Code:
/ Dotnetfx_Vista_SP2 / Dotnetfx_Vista_SP2 / 8.0.50727.4016 / DEVDIV / depot / DevDiv / releases / whidbey / NetFxQFE / ndp / fx / src / Xml / System / Xml / HWStack.cs / 1 / HWStack.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; namespace System.Xml { // This stack is designed to minimize object creation for the // objects being stored in the stack by allowing them to be // re-used over time. It basically pushes the objects creating // a high water mark then as Pop() is called they are not removed // so that next time Push() is called it simply returns the last // object that was already on the stack. internal class HWStack : ICloneable { internal HWStack(int GrowthRate) : this (GrowthRate, int.MaxValue) {} internal HWStack(int GrowthRate, int limit) { this.growthRate = GrowthRate; this.used = 0; this.stack = new Object[GrowthRate]; this.size = GrowthRate; this.limit = limit; } internal Object Push() { if (this.used == this.size) { if (this.limit <= this.used) { throw new XmlException(Res.Xml_StackOverflow, string.Empty); } Object[] newstack = new Object[this.size + this.growthRate]; if (this.used > 0) { System.Array.Copy(this.stack, 0, newstack, 0, this.used); } this.stack = newstack; this.size += this.growthRate; } return this.stack[this.used++]; } internal Object Pop() { if (0 < this.used) { this.used--; Object result = this.stack[this.used]; return result; } return null; } internal object Peek() { return this.used > 0 ? this.stack[this.used - 1] : null; } internal void AddToTop(object o) { if (this.used > 0) { this.stack[this.used - 1] = o; } } internal Object this[int index] { get { if (index >= 0 && index < this.used) { Object result = this.stack[index]; return result; } else { throw new IndexOutOfRangeException(); } } set { if (index >= 0 && index < this.used) { this.stack[index] = value; } else { throw new IndexOutOfRangeException(); } } } internal int Length { get { return this.used;} } // // ICloneable // private HWStack(object[] stack, int growthRate, int used, int size) { this.stack = stack; this.growthRate = growthRate; this.used = used; this.size = size; } public object Clone() { return new HWStack((object[]) this.stack.Clone(), this.growthRate, this.used, this.size); } private Object[] stack; private int growthRate; private int used; private int size; private int limit; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System; namespace System.Xml { // This stack is designed to minimize object creation for the // objects being stored in the stack by allowing them to be // re-used over time. It basically pushes the objects creating // a high water mark then as Pop() is called they are not removed // so that next time Push() is called it simply returns the last // object that was already on the stack. internal class HWStack : ICloneable { internal HWStack(int GrowthRate) : this (GrowthRate, int.MaxValue) {} internal HWStack(int GrowthRate, int limit) { this.growthRate = GrowthRate; this.used = 0; this.stack = new Object[GrowthRate]; this.size = GrowthRate; this.limit = limit; } internal Object Push() { if (this.used == this.size) { if (this.limit <= this.used) { throw new XmlException(Res.Xml_StackOverflow, string.Empty); } Object[] newstack = new Object[this.size + this.growthRate]; if (this.used > 0) { System.Array.Copy(this.stack, 0, newstack, 0, this.used); } this.stack = newstack; this.size += this.growthRate; } return this.stack[this.used++]; } internal Object Pop() { if (0 < this.used) { this.used--; Object result = this.stack[this.used]; return result; } return null; } internal object Peek() { return this.used > 0 ? this.stack[this.used - 1] : null; } internal void AddToTop(object o) { if (this.used > 0) { this.stack[this.used - 1] = o; } } internal Object this[int index] { get { if (index >= 0 && index < this.used) { Object result = this.stack[index]; return result; } else { throw new IndexOutOfRangeException(); } } set { if (index >= 0 && index < this.used) { this.stack[index] = value; } else { throw new IndexOutOfRangeException(); } } } internal int Length { get { return this.used;} } // // ICloneable // private HWStack(object[] stack, int growthRate, int used, int size) { this.stack = stack; this.growthRate = growthRate; this.used = used; this.size = size; } public object Clone() { return new HWStack((object[]) this.stack.Clone(), this.growthRate, this.used, this.size); } private Object[] stack; private int growthRate; private int used; private int size; private int limit; }; } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
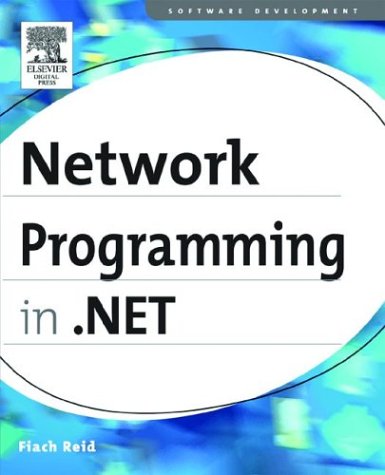
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextRunProperties.cs
- SpeechAudioFormatInfo.cs
- GroupItem.cs
- ToolStripItemRenderEventArgs.cs
- ListViewEditEventArgs.cs
- MetadataArtifactLoaderXmlReaderWrapper.cs
- PageContentAsyncResult.cs
- WsatServiceAddress.cs
- DbParameterCollectionHelper.cs
- SizeAnimationUsingKeyFrames.cs
- SubstitutionList.cs
- ConfigurationSectionGroupCollection.cs
- SQLSingle.cs
- Predicate.cs
- PropertyNames.cs
- DesignerWidgets.cs
- Events.cs
- DataGridViewCellStyleEditor.cs
- ImageUrlEditor.cs
- While.cs
- SectionInput.cs
- ECDiffieHellmanCngPublicKey.cs
- CharEntityEncoderFallback.cs
- LinqDataSourceDisposeEventArgs.cs
- PublishLicense.cs
- CodeArgumentReferenceExpression.cs
- XPathEmptyIterator.cs
- StorageEntityTypeMapping.cs
- HtmlTableRowCollection.cs
- CommandID.cs
- StorageTypeMapping.cs
- NativeMethods.cs
- TransformConverter.cs
- HandledMouseEvent.cs
- RelationshipManager.cs
- SqlXml.cs
- parserscommon.cs
- SqlExpressionNullability.cs
- cookiecontainer.cs
- KeyValuePair.cs
- InstanceKey.cs
- TransactionContextValidator.cs
- FreezableDefaultValueFactory.cs
- EntityReference.cs
- OdbcConnectionHandle.cs
- StateMachineWorkflowInstance.cs
- PositiveTimeSpanValidatorAttribute.cs
- ExtensionWindowHeader.cs
- ControlFilterExpression.cs
- ClientBuildManagerCallback.cs
- InstanceHandle.cs
- UserValidatedEventArgs.cs
- HandleCollector.cs
- LoginView.cs
- Flattener.cs
- EdmTypeAttribute.cs
- XmlSchemaFacet.cs
- COM2ColorConverter.cs
- ProgressPage.cs
- DbConnectionHelper.cs
- TableParagraph.cs
- SerialStream.cs
- Point3D.cs
- DataServiceQuery.cs
- XmlTextReaderImplHelpers.cs
- PropertyDescriptorCollection.cs
- SetStateEventArgs.cs
- CharAnimationUsingKeyFrames.cs
- CompiledQueryCacheEntry.cs
- CalendarKeyboardHelper.cs
- ConvertersCollection.cs
- StringExpressionSet.cs
- TextDocumentView.cs
- ArrayList.cs
- XmlMtomWriter.cs
- TargetInvocationException.cs
- DataConnectionHelper.cs
- TextRangeEditTables.cs
- QuotedPrintableStream.cs
- BitmapEffectInputData.cs
- ProtocolImporter.cs
- ThrowHelper.cs
- ApplicationHost.cs
- DataGridTable.cs
- DocumentGridContextMenu.cs
- DbConnectionPoolGroup.cs
- RegexCapture.cs
- TemplateAction.cs
- Object.cs
- SqlDataSourceConfigureSelectPanel.cs
- ObjectQueryProvider.cs
- SHA1CryptoServiceProvider.cs
- UICuesEvent.cs
- FontInfo.cs
- ScriptIgnoreAttribute.cs
- remotingproxy.cs
- CompositeActivityMarkupSerializer.cs
- BinaryCommonClasses.cs
- TaskbarItemInfo.cs
- ApplicationInfo.cs