Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / ndp / fx / src / DataWebControls / System / Data / WebControls / WebControlParameterProxy.cs / 1 / WebControlParameterProxy.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner objsdev //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Web.UI.WebControls; using System.Diagnostics; using System.Data; using System.Globalization; namespace System.Web.UI.WebControls { internal class WebControlParameterProxy { ParameterCollection _collection; EntityDataSource _entityDataSource; Parameter _parameter; // Can be null, that's why this class doesn't subclass Parameter internal WebControlParameterProxy(string propertyName, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); Debug.Assert(!String.IsNullOrEmpty(propertyName)); _parameter = EntityDataSourceUtil.GetParameter(propertyName, parameterCollection); _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal WebControlParameterProxy(Parameter parameter, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); _parameter = parameter; _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal string Name { get { if (null != _parameter) { return _parameter.Name; } return null; } } internal bool HasValue { get { return null != _parameter && null != Value; } } internal bool ConvertEmptyStringToNull { get { if (null != _parameter) { return _parameter.ConvertEmptyStringToNull; } return false; } } internal TypeCode TypeCode { get { if (null != _parameter) { return _parameter.Type; } return TypeCode.Empty; } } internal DbType DbType { get { if (null != _parameter) { return _parameter.DbType; } return DbType.Object; } } internal Type ClrType { get { Debug.Assert(this.TypeCode != TypeCode.Empty || this.DbType != DbType.Object, "Need to have TypeCode or DbType to get a ClrType"); if (this.TypeCode != TypeCode.Empty) { return EntityDataSourceUtil.ConvertTypeCodeToType(this.TypeCode); } return EntityDataSourceUtil.ConvertTypeCodeToType( EntityDataSourceUtil.ConvertDbTypeToTypeCode(this.DbType)); } } internal object Value { get { if (_parameter != null) { object paramValue = EntityDataSourceUtil.GetParameterValue(_parameter.Name, _collection, _entityDataSource); if (paramValue != null) { if (this.DbType == DbType.DateTimeOffset) { object value = (paramValue is DateTimeOffset) ? paramValue : DateTimeOffset.Parse(this.Value.ToString(), CultureInfo.CurrentCulture); return value; } else if (this.DbType == DbType.Time) { object value = (paramValue is TimeSpan) ? paramValue : TimeSpan.Parse(paramValue.ToString()); return value; } else if (this.DbType == DbType.Guid) { object value = (paramValue is Guid) ? paramValue : new Guid(paramValue.ToString()); return value; } } return paramValue; } return null; } } private static void VerifyUniqueType(Parameter parameter) { if (parameter != null && parameter.Type == TypeCode.Empty && parameter.DbType == DbType.Object) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } if (parameter != null && parameter.DbType != DbType.Object && parameter.Type != TypeCode.Empty) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner objsdev //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Web.UI.WebControls; using System.Diagnostics; using System.Data; using System.Globalization; namespace System.Web.UI.WebControls { internal class WebControlParameterProxy { ParameterCollection _collection; EntityDataSource _entityDataSource; Parameter _parameter; // Can be null, that's why this class doesn't subclass Parameter internal WebControlParameterProxy(string propertyName, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); Debug.Assert(!String.IsNullOrEmpty(propertyName)); _parameter = EntityDataSourceUtil.GetParameter(propertyName, parameterCollection); _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal WebControlParameterProxy(Parameter parameter, ParameterCollection parameterCollection, EntityDataSource entityDataSource) { Debug.Assert(null != entityDataSource); _parameter = parameter; _collection = parameterCollection; _entityDataSource = entityDataSource; VerifyUniqueType(_parameter); } internal string Name { get { if (null != _parameter) { return _parameter.Name; } return null; } } internal bool HasValue { get { return null != _parameter && null != Value; } } internal bool ConvertEmptyStringToNull { get { if (null != _parameter) { return _parameter.ConvertEmptyStringToNull; } return false; } } internal TypeCode TypeCode { get { if (null != _parameter) { return _parameter.Type; } return TypeCode.Empty; } } internal DbType DbType { get { if (null != _parameter) { return _parameter.DbType; } return DbType.Object; } } internal Type ClrType { get { Debug.Assert(this.TypeCode != TypeCode.Empty || this.DbType != DbType.Object, "Need to have TypeCode or DbType to get a ClrType"); if (this.TypeCode != TypeCode.Empty) { return EntityDataSourceUtil.ConvertTypeCodeToType(this.TypeCode); } return EntityDataSourceUtil.ConvertTypeCodeToType( EntityDataSourceUtil.ConvertDbTypeToTypeCode(this.DbType)); } } internal object Value { get { if (_parameter != null) { object paramValue = EntityDataSourceUtil.GetParameterValue(_parameter.Name, _collection, _entityDataSource); if (paramValue != null) { if (this.DbType == DbType.DateTimeOffset) { object value = (paramValue is DateTimeOffset) ? paramValue : DateTimeOffset.Parse(this.Value.ToString(), CultureInfo.CurrentCulture); return value; } else if (this.DbType == DbType.Time) { object value = (paramValue is TimeSpan) ? paramValue : TimeSpan.Parse(paramValue.ToString()); return value; } else if (this.DbType == DbType.Guid) { object value = (paramValue is Guid) ? paramValue : new Guid(paramValue.ToString()); return value; } } return paramValue; } return null; } } private static void VerifyUniqueType(Parameter parameter) { if (parameter != null && parameter.Type == TypeCode.Empty && parameter.DbType == DbType.Object) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } if (parameter != null && parameter.DbType != DbType.Object && parameter.Type != TypeCode.Empty) { throw new InvalidOperationException(Strings.WebControlParameterProxy_TypeDbTypeMutuallyExclusive); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
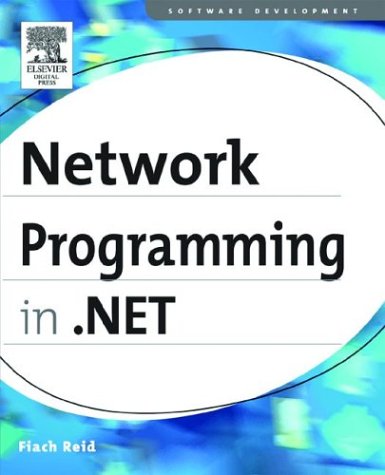
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TableCell.cs
- SqlDataSourceEnumerator.cs
- StreamBodyWriter.cs
- UrlPath.cs
- ListItemConverter.cs
- DataMisalignedException.cs
- RuntimeArgument.cs
- HtmlTernaryTree.cs
- WinFormsComponentEditor.cs
- SqlFlattener.cs
- OutputCacheModule.cs
- CodeRegionDirective.cs
- Adorner.cs
- NativeMethods.cs
- Dump.cs
- BlurBitmapEffect.cs
- SelectionChangedEventArgs.cs
- ResourceDisplayNameAttribute.cs
- ButtonBase.cs
- TransactionBridgeSection.cs
- PrintPreviewControl.cs
- HtmlInputRadioButton.cs
- SchemaContext.cs
- HttpHostedTransportConfiguration.cs
- keycontainerpermission.cs
- DefaultBinder.cs
- ComponentRenameEvent.cs
- QueuePathEditor.cs
- ModelPropertyImpl.cs
- CodeAttributeDeclarationCollection.cs
- Sentence.cs
- ProgressBar.cs
- ZoneIdentityPermission.cs
- OdbcException.cs
- ColumnReorderedEventArgs.cs
- Scanner.cs
- _ListenerResponseStream.cs
- ResourceProviderFactory.cs
- EdgeProfileValidation.cs
- XmlSerializer.cs
- CallbackException.cs
- BitmapPalette.cs
- PopupRoot.cs
- DatatypeImplementation.cs
- PropertyEmitterBase.cs
- PaginationProgressEventArgs.cs
- DbUpdateCommandTree.cs
- ValidationSummary.cs
- BooleanConverter.cs
- ADMembershipProvider.cs
- NavigateEvent.cs
- counter.cs
- ResourceAttributes.cs
- StoryFragments.cs
- DynamicObjectAccessor.cs
- SafePEFileHandle.cs
- PreProcessor.cs
- ReadContentAsBinaryHelper.cs
- WsdlBuildProvider.cs
- ClientProxyGenerator.cs
- AppSettingsExpressionBuilder.cs
- FragmentQueryProcessor.cs
- MetadataPropertyCollection.cs
- Bidi.cs
- AsymmetricSecurityBindingElement.cs
- AnnotationMap.cs
- RC2CryptoServiceProvider.cs
- ShapeTypeface.cs
- StylusPointPropertyInfoDefaults.cs
- PaginationProgressEventArgs.cs
- SecurityElement.cs
- IsolatedStorageException.cs
- DbExpressionVisitor_TResultType.cs
- Model3DGroup.cs
- serverconfig.cs
- IPCCacheManager.cs
- CodeMethodReturnStatement.cs
- SapiRecoContext.cs
- ClientUtils.cs
- BindingNavigator.cs
- storepermission.cs
- PersonalizableAttribute.cs
- CoreSwitches.cs
- DefaultProxySection.cs
- Vars.cs
- KeyProperty.cs
- CaretElement.cs
- MyContact.cs
- ConfigXmlDocument.cs
- PointHitTestResult.cs
- EventLogEntryCollection.cs
- ImpersonationContext.cs
- ContainerVisual.cs
- SpotLight.cs
- WebPartCatalogAddVerb.cs
- Menu.cs
- ObjectManager.cs
- MetabaseServerConfig.cs
- CommittableTransaction.cs
- Msec.cs