Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / ViewUtilities.cs / 1407647 / ViewUtilities.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Activities.Presentation.Model; using System.Activities.Presentation.Services; static class ViewUtilities { const string ExpandViewStateKey = "IsExpanded"; internal static bool DoesParentAlwaysExpandChildren(ModelItem modelItem, EditingContext context) { return IsParentOfType(modelItem, typeof(IExpandChild), context); } internal static bool DoesParentAlwaysCollapseChildren(ModelItem modelItem, EditingContext context) { bool parentAlwaysCollapsesChild = false; Type parentDesignerType = GetParentDesignerType(modelItem, context); if (typeof(WorkflowViewElement).IsAssignableFrom(parentDesignerType)) { ActivityDesignerOptionsAttribute options = WorkflowViewService.GetAttribute(parentDesignerType); parentAlwaysCollapsesChild = (options != null && options.AlwaysCollapseChildren); } return parentAlwaysCollapsesChild; } // Determines whether a particular ModelItem's view will be visible for a given breadcrumb root. //It depends on whether the intermediate designers are expanded or collapsed. internal static bool IsViewVisible(ModelItem child, ModelItem root, EditingContext context) { ModelItem parent = child; while (parent != null && !object.Equals(parent, root)) { parent = GetParentModelItemWithView(parent, context, false); if (!IsViewExpanded(parent, context)) { break; } } return object.Equals(parent, root); } // Get the first parent ModelItem that has a view internal static ModelItem GetParentModelItemWithView(ModelItem modelItem, EditingContext context, bool allowDrillIn) { if (modelItem == null) { return null; } ModelItem parent = modelItem.Parent; WorkflowViewService viewService = GetViewService(context); while (parent != null) { ActivityDesignerOptionsAttribute options = WorkflowViewService.GetAttribute (parent.ItemType); Type viewType = viewService.GetDesignerType(parent.ItemType); if (typeof(WorkflowViewElement).IsAssignableFrom(viewType) && (!allowDrillIn || options == null || options.AllowDrillIn)) { break; } parent = parent.Parent; } return parent; } // Determine whether the view of a ModelItem is expanded without querying the view itself - the view may have not been constructed. internal static bool IsViewExpanded(ModelItem modelItem, EditingContext context) { if (modelItem == null) { return false; } bool isDesignerExpanded = true; bool isDesignerPinned = false; object isExpandedViewState = GetViewStateService(context).RetrieveViewState(modelItem, ExpandViewStateKey); object isPinnedViewState = GetViewStateService(context).RetrieveViewState(modelItem, WorkflowViewElement.PinnedViewStateKey); if (isExpandedViewState != null) { isDesignerExpanded = (bool)isExpandedViewState; } if (isPinnedViewState != null) { isDesignerPinned = (bool)isPinnedViewState; } DesignerView designerView = context.Services.GetService (); return ShouldShowExpanded(IsBreadcrumbRoot(modelItem, context), DoesParentAlwaysExpandChildren(modelItem, context), DoesParentAlwaysCollapseChildren(modelItem, context), isDesignerExpanded, designerView.ShouldExpandAll, designerView.ShouldCollapseAll, isDesignerPinned); } internal static bool IsBreadcrumbRoot(ModelItem modelItem, EditingContext context) { DesignerView designerView = context.Services.GetService (); return modelItem != null && modelItem.View != null && modelItem.View.Equals(designerView.RootDesigner); } internal static bool ShouldShowExpanded( bool isRootDesigner, bool parentAlwaysExpandChildren, bool parentAlwaysCollapseChildren, bool expandState, bool expandAll, bool collapseAll, bool pinState) { //ShowExpanded based on ExpandAll, CollapseAll, PinState, ExpandState bool showExpanded; if (pinState) { showExpanded = expandState; } else { showExpanded = !collapseAll && (expandAll || expandState); } //return value based on the position of the element in the workflow tree. return (isRootDesigner || parentAlwaysExpandChildren || (!parentAlwaysCollapseChildren && showExpanded)); } static WorkflowViewService GetViewService(EditingContext context) { return context.Services.GetService () as WorkflowViewService; } static ViewStateService GetViewStateService(EditingContext context) { return context.Services.GetService (); } //Checks to see if the immediate parent WorkflowViewElement is of type "parentType". static bool IsParentOfType(ModelItem modelItem, Type parentType, EditingContext context) { Type parentDesignerType = GetParentDesignerType(modelItem, context); return parentType.IsAssignableFrom(parentDesignerType); } static Type GetParentDesignerType(ModelItem modelItem, EditingContext context) { ModelItem parent = GetParentModelItemWithView(modelItem, context, false); if (parent != null) { return GetViewService(context).GetDesignerType(parent.ItemType); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Activities.Presentation.Model; using System.Activities.Presentation.Services; static class ViewUtilities { const string ExpandViewStateKey = "IsExpanded"; internal static bool DoesParentAlwaysExpandChildren(ModelItem modelItem, EditingContext context) { return IsParentOfType(modelItem, typeof(IExpandChild), context); } internal static bool DoesParentAlwaysCollapseChildren(ModelItem modelItem, EditingContext context) { bool parentAlwaysCollapsesChild = false; Type parentDesignerType = GetParentDesignerType(modelItem, context); if (typeof(WorkflowViewElement).IsAssignableFrom(parentDesignerType)) { ActivityDesignerOptionsAttribute options = WorkflowViewService.GetAttribute (parentDesignerType); parentAlwaysCollapsesChild = (options != null && options.AlwaysCollapseChildren); } return parentAlwaysCollapsesChild; } // Determines whether a particular ModelItem's view will be visible for a given breadcrumb root. //It depends on whether the intermediate designers are expanded or collapsed. internal static bool IsViewVisible(ModelItem child, ModelItem root, EditingContext context) { ModelItem parent = child; while (parent != null && !object.Equals(parent, root)) { parent = GetParentModelItemWithView(parent, context, false); if (!IsViewExpanded(parent, context)) { break; } } return object.Equals(parent, root); } // Get the first parent ModelItem that has a view internal static ModelItem GetParentModelItemWithView(ModelItem modelItem, EditingContext context, bool allowDrillIn) { if (modelItem == null) { return null; } ModelItem parent = modelItem.Parent; WorkflowViewService viewService = GetViewService(context); while (parent != null) { ActivityDesignerOptionsAttribute options = WorkflowViewService.GetAttribute (parent.ItemType); Type viewType = viewService.GetDesignerType(parent.ItemType); if (typeof(WorkflowViewElement).IsAssignableFrom(viewType) && (!allowDrillIn || options == null || options.AllowDrillIn)) { break; } parent = parent.Parent; } return parent; } // Determine whether the view of a ModelItem is expanded without querying the view itself - the view may have not been constructed. internal static bool IsViewExpanded(ModelItem modelItem, EditingContext context) { if (modelItem == null) { return false; } bool isDesignerExpanded = true; bool isDesignerPinned = false; object isExpandedViewState = GetViewStateService(context).RetrieveViewState(modelItem, ExpandViewStateKey); object isPinnedViewState = GetViewStateService(context).RetrieveViewState(modelItem, WorkflowViewElement.PinnedViewStateKey); if (isExpandedViewState != null) { isDesignerExpanded = (bool)isExpandedViewState; } if (isPinnedViewState != null) { isDesignerPinned = (bool)isPinnedViewState; } DesignerView designerView = context.Services.GetService (); return ShouldShowExpanded(IsBreadcrumbRoot(modelItem, context), DoesParentAlwaysExpandChildren(modelItem, context), DoesParentAlwaysCollapseChildren(modelItem, context), isDesignerExpanded, designerView.ShouldExpandAll, designerView.ShouldCollapseAll, isDesignerPinned); } internal static bool IsBreadcrumbRoot(ModelItem modelItem, EditingContext context) { DesignerView designerView = context.Services.GetService (); return modelItem != null && modelItem.View != null && modelItem.View.Equals(designerView.RootDesigner); } internal static bool ShouldShowExpanded( bool isRootDesigner, bool parentAlwaysExpandChildren, bool parentAlwaysCollapseChildren, bool expandState, bool expandAll, bool collapseAll, bool pinState) { //ShowExpanded based on ExpandAll, CollapseAll, PinState, ExpandState bool showExpanded; if (pinState) { showExpanded = expandState; } else { showExpanded = !collapseAll && (expandAll || expandState); } //return value based on the position of the element in the workflow tree. return (isRootDesigner || parentAlwaysExpandChildren || (!parentAlwaysCollapseChildren && showExpanded)); } static WorkflowViewService GetViewService(EditingContext context) { return context.Services.GetService () as WorkflowViewService; } static ViewStateService GetViewStateService(EditingContext context) { return context.Services.GetService (); } //Checks to see if the immediate parent WorkflowViewElement is of type "parentType". static bool IsParentOfType(ModelItem modelItem, Type parentType, EditingContext context) { Type parentDesignerType = GetParentDesignerType(modelItem, context); return parentType.IsAssignableFrom(parentDesignerType); } static Type GetParentDesignerType(ModelItem modelItem, EditingContext context) { ModelItem parent = GetParentModelItemWithView(modelItem, context, false); if (parent != null) { return GetViewService(context).GetDesignerType(parent.ItemType); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
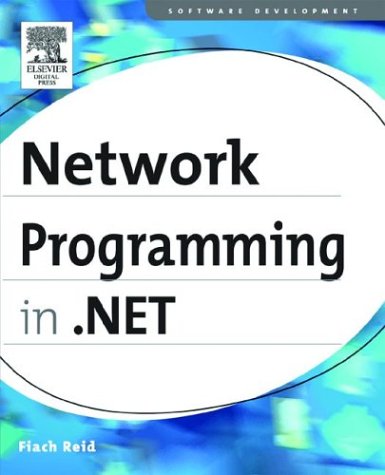
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- followingsibling.cs
- FormsAuthenticationUser.cs
- HitTestFilterBehavior.cs
- EventInfo.cs
- ObjectViewEntityCollectionData.cs
- DeclaredTypeValidatorAttribute.cs
- StrokeNode.cs
- FastPropertyAccessor.cs
- PointIndependentAnimationStorage.cs
- StylusEventArgs.cs
- ReturnType.cs
- ApplicationSettingsBase.cs
- CharacterString.cs
- InputScopeConverter.cs
- HostVisual.cs
- TableCell.cs
- DbReferenceCollection.cs
- QilUnary.cs
- DoubleLinkList.cs
- DataGridItemAttachedStorage.cs
- GenericEnumConverter.cs
- HttpWriter.cs
- GeneralTransform2DTo3D.cs
- CodeTypeReferenceExpression.cs
- InvalidStoreProtectionKeyException.cs
- VisualTarget.cs
- TiffBitmapDecoder.cs
- PermissionToken.cs
- FileDialog.cs
- _ChunkParse.cs
- ShutDownListener.cs
- cryptoapiTransform.cs
- TextElementEnumerator.cs
- RegexTypeEditor.cs
- ExclusiveTcpListener.cs
- Triplet.cs
- FullTextBreakpoint.cs
- DBSchemaRow.cs
- ToolStripControlHost.cs
- ContractValidationHelper.cs
- LinearQuaternionKeyFrame.cs
- ValueExpressions.cs
- SqlAliasesReferenced.cs
- activationcontext.cs
- Label.cs
- BaseParaClient.cs
- HandleExceptionArgs.cs
- ElementsClipboardData.cs
- OdbcConnectionString.cs
- documentsequencetextcontainer.cs
- BehaviorEditorPart.cs
- PropertyGrid.cs
- TypedRowHandler.cs
- SetterBaseCollection.cs
- StylusButtonEventArgs.cs
- Gdiplus.cs
- GenerateTemporaryTargetAssembly.cs
- DataSet.cs
- DataContract.cs
- ListBindableAttribute.cs
- ThaiBuddhistCalendar.cs
- DoWorkEventArgs.cs
- ListViewItemEventArgs.cs
- DriveNotFoundException.cs
- PopupEventArgs.cs
- SecurityProtocolCorrelationState.cs
- GeneralTransform2DTo3D.cs
- EncoderReplacementFallback.cs
- EntityDataSourceView.cs
- XPathParser.cs
- TouchesOverProperty.cs
- UserNameSecurityTokenAuthenticator.cs
- PriorityChain.cs
- ListViewItemCollectionEditor.cs
- DoubleAnimation.cs
- StringSource.cs
- PersonalizationAdministration.cs
- XmlSchemaComplexContentExtension.cs
- ToolStripSplitButton.cs
- BoolExpr.cs
- XmlCompatibilityReader.cs
- AnimationClockResource.cs
- UserMapPath.cs
- BCLDebug.cs
- SettingsPropertyCollection.cs
- ListBoxItem.cs
- HostAdapter.cs
- TextTreeInsertElementUndoUnit.cs
- ObjectCloneHelper.cs
- PersonalizationProvider.cs
- GuidConverter.cs
- SchemaLookupTable.cs
- PageContent.cs
- recordstatefactory.cs
- DictionaryKeyPropertyAttribute.cs
- RuntimeConfigLKG.cs
- WebPartPersonalization.cs
- CookieHandler.cs
- WindowsRichEditRange.cs
- StoreAnnotationsMap.cs