Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Common / EntitySql / ParserOptions.cs / 3 / ParserOptions.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Globalization; // // disables XML doc overloads warning as error // #pragma warning disable 0419 ////// Represents eSql Text Compilation options. /// ParserOptions can be optionaly passed to CqlQuery.Compile or CqlQuery.Parse methods /// internal sealed class ParserOptions { //////
///Thrown if the properties are changed after being consumed by CqlQuery.Parse or CqlQuery.Compile ////// Once ParserOptions is passed and consumed by Compile or Parse Methods, it cannot be changed. /// If a setter is called after a ParserOptions instance is consumed by Parse or Compile methods, a EntityException will be raised. /// ///- ///
Compile Method - ///
Parse Method /// eSql enum Case Sensitivity values /// internal enum CaseSensitiveness { ////// Case Sensitive /// CaseSensitive, ////// Case Insensitive /// CaseInsensitive } private bool _bReadOnly = false; ////// AllowQuotedIdentifiers:={true|false}, default value: {false} /// Causes eSql to follow the SQL-92 rules regarding quotation mark /// delimiting identifiers and literal strings. Identifiers delimited /// by double quotation marks can be either eSql reserved keywords /// or can contain characters not usually allowed by the eSql syntax /// rules for identifiers. /// ////// The default setting for this option is to interpret double quotes as literal strings. /// ///true or false ///Thrown if the option is changed after being consumed by Compile or Parse methods internal bool AllowQuotedIdentifiers { get { return _allowQuotedIdentifiers; } #if WHEN_NEEDED set { CheckIfReadOnly(); _allowQuotedIdentifiers = value; } #endif } private bool _allowQuotedIdentifiers = false; ////// IdentifierCaseSensitiveness:={CaseSensitiveness.CaseSensitive|CaseSensitiveness.CaseInsensitive}, Default:={CaseSensitiveness.CaseSensitive} /// Define the eSql compiler behavior regarding case sensitiveness of variables and aliases. /// ////// The default setting for this option is Case Sensitive. /// Types names are not affected by this option and depend on metadata service case-sensitivity rules. /// ///CaseSensitiveness.CaseSensitive or CaseSensitiveness.CaseInsensitive ///Thrown if the option is changed after being consumed by Compile or Parse methods internal CaseSensitiveness IdentifierCaseSensitiveness { get { return _identifierCaseSensitiveness; } #if WHEN_NEEDED set { CheckIfReadOnly(); _identifierCaseSensitiveness = value; } #endif } private CaseSensitiveness _identifierCaseSensitiveness = CaseSensitiveness.CaseInsensitive; ////// Defines OrderBy Collation specification. /// ////// The default is no collation specification. /// the COLLATION sub-clause in ORDER BY clause always overrides this property. In other words, this property takes effect only if /// there is no COLLATION sub-clause defined in a eSql ORDER BY Statement. /// ///Sort Collation name ///Thrown if the option is changed after being consumed by Compile or Parse methods internal string DefaultOrderByCollation { get { return _defaultOrderByCollation ?? String.Empty; } #if WHEN_NEEDED set { CheckIfReadOnly(); _defaultOrderByCollation = (null != value) ? value.Trim() : value; } #endif } private string _defaultOrderByCollation = null; ////// Makes options read-only /// ///internal ParserOptions MakeReadOnly() { #if WHEN_NEEDED _bReadOnly = true; #endif return this; } internal enum CompilationMode { /// /// Normal mode. Compiles all statements in the query. /// NormalMode, ////// View generation mode, optimizes compilation process to ignore uncessary eSql constructs. /// IMPORTANT NOTE: In this setting, the eSql query will be compiled in a restricted mode in /// which GROUP BY, HAVING and ORDER BY clauses will be short-circuited and ignored. If your /// view or query has *and* requires these constructs to be compiled, *do not use this option*. /// This optimization applies to all levels and sub-queries not only the topmost query. /// RestrictedViewGenerationMode, ////// Enables full eSql compilation without optimizations and enables type constructor with WITH RELATIONSHIP clause /// UserViewGenerationMode } ////// Sets/Gets eSQL parser compilation mode. /// internal CompilationMode ParserCompilationMode { get { return _compilationMode; } set { CheckIfReadOnly(); _compilationMode = value; } } private CompilationMode _compilationMode = CompilationMode.NormalMode; ////// Verify if setters are allowed. /// private void CheckIfReadOnly() { if (_bReadOnly) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.PropertyCannotBeChangedAtThisTime); } } } #pragma warning restore 0419 } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Common.EntitySql { using System; using System.Globalization; // // disables XML doc overloads warning as error // #pragma warning disable 0419 ////// Represents eSql Text Compilation options. /// ParserOptions can be optionaly passed to CqlQuery.Compile or CqlQuery.Parse methods /// internal sealed class ParserOptions { //////
///Thrown if the properties are changed after being consumed by CqlQuery.Parse or CqlQuery.Compile ////// Once ParserOptions is passed and consumed by Compile or Parse Methods, it cannot be changed. /// If a setter is called after a ParserOptions instance is consumed by Parse or Compile methods, a EntityException will be raised. /// ///- ///
Compile Method - ///
Parse Method /// eSql enum Case Sensitivity values /// internal enum CaseSensitiveness { ////// Case Sensitive /// CaseSensitive, ////// Case Insensitive /// CaseInsensitive } private bool _bReadOnly = false; ////// AllowQuotedIdentifiers:={true|false}, default value: {false} /// Causes eSql to follow the SQL-92 rules regarding quotation mark /// delimiting identifiers and literal strings. Identifiers delimited /// by double quotation marks can be either eSql reserved keywords /// or can contain characters not usually allowed by the eSql syntax /// rules for identifiers. /// ////// The default setting for this option is to interpret double quotes as literal strings. /// ///true or false ///Thrown if the option is changed after being consumed by Compile or Parse methods internal bool AllowQuotedIdentifiers { get { return _allowQuotedIdentifiers; } #if WHEN_NEEDED set { CheckIfReadOnly(); _allowQuotedIdentifiers = value; } #endif } private bool _allowQuotedIdentifiers = false; ////// IdentifierCaseSensitiveness:={CaseSensitiveness.CaseSensitive|CaseSensitiveness.CaseInsensitive}, Default:={CaseSensitiveness.CaseSensitive} /// Define the eSql compiler behavior regarding case sensitiveness of variables and aliases. /// ////// The default setting for this option is Case Sensitive. /// Types names are not affected by this option and depend on metadata service case-sensitivity rules. /// ///CaseSensitiveness.CaseSensitive or CaseSensitiveness.CaseInsensitive ///Thrown if the option is changed after being consumed by Compile or Parse methods internal CaseSensitiveness IdentifierCaseSensitiveness { get { return _identifierCaseSensitiveness; } #if WHEN_NEEDED set { CheckIfReadOnly(); _identifierCaseSensitiveness = value; } #endif } private CaseSensitiveness _identifierCaseSensitiveness = CaseSensitiveness.CaseInsensitive; ////// Defines OrderBy Collation specification. /// ////// The default is no collation specification. /// the COLLATION sub-clause in ORDER BY clause always overrides this property. In other words, this property takes effect only if /// there is no COLLATION sub-clause defined in a eSql ORDER BY Statement. /// ///Sort Collation name ///Thrown if the option is changed after being consumed by Compile or Parse methods internal string DefaultOrderByCollation { get { return _defaultOrderByCollation ?? String.Empty; } #if WHEN_NEEDED set { CheckIfReadOnly(); _defaultOrderByCollation = (null != value) ? value.Trim() : value; } #endif } private string _defaultOrderByCollation = null; ////// Makes options read-only /// ///internal ParserOptions MakeReadOnly() { #if WHEN_NEEDED _bReadOnly = true; #endif return this; } internal enum CompilationMode { /// /// Normal mode. Compiles all statements in the query. /// NormalMode, ////// View generation mode, optimizes compilation process to ignore uncessary eSql constructs. /// IMPORTANT NOTE: In this setting, the eSql query will be compiled in a restricted mode in /// which GROUP BY, HAVING and ORDER BY clauses will be short-circuited and ignored. If your /// view or query has *and* requires these constructs to be compiled, *do not use this option*. /// This optimization applies to all levels and sub-queries not only the topmost query. /// RestrictedViewGenerationMode, ////// Enables full eSql compilation without optimizations and enables type constructor with WITH RELATIONSHIP clause /// UserViewGenerationMode } ////// Sets/Gets eSQL parser compilation mode. /// internal CompilationMode ParserCompilationMode { get { return _compilationMode; } set { CheckIfReadOnly(); _compilationMode = value; } } private CompilationMode _compilationMode = CompilationMode.NormalMode; ////// Verify if setters are allowed. /// private void CheckIfReadOnly() { if (_bReadOnly) { throw EntityUtil.EntitySqlError(System.Data.Entity.Strings.PropertyCannotBeChangedAtThisTime); } } } #pragma warning restore 0419 } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
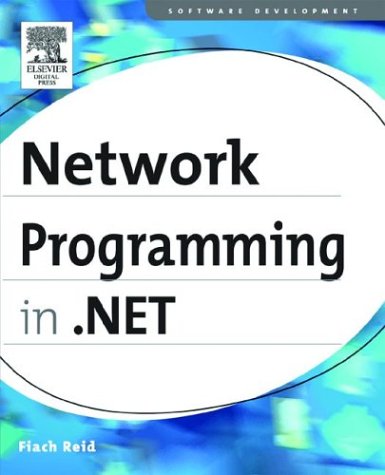
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SettingsPropertyIsReadOnlyException.cs
- ResourcesGenerator.cs
- IndexedString.cs
- DataGridViewCellValueEventArgs.cs
- FontInfo.cs
- CheckBoxBaseAdapter.cs
- ToolStripPanelSelectionBehavior.cs
- HelpKeywordAttribute.cs
- sapiproxy.cs
- bindurihelper.cs
- DataGridViewElement.cs
- Compiler.cs
- SchemaImporterExtensionElement.cs
- Mappings.cs
- SafeEventLogWriteHandle.cs
- TraceLevelHelper.cs
- ContentFilePart.cs
- Random.cs
- WebPartUserCapability.cs
- MsmqReceiveHelper.cs
- XmlSerializationWriter.cs
- FlowDocumentReader.cs
- OlePropertyStructs.cs
- IMembershipProvider.cs
- ScriptModule.cs
- TemplateContentLoader.cs
- TextSpanModifier.cs
- DesignerGenericWebPart.cs
- RemotingAttributes.cs
- ViewGenResults.cs
- Queue.cs
- UnauthorizedAccessException.cs
- HttpModulesSection.cs
- AmbientEnvironment.cs
- BitmapSourceSafeMILHandle.cs
- CodeAccessPermission.cs
- BitmapEffectrendercontext.cs
- MouseCaptureWithinProperty.cs
- BezierSegment.cs
- LayoutEditorPart.cs
- OleDbCommandBuilder.cs
- MarshalDirectiveException.cs
- ObjectCache.cs
- PartitionResolver.cs
- TemplateAction.cs
- SharedStatics.cs
- SoapProtocolReflector.cs
- StrokeCollectionConverter.cs
- TdsValueSetter.cs
- ClientApiGenerator.cs
- EntityObject.cs
- SiteMap.cs
- CollectionViewProxy.cs
- CurrencyWrapper.cs
- ListBoxChrome.cs
- Queue.cs
- BinaryKeyIdentifierClause.cs
- UriTemplateHelpers.cs
- AuthorizationRuleCollection.cs
- SocketElement.cs
- BitmapCodecInfoInternal.cs
- ViewKeyConstraint.cs
- StructuredTypeEmitter.cs
- StylusPlugInCollection.cs
- WebPartUserCapability.cs
- WorkflowOperationFault.cs
- SineEase.cs
- LambdaSerializationException.cs
- UInt64.cs
- EntityDesignPluralizationHandler.cs
- Native.cs
- SQLBoolean.cs
- SHA384Managed.cs
- Win32Exception.cs
- InvalidTimeZoneException.cs
- Label.cs
- EdmSchemaError.cs
- Int64KeyFrameCollection.cs
- TabControlEvent.cs
- ListSortDescription.cs
- AssociativeAggregationOperator.cs
- ChangeTracker.cs
- PeerName.cs
- TemplateField.cs
- TimeZone.cs
- SerializationInfoEnumerator.cs
- OdbcDataReader.cs
- WmfPlaceableFileHeader.cs
- BaseCodePageEncoding.cs
- HtmlTernaryTree.cs
- Opcode.cs
- Point3DAnimation.cs
- TdsParserStaticMethods.cs
- CollectionViewGroupRoot.cs
- Thumb.cs
- HttpContextWrapper.cs
- ImplicitInputBrush.cs
- AssemblyHash.cs
- TextBoxLine.cs
- CultureTableRecord.cs