Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Serializer / CollectionMarkupSerializer.cs / 1305376 / CollectionMarkupSerializer.cs
namespace System.Workflow.ComponentModel.Serialization { using System; using System.IO; using System.CodeDom; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Xml; using System.Xml.Serialization; using System.Reflection; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Globalization; using System.Workflow.ComponentModel.Compiler; using System.Workflow.ComponentModel.Design; using System.Runtime.Serialization; using System.Security.Permissions; using System.Collections.ObjectModel; using System.Drawing; #region Class CollectionMarkupSerializer internal class CollectionMarkupSerializer : WorkflowMarkupSerializer { protected internal override IList GetChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (obj == null) throw new ArgumentNullException("obj"); if (!IsValidCollectionType(obj.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, obj.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); IEnumerable enumerable = obj as IEnumerable; ArrayList arrayList = new ArrayList(); foreach (object containedObj in enumerable) arrayList.Add(containedObj); return arrayList; } protected internal override PropertyInfo[] GetProperties(WorkflowMarkupSerializationManager serializationManager, object obj) { return new PropertyInfo[] { }; } protected internal override bool ShouldSerializeValue(WorkflowMarkupSerializationManager serializationManager, object value) { if (value == null) return false; if (!IsValidCollectionType(value.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, value.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); IEnumerable enumerable = value as IEnumerable; foreach (object obj in enumerable) return true; return false; } protected internal override void ClearChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (obj == null) throw new ArgumentNullException("obj"); if (!IsValidCollectionType(obj.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, obj.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); ICollection collection = obj as ICollection; if (collection == null) obj.GetType().InvokeMember("Clear", BindingFlags.Public | BindingFlags.InvokeMethod | BindingFlags.Instance, null, obj, new object[] {}, CultureInfo.InvariantCulture); } protected internal override void AddChild(WorkflowMarkupSerializationManager serializationManager, object parentObj, object childObj) { if (parentObj == null) throw new ArgumentNullException("parentObj"); if (!IsValidCollectionType(parentObj.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, parentObj.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); parentObj.GetType().InvokeMember("Add", BindingFlags.Public | BindingFlags.InvokeMethod | BindingFlags.Instance, null, parentObj, new object[] { childObj }, CultureInfo.InvariantCulture); } internal static bool IsValidCollectionType(Type collectionType) { if (collectionType == null) return false; if (typeof(Array).IsAssignableFrom(collectionType)) return false; return (typeof(ICollection).IsAssignableFrom(collectionType) || (collectionType.IsGenericType && (typeof(ICollection<>).IsAssignableFrom(collectionType.GetGenericTypeDefinition()) || typeof(IList<>).IsAssignableFrom(collectionType.GetGenericTypeDefinition())))); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Serialization { using System; using System.IO; using System.CodeDom; using System.ComponentModel; using System.ComponentModel.Design; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Xml; using System.Xml.Serialization; using System.Reflection; using System.Collections.Generic; using System.Diagnostics; using System.Text; using System.Globalization; using System.Workflow.ComponentModel.Compiler; using System.Workflow.ComponentModel.Design; using System.Runtime.Serialization; using System.Security.Permissions; using System.Collections.ObjectModel; using System.Drawing; #region Class CollectionMarkupSerializer internal class CollectionMarkupSerializer : WorkflowMarkupSerializer { protected internal override IList GetChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (obj == null) throw new ArgumentNullException("obj"); if (!IsValidCollectionType(obj.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, obj.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); IEnumerable enumerable = obj as IEnumerable; ArrayList arrayList = new ArrayList(); foreach (object containedObj in enumerable) arrayList.Add(containedObj); return arrayList; } protected internal override PropertyInfo[] GetProperties(WorkflowMarkupSerializationManager serializationManager, object obj) { return new PropertyInfo[] { }; } protected internal override bool ShouldSerializeValue(WorkflowMarkupSerializationManager serializationManager, object value) { if (value == null) return false; if (!IsValidCollectionType(value.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, value.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); IEnumerable enumerable = value as IEnumerable; foreach (object obj in enumerable) return true; return false; } protected internal override void ClearChildren(WorkflowMarkupSerializationManager serializationManager, object obj) { if (obj == null) throw new ArgumentNullException("obj"); if (!IsValidCollectionType(obj.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, obj.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); ICollection collection = obj as ICollection; if (collection == null) obj.GetType().InvokeMember("Clear", BindingFlags.Public | BindingFlags.InvokeMethod | BindingFlags.Instance, null, obj, new object[] {}, CultureInfo.InvariantCulture); } protected internal override void AddChild(WorkflowMarkupSerializationManager serializationManager, object parentObj, object childObj) { if (parentObj == null) throw new ArgumentNullException("parentObj"); if (!IsValidCollectionType(parentObj.GetType())) throw new Exception(SR.GetString(SR.Error_SerializerTypeRequirement, parentObj.GetType().FullName, typeof(ICollection).FullName, typeof(ICollection<>).FullName)); parentObj.GetType().InvokeMember("Add", BindingFlags.Public | BindingFlags.InvokeMethod | BindingFlags.Instance, null, parentObj, new object[] { childObj }, CultureInfo.InvariantCulture); } internal static bool IsValidCollectionType(Type collectionType) { if (collectionType == null) return false; if (typeof(Array).IsAssignableFrom(collectionType)) return false; return (typeof(ICollection).IsAssignableFrom(collectionType) || (collectionType.IsGenericType && (typeof(ICollection<>).IsAssignableFrom(collectionType.GetGenericTypeDefinition()) || typeof(IList<>).IsAssignableFrom(collectionType.GetGenericTypeDefinition())))); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
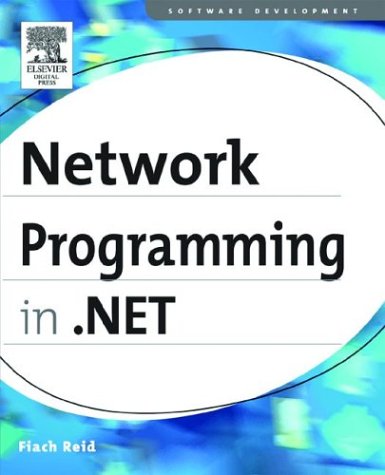
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MatrixAnimationUsingKeyFrames.cs
- BaseAutoFormat.cs
- UiaCoreApi.cs
- HttpModuleAction.cs
- SynchronizationScope.cs
- BitmapCodecInfo.cs
- WsatServiceAddress.cs
- SecurityKeyType.cs
- TableRowsCollectionEditor.cs
- DSASignatureFormatter.cs
- ButtonBaseAdapter.cs
- EvidenceTypeDescriptor.cs
- CompositeKey.cs
- ForeignKeyFactory.cs
- ObjectFullSpanRewriter.cs
- RuleSetDialog.Designer.cs
- HashRepartitionEnumerator.cs
- OpacityConverter.cs
- ChangeConflicts.cs
- DataGridViewCellFormattingEventArgs.cs
- CodeSnippetExpression.cs
- ValidationResult.cs
- PageFunction.cs
- PartBasedPackageProperties.cs
- TextSearch.cs
- EventWaitHandleSecurity.cs
- ThreadSafeList.cs
- ConnectionStringsSection.cs
- AlternateViewCollection.cs
- ToolStripCodeDomSerializer.cs
- SoapMessage.cs
- DiscreteKeyFrames.cs
- SafeProcessHandle.cs
- PropertyMapper.cs
- EntityCommand.cs
- ColumnCollection.cs
- XmlILOptimizerVisitor.cs
- EncryptedKey.cs
- CodeDirectiveCollection.cs
- ClientTargetCollection.cs
- HtmlInputCheckBox.cs
- BindingWorker.cs
- RC2.cs
- PerformanceCounterPermissionEntry.cs
- ProfileInfo.cs
- EnumType.cs
- CapabilitiesPattern.cs
- SqlCommand.cs
- ListView.cs
- UniqueSet.cs
- WindowHideOrCloseTracker.cs
- _OSSOCK.cs
- DefinitionUpdate.cs
- RootBuilder.cs
- MetaTableHelper.cs
- BitStack.cs
- VBIdentifierDesigner.xaml.cs
- CompilationSection.cs
- TreeNodeStyleCollection.cs
- BezierSegment.cs
- DockPanel.cs
- PackageDigitalSignatureManager.cs
- FtpWebRequest.cs
- RepeatInfo.cs
- Column.cs
- BamlBinaryReader.cs
- XmlNotation.cs
- InputChannelAcceptor.cs
- NameValueCollection.cs
- DataGridViewSelectedRowCollection.cs
- Vector3DCollection.cs
- MsmqChannelFactoryBase.cs
- BufferedGraphics.cs
- DesignSurface.cs
- SerializationSectionGroup.cs
- VirtualizedContainerService.cs
- NavigationWindowAutomationPeer.cs
- DataTableTypeConverter.cs
- ShaderRenderModeValidation.cs
- SchemaInfo.cs
- MenuBase.cs
- ContainerActivationHelper.cs
- AutoGeneratedFieldProperties.cs
- SyndicationElementExtension.cs
- CapabilitiesRule.cs
- ISessionStateStore.cs
- FileIOPermission.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- AxisAngleRotation3D.cs
- CodeTypeDeclarationCollection.cs
- HttpPostProtocolImporter.cs
- LinqDataSource.cs
- SqlConnectionPoolGroupProviderInfo.cs
- DiagnosticTraceSource.cs
- XmlSchemaDocumentation.cs
- PackageDigitalSignature.cs
- PrintDialogException.cs
- UInt32.cs
- WinFormsUtils.cs
- CodeNamespaceCollection.cs