Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / ExtensionWindowResizeGrip.cs / 1305376 / ExtensionWindowResizeGrip.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Runtime; using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.Windows.Media; //This class is visual representation of ResizeGrip like control, which is used with ExtenstionWindows to allow //resizing. Actual resize logic is handled by ExtensionSurface class [TemplatePart(Name = "PART_ResizeGrip")] class ExtensionWindowResizeGrip : Control { public static readonly DependencyProperty IconProperty = DependencyProperty.Register("Icon", typeof(DrawingBrush), typeof(ExtensionWindowResizeGrip)); ExtensionWindow parent; ExtensionSurface surface; Point offset; [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.InitializeReferenceTypeStaticFieldsInline, Justification = "Overriding metadata for dependency properties in static constructor is the way suggested by WPF")] static ExtensionWindowResizeGrip() { DefaultStyleKeyProperty.OverrideMetadata( typeof(ExtensionWindowResizeGrip), new FrameworkPropertyMetadata(typeof(ExtensionWindowResizeGrip))); } public DrawingBrush Icon { get { return (DrawingBrush)GetValue(IconProperty); } set { SetValue(IconProperty, value); } } protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { base.OnMouseLeftButtonDown(e); if (this.parent.IsResizable) { this.Cursor = Cursors.SizeNWSE; this.offset = e.GetPosition(this); CaptureMouse(); } } protected override void OnMouseMove(MouseEventArgs args) { base.OnMouseMove(args); if (args.LeftButton == MouseButtonState.Pressed && this.IsMouseCaptured) { Point currentPosition = Mouse.GetPosition(this.parent); currentPosition.Offset(this.offset.X, this.offset.Y); Size newSize = new Size(); newSize.Width = Math.Min(Math.Max(this.parent.MinWidth, currentPosition.X), this.parent.MaxWidth); newSize.Height = Math.Min(Math.Max(this.parent.MinHeight, currentPosition.Y), this.parent.MaxHeight); System.Diagnostics.Debug.WriteLine("NewSize = (" + newSize.Width + "," + newSize.Height + ")"); this.surface.SetSize(this.parent, newSize); } } protected override void OnMouseLeftButtonUp(MouseButtonEventArgs e) { base.OnMouseLeftButtonUp(e); Mouse.OverrideCursor = null; Mouse.Capture(null); } protected override void OnVisualParentChanged(DependencyObject oldParent) { base.OnVisualParentChanged(oldParent); if (!DesignerProperties.GetIsInDesignMode(this) && !ExtensionWindow.TryGetParentExtensionWindow(this, out this.parent, out this.surface)) { Fx.Assert("ExtensionWindowHeader cannot be used outside ExtensionWindow"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Runtime; using System.Windows; using System.Windows.Controls; using System.Windows.Input; using System.Windows.Media; //This class is visual representation of ResizeGrip like control, which is used with ExtenstionWindows to allow //resizing. Actual resize logic is handled by ExtensionSurface class [TemplatePart(Name = "PART_ResizeGrip")] class ExtensionWindowResizeGrip : Control { public static readonly DependencyProperty IconProperty = DependencyProperty.Register("Icon", typeof(DrawingBrush), typeof(ExtensionWindowResizeGrip)); ExtensionWindow parent; ExtensionSurface surface; Point offset; [SuppressMessage(FxCop.Category.Performance, FxCop.Rule.InitializeReferenceTypeStaticFieldsInline, Justification = "Overriding metadata for dependency properties in static constructor is the way suggested by WPF")] static ExtensionWindowResizeGrip() { DefaultStyleKeyProperty.OverrideMetadata( typeof(ExtensionWindowResizeGrip), new FrameworkPropertyMetadata(typeof(ExtensionWindowResizeGrip))); } public DrawingBrush Icon { get { return (DrawingBrush)GetValue(IconProperty); } set { SetValue(IconProperty, value); } } protected override void OnMouseLeftButtonDown(MouseButtonEventArgs e) { base.OnMouseLeftButtonDown(e); if (this.parent.IsResizable) { this.Cursor = Cursors.SizeNWSE; this.offset = e.GetPosition(this); CaptureMouse(); } } protected override void OnMouseMove(MouseEventArgs args) { base.OnMouseMove(args); if (args.LeftButton == MouseButtonState.Pressed && this.IsMouseCaptured) { Point currentPosition = Mouse.GetPosition(this.parent); currentPosition.Offset(this.offset.X, this.offset.Y); Size newSize = new Size(); newSize.Width = Math.Min(Math.Max(this.parent.MinWidth, currentPosition.X), this.parent.MaxWidth); newSize.Height = Math.Min(Math.Max(this.parent.MinHeight, currentPosition.Y), this.parent.MaxHeight); System.Diagnostics.Debug.WriteLine("NewSize = (" + newSize.Width + "," + newSize.Height + ")"); this.surface.SetSize(this.parent, newSize); } } protected override void OnMouseLeftButtonUp(MouseButtonEventArgs e) { base.OnMouseLeftButtonUp(e); Mouse.OverrideCursor = null; Mouse.Capture(null); } protected override void OnVisualParentChanged(DependencyObject oldParent) { base.OnVisualParentChanged(oldParent); if (!DesignerProperties.GetIsInDesignMode(this) && !ExtensionWindow.TryGetParentExtensionWindow(this, out this.parent, out this.surface)) { Fx.Assert("ExtensionWindowHeader cannot be used outside ExtensionWindow"); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
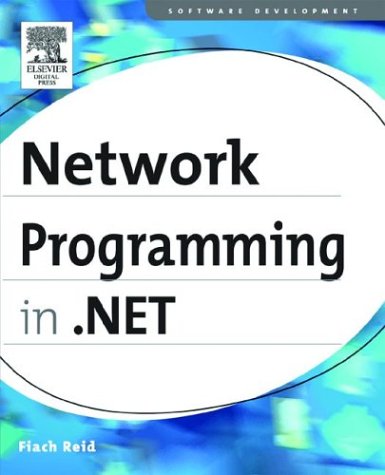
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EditingScopeUndoUnit.cs
- ToolboxBitmapAttribute.cs
- Condition.cs
- StatusBarPanelClickEvent.cs
- WebPartAuthorizationEventArgs.cs
- PreApplicationStartMethodAttribute.cs
- XmlAutoDetectWriter.cs
- MatrixAnimationBase.cs
- ReadOnlyMetadataCollection.cs
- XmlWriter.cs
- CommandEventArgs.cs
- BaseHashHelper.cs
- WebHttpEndpoint.cs
- XmlnsDefinitionAttribute.cs
- WorkflowServiceBuildProvider.cs
- OutputScopeManager.cs
- AuthenticationServiceManager.cs
- ADRoleFactoryConfiguration.cs
- DebugView.cs
- WebPartMenu.cs
- CaseInsensitiveComparer.cs
- WebPartCancelEventArgs.cs
- WebPartMenuStyle.cs
- DataList.cs
- NullableFloatAverageAggregationOperator.cs
- InternalBase.cs
- BitmapEffectCollection.cs
- MessagePartDescriptionCollection.cs
- GridViewUpdateEventArgs.cs
- DataListItem.cs
- FigureParaClient.cs
- Accessors.cs
- TypeUtil.cs
- WebConfigurationHost.cs
- SmiSettersStream.cs
- ExtensibleClassFactory.cs
- LinkLabelLinkClickedEvent.cs
- ComponentDispatcherThread.cs
- DropShadowEffect.cs
- Query.cs
- OutOfMemoryException.cs
- SecurityRuntime.cs
- RegexCompilationInfo.cs
- webclient.cs
- ClrPerspective.cs
- BitmapEffectInputData.cs
- AssertSection.cs
- HtmlContainerControl.cs
- TextComposition.cs
- _SSPISessionCache.cs
- MetabaseSettingsIis7.cs
- LocatorManager.cs
- PageEventArgs.cs
- ModelProperty.cs
- TypeHelper.cs
- FactorySettingsElement.cs
- CodeSnippetExpression.cs
- TransactionManagerProxy.cs
- DesignTimeTemplateParser.cs
- XsltArgumentList.cs
- AlternationConverter.cs
- SQLByteStorage.cs
- GifBitmapEncoder.cs
- StringUtil.cs
- NullableIntMinMaxAggregationOperator.cs
- ExpressionParser.cs
- ModelTreeEnumerator.cs
- HttpVersion.cs
- ObjectViewListener.cs
- IPAddressCollection.cs
- DSGeneratorProblem.cs
- WebColorConverter.cs
- StackOverflowException.cs
- SchemaImporter.cs
- SHA1CryptoServiceProvider.cs
- UIElement.cs
- CompilerTypeWithParams.cs
- ActivityWithResultWrapper.cs
- BasicKeyConstraint.cs
- PathFigureCollection.cs
- SortedList.cs
- WebPermission.cs
- BreakRecordTable.cs
- Tokenizer.cs
- CategoryNameCollection.cs
- BaseUriHelper.cs
- HTMLTextWriter.cs
- BinaryObjectInfo.cs
- SurrogateSelector.cs
- ControlValuePropertyAttribute.cs
- AppDomainProtocolHandler.cs
- Header.cs
- ServiceModelStringsVersion1.cs
- BamlLocalizationDictionary.cs
- TreeSet.cs
- XslTransform.cs
- AuthenticationServiceManager.cs
- GenericAuthenticationEventArgs.cs
- DoubleAnimation.cs
- ProtocolsConfigurationHandler.cs