Code:
/ FXUpdate3074 / FXUpdate3074 / 1.1 / untmp / whidbey / QFE / ndp / fx / src / xsp / System / Web / Compilation / CompilerTypeWithParams.cs / 2 / CompilerTypeWithParams.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Compilation { using System; using System.Security.Permissions; using System.IO; using System.Collections; using System.Globalization; using System.CodeDom; using System.CodeDom.Compiler; using System.Web.Hosting; using System.Web.Util; using System.Web.UI; using System.Web.Configuration; /* * This class describes a CodeDom compiler, along with the parameters that it uses. * The reason we need this class is that if two files both use the same language, * but ask for different command line options (e.g. debug vs retail), we will not * be able to compile them together. So effectively, we need to treat them as * different languages. */ [AspNetHostingPermission(SecurityAction.LinkDemand, Level=AspNetHostingPermissionLevel.Minimal)] public sealed class CompilerType { private Type _codeDomProviderType; public Type CodeDomProviderType { get { return _codeDomProviderType; } } private CompilerParameters _compilParams; public CompilerParameters CompilerParameters { get { return _compilParams; } } internal CompilerType(Type codeDomProviderType, CompilerParameters compilParams) { Debug.Assert(codeDomProviderType != null); _codeDomProviderType = codeDomProviderType; if (compilParams == null) _compilParams = new CompilerParameters(); else _compilParams = compilParams; } internal CompilerType Clone() { // Clone the CompilerParameters to make sure the original is untouched return new CompilerType(_codeDomProviderType, CloneCompilerParameters()); } private CompilerParameters CloneCompilerParameters() { CompilerParameters copy = new CompilerParameters(); copy.IncludeDebugInformation = _compilParams.IncludeDebugInformation; copy.TreatWarningsAsErrors = _compilParams.TreatWarningsAsErrors; copy.WarningLevel = _compilParams.WarningLevel; copy.CompilerOptions = _compilParams.CompilerOptions; return copy; } public override int GetHashCode() { return _codeDomProviderType.GetHashCode(); } public override bool Equals(Object o) { CompilerType other = o as CompilerType; if (o == null) return false; return _codeDomProviderType == other._codeDomProviderType && _compilParams.WarningLevel == other._compilParams.WarningLevel && _compilParams.IncludeDebugInformation == other._compilParams.IncludeDebugInformation && _compilParams.CompilerOptions == other._compilParams.CompilerOptions; } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies) { return CreateAssemblyBuilder(compConfig, referencedAssemblies, null /*generatedFilesDir*/, null /*outputAssemblyName*/); } internal AssemblyBuilder CreateAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, string generatedFilesDir, string outputAssemblyName) { // Create a special AssemblyBuilder when we're only supposed to generate // source files but not compile them (for ClientBuildManager.GetCodeDirectoryInformation) if (generatedFilesDir != null) { return new CbmCodeGeneratorBuildProviderHost(compConfig, referencedAssemblies, this, generatedFilesDir, outputAssemblyName); } return new AssemblyBuilder(compConfig, referencedAssemblies, this, outputAssemblyName); } private static CompilerType GetDefaultCompilerTypeWithParams( CompilationSection compConfig, VirtualPath configPath) { // By default, use C# when no provider is asking for a specific language return CompilationUtil.GetCSharpCompilerInfo(compConfig, configPath); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string outputAssemblyName) { return GetDefaultAssemblyBuilder(compConfig, referencedAssemblies, configPath, null /*generatedFilesDir*/, outputAssemblyName); } internal static AssemblyBuilder GetDefaultAssemblyBuilder(CompilationSection compConfig, ICollection referencedAssemblies, VirtualPath configPath, string generatedFilesDir, string outputAssemblyName) { CompilerType ctwp = GetDefaultCompilerTypeWithParams(compConfig, configPath); return ctwp.CreateAssemblyBuilder(compConfig, referencedAssemblies, generatedFilesDir, outputAssemblyName); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
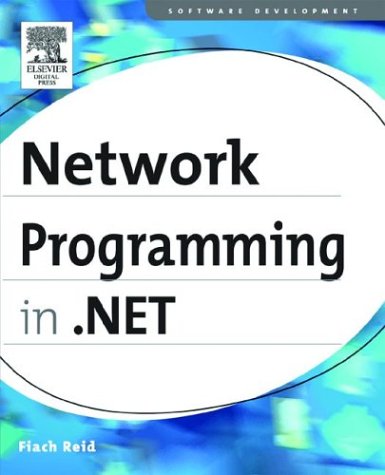
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ListViewItemSelectionChangedEvent.cs
- Table.cs
- DoubleIndependentAnimationStorage.cs
- OleServicesContext.cs
- AssociatedControlConverter.cs
- UrlPath.cs
- ContextQuery.cs
- BamlRecords.cs
- TargetPerspective.cs
- VarRemapper.cs
- XmlWrappingReader.cs
- Application.cs
- OracleCommandSet.cs
- OracleConnection.cs
- StyleTypedPropertyAttribute.cs
- OrCondition.cs
- ClientTargetSection.cs
- TemplateControl.cs
- EntityContainer.cs
- ImpersonateTokenRef.cs
- PenThreadPool.cs
- Section.cs
- RequestTimeoutManager.cs
- PageClientProxyGenerator.cs
- SqlRowUpdatedEvent.cs
- PageFunction.cs
- OleDbRowUpdatedEvent.cs
- DefaultObjectMappingItemCollection.cs
- InputLanguage.cs
- UpdateException.cs
- InfoCardRSAPKCS1SignatureFormatter.cs
- UserPreferenceChangedEventArgs.cs
- AppSettingsExpressionBuilder.cs
- DropDownButton.cs
- NextPreviousPagerField.cs
- TemplateParser.cs
- Bookmark.cs
- DbConnectionPoolGroupProviderInfo.cs
- WsdlWriter.cs
- FileUtil.cs
- DesignerEditorPartChrome.cs
- Stack.cs
- ClickablePoint.cs
- Update.cs
- UnitySerializationHolder.cs
- ExtendedProperty.cs
- SpStreamWrapper.cs
- CardSpaceSelector.cs
- CapabilitiesUse.cs
- DynamicILGenerator.cs
- CodeRegionDirective.cs
- ErrorsHelper.cs
- RequestValidator.cs
- DuplicateWaitObjectException.cs
- TreeIterators.cs
- SignerInfo.cs
- RoamingStoreFile.cs
- Update.cs
- BoolExpression.cs
- PropertyGridEditorPart.cs
- RectIndependentAnimationStorage.cs
- PropertyCondition.cs
- PeerToPeerException.cs
- CryptoConfig.cs
- CellQuery.cs
- ColorKeyFrameCollection.cs
- WindowsAltTab.cs
- GlyphingCache.cs
- GridViewCommandEventArgs.cs
- GZipStream.cs
- ImageMetadata.cs
- ConversionValidationRule.cs
- WebMessageEncodingElement.cs
- SimpleType.cs
- DesignerDataTableBase.cs
- XmlSchemaInclude.cs
- ToolStripButton.cs
- HttpChannelBindingToken.cs
- ReadWriteObjectLock.cs
- QueryOutputWriter.cs
- RequestQueue.cs
- ProfileEventArgs.cs
- DataSourceProvider.cs
- ListBoxItem.cs
- _ServiceNameStore.cs
- DiscoveryReferences.cs
- PngBitmapDecoder.cs
- DataGridViewLayoutData.cs
- ColorKeyFrameCollection.cs
- WebPartTransformerAttribute.cs
- CapabilitiesAssignment.cs
- CodeLinePragma.cs
- TextPattern.cs
- OleDbFactory.cs
- ResourceProviderFactory.cs
- QilStrConcat.cs
- ObjectDataSourceDesigner.cs
- CharacterString.cs
- TypeConverter.cs
- SimpleWorkerRequest.cs