Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / WinForms / Managed / System / WinForms / HtmlWindow.cs / 1 / HtmlWindow.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Drawing.Printing; using System.IO; using System.Net; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; namespace System.Windows.Forms { ////// /// [PermissionSetAttribute(SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class HtmlWindow { internal static readonly object EventError = new object(); internal static readonly object EventGotFocus = new object(); internal static readonly object EventLoad = new object(); internal static readonly object EventLostFocus = new object(); internal static readonly object EventResize = new object(); internal static readonly object EventScroll = new object(); internal static readonly object EventUnload = new object(); private HtmlShimManager shimManager; private UnsafeNativeMethods.IHTMLWindow2 htmlWindow2; [PermissionSet(SecurityAction.Demand, Name = "FullTrust")] internal HtmlWindow(HtmlShimManager shimManager, UnsafeNativeMethods.IHTMLWindow2 win) { this.htmlWindow2 = win; Debug.Assert(this.NativeHtmlWindow != null, "The window object should implement IHTMLWindow2"); this.shimManager = shimManager; } internal UnsafeNativeMethods.IHTMLWindow2 NativeHtmlWindow { get { return this.htmlWindow2; } } private HtmlShimManager ShimManager { get { return shimManager; } } private HtmlWindowShim WindowShim { get { if (ShimManager != null) { HtmlWindowShim shim = ShimManager.GetWindowShim(this); if (shim == null) { shimManager.AddWindowShim(this); shim = ShimManager.GetWindowShim(this); } return shim; } return null; } } ///[To be supplied.] ////// /// public HtmlDocument Document { get { UnsafeNativeMethods.IHTMLDocument iHTMLDocument = this.NativeHtmlWindow.GetDocument() as UnsafeNativeMethods.IHTMLDocument; return iHTMLDocument != null ? new HtmlDocument(ShimManager, iHTMLDocument) : null; } } ///[To be supplied.] ////// /// public object DomWindow { get { return this.NativeHtmlWindow; } } ///[To be supplied.] ////// /// public HtmlWindowCollection Frames { get { UnsafeNativeMethods.IHTMLFramesCollection2 iHTMLFramesCollection2 = this.NativeHtmlWindow.GetFrames(); return (iHTMLFramesCollection2 != null) ? new HtmlWindowCollection(ShimManager, iHTMLFramesCollection2) : null; } } ///[To be supplied.] ////// /// public HtmlHistory History { get { UnsafeNativeMethods.IOmHistory iOmHistory = this.NativeHtmlWindow.GetHistory(); return iOmHistory != null ? new HtmlHistory(iOmHistory) : null; } } ///[To be supplied.] ////// /// public bool IsClosed { get { return this.NativeHtmlWindow.GetClosed(); } } ///[To be supplied.] ////// /// public string Name { get { return this.NativeHtmlWindow.GetName(); } set { this.NativeHtmlWindow.SetName(value); } } ///Name of the NativeHtmlWindow ////// /// public HtmlWindow Opener { get { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.GetOpener() as UnsafeNativeMethods.IHTMLWindow2; return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } } ///[To be supplied.] ////// /// public HtmlWindow Parent { get { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.GetParent(); return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } } ///[To be supplied.] ////// /// public Point Position { get { return new Point(((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).GetScreenLeft(), ((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).GetScreenTop()); } } ///[To be supplied.] ////// /// public Size Size { get { UnsafeNativeMethods.IHTMLElement bodyElement = this.NativeHtmlWindow.GetDocument().GetBody(); return new Size(bodyElement.GetOffsetWidth(), bodyElement.GetOffsetHeight()); } set { ResizeTo(value.Width, value.Height); } } ///Gets or sets size for the window ////// /// public string StatusBarText { get { return this.NativeHtmlWindow.GetStatus(); } set { this.NativeHtmlWindow.SetStatus(value); } } ///[To be supplied.] ////// /// public Uri Url { get { UnsafeNativeMethods.IHTMLLocation iHtmlLocation = this.NativeHtmlWindow.GetLocation(); string stringLocation = (iHtmlLocation == null) ? "" : iHtmlLocation.GetHref(); return string.IsNullOrEmpty(stringLocation) ? null : new Uri(stringLocation); } } ///[To be supplied.] ////// /// public HtmlElement WindowFrameElement { get { UnsafeNativeMethods.IHTMLElement htmlElement = ((UnsafeNativeMethods.IHTMLWindow4)this.NativeHtmlWindow).frameElement() as UnsafeNativeMethods.IHTMLElement; return (htmlElement != null) ? new HtmlElement(ShimManager, htmlElement) : null; } } ///[To be supplied.] ////// /// public void Alert(string message) { this.NativeHtmlWindow.Alert(message); } ///[To be supplied.] ////// /// public void AttachEventHandler(string eventName, EventHandler eventHandler) { WindowShim.AttachEventHandler(eventName, eventHandler); } ///[To be supplied.] ////// /// public void Close() { this.NativeHtmlWindow.Close(); } ///[To be supplied.] ////// /// public bool Confirm(string message) { return this.NativeHtmlWindow.Confirm(message); } ///[To be supplied.] ////// /// public void DetachEventHandler(string eventName, EventHandler eventHandler) { WindowShim.DetachEventHandler(eventName, eventHandler); } ///[To be supplied.] ////// /// public void Focus() { this.NativeHtmlWindow.Focus(); } ///[To be supplied.] ////// /// public void MoveTo(int x, int y) { this.NativeHtmlWindow.MoveTo(x, y); } ///Moves the Window to the position requested ////// /// public void MoveTo(Point point) { this.NativeHtmlWindow.MoveTo(point.X, point.Y); } ///Moves the Window to the point requested ////// /// public void Navigate(Uri url) { this.NativeHtmlWindow.Navigate(url.ToString()); } ///[To be supplied.] ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public void Navigate(string urlString) { this.NativeHtmlWindow.Navigate(urlString); } ///[To be supplied.] ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public HtmlWindow Open(string urlString, string target, string windowOptions, bool replaceEntry) { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.Open(urlString, target, windowOptions, replaceEntry); return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Usage", "CA2234:PassSystemUriObjectsInsteadOfStrings")] public HtmlWindow Open(Uri url, string target, string windowOptions, bool replaceEntry) { return Open(url.ToString(), target, windowOptions, replaceEntry); } ///[To be supplied.] ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public HtmlWindow OpenNew(string urlString, string windowOptions) { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.Open(urlString, "_blank", windowOptions, true); return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Usage", "CA2234:PassSystemUriObjectsInsteadOfStrings")] public HtmlWindow OpenNew(Uri url, string windowOptions) { return OpenNew(url.ToString(), windowOptions); } ///[To be supplied.] ////// /// public string Prompt(string message, string defaultInputValue) { return this.NativeHtmlWindow.Prompt(message, defaultInputValue).ToString(); } ///[To be supplied.] ////// /// public void RemoveFocus() { this.NativeHtmlWindow.Blur(); } ///[To be supplied.] ////// /// public void ResizeTo(int width, int height) { this.NativeHtmlWindow.ResizeTo(width, height); } ///Resize the window to the width/height requested ////// public void ResizeTo(Size size) { this.NativeHtmlWindow.ResizeTo(size.Width, size.Height); } ///Resize the window to the Size requested ////// /// public void ScrollTo(int x, int y) { this.NativeHtmlWindow.ScrollTo(x, y); } ///Scroll the window to the position requested ////// /// public void ScrollTo(Point point) { this.NativeHtmlWindow.ScrollTo(point.X, point.Y); } // // Events // ///Scroll the window to the point requested ////// /// public event HtmlElementErrorEventHandler Error { add { WindowShim.AddHandler(EventError, value); } remove { WindowShim.RemoveHandler(EventError, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler GotFocus { add { WindowShim.AddHandler(EventGotFocus, value); } remove { WindowShim.RemoveHandler(EventGotFocus, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Load { add { WindowShim.AddHandler(EventLoad, value); } remove { WindowShim.RemoveHandler(EventLoad, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler LostFocus { add { WindowShim.AddHandler(EventLostFocus, value); } remove { WindowShim.RemoveHandler(EventLostFocus, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Resize { add { WindowShim.AddHandler(EventResize, value); } remove { WindowShim.RemoveHandler(EventResize, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Scroll { add { WindowShim.AddHandler(EventScroll, value); } remove { WindowShim.RemoveHandler(EventScroll, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Unload { add { WindowShim.AddHandler(EventUnload, value); } remove { WindowShim.RemoveHandler(EventUnload, value); } } // // Private classes: // [ClassInterface(ClassInterfaceType.None)] private class HTMLWindowEvents2 : StandardOleMarshalObject, /*Enforce calling back on the same thread*/ UnsafeNativeMethods.DHTMLWindowEvents2 { private HtmlWindow parent; public HTMLWindowEvents2(HtmlWindow htmlWindow) { this.parent = htmlWindow; } private void FireEvent(object key, EventArgs e) { if (this.parent != null) { parent.WindowShim.FireEvent(key, e); } } public void onfocus(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventGotFocus, e); } public void onblur(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventLostFocus, e); } public bool onerror(string description, string urlString, int line) { HtmlElementErrorEventArgs e = new HtmlElementErrorEventArgs(description, urlString, line); FireEvent(HtmlWindow.EventError, e); return e.Handled; } public void onload(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventLoad, e); } public void onunload(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventUnload, e); if (parent != null) { parent.WindowShim.OnWindowUnload(); } } public void onscroll(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventScroll, e); } public void onresize(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventResize, e); } public bool onhelp(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); return e.ReturnValue; } public void onbeforeunload(UnsafeNativeMethods.IHTMLEventObj evtObj) { } public void onbeforeprint(UnsafeNativeMethods.IHTMLEventObj evtObj) { } public void onafterprint(UnsafeNativeMethods.IHTMLEventObj evtObj) { } } ///[To be supplied.] ////// HtmlWindowShim - this is the glue between the DOM eventing mechanisms /// and our CLR callbacks. /// /// There are two kinds of events: HTMLWindowEvents2 and IHtmlWindow3.AttachHandler style /// HTMLWindowEvents2: we create an IConnectionPoint (via ConnectionPointCookie) between us and MSHTML and it calls back /// on an instance of HTMLWindowEvents2. The HTMLWindowEvents2 class then fires the event. /// /// IHTMLWindow3.AttachHandler: MSHML calls back on an HtmlToClrEventProxy that we've created, looking /// for a method named DISPID=0. For each event that's subscribed, we create /// a new HtmlToClrEventProxy, detect the callback and fire the corresponding /// CLR event. /// internal class HtmlWindowShim : HtmlShim { private AxHost.ConnectionPointCookie cookie; private HtmlWindow htmlWindow; public HtmlWindowShim(HtmlWindow window) { this.htmlWindow = window; } public UnsafeNativeMethods.IHTMLWindow2 NativeHtmlWindow { get { return htmlWindow.NativeHtmlWindow; } } public override UnsafeNativeMethods.IHTMLWindow2 AssociatedWindow { get { return htmlWindow.NativeHtmlWindow; } } /// Support IHtmlDocument3.AttachHandler public override void AttachEventHandler(string eventName, System.EventHandler eventHandler) { // IE likes to call back on an IDispatch of DISPID=0 when it has an event, // the HtmlToClrEventProxy helps us fake out the CLR so that we can call back on // our EventHandler properly. HtmlToClrEventProxy proxy = AddEventProxy(eventName, eventHandler); bool success = ((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).AttachEvent(eventName, proxy); Debug.Assert(success, "failed to add event"); } /// Support HTMLWindowEvents2 public override void ConnectToEvents() { if (cookie == null || !cookie.Connected) { this.cookie = new AxHost.ConnectionPointCookie(NativeHtmlWindow, new HTMLWindowEvents2(htmlWindow), typeof(UnsafeNativeMethods.DHTMLWindowEvents2), /*throwException*/ false); if (!cookie.Connected) { cookie = null; } } } /// Support IHTMLWindow3.DetachHandler public override void DetachEventHandler(string eventName, System.EventHandler eventHandler) { HtmlToClrEventProxy proxy = RemoveEventProxy(eventHandler); if (proxy != null) { ((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).DetachEvent(eventName, proxy); } } public override void DisconnectFromEvents() { if (this.cookie != null) { this.cookie.Disconnect(); this.cookie = null; } } protected override void Dispose(bool disposing) { base.Dispose(disposing); if (disposing) { if (htmlWindow != null && htmlWindow.NativeHtmlWindow != null) { Marshal.FinalReleaseComObject(htmlWindow.NativeHtmlWindow); } htmlWindow = null; } } protected override object GetEventSender() { return htmlWindow; } public void OnWindowUnload() { if (htmlWindow != null) { htmlWindow.ShimManager.OnWindowUnloaded(htmlWindow); } } } #region operators ///[SuppressMessage("Microsoft.Design", "CA1046:DoNotOverrideOperatorEqualsOnReferenceTypes")] public static bool operator ==(HtmlWindow left, HtmlWindow right) { //Not equal if only one's null. if (object.ReferenceEquals(left, null) != object.ReferenceEquals(right, null)) { return false; } //Equal if both are null. if (object.ReferenceEquals(left, null)) { return true; } //Neither are null. Get the IUnknowns and compare them. IntPtr leftPtr = IntPtr.Zero; IntPtr rightPtr = IntPtr.Zero; try { leftPtr = Marshal.GetIUnknownForObject(left.NativeHtmlWindow); rightPtr = Marshal.GetIUnknownForObject(right.NativeHtmlWindow); return leftPtr == rightPtr; } finally { if (leftPtr != IntPtr.Zero) { Marshal.Release(leftPtr); } if (rightPtr != IntPtr.Zero) { Marshal.Release(rightPtr); } } } /// public static bool operator !=(HtmlWindow left, HtmlWindow right) { return !(left == right); } /// public override int GetHashCode() { return htmlWindow2 == null ? 0 : htmlWindow2.GetHashCode(); } /// public override bool Equals(object obj) { return (this == (HtmlWindow)obj); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System.Drawing; using System.Drawing.Printing; using System.IO; using System.Net; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; namespace System.Windows.Forms { ////// /// [PermissionSetAttribute(SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class HtmlWindow { internal static readonly object EventError = new object(); internal static readonly object EventGotFocus = new object(); internal static readonly object EventLoad = new object(); internal static readonly object EventLostFocus = new object(); internal static readonly object EventResize = new object(); internal static readonly object EventScroll = new object(); internal static readonly object EventUnload = new object(); private HtmlShimManager shimManager; private UnsafeNativeMethods.IHTMLWindow2 htmlWindow2; [PermissionSet(SecurityAction.Demand, Name = "FullTrust")] internal HtmlWindow(HtmlShimManager shimManager, UnsafeNativeMethods.IHTMLWindow2 win) { this.htmlWindow2 = win; Debug.Assert(this.NativeHtmlWindow != null, "The window object should implement IHTMLWindow2"); this.shimManager = shimManager; } internal UnsafeNativeMethods.IHTMLWindow2 NativeHtmlWindow { get { return this.htmlWindow2; } } private HtmlShimManager ShimManager { get { return shimManager; } } private HtmlWindowShim WindowShim { get { if (ShimManager != null) { HtmlWindowShim shim = ShimManager.GetWindowShim(this); if (shim == null) { shimManager.AddWindowShim(this); shim = ShimManager.GetWindowShim(this); } return shim; } return null; } } ///[To be supplied.] ////// /// public HtmlDocument Document { get { UnsafeNativeMethods.IHTMLDocument iHTMLDocument = this.NativeHtmlWindow.GetDocument() as UnsafeNativeMethods.IHTMLDocument; return iHTMLDocument != null ? new HtmlDocument(ShimManager, iHTMLDocument) : null; } } ///[To be supplied.] ////// /// public object DomWindow { get { return this.NativeHtmlWindow; } } ///[To be supplied.] ////// /// public HtmlWindowCollection Frames { get { UnsafeNativeMethods.IHTMLFramesCollection2 iHTMLFramesCollection2 = this.NativeHtmlWindow.GetFrames(); return (iHTMLFramesCollection2 != null) ? new HtmlWindowCollection(ShimManager, iHTMLFramesCollection2) : null; } } ///[To be supplied.] ////// /// public HtmlHistory History { get { UnsafeNativeMethods.IOmHistory iOmHistory = this.NativeHtmlWindow.GetHistory(); return iOmHistory != null ? new HtmlHistory(iOmHistory) : null; } } ///[To be supplied.] ////// /// public bool IsClosed { get { return this.NativeHtmlWindow.GetClosed(); } } ///[To be supplied.] ////// /// public string Name { get { return this.NativeHtmlWindow.GetName(); } set { this.NativeHtmlWindow.SetName(value); } } ///Name of the NativeHtmlWindow ////// /// public HtmlWindow Opener { get { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.GetOpener() as UnsafeNativeMethods.IHTMLWindow2; return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } } ///[To be supplied.] ////// /// public HtmlWindow Parent { get { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.GetParent(); return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } } ///[To be supplied.] ////// /// public Point Position { get { return new Point(((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).GetScreenLeft(), ((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).GetScreenTop()); } } ///[To be supplied.] ////// /// public Size Size { get { UnsafeNativeMethods.IHTMLElement bodyElement = this.NativeHtmlWindow.GetDocument().GetBody(); return new Size(bodyElement.GetOffsetWidth(), bodyElement.GetOffsetHeight()); } set { ResizeTo(value.Width, value.Height); } } ///Gets or sets size for the window ////// /// public string StatusBarText { get { return this.NativeHtmlWindow.GetStatus(); } set { this.NativeHtmlWindow.SetStatus(value); } } ///[To be supplied.] ////// /// public Uri Url { get { UnsafeNativeMethods.IHTMLLocation iHtmlLocation = this.NativeHtmlWindow.GetLocation(); string stringLocation = (iHtmlLocation == null) ? "" : iHtmlLocation.GetHref(); return string.IsNullOrEmpty(stringLocation) ? null : new Uri(stringLocation); } } ///[To be supplied.] ////// /// public HtmlElement WindowFrameElement { get { UnsafeNativeMethods.IHTMLElement htmlElement = ((UnsafeNativeMethods.IHTMLWindow4)this.NativeHtmlWindow).frameElement() as UnsafeNativeMethods.IHTMLElement; return (htmlElement != null) ? new HtmlElement(ShimManager, htmlElement) : null; } } ///[To be supplied.] ////// /// public void Alert(string message) { this.NativeHtmlWindow.Alert(message); } ///[To be supplied.] ////// /// public void AttachEventHandler(string eventName, EventHandler eventHandler) { WindowShim.AttachEventHandler(eventName, eventHandler); } ///[To be supplied.] ////// /// public void Close() { this.NativeHtmlWindow.Close(); } ///[To be supplied.] ////// /// public bool Confirm(string message) { return this.NativeHtmlWindow.Confirm(message); } ///[To be supplied.] ////// /// public void DetachEventHandler(string eventName, EventHandler eventHandler) { WindowShim.DetachEventHandler(eventName, eventHandler); } ///[To be supplied.] ////// /// public void Focus() { this.NativeHtmlWindow.Focus(); } ///[To be supplied.] ////// /// public void MoveTo(int x, int y) { this.NativeHtmlWindow.MoveTo(x, y); } ///Moves the Window to the position requested ////// /// public void MoveTo(Point point) { this.NativeHtmlWindow.MoveTo(point.X, point.Y); } ///Moves the Window to the point requested ////// /// public void Navigate(Uri url) { this.NativeHtmlWindow.Navigate(url.ToString()); } ///[To be supplied.] ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public void Navigate(string urlString) { this.NativeHtmlWindow.Navigate(urlString); } ///[To be supplied.] ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public HtmlWindow Open(string urlString, string target, string windowOptions, bool replaceEntry) { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.Open(urlString, target, windowOptions, replaceEntry); return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Usage", "CA2234:PassSystemUriObjectsInsteadOfStrings")] public HtmlWindow Open(Uri url, string target, string windowOptions, bool replaceEntry) { return Open(url.ToString(), target, windowOptions, replaceEntry); } ///[To be supplied.] ////// /// /// Note: We intentionally have a string overload (apparently Mort wants one). We don't have /// string overloads call Uri overloads because that breaks Uris that aren't fully qualified /// (things like "www.microsoft.com") that the underlying objects support and we don't want to /// break. [SuppressMessage("Microsoft.Design", "CA1057:StringUriOverloadsCallSystemUriOverloads")] public HtmlWindow OpenNew(string urlString, string windowOptions) { UnsafeNativeMethods.IHTMLWindow2 iHTMLWindow2 = this.NativeHtmlWindow.Open(urlString, "_blank", windowOptions, true); return (iHTMLWindow2 != null) ? new HtmlWindow(ShimManager, iHTMLWindow2) : null; } ///[To be supplied.] ////// /// [SuppressMessage("Microsoft.Usage", "CA2234:PassSystemUriObjectsInsteadOfStrings")] public HtmlWindow OpenNew(Uri url, string windowOptions) { return OpenNew(url.ToString(), windowOptions); } ///[To be supplied.] ////// /// public string Prompt(string message, string defaultInputValue) { return this.NativeHtmlWindow.Prompt(message, defaultInputValue).ToString(); } ///[To be supplied.] ////// /// public void RemoveFocus() { this.NativeHtmlWindow.Blur(); } ///[To be supplied.] ////// /// public void ResizeTo(int width, int height) { this.NativeHtmlWindow.ResizeTo(width, height); } ///Resize the window to the width/height requested ////// public void ResizeTo(Size size) { this.NativeHtmlWindow.ResizeTo(size.Width, size.Height); } ///Resize the window to the Size requested ////// /// public void ScrollTo(int x, int y) { this.NativeHtmlWindow.ScrollTo(x, y); } ///Scroll the window to the position requested ////// /// public void ScrollTo(Point point) { this.NativeHtmlWindow.ScrollTo(point.X, point.Y); } // // Events // ///Scroll the window to the point requested ////// /// public event HtmlElementErrorEventHandler Error { add { WindowShim.AddHandler(EventError, value); } remove { WindowShim.RemoveHandler(EventError, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler GotFocus { add { WindowShim.AddHandler(EventGotFocus, value); } remove { WindowShim.RemoveHandler(EventGotFocus, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Load { add { WindowShim.AddHandler(EventLoad, value); } remove { WindowShim.RemoveHandler(EventLoad, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler LostFocus { add { WindowShim.AddHandler(EventLostFocus, value); } remove { WindowShim.RemoveHandler(EventLostFocus, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Resize { add { WindowShim.AddHandler(EventResize, value); } remove { WindowShim.RemoveHandler(EventResize, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Scroll { add { WindowShim.AddHandler(EventScroll, value); } remove { WindowShim.RemoveHandler(EventScroll, value); } } ///[To be supplied.] ////// /// public event HtmlElementEventHandler Unload { add { WindowShim.AddHandler(EventUnload, value); } remove { WindowShim.RemoveHandler(EventUnload, value); } } // // Private classes: // [ClassInterface(ClassInterfaceType.None)] private class HTMLWindowEvents2 : StandardOleMarshalObject, /*Enforce calling back on the same thread*/ UnsafeNativeMethods.DHTMLWindowEvents2 { private HtmlWindow parent; public HTMLWindowEvents2(HtmlWindow htmlWindow) { this.parent = htmlWindow; } private void FireEvent(object key, EventArgs e) { if (this.parent != null) { parent.WindowShim.FireEvent(key, e); } } public void onfocus(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventGotFocus, e); } public void onblur(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventLostFocus, e); } public bool onerror(string description, string urlString, int line) { HtmlElementErrorEventArgs e = new HtmlElementErrorEventArgs(description, urlString, line); FireEvent(HtmlWindow.EventError, e); return e.Handled; } public void onload(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventLoad, e); } public void onunload(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventUnload, e); if (parent != null) { parent.WindowShim.OnWindowUnload(); } } public void onscroll(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventScroll, e); } public void onresize(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); FireEvent(HtmlWindow.EventResize, e); } public bool onhelp(UnsafeNativeMethods.IHTMLEventObj evtObj) { HtmlElementEventArgs e = new HtmlElementEventArgs(parent.ShimManager, evtObj); return e.ReturnValue; } public void onbeforeunload(UnsafeNativeMethods.IHTMLEventObj evtObj) { } public void onbeforeprint(UnsafeNativeMethods.IHTMLEventObj evtObj) { } public void onafterprint(UnsafeNativeMethods.IHTMLEventObj evtObj) { } } ///[To be supplied.] ////// HtmlWindowShim - this is the glue between the DOM eventing mechanisms /// and our CLR callbacks. /// /// There are two kinds of events: HTMLWindowEvents2 and IHtmlWindow3.AttachHandler style /// HTMLWindowEvents2: we create an IConnectionPoint (via ConnectionPointCookie) between us and MSHTML and it calls back /// on an instance of HTMLWindowEvents2. The HTMLWindowEvents2 class then fires the event. /// /// IHTMLWindow3.AttachHandler: MSHML calls back on an HtmlToClrEventProxy that we've created, looking /// for a method named DISPID=0. For each event that's subscribed, we create /// a new HtmlToClrEventProxy, detect the callback and fire the corresponding /// CLR event. /// internal class HtmlWindowShim : HtmlShim { private AxHost.ConnectionPointCookie cookie; private HtmlWindow htmlWindow; public HtmlWindowShim(HtmlWindow window) { this.htmlWindow = window; } public UnsafeNativeMethods.IHTMLWindow2 NativeHtmlWindow { get { return htmlWindow.NativeHtmlWindow; } } public override UnsafeNativeMethods.IHTMLWindow2 AssociatedWindow { get { return htmlWindow.NativeHtmlWindow; } } /// Support IHtmlDocument3.AttachHandler public override void AttachEventHandler(string eventName, System.EventHandler eventHandler) { // IE likes to call back on an IDispatch of DISPID=0 when it has an event, // the HtmlToClrEventProxy helps us fake out the CLR so that we can call back on // our EventHandler properly. HtmlToClrEventProxy proxy = AddEventProxy(eventName, eventHandler); bool success = ((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).AttachEvent(eventName, proxy); Debug.Assert(success, "failed to add event"); } /// Support HTMLWindowEvents2 public override void ConnectToEvents() { if (cookie == null || !cookie.Connected) { this.cookie = new AxHost.ConnectionPointCookie(NativeHtmlWindow, new HTMLWindowEvents2(htmlWindow), typeof(UnsafeNativeMethods.DHTMLWindowEvents2), /*throwException*/ false); if (!cookie.Connected) { cookie = null; } } } /// Support IHTMLWindow3.DetachHandler public override void DetachEventHandler(string eventName, System.EventHandler eventHandler) { HtmlToClrEventProxy proxy = RemoveEventProxy(eventHandler); if (proxy != null) { ((UnsafeNativeMethods.IHTMLWindow3)this.NativeHtmlWindow).DetachEvent(eventName, proxy); } } public override void DisconnectFromEvents() { if (this.cookie != null) { this.cookie.Disconnect(); this.cookie = null; } } protected override void Dispose(bool disposing) { base.Dispose(disposing); if (disposing) { if (htmlWindow != null && htmlWindow.NativeHtmlWindow != null) { Marshal.FinalReleaseComObject(htmlWindow.NativeHtmlWindow); } htmlWindow = null; } } protected override object GetEventSender() { return htmlWindow; } public void OnWindowUnload() { if (htmlWindow != null) { htmlWindow.ShimManager.OnWindowUnloaded(htmlWindow); } } } #region operators ///[SuppressMessage("Microsoft.Design", "CA1046:DoNotOverrideOperatorEqualsOnReferenceTypes")] public static bool operator ==(HtmlWindow left, HtmlWindow right) { //Not equal if only one's null. if (object.ReferenceEquals(left, null) != object.ReferenceEquals(right, null)) { return false; } //Equal if both are null. if (object.ReferenceEquals(left, null)) { return true; } //Neither are null. Get the IUnknowns and compare them. IntPtr leftPtr = IntPtr.Zero; IntPtr rightPtr = IntPtr.Zero; try { leftPtr = Marshal.GetIUnknownForObject(left.NativeHtmlWindow); rightPtr = Marshal.GetIUnknownForObject(right.NativeHtmlWindow); return leftPtr == rightPtr; } finally { if (leftPtr != IntPtr.Zero) { Marshal.Release(leftPtr); } if (rightPtr != IntPtr.Zero) { Marshal.Release(rightPtr); } } } /// public static bool operator !=(HtmlWindow left, HtmlWindow right) { return !(left == right); } /// public override int GetHashCode() { return htmlWindow2 == null ? 0 : htmlWindow2.GetHashCode(); } /// public override bool Equals(object obj) { return (this == (HtmlWindow)obj); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
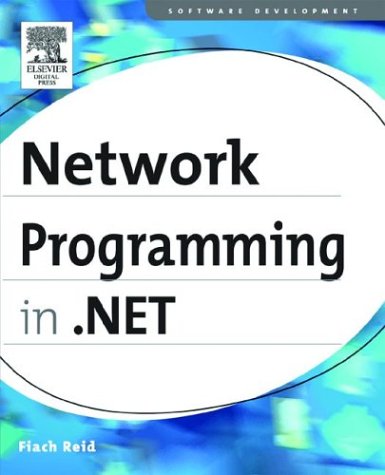
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TransportDefaults.cs
- CompiledXpathExpr.cs
- ForEachAction.cs
- Metadata.cs
- SqlCacheDependency.cs
- Composition.cs
- InteropAutomationProvider.cs
- TreeChangeInfo.cs
- ThrowHelper.cs
- CookieParameter.cs
- Console.cs
- BindableTemplateBuilder.cs
- ImmutableAssemblyCacheEntry.cs
- ShapingEngine.cs
- EntityDataSourceDesignerHelper.cs
- ProcessModelInfo.cs
- TextEditorContextMenu.cs
- TextEncodedRawTextWriter.cs
- GridProviderWrapper.cs
- IntranetCredentialPolicy.cs
- ModulesEntry.cs
- RestHandler.cs
- _NegoStream.cs
- ProfileEventArgs.cs
- SecurityTokenAttachmentMode.cs
- MatrixTransform.cs
- HealthMonitoringSection.cs
- CompositeDispatchFormatter.cs
- WebFaultClientMessageInspector.cs
- SystemFonts.cs
- PackageRelationship.cs
- PageAsyncTaskManager.cs
- MaskedTextProvider.cs
- SchemaInfo.cs
- SpecularMaterial.cs
- DynamicExpression.cs
- AmbientProperties.cs
- ProxyFragment.cs
- AttributeSetAction.cs
- DesignerExtenders.cs
- _KerberosClient.cs
- HTTPNotFoundHandler.cs
- X509ClientCertificateCredentialsElement.cs
- MailHeaderInfo.cs
- DocumentXmlWriter.cs
- InputMethodStateTypeInfo.cs
- ExternalDataExchangeService.cs
- BinHexDecoder.cs
- RoutedEventHandlerInfo.cs
- CompoundFileStorageReference.cs
- ExpressionEditor.cs
- Int32Animation.cs
- Timeline.cs
- FlowDocumentScrollViewerAutomationPeer.cs
- PenLineJoinValidation.cs
- XpsSerializerFactory.cs
- NoResizeSelectionBorderGlyph.cs
- SqlTriggerContext.cs
- Region.cs
- ObjectTypeMapping.cs
- ByteRangeDownloader.cs
- ClientOptions.cs
- Touch.cs
- DeliveryRequirementsAttribute.cs
- ClientSettings.cs
- BypassElementCollection.cs
- SplayTreeNode.cs
- ResizeGrip.cs
- Int32Animation.cs
- NonDualMessageSecurityOverHttp.cs
- NetMsmqBindingElement.cs
- PolyLineSegmentFigureLogic.cs
- HideDisabledControlAdapter.cs
- ValidationErrorCollection.cs
- OutputCacheProfile.cs
- CheckBoxPopupAdapter.cs
- ReachDocumentReferenceCollectionSerializer.cs
- GridEntryCollection.cs
- VectorAnimation.cs
- FixedNode.cs
- DataGridState.cs
- XmlAggregates.cs
- DataGridViewCellFormattingEventArgs.cs
- WindowInteractionStateTracker.cs
- DataTable.cs
- BindingValueChangedEventArgs.cs
- PowerStatus.cs
- PopOutPanel.cs
- ParameterElement.cs
- EarlyBoundInfo.cs
- WebPartTransformerAttribute.cs
- RuntimeCompatibilityAttribute.cs
- XmlNodeChangedEventArgs.cs
- HitTestDrawingContextWalker.cs
- OrderedDictionary.cs
- ExternalFile.cs
- RelationshipDetailsRow.cs
- SymbolEqualComparer.cs
- CommandArguments.cs
- StyleTypedPropertyAttribute.cs