Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / ndp / fx / src / DataEntity / System / Data / Objects / objectresult_tresulttype.cs / 2 / objectresult_tresulttype.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- namespace System.Data.Objects { using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Mapping; using System.Data.Objects.DataClasses; using System.Diagnostics; using System.Linq.Expressions; using System.Data.Common.Internal.Materialization; ////// This class implements IEnumerable of T and IDisposable. Instance of this class /// is returned from ObjectQuery<T>.Execute method. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public sealed class ObjectResult: ObjectResult, IEnumerable { private Shaper _shaper; private DbDataReader _reader; private readonly EntitySet _singleEntitySet; private readonly TypeUsage _resultItemType; private IBindingList _cachedBindingList; internal ObjectResult(Shaper shaper, EntitySet singleEntitySet, TypeUsage resultItemType) { _shaper = shaper; _reader = _shaper.Reader; _singleEntitySet = singleEntitySet; _resultItemType = resultItemType; } private void EnsureCanEnumerateResults() { if (null == _shaper) { // Enumerating more than once is not allowed. throw EntityUtil.CannotReEnumerateQueryResults(); } } /// /// Returns an enumerator that iterates through the collection. /// public IEnumeratorGetEnumerator() { EnsureCanEnumerateResults(); Shaper shaper = _shaper; _shaper = null; IEnumerator result = shaper.GetEnumerator(); return result; } /// /// Performs tasks associated with freeing, releasing, or resetting resources. /// public override void Dispose() { DbDataReader reader = _reader; _reader = null; if (null != reader) { reader.Dispose(); } if (_shaper != null) { // This case includes when the ObjectResult is disposed before it // created an ObjectQueryEnumeration; at this time, the connection can be released if (_shaper.Context != null) { _shaper.Context.ReleaseConnection(); } _shaper = null; } } internal override IEnumerator GetEnumeratorInternal() { return ((IEnumerable)this).GetEnumerator(); } internal override IList GetIListSourceListInternal() { // You can only enumerate the query results once, and the creation of an ObjectView consumes this enumeration. // However, there are situations where setting the DataSource of a control can result in multiple calls to this method. // In order to enable this scenario and allow direct binding to the ObjectResult instance, // the ObjectView is cached and returned on subsequent calls to this method. if (_cachedBindingList == null) { EnsureCanEnumerateResults(); bool forceReadOnly = this._shaper.MergeOption == MergeOption.NoTracking; _cachedBindingList = ObjectViewFactory.CreateViewForQuery (this._resultItemType, this, this._shaper.Context, forceReadOnly, this._singleEntitySet); } return _cachedBindingList; } public override Type ElementType { get { return typeof(T); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupowner [....] //--------------------------------------------------------------------- namespace System.Data.Objects { using System; using System.Collections; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Data; using System.Data.Common; using System.Data.Metadata.Edm; using System.Data.Mapping; using System.Data.Objects.DataClasses; using System.Diagnostics; using System.Linq.Expressions; using System.Data.Common.Internal.Materialization; ////// This class implements IEnumerable of T and IDisposable. Instance of this class /// is returned from ObjectQuery<T>.Execute method. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public sealed class ObjectResult: ObjectResult, IEnumerable { private Shaper _shaper; private DbDataReader _reader; private readonly EntitySet _singleEntitySet; private readonly TypeUsage _resultItemType; private IBindingList _cachedBindingList; internal ObjectResult(Shaper shaper, EntitySet singleEntitySet, TypeUsage resultItemType) { _shaper = shaper; _reader = _shaper.Reader; _singleEntitySet = singleEntitySet; _resultItemType = resultItemType; } private void EnsureCanEnumerateResults() { if (null == _shaper) { // Enumerating more than once is not allowed. throw EntityUtil.CannotReEnumerateQueryResults(); } } /// /// Returns an enumerator that iterates through the collection. /// public IEnumeratorGetEnumerator() { EnsureCanEnumerateResults(); Shaper shaper = _shaper; _shaper = null; IEnumerator result = shaper.GetEnumerator(); return result; } /// /// Performs tasks associated with freeing, releasing, or resetting resources. /// public override void Dispose() { DbDataReader reader = _reader; _reader = null; if (null != reader) { reader.Dispose(); } if (_shaper != null) { // This case includes when the ObjectResult is disposed before it // created an ObjectQueryEnumeration; at this time, the connection can be released if (_shaper.Context != null) { _shaper.Context.ReleaseConnection(); } _shaper = null; } } internal override IEnumerator GetEnumeratorInternal() { return ((IEnumerable)this).GetEnumerator(); } internal override IList GetIListSourceListInternal() { // You can only enumerate the query results once, and the creation of an ObjectView consumes this enumeration. // However, there are situations where setting the DataSource of a control can result in multiple calls to this method. // In order to enable this scenario and allow direct binding to the ObjectResult instance, // the ObjectView is cached and returned on subsequent calls to this method. if (_cachedBindingList == null) { EnsureCanEnumerateResults(); bool forceReadOnly = this._shaper.MergeOption == MergeOption.NoTracking; _cachedBindingList = ObjectViewFactory.CreateViewForQuery (this._resultItemType, this, this._shaper.Context, forceReadOnly, this._singleEntitySet); } return _cachedBindingList; } public override Type ElementType { get { return typeof(T); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
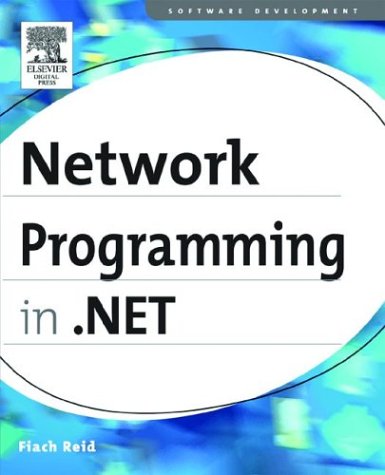
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SpellCheck.cs
- FocusChangedEventArgs.cs
- DataControlButton.cs
- MessageDesigner.cs
- ScrollItemProviderWrapper.cs
- AssemblyInfo.cs
- DependencyPropertyChangedEventArgs.cs
- VideoDrawing.cs
- RootProfilePropertySettingsCollection.cs
- SystemIcons.cs
- OneToOneMappingSerializer.cs
- MsmqProcessProtocolHandler.cs
- Camera.cs
- BitmapEffectGroup.cs
- _SslSessionsCache.cs
- ListViewItem.cs
- BaseResourcesBuildProvider.cs
- Label.cs
- DocumentReference.cs
- ParallelLoopState.cs
- CatalogPartCollection.cs
- IdentityVerifier.cs
- TableCellAutomationPeer.cs
- DetailsViewDeletedEventArgs.cs
- RegistrationServices.cs
- MsdtcWrapper.cs
- IdentityModelDictionary.cs
- AnnotationDocumentPaginator.cs
- FixedBufferAttribute.cs
- dtdvalidator.cs
- DataGridViewCellConverter.cs
- TextMessageEncoder.cs
- MailHeaderInfo.cs
- Timer.cs
- OleDbReferenceCollection.cs
- DomainUpDown.cs
- HWStack.cs
- XPathDocumentBuilder.cs
- DateTimeOffset.cs
- ExceptionUtility.cs
- IndexedString.cs
- ELinqQueryState.cs
- UnsafeNativeMethods.cs
- SplineQuaternionKeyFrame.cs
- ScopedKnownTypes.cs
- ComponentResourceManager.cs
- ClientRuntime.cs
- ClientScriptManager.cs
- XmlWriterDelegator.cs
- WindowsEditBoxRange.cs
- SubpageParaClient.cs
- RayHitTestParameters.cs
- SerializationStore.cs
- TargetPerspective.cs
- ContractReference.cs
- ClientSession.cs
- WebConfigManager.cs
- ReadWriteObjectLock.cs
- FamilyMap.cs
- XsdDateTime.cs
- XmlEncoding.cs
- ErrorProvider.cs
- ServiceDescriptionSerializer.cs
- DataTableTypeConverter.cs
- HybridObjectCache.cs
- BaseDataBoundControl.cs
- webeventbuffer.cs
- DataTableCollection.cs
- TdsParserSessionPool.cs
- EnlistmentTraceIdentifier.cs
- CheckBox.cs
- DescendantOverDescendantQuery.cs
- EntityClassGenerator.cs
- EntityContainerRelationshipSet.cs
- UnitySerializationHolder.cs
- InstanceLockException.cs
- OdbcConnectionStringbuilder.cs
- Error.cs
- TimeSpanConverter.cs
- SizeChangedInfo.cs
- Globals.cs
- MemberInitExpression.cs
- TranslateTransform.cs
- RtfToXamlReader.cs
- CodeEventReferenceExpression.cs
- DiffuseMaterial.cs
- remotingproxy.cs
- ColorTransformHelper.cs
- EntityDesignerBuildProvider.cs
- XmlSchemaSimpleType.cs
- ScrollEventArgs.cs
- SqlBulkCopyColumnMappingCollection.cs
- Splitter.cs
- OleDbException.cs
- StatusBarAutomationPeer.cs
- EndpointBehaviorElementCollection.cs
- GridViewRowEventArgs.cs
- Light.cs
- Style.cs
- TileBrush.cs