Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / Xml / System / Xml / XPath / XPathNodeIterator.cs / 3 / XPathNodeIterator.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- namespace System.Xml.XPath { using System; using System.Collections; using System.Diagnostics; using System.Text; [DebuggerDisplay("Position={CurrentPosition}, Current={debuggerDisplayProxy}")] public abstract class XPathNodeIterator : ICloneable, IEnumerable { internal int count = -1; object ICloneable.Clone() { return this.Clone(); } public abstract XPathNodeIterator Clone(); public abstract bool MoveNext(); public abstract XPathNavigator Current { get; } public abstract int CurrentPosition { get; } public virtual int Count { get { if (count == -1) { XPathNodeIterator clone = this.Clone(); while(clone.MoveNext()) ; count = clone.CurrentPosition; } return count; } } public virtual IEnumerator GetEnumerator() { return new Enumerator(this); } private object debuggerDisplayProxy { get { return Current == null ? null : (object)new XPathNavigator.DebuggerDisplayProxy(Current); } } ////// Implementation of a resetable enumerator that is linked to the XPathNodeIterator used to create it. /// private class Enumerator : IEnumerator { private XPathNodeIterator original; // Keep original XPathNodeIterator in case Reset() is called private XPathNodeIterator current; private bool iterationStarted; public Enumerator(XPathNodeIterator original) { this.original = original.Clone(); } public virtual object Current { get { // 1. Do not reuse the XPathNavigator, as we do in XPathNodeIterator // 2. Throw exception if current position is before first node or after the last node if (this.iterationStarted) { // Current is null if iterator is positioned after the last node if (this.current == null) throw new InvalidOperationException(Res.GetString(Res.Sch_EnumFinished, string.Empty)); return this.current.Current.Clone(); } // User must call MoveNext before accessing Current property throw new InvalidOperationException(Res.GetString(Res.Sch_EnumNotStarted, string.Empty)); } } public virtual bool MoveNext() { // Delegate to XPathNodeIterator if (!this.iterationStarted) { // Reset iteration to original position this.current = this.original.Clone(); this.iterationStarted = true; } if (this.current == null || !this.current.MoveNext()) { // Iteration complete this.current = null; return false; } return true; } public virtual void Reset() { this.iterationStarted = false; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
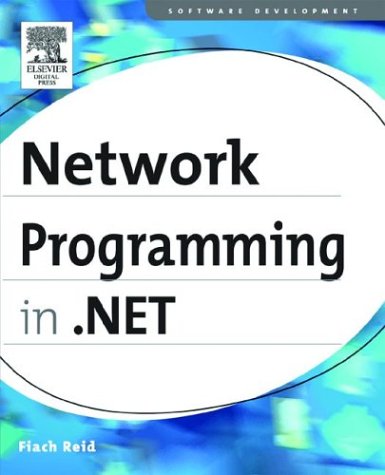
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProviderCommandInfoUtils.cs
- ClientUtils.cs
- InstancePersistenceException.cs
- TextPointerBase.cs
- BaseUriHelper.cs
- SemaphoreFullException.cs
- DataObjectCopyingEventArgs.cs
- CryptoKeySecurity.cs
- x509utils.cs
- BitmapEffectDrawingContent.cs
- PrimitiveXmlSerializers.cs
- RuleSettings.cs
- SingleAnimationBase.cs
- UrlParameterReader.cs
- WorkflowApplicationIdleEventArgs.cs
- TypeElementCollection.cs
- MultiByteCodec.cs
- SignedXmlDebugLog.cs
- StateMachineWorkflowDesigner.cs
- BinaryCommonClasses.cs
- RootBrowserWindowAutomationPeer.cs
- TextRange.cs
- DiagnosticsConfigurationHandler.cs
- ToolStripButton.cs
- VisualBasicImportReference.cs
- AbstractExpressions.cs
- WebPartActionVerb.cs
- SoundPlayerAction.cs
- BadImageFormatException.cs
- OdbcCommand.cs
- HighlightVisual.cs
- EventRoute.cs
- NumberEdit.cs
- HttpsChannelFactory.cs
- WebPartConnectVerb.cs
- UnsafeNativeMethods.cs
- TransformerInfo.cs
- XPathCompileException.cs
- Rijndael.cs
- DataGridSortCommandEventArgs.cs
- ArgumentValue.cs
- XPathException.cs
- TabletDevice.cs
- ReferentialConstraint.cs
- WindowsScrollBarBits.cs
- SystemKeyConverter.cs
- FileLogRecord.cs
- SpnegoTokenProvider.cs
- XmlDomTextWriter.cs
- XmlSchemaAnyAttribute.cs
- DataGridRowAutomationPeer.cs
- Scheduling.cs
- UserControlParser.cs
- SQLInt16.cs
- ScalarType.cs
- ForeignKeyConstraint.cs
- DataServiceQueryException.cs
- XmlTextEncoder.cs
- MultiTouchSystemGestureLogic.cs
- XmlSchemaResource.cs
- InheritablePropertyChangeInfo.cs
- DispatcherSynchronizationContext.cs
- SrgsDocument.cs
- BindingListCollectionView.cs
- WebPartEditorOkVerb.cs
- ConfigurationConverterBase.cs
- HttpRuntime.cs
- DataPagerField.cs
- SemanticBasicElement.cs
- TableLayoutStyleCollection.cs
- ResXResourceReader.cs
- WorkflowApplicationUnhandledExceptionEventArgs.cs
- DataGridViewAutoSizeModeEventArgs.cs
- odbcmetadatafactory.cs
- StoreContentChangedEventArgs.cs
- ReferenceSchema.cs
- HwndSubclass.cs
- SemanticResultValue.cs
- LinearKeyFrames.cs
- AppSettingsExpressionBuilder.cs
- MethodMessage.cs
- DatagridviewDisplayedBandsData.cs
- CacheHelper.cs
- Stopwatch.cs
- ThousandthOfEmRealPoints.cs
- HttpListenerContext.cs
- AttributeTable.cs
- CapabilitiesAssignment.cs
- AutomationElementCollection.cs
- ReverseInheritProperty.cs
- SortDescriptionCollection.cs
- ServiceAppDomainAssociationProvider.cs
- AlphaSortedEnumConverter.cs
- FrameAutomationPeer.cs
- DataPagerCommandEventArgs.cs
- SafeMarshalContext.cs
- ProjectionCamera.cs
- WindowsFormsHostPropertyMap.cs
- WebPartZone.cs
- DataGridViewUtilities.cs