Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / Orcas / SP / wpf / src / Framework / System / Windows / Documents / TableCell.cs / 1 / TableCell.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Implementation of table cell // // See spec at http://avalon/layout/Tables/WPP%20TableOM.doc // // History: // 05/19/2003 : olego - Created // // Todo list: // 1) OnPropertyInvalidated is expensive and requires investigation: // a) Cell calls to base class implementation which fires back tree // change event - Cell.OnTextContainerChange. As a result Cell.Invalidate // format is called twice. // b) base.OnPropertyInvalidated *always* creates DTR for the whole cell. // Why even AffectsRender causes it? //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PtsHost; using MS.Internal.PtsTable; using MS.Internal.Text; using MS.Utility; using System.Diagnostics; using System.Security; using System.Windows.Threading; using System.Collections; using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Controls; using System.Windows.Markup; using System.ComponentModel; // TypeConverter using System; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace System.Windows.Documents { ////// Implementation of table cell /// [ContentProperty("Blocks")] public class TableCell : TextElement { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor /// public TableCell() : base() { PrivateInitialize(); } ////// Default constructor /// public TableCell(Block blockItem) : base() { PrivateInitialize(); if (blockItem == null) { throw new ArgumentNullException("blockItem"); } this.Blocks.Add(blockItem); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Called when tablecell gets new parent /// /// /// New parent of cell /// internal override void OnNewParent(DependencyObject newParent) { DependencyObject oldParent = this.Parent; TableRow newParentTR = newParent as TableRow; if((newParent != null) && (newParentTR == null)) { throw new InvalidOperationException(SR.Get(SRID.TableInvalidParentNodeType, newParent.GetType().ToString())); } if(oldParent != null) { ((TableRow)oldParent).Cells.InternalRemove(this); } base.OnNewParent(newParent); if ((newParentTR != null) && (newParentTR.Cells != null)) // keep PreSharp happy { newParentTR.Cells.InternalAdd(this); } } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Collection of Blocks contained in this Section. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public BlockCollection Blocks { get { return new BlockCollection(this, /*isOwnerParent*/true); } } ////// Column span property. /// public int ColumnSpan { get { return (int) GetValue(ColumnSpanProperty); } set { SetValue(ColumnSpanProperty, value); } } ////// Row span property. /// public int RowSpan { get { return (int) GetValue(RowSpanProperty); } set { SetValue(RowSpanProperty, value); } } //------------------------------------------------------------------- // // Protected Methods // //---------------------------------------------------------------------- #region Protected Methods ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new TableCellAutomationPeer(this); } #endregion Protected Methods //..................................................................... // // Block Properties // //..................................................................... #region Block Properties ///) /// /// DependencyProperty for public static readonly DependencyProperty PaddingProperty = Block.PaddingProperty.AddOwner( typeof(TableCell), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = Block.BorderThicknessProperty.AddOwner( typeof(TableCell), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = Block.BorderBrushProperty.AddOwner( typeof(TableCell), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = Block.TextAlignmentProperty.AddOwner(typeof(TableCell)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = Block.FlowDirectionProperty.AddOwner(typeof(TableCell)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = Block.LineHeightProperty.AddOwner(typeof(TableCell)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = Block.LineStackingStrategyProperty.AddOwner(typeof(TableCell)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } #endregion Block Properties #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Callback used to notify the Cell about entering model tree. /// internal void OnEnterParentTree() { if(Table != null) { Table.OnStructureChanged(); } } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnExitParentTree() { } ////// Callback used to notify the Cell after it has exitted model tree. (Structures are all updated) /// internal void OnAfterExitParentTree(TableRow row) { if(row.Table != null) { row.Table.OnStructureChanged(); } } ////// ValidateStructure -- caches column index /// internal void ValidateStructure(int columnIndex) { Invariant.Assert(columnIndex >= 0); _columnIndex = columnIndex; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Row owner accessor /// internal TableRow Row { get { return Parent as TableRow; } } ////// Table owner accessor /// internal Table Table { get { return Row != null ? Row.Table : null; } } ////// Cell's index in the parents collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert(value >= -1 && _parentIndex != value); _parentIndex = value; } } ////// Returns cell's parenting row index. /// internal int RowIndex { get { return (Row.Index); } } ////// Returns cell's parenting row group index. /// internal int RowGroupIndex { get { return (Row.RowGroup.Index); } } ////// Returns or sets cell's parenting column index. /// ////// Called by the parent Row during (re)build of the StructuralCache. /// Change of column index causes Layout Dirtyness of the cell. /// internal int ColumnIndex { get { return (_columnIndex); } set { _columnIndex = value; } } ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Private ctor time initialization. /// private void PrivateInitialize() { _parentIndex = -1; _columnIndex = -1; } #endregion Private Methods //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- #region Private Properties #endregion Private Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private int _parentIndex; // cell's index in parent's children collection private int _columnIndex; // Column index for cell. #endregion Private Fields //----------------------------------------------------- // // Properties // //----------------------------------------------------- #region Properties ////// Column span property. /// public static readonly DependencyProperty ColumnSpanProperty = DependencyProperty.Register( "ColumnSpan", typeof(int), typeof(TableCell), new FrameworkPropertyMetadata( 1, FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnColumnSpanChanged)), new ValidateValueCallback(IsValidColumnSpan)); ////// Row span property. /// public static readonly DependencyProperty RowSpanProperty = DependencyProperty.Register( "RowSpan", typeof(int), typeof(TableCell), new FrameworkPropertyMetadata( 1, FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnRowSpanChanged)), new ValidateValueCallback(IsValidRowSpan)); #endregion Properties //------------------------------------------------------ // // Property Invalidation // //----------------------------------------------------- #region Property Invalidation ////// private static bool IsValidRowSpan(object value) { // Maximum row span is limited to 1000000. We do not have any limits from PTS or other formatting restrictions for this value. int span = (int)value; return (span >= 1 && span <= 1000000); } ////// /// private static bool IsValidColumnSpan(object value) { int span = (int)value; const int maxSpan = PTS.Restrictions.tscTableColumnsRestriction; return (span >= 1 && span <= maxSpan); } ////// /// private static void OnColumnSpanChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TableCell cell = (TableCell) d; if(cell.Table != null) { cell.Table.OnStructureChanged(); } // Update AutomaitonPeer. TableCellAutomationPeer peer = ContentElementAutomationPeer.FromElement(cell) as TableCellAutomationPeer; if (peer != null) { peer.OnColumnSpanChanged((int)e.OldValue, (int)e.NewValue); } } ////// /// private static void OnRowSpanChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TableCell cell = (TableCell) d; if(cell.Table != null) { cell.Table.OnStructureChanged(); } // Update AutomaitonPeer. TableCellAutomationPeer peer = ContentElementAutomationPeer.FromElement(cell) as TableCellAutomationPeer; if (peer != null) { peer.OnRowSpanChanged((int)e.OldValue, (int)e.NewValue); } } #endregion Property Invalidation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // ///// // Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Implementation of table cell // // See spec at http://avalon/layout/Tables/WPP%20TableOM.doc // // History: // 05/19/2003 : olego - Created // // Todo list: // 1) OnPropertyInvalidated is expensive and requires investigation: // a) Cell calls to base class implementation which fires back tree // change event - Cell.OnTextContainerChange. As a result Cell.Invalidate // format is called twice. // b) base.OnPropertyInvalidated *always* creates DTR for the whole cell. // Why even AffectsRender causes it? //--------------------------------------------------------------------------- using MS.Internal; using MS.Internal.PtsHost; using MS.Internal.PtsTable; using MS.Internal.Text; using MS.Utility; using System.Diagnostics; using System.Security; using System.Windows.Threading; using System.Collections; using System.Windows; using System.Windows.Automation.Peers; using System.Windows.Media; using System.Windows.Controls; using System.Windows.Markup; using System.ComponentModel; // TypeConverter using System; using MS.Internal.PtsHost.UnsafeNativeMethods; namespace System.Windows.Documents { ////// Implementation of table cell /// [ContentProperty("Blocks")] public class TableCell : TextElement { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors ////// Default constructor /// public TableCell() : base() { PrivateInitialize(); } ////// Default constructor /// public TableCell(Block blockItem) : base() { PrivateInitialize(); if (blockItem == null) { throw new ArgumentNullException("blockItem"); } this.Blocks.Add(blockItem); } #endregion Constructors //------------------------------------------------------ // // Public Methods // //----------------------------------------------------- #region Public Methods ////// Called when tablecell gets new parent /// /// /// New parent of cell /// internal override void OnNewParent(DependencyObject newParent) { DependencyObject oldParent = this.Parent; TableRow newParentTR = newParent as TableRow; if((newParent != null) && (newParentTR == null)) { throw new InvalidOperationException(SR.Get(SRID.TableInvalidParentNodeType, newParent.GetType().ToString())); } if(oldParent != null) { ((TableRow)oldParent).Cells.InternalRemove(this); } base.OnNewParent(newParent); if ((newParentTR != null) && (newParentTR.Cells != null)) // keep PreSharp happy { newParentTR.Cells.InternalAdd(this); } } #endregion Public Methods //------------------------------------------------------ // // Public Properties // //------------------------------------------------------ #region Public Properties ////// Collection of Blocks contained in this Section. /// [DesignerSerializationVisibility(DesignerSerializationVisibility.Content)] public BlockCollection Blocks { get { return new BlockCollection(this, /*isOwnerParent*/true); } } ////// Column span property. /// public int ColumnSpan { get { return (int) GetValue(ColumnSpanProperty); } set { SetValue(ColumnSpanProperty, value); } } ////// Row span property. /// public int RowSpan { get { return (int) GetValue(RowSpanProperty); } set { SetValue(RowSpanProperty, value); } } //------------------------------------------------------------------- // // Protected Methods // //---------------------------------------------------------------------- #region Protected Methods ////// Creates AutomationPeer ( protected override AutomationPeer OnCreateAutomationPeer() { return new TableCellAutomationPeer(this); } #endregion Protected Methods //..................................................................... // // Block Properties // //..................................................................... #region Block Properties ///) /// /// DependencyProperty for public static readonly DependencyProperty PaddingProperty = Block.PaddingProperty.AddOwner( typeof(TableCell), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The Padding property specifies the padding of the element. /// public Thickness Padding { get { return (Thickness)GetValue(PaddingProperty); } set { SetValue(PaddingProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderThicknessProperty = Block.BorderThicknessProperty.AddOwner( typeof(TableCell), new FrameworkPropertyMetadata( new Thickness(), FrameworkPropertyMetadataOptions.AffectsMeasure)); ///property. /// /// The BorderThickness property specifies the border of the element. /// public Thickness BorderThickness { get { return (Thickness)GetValue(BorderThicknessProperty); } set { SetValue(BorderThicknessProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty BorderBrushProperty = Block.BorderBrushProperty.AddOwner( typeof(TableCell), new FrameworkPropertyMetadata( null, FrameworkPropertyMetadataOptions.AffectsRender)); ///property. /// /// The BorderBrush property specifies the brush of the border. /// public Brush BorderBrush { get { return (Brush)GetValue(BorderBrushProperty); } set { SetValue(BorderBrushProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty TextAlignmentProperty = Block.TextAlignmentProperty.AddOwner(typeof(TableCell)); ///property. /// /// /// public TextAlignment TextAlignment { get { return (TextAlignment)GetValue(TextAlignmentProperty); } set { SetValue(TextAlignmentProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty FlowDirectionProperty = Block.FlowDirectionProperty.AddOwner(typeof(TableCell)); ///property. /// /// The FlowDirection property specifies the flow direction of the element. /// public FlowDirection FlowDirection { get { return (FlowDirection)GetValue(FlowDirectionProperty); } set { SetValue(FlowDirectionProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineHeightProperty = Block.LineHeightProperty.AddOwner(typeof(TableCell)); ///property. /// /// The LineHeight property specifies the height of each generated line box. /// [TypeConverter(typeof(LengthConverter))] public double LineHeight { get { return (double)GetValue(LineHeightProperty); } set { SetValue(LineHeightProperty, value); } } ////// DependencyProperty for public static readonly DependencyProperty LineStackingStrategyProperty = Block.LineStackingStrategyProperty.AddOwner(typeof(TableCell)); ///property. /// /// The LineStackingStrategy property specifies how lines are placed /// public LineStackingStrategy LineStackingStrategy { get { return (LineStackingStrategy)GetValue(LineStackingStrategyProperty); } set { SetValue(LineStackingStrategyProperty, value); } } #endregion Block Properties #endregion Public Properties //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods ////// Callback used to notify the Cell about entering model tree. /// internal void OnEnterParentTree() { if(Table != null) { Table.OnStructureChanged(); } } ////// Callback used to notify the RowGroup about exitting model tree. /// internal void OnExitParentTree() { } ////// Callback used to notify the Cell after it has exitted model tree. (Structures are all updated) /// internal void OnAfterExitParentTree(TableRow row) { if(row.Table != null) { row.Table.OnStructureChanged(); } } ////// ValidateStructure -- caches column index /// internal void ValidateStructure(int columnIndex) { Invariant.Assert(columnIndex >= 0); _columnIndex = columnIndex; } #endregion Internal Methods //----------------------------------------------------- // // Internal Properties // //------------------------------------------------------ #region Internal Properties ////// Row owner accessor /// internal TableRow Row { get { return Parent as TableRow; } } ////// Table owner accessor /// internal Table Table { get { return Row != null ? Row.Table : null; } } ////// Cell's index in the parents collection. /// internal int Index { get { return (_parentIndex); } set { Debug.Assert(value >= -1 && _parentIndex != value); _parentIndex = value; } } ////// Returns cell's parenting row index. /// internal int RowIndex { get { return (Row.Index); } } ////// Returns cell's parenting row group index. /// internal int RowGroupIndex { get { return (Row.RowGroup.Index); } } ////// Returns or sets cell's parenting column index. /// ////// Called by the parent Row during (re)build of the StructuralCache. /// Change of column index causes Layout Dirtyness of the cell. /// internal int ColumnIndex { get { return (_columnIndex); } set { _columnIndex = value; } } ////// Marks this element's left edge as visible to IMEs. /// This means element boundaries will act as word breaks. /// internal override bool IsIMEStructuralElement { get { return true; } } #endregion Internal Properties //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods ////// Private ctor time initialization. /// private void PrivateInitialize() { _parentIndex = -1; _columnIndex = -1; } #endregion Private Methods //------------------------------------------------------ // // Private Properties // //----------------------------------------------------- #region Private Properties #endregion Private Properties //------------------------------------------------------ // // Private Fields // //----------------------------------------------------- #region Private Fields private int _parentIndex; // cell's index in parent's children collection private int _columnIndex; // Column index for cell. #endregion Private Fields //----------------------------------------------------- // // Properties // //----------------------------------------------------- #region Properties ////// Column span property. /// public static readonly DependencyProperty ColumnSpanProperty = DependencyProperty.Register( "ColumnSpan", typeof(int), typeof(TableCell), new FrameworkPropertyMetadata( 1, FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnColumnSpanChanged)), new ValidateValueCallback(IsValidColumnSpan)); ////// Row span property. /// public static readonly DependencyProperty RowSpanProperty = DependencyProperty.Register( "RowSpan", typeof(int), typeof(TableCell), new FrameworkPropertyMetadata( 1, FrameworkPropertyMetadataOptions.AffectsMeasure, new PropertyChangedCallback(OnRowSpanChanged)), new ValidateValueCallback(IsValidRowSpan)); #endregion Properties //------------------------------------------------------ // // Property Invalidation // //----------------------------------------------------- #region Property Invalidation ////// private static bool IsValidRowSpan(object value) { // Maximum row span is limited to 1000000. We do not have any limits from PTS or other formatting restrictions for this value. int span = (int)value; return (span >= 1 && span <= 1000000); } ////// /// private static bool IsValidColumnSpan(object value) { int span = (int)value; const int maxSpan = PTS.Restrictions.tscTableColumnsRestriction; return (span >= 1 && span <= maxSpan); } ////// /// private static void OnColumnSpanChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TableCell cell = (TableCell) d; if(cell.Table != null) { cell.Table.OnStructureChanged(); } // Update AutomaitonPeer. TableCellAutomationPeer peer = ContentElementAutomationPeer.FromElement(cell) as TableCellAutomationPeer; if (peer != null) { peer.OnColumnSpanChanged((int)e.OldValue, (int)e.NewValue); } } ////// /// private static void OnRowSpanChanged(DependencyObject d, DependencyPropertyChangedEventArgs e) { TableCell cell = (TableCell) d; if(cell.Table != null) { cell.Table.OnStructureChanged(); } // Update AutomaitonPeer. TableCellAutomationPeer peer = ContentElementAutomationPeer.FromElement(cell) as TableCellAutomationPeer; if (peer != null) { peer.OnRowSpanChanged((int)e.OldValue, (int)e.NewValue); } } #endregion Property Invalidation } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.///
Link Menu
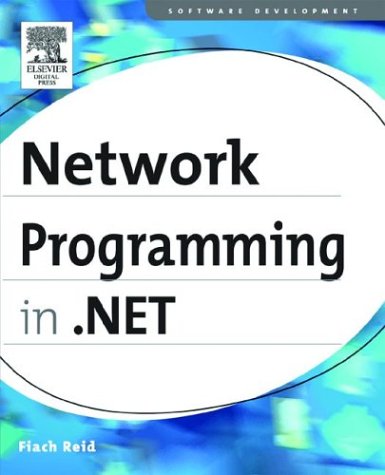
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttributeCollection.cs
- LedgerEntry.cs
- ResourcePart.cs
- _TLSstream.cs
- DrawingImage.cs
- ServiceNameCollection.cs
- SizeConverter.cs
- manifestimages.cs
- RuntimeWrappedException.cs
- Stackframe.cs
- XmlSerializerOperationGenerator.cs
- DelegatingTypeDescriptionProvider.cs
- OracleCommandSet.cs
- AppDomainUnloadedException.cs
- XmlSchemaSimpleContentRestriction.cs
- IdentityModelStringsVersion1.cs
- PixelFormatConverter.cs
- TextRange.cs
- ToolStripSplitButton.cs
- FormViewModeEventArgs.cs
- SignatureHelper.cs
- DataColumn.cs
- DataTableMappingCollection.cs
- ElementAtQueryOperator.cs
- TypeExtensionConverter.cs
- DictionaryTraceRecord.cs
- AmbientProperties.cs
- PackageRelationship.cs
- XmlSchemaSet.cs
- ListViewTableCell.cs
- UnsafeNativeMethods.cs
- ColorDialog.cs
- SRDisplayNameAttribute.cs
- EpmSyndicationContentSerializer.cs
- TagMapCollection.cs
- UpWmlPageAdapter.cs
- WorkflowViewStateService.cs
- IisTraceWebEventProvider.cs
- PromptEventArgs.cs
- FontNamesConverter.cs
- DispatcherHookEventArgs.cs
- GorillaCodec.cs
- SchemaComplexType.cs
- CommandField.cs
- XmlIgnoreAttribute.cs
- ReachDocumentReferenceCollectionSerializer.cs
- CodeArgumentReferenceExpression.cs
- UnsafeCollabNativeMethods.cs
- Property.cs
- Ops.cs
- SchemaImporterExtension.cs
- RayHitTestParameters.cs
- XsltContext.cs
- MembershipValidatePasswordEventArgs.cs
- Certificate.cs
- CompositionTarget.cs
- _ChunkParse.cs
- SqlClientPermission.cs
- ReadOnlyDictionary.cs
- NativeDirectoryServicesQueryAPIs.cs
- EqualityComparer.cs
- ConfigurationManagerHelper.cs
- BrowserDefinitionCollection.cs
- CertificateManager.cs
- DrawingContextWalker.cs
- EventSourceCreationData.cs
- MarkedHighlightComponent.cs
- BamlRecordReader.cs
- ExceptionValidationRule.cs
- HtmlInputControl.cs
- DetailsViewAutoFormat.cs
- SafeRegistryKey.cs
- IConvertible.cs
- Assembly.cs
- WebPartDisplayMode.cs
- QueryableFilterUserControl.cs
- DockPattern.cs
- CircleHotSpot.cs
- BitmapData.cs
- ResXResourceReader.cs
- NullableLongMinMaxAggregationOperator.cs
- PropertyGrid.cs
- DataGridViewCellLinkedList.cs
- StorageMappingFragment.cs
- TableLayoutPanelDesigner.cs
- AnnotationService.cs
- RootBuilder.cs
- NumericUpDownAccelerationCollection.cs
- FormViewInsertedEventArgs.cs
- IdentifierService.cs
- ErrorFormatter.cs
- ViewValidator.cs
- BindingWorker.cs
- ResourcePool.cs
- MemberHolder.cs
- _LocalDataStoreMgr.cs
- InkCanvasSelectionAdorner.cs
- InvokeMethodActivityDesigner.cs
- ImageFormat.cs
- SqlSupersetValidator.cs