Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Runtime / Serialization / Formatters / SoapFault.cs / 1305376 / SoapFault.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SoapFault ** **Author: Peter de Jong ([....]) ** ** Purpose: Specifies information for a Soap Fault ** ** Date: June 27, 2000 ** ===========================================================*/ #if FEATURE_REMOTING namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Globalization; using System.Security.Permissions; //* Class holds soap fault information [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class SoapFault : ISerializable { String faultCode; String faultString; String faultActor; [SoapField(Embedded=true)] Object detail; public SoapFault() { } public SoapFault(String faultCode, String faultString, String faultActor, ServerFault serverFault) { this.faultCode = faultCode; this.faultString = faultString; this.faultActor = faultActor; this.detail = serverFault; } internal SoapFault(SerializationInfo info, StreamingContext context) { SerializationInfoEnumerator siEnum = info.GetEnumerator(); while(siEnum.MoveNext()) { String name = siEnum.Name; Object value = siEnum.Value; SerTrace.Log(this, "SetObjectData enum ",name," value ",value); if (String.Compare(name, "faultCode", true, CultureInfo.InvariantCulture) == 0) { int index = ((String)value).IndexOf(':'); if (index > -1) faultCode = ((String)value).Substring(++index); else faultCode = (String)value; } else if (String.Compare(name, "faultString", true, CultureInfo.InvariantCulture) == 0) faultString = (String)value; else if (String.Compare(name, "faultActor", true, CultureInfo.InvariantCulture) == 0) faultActor = (String)value; else if (String.Compare(name, "detail", true, CultureInfo.InvariantCulture) == 0) detail = value; } } [System.Security.SecurityCritical] // auto-generated_required public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("faultcode", "SOAP-ENV:"+faultCode); info.AddValue("faultstring", faultString); if (faultActor != null) info.AddValue("faultactor", faultActor); info.AddValue("detail", detail, typeof(Object)); } public String FaultCode { get {return faultCode;} set { faultCode = value;} } public String FaultString { get {return faultString;} set { faultString = value;} } public String FaultActor { get {return faultActor;} set { faultActor = value;} } public Object Detail { get {return detail;} set {detail = value;} } } [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class ServerFault { String exceptionType; String message; String stackTrace; Exception exception; internal ServerFault(Exception exception) { this.exception = exception; //this.exceptionType = exception.GetType().AssemblyQualifiedName; //this.message = exception.Message; } public ServerFault(String exceptionType, String message, String stackTrace) { this.exceptionType = exceptionType; this.message = message; this.stackTrace = stackTrace; } public String ExceptionType { get {return exceptionType;} set { exceptionType = value;} } public String ExceptionMessage { get {return message;} set { message = value;} } public String StackTrace { get {return stackTrace;} set {stackTrace = value;} } internal Exception Exception { get {return exception;} } } } #endif // FEATURE_REMOTING // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== /*============================================================ ** ** Class: SoapFault ** **Author: Peter de Jong ([....]) ** ** Purpose: Specifies information for a Soap Fault ** ** Date: June 27, 2000 ** ===========================================================*/ #if FEATURE_REMOTING namespace System.Runtime.Serialization.Formatters { using System; using System.Runtime.Serialization; using System.Runtime.Remoting; using System.Runtime.Remoting.Metadata; using System.Globalization; using System.Security.Permissions; //* Class holds soap fault information [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class SoapFault : ISerializable { String faultCode; String faultString; String faultActor; [SoapField(Embedded=true)] Object detail; public SoapFault() { } public SoapFault(String faultCode, String faultString, String faultActor, ServerFault serverFault) { this.faultCode = faultCode; this.faultString = faultString; this.faultActor = faultActor; this.detail = serverFault; } internal SoapFault(SerializationInfo info, StreamingContext context) { SerializationInfoEnumerator siEnum = info.GetEnumerator(); while(siEnum.MoveNext()) { String name = siEnum.Name; Object value = siEnum.Value; SerTrace.Log(this, "SetObjectData enum ",name," value ",value); if (String.Compare(name, "faultCode", true, CultureInfo.InvariantCulture) == 0) { int index = ((String)value).IndexOf(':'); if (index > -1) faultCode = ((String)value).Substring(++index); else faultCode = (String)value; } else if (String.Compare(name, "faultString", true, CultureInfo.InvariantCulture) == 0) faultString = (String)value; else if (String.Compare(name, "faultActor", true, CultureInfo.InvariantCulture) == 0) faultActor = (String)value; else if (String.Compare(name, "detail", true, CultureInfo.InvariantCulture) == 0) detail = value; } } [System.Security.SecurityCritical] // auto-generated_required public void GetObjectData(SerializationInfo info, StreamingContext context) { info.AddValue("faultcode", "SOAP-ENV:"+faultCode); info.AddValue("faultstring", faultString); if (faultActor != null) info.AddValue("faultactor", faultActor); info.AddValue("detail", detail, typeof(Object)); } public String FaultCode { get {return faultCode;} set { faultCode = value;} } public String FaultString { get {return faultString;} set { faultString = value;} } public String FaultActor { get {return faultActor;} set { faultActor = value;} } public Object Detail { get {return detail;} set {detail = value;} } } [Serializable] [SoapType(Embedded=true)] [System.Runtime.InteropServices.ComVisible(true)] public sealed class ServerFault { String exceptionType; String message; String stackTrace; Exception exception; internal ServerFault(Exception exception) { this.exception = exception; //this.exceptionType = exception.GetType().AssemblyQualifiedName; //this.message = exception.Message; } public ServerFault(String exceptionType, String message, String stackTrace) { this.exceptionType = exceptionType; this.message = message; this.stackTrace = stackTrace; } public String ExceptionType { get {return exceptionType;} set { exceptionType = value;} } public String ExceptionMessage { get {return message;} set { message = value;} } public String StackTrace { get {return stackTrace;} set {stackTrace = value;} } internal Exception Exception { get {return exception;} } } } #endif // FEATURE_REMOTING // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
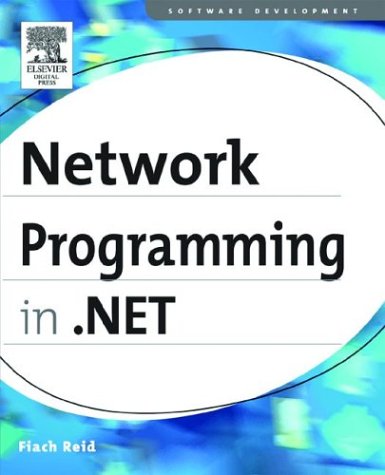
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CTreeGenerator.cs
- EnumConverter.cs
- Utilities.cs
- mediaeventshelper.cs
- FormViewRow.cs
- CodeDomConfigurationHandler.cs
- ComponentEditorForm.cs
- TemplateBuilder.cs
- ToolStripSplitStackLayout.cs
- PageResolution.cs
- StatusBarAutomationPeer.cs
- ValuePattern.cs
- SqlCommand.cs
- IntSecurity.cs
- WebSysDefaultValueAttribute.cs
- ControlAdapter.cs
- ImageButton.cs
- ConditionCollection.cs
- OleDbPermission.cs
- TabPage.cs
- RoutingChannelExtension.cs
- ListViewDeleteEventArgs.cs
- SqlMethodAttribute.cs
- ParserStreamGeometryContext.cs
- TypeToTreeConverter.cs
- ClientSettings.cs
- SchemaImporterExtensionElement.cs
- FreezableOperations.cs
- SymLanguageType.cs
- DataGridHeaderBorder.cs
- ThicknessAnimationBase.cs
- PrtTicket_Editor.cs
- EventLogPermissionAttribute.cs
- PageClientProxyGenerator.cs
- TokenCreationException.cs
- HtmlLinkAdapter.cs
- HasCopySemanticsAttribute.cs
- XamlPoint3DCollectionSerializer.cs
- WrappedIUnknown.cs
- ResourceProviderFactory.cs
- BrowserCapabilitiesCompiler.cs
- SectionInput.cs
- CodePrimitiveExpression.cs
- ExtenderProvidedPropertyAttribute.cs
- ByteStack.cs
- DBConnection.cs
- DesignerSerializationVisibilityAttribute.cs
- dtdvalidator.cs
- NonSerializedAttribute.cs
- ReferentialConstraint.cs
- RotateTransform3D.cs
- UIEndRequest.cs
- X509InitiatorCertificateClientElement.cs
- ListenerElementsCollection.cs
- TabControl.cs
- ReferentialConstraint.cs
- MachinePropertyVariants.cs
- HMACSHA384.cs
- FieldTemplateFactory.cs
- Journal.cs
- GroupItemAutomationPeer.cs
- ModelItemCollectionImpl.cs
- DesignerActionVerbItem.cs
- XmlSchemaFacet.cs
- Mutex.cs
- NotificationContext.cs
- XmlDigitalSignatureProcessor.cs
- WebPartDescriptionCollection.cs
- XmlSchemaSubstitutionGroup.cs
- TextCompositionManager.cs
- GetWinFXPath.cs
- RecognizerStateChangedEventArgs.cs
- CodeArgumentReferenceExpression.cs
- MaskInputRejectedEventArgs.cs
- BinaryMessageFormatter.cs
- ClrProviderManifest.cs
- CodeDelegateCreateExpression.cs
- ThemeDictionaryExtension.cs
- Cursor.cs
- __Error.cs
- RegisteredArrayDeclaration.cs
- CodeTypeReferenceExpression.cs
- ClientScriptManagerWrapper.cs
- ControlLocalizer.cs
- DesignerTextWriter.cs
- AccessText.cs
- InplaceBitmapMetadataWriter.cs
- ConfigurationManagerHelper.cs
- Nullable.cs
- FixedSOMElement.cs
- ChildrenQuery.cs
- FormViewUpdatedEventArgs.cs
- PathFigureCollectionValueSerializer.cs
- DataSysAttribute.cs
- XmlArrayItemAttribute.cs
- CollaborationHelperFunctions.cs
- namescope.cs
- DataGridViewRowHeaderCell.cs
- WindowsNonControl.cs
- ProfileModule.cs