Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / Orcas / NetFXw7 / wpf / src / Core / CSharp / System / Windows / Media / Generated / Int32CollectionConverter.cs / 1 / Int32CollectionConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { ////// Int32CollectionConverter - Converter class for converting instances of other types to and from Int32Collection instances /// public sealed class Int32CollectionConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Int32Collection from the given object. /// ////// The Int32Collection which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Int32Collection. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Int32Collection. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Int32Collection.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Int32Collection to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Int32Collection, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Int32Collection instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Int32Collection) { Int32Collection instance = (Int32Collection)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // This file was generated, please do not edit it directly. // // Please see http://wiki/default.aspx/Microsoft.Projects.Avalon/MilCodeGen.html for more information. // //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.KnownBoxes; using MS.Internal.Collections; using MS.Internal.PresentationCore; using MS.Utility; using System; using System.Collections; using System.Collections.Generic; using System.ComponentModel; using System.Diagnostics; using System.Globalization; using System.Reflection; using System.Runtime.InteropServices; using System.ComponentModel.Design.Serialization; using System.Text; using System.Windows; using System.Windows.Media; using System.Windows.Media.Effects; using System.Windows.Media.Media3D; using System.Windows.Media.Animation; using System.Windows.Media.Composition; using System.Windows.Media.Imaging; using System.Windows.Markup; using System.Windows.Media.Converters; using System.Security; using System.Security.Permissions; using SR=MS.Internal.PresentationCore.SR; using SRID=MS.Internal.PresentationCore.SRID; #pragma warning disable 1634, 1691 // suppressing PreSharp warnings namespace System.Windows.Media { ////// Int32CollectionConverter - Converter class for converting instances of other types to and from Int32Collection instances /// public sealed class Int32CollectionConverter : TypeConverter { ////// Returns true if this type converter can convert from a given type. /// ////// bool - True if this converter can convert from the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertFrom(ITypeDescriptorContext context, Type sourceType) { if (sourceType == typeof(string)) { return true; } return base.CanConvertFrom(context, sourceType); } ////// Returns true if this type converter can convert to the given type. /// ////// bool - True if this converter can convert to the provided type, false if not. /// /// The ITypeDescriptorContext for this call. /// The Type being queried for support. public override bool CanConvertTo(ITypeDescriptorContext context, Type destinationType) { if (destinationType == typeof(string)) { return true; } return base.CanConvertTo(context, destinationType); } ////// Attempts to convert to a Int32Collection from the given object. /// ////// The Int32Collection which was constructed. /// ////// A NotSupportedException is thrown if the example object is null or is not a valid type /// which can be converted to a Int32Collection. /// /// The ITypeDescriptorContext for this call. /// The requested CultureInfo. Note that conversion uses "en-US" rather than this parameter. /// The object to convert to an instance of Int32Collection. public override object ConvertFrom(ITypeDescriptorContext context, CultureInfo culture, object value) { if (value == null) { throw GetConvertFromException(value); } String source = value as string; if (source != null) { return Int32Collection.Parse(source); } return base.ConvertFrom(context, culture, value); } ////// ConvertTo - Attempt to convert an instance of Int32Collection to the given type /// ////// The object which was constructoed. /// ////// A NotSupportedException is thrown if "value" is null or not an instance of Int32Collection, /// or if the destinationType isn't one of the valid destination types. /// /// The ITypeDescriptorContext for this call. /// The CultureInfo which is respected when converting. /// The object to convert to an instance of "destinationType". /// The type to which this will convert the Int32Collection instance. public override object ConvertTo(ITypeDescriptorContext context, CultureInfo culture, object value, Type destinationType) { if (destinationType != null && value is Int32Collection) { Int32Collection instance = (Int32Collection)value; if (destinationType == typeof(string)) { // Delegate to the formatting/culture-aware ConvertToString method. #pragma warning suppress 6506 // instance is obviously not null return instance.ConvertToString(null, culture); } } // Pass unhandled cases to base class (which will throw exceptions for null value or destinationType.) return base.ConvertTo(context, culture, value, destinationType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
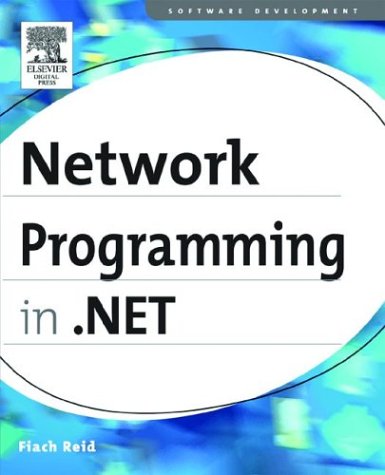
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RowToFieldTransformer.cs
- SqlConnection.cs
- HeaderedItemsControl.cs
- DispatchWrapper.cs
- WindowsListViewGroup.cs
- AuthenticationService.cs
- httpapplicationstate.cs
- XhtmlBasicPanelAdapter.cs
- PenLineCapValidation.cs
- ControlAdapter.cs
- EventDriven.cs
- BulletedListDesigner.cs
- AuditLog.cs
- ColumnMap.cs
- AssociationTypeEmitter.cs
- _SecureChannel.cs
- XmlWriter.cs
- MdImport.cs
- IISMapPath.cs
- OdbcParameter.cs
- SmiSettersStream.cs
- ContentHostHelper.cs
- MessagePropertyFilter.cs
- SecurityCredentialsManager.cs
- PropertyContainer.cs
- TemplateKey.cs
- DataObjectCopyingEventArgs.cs
- ContextStack.cs
- CircleEase.cs
- EventDescriptorCollection.cs
- WorkflowItemsPresenter.cs
- Control.cs
- ManualResetEvent.cs
- KnownBoxes.cs
- GridItemCollection.cs
- UpDownEvent.cs
- WindowsToolbarItemAsMenuItem.cs
- SecureStringHasher.cs
- BamlLocalizerErrorNotifyEventArgs.cs
- ScrollBar.cs
- ImageEditor.cs
- WorkflowClientDeliverMessageWrapper.cs
- DataObject.cs
- DataPagerFieldCommandEventArgs.cs
- HtmlInputCheckBox.cs
- TimerElapsedEvenArgs.cs
- PathSegment.cs
- EastAsianLunisolarCalendar.cs
- MenuItemStyle.cs
- CommandField.cs
- WebPartManagerInternals.cs
- CreateUserWizard.cs
- DesignerDataParameter.cs
- Expression.cs
- Int16AnimationUsingKeyFrames.cs
- ListViewInsertEventArgs.cs
- ProtocolViolationException.cs
- LinqDataSourceDeleteEventArgs.cs
- EntitySqlException.cs
- XsltContext.cs
- StateManagedCollection.cs
- controlskin.cs
- InstalledVoice.cs
- ScrollViewer.cs
- PositiveTimeSpanValidatorAttribute.cs
- InvalidPipelineStoreException.cs
- DockProviderWrapper.cs
- XamlFilter.cs
- DurableErrorHandler.cs
- ButtonDesigner.cs
- RegexParser.cs
- columnmapkeybuilder.cs
- XmlFormatWriterGenerator.cs
- NullableBoolConverter.cs
- BitmapPalettes.cs
- WindowsEditBox.cs
- PrintController.cs
- DrawingState.cs
- TdsParserStateObject.cs
- ConnectivityStatus.cs
- AmbientLight.cs
- SpnEndpointIdentityExtension.cs
- WindowsFormsHelpers.cs
- PathFigureCollection.cs
- DesignerRegion.cs
- DefaultBindingPropertyAttribute.cs
- Buffer.cs
- RegularExpressionValidator.cs
- ComponentSerializationService.cs
- ToolBarOverflowPanel.cs
- PipelineModuleStepContainer.cs
- RegistryPermission.cs
- InfoCardRSAPKCS1SignatureDeformatter.cs
- InternalSafeNativeMethods.cs
- GenericAuthenticationEventArgs.cs
- TextParagraphView.cs
- FilteredAttributeCollection.cs
- SerializerWriterEventHandlers.cs
- ProxyAttribute.cs
- Viewport3DAutomationPeer.cs