Code:
/ Dotnetfx_Win7_3.5.1 / Dotnetfx_Win7_3.5.1 / 3.5.1 / DEVDIV / depot / DevDiv / releases / whidbey / NetFXspW7 / ndp / fx / src / xsp / System / Web / Configuration / HttpHandlersSection.cs / 1 / HttpHandlersSection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.Compilation; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpHandlersSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propHandlers = new ConfigurationProperty(null, typeof(HttpHandlerActionCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private bool _validated; static HttpHandlersSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propHandlers); } public HttpHandlersSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("", IsDefaultCollection = true)] public HttpHandlerActionCollection Handlers { get { return (HttpHandlerActionCollection)base[_propHandlers]; } } internal bool ValidateHandlers() { if (!_validated) { lock (this) { if (!_validated) { foreach (HttpHandlerAction ha in Handlers) { ha.InitValidateInternal(); } _validated = true; } } } return _validated; } internal HttpHandlerAction FindMapping(String verb, VirtualPath path) { ValidateHandlers(); for (int i = 0; i < Handlers.Count; i++) { HttpHandlerAction m = (HttpHandlerAction)Handlers[i]; if (m.IsMatch(verb, path)) { return m; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Configuration { using System; using System.Xml; using System.Configuration; using System.Collections.Specialized; using System.Collections; using System.IO; using System.Text; using System.Web.Util; using System.Web.Compilation; using System.Globalization; using System.Security.Permissions; [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class HttpHandlersSection : ConfigurationSection { private static ConfigurationPropertyCollection _properties; private static readonly ConfigurationProperty _propHandlers = new ConfigurationProperty(null, typeof(HttpHandlerActionCollection), null, ConfigurationPropertyOptions.IsDefaultCollection); private bool _validated; static HttpHandlersSection() { // Property initialization _properties = new ConfigurationPropertyCollection(); _properties.Add(_propHandlers); } public HttpHandlersSection() { } protected override ConfigurationPropertyCollection Properties { get { return _properties; } } [ConfigurationProperty("", IsDefaultCollection = true)] public HttpHandlerActionCollection Handlers { get { return (HttpHandlerActionCollection)base[_propHandlers]; } } internal bool ValidateHandlers() { if (!_validated) { lock (this) { if (!_validated) { foreach (HttpHandlerAction ha in Handlers) { ha.InitValidateInternal(); } _validated = true; } } } return _validated; } internal HttpHandlerAction FindMapping(String verb, VirtualPath path) { ValidateHandlers(); for (int i = 0; i < Handlers.Count; i++) { HttpHandlerAction m = (HttpHandlerAction)Handlers[i]; if (m.IsMatch(verb, path)) { return m; } } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
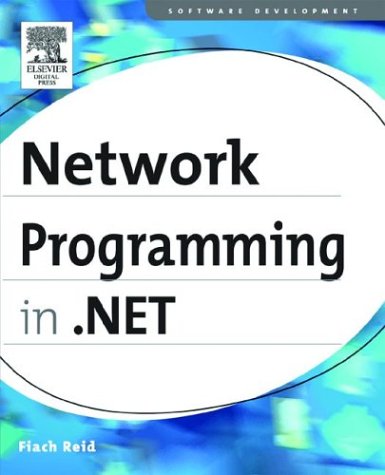
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BroadcastEventHelper.cs
- Stopwatch.cs
- ToolStripSeparatorRenderEventArgs.cs
- ListBox.cs
- X509CertificateEndpointIdentity.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- FormViewPagerRow.cs
- UIElementAutomationPeer.cs
- AsymmetricKeyExchangeDeformatter.cs
- LogicalCallContext.cs
- COAUTHIDENTITY.cs
- ItemDragEvent.cs
- ExtendedPropertiesHandler.cs
- TableLayoutColumnStyleCollection.cs
- PanelContainerDesigner.cs
- DataGridViewRowConverter.cs
- PrimitiveXmlSerializers.cs
- OleCmdHelper.cs
- AbsoluteQuery.cs
- TrackingCondition.cs
- AsyncCompletedEventArgs.cs
- SystemThemeKey.cs
- TimeZoneNotFoundException.cs
- DrawingVisual.cs
- TriggerActionCollection.cs
- PopOutPanel.cs
- ControlOperationInvoker.cs
- CodeStatement.cs
- SecurityContext.cs
- InteropEnvironment.cs
- TypeBuilderInstantiation.cs
- WebZone.cs
- ResourceIDHelper.cs
- LoadWorkflowByInstanceKeyCommand.cs
- Menu.cs
- RelatedPropertyManager.cs
- PropertyValueChangedEvent.cs
- AnnouncementInnerClient11.cs
- RecognizedPhrase.cs
- HttpWriter.cs
- BitmapCacheBrush.cs
- ObjectDisposedException.cs
- TypeLoader.cs
- Page.cs
- XmlCustomFormatter.cs
- HostProtectionException.cs
- ActiveDocumentEvent.cs
- UpdatePanelTrigger.cs
- HtmlContainerControl.cs
- SuppressMergeCheckAttribute.cs
- PeerObject.cs
- WorkflowTimerService.cs
- XmlCharacterData.cs
- XmlNamedNodeMap.cs
- PersonalizableAttribute.cs
- ProfileModule.cs
- FocusWithinProperty.cs
- coordinator.cs
- AutoResizedEvent.cs
- CryptoStream.cs
- IApplicationTrustManager.cs
- PeerName.cs
- XmlEncodedRawTextWriter.cs
- OleDbEnumerator.cs
- DeadCharTextComposition.cs
- ConfigUtil.cs
- EncodingInfo.cs
- LessThanOrEqual.cs
- DayRenderEvent.cs
- UIElementParaClient.cs
- ManifestResourceInfo.cs
- PasswordTextNavigator.cs
- XmlResolver.cs
- Rules.cs
- ImageCodecInfoPrivate.cs
- HasCopySemanticsAttribute.cs
- VectorCollectionConverter.cs
- DynamicDataResources.Designer.cs
- ItemCheckedEvent.cs
- TabControlCancelEvent.cs
- BinaryObjectReader.cs
- RuntimeHandles.cs
- SymbolPair.cs
- ForwardPositionQuery.cs
- WindowsSysHeader.cs
- DeobfuscatingStream.cs
- XmlSchemaAnnotation.cs
- SchemaReference.cs
- DataRowCollection.cs
- TreeIterators.cs
- AddingNewEventArgs.cs
- PackageRelationshipSelector.cs
- Control.cs
- XmlAttributeAttribute.cs
- Publisher.cs
- WorkflowRuntime.cs
- DbBuffer.cs
- QueryOutputWriter.cs
- ObjectComplexPropertyMapping.cs
- DebugView.cs