerator.cs
- TraceHwndHost.cs
- AttributeProviderAttribute.cs
- SqlConnectionStringBuilder.cs
- EntityDataSourceWrapperCollection.cs
- MissingMemberException.cs
- ArrayTypeMismatchException.cs
- TextSelectionProcessor.cs
- ObjectConverter.cs
- Effect.cs
- GenerateTemporaryTargetAssembly.cs
- PseudoWebRequest.cs
- FormViewPageEventArgs.cs