Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / ModelItemCollectionImpl.cs / 1305376 / ModelItemCollectionImpl.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.ComponentModel; using System.Diagnostics; using System.Windows; using System.Windows.Markup; using System.Activities.Presentation.Services; using System.Linq; using System.Runtime; // This class provides the implementation for the ModelItemCollection. This provides // a container for the child modelItems for the entries in a collection. class ModelItemCollectionImpl : ModelItemCollection, IModelTreeItem { ModelProperty contentProperty; object instance; Type itemType; ListmodelItems; Dictionary modelPropertyStore; ModelTreeManager modelTreeManager; ModelProperty nameProperty; List parents; ModelPropertyCollectionImpl properties; List sources; List subTreeNodesThatNeedBackLinkPatching; DependencyObject view; public ModelItemCollectionImpl(ModelTreeManager modelTreeManager, Type itemType, Object instance, ModelItem parent) { Fx.Assert(modelTreeManager != null, "modelTreeManager cannot be null"); Fx.Assert(itemType != null, "item type cannot be null"); Fx.Assert(instance != null, "instance cannot be null"); this.itemType = itemType; this.instance = instance; this.modelTreeManager = modelTreeManager; this.parents = new List (1); this.sources = new List (1); if (parent != null) { this.parents.Add(parent); } this.modelPropertyStore = new Dictionary (); this.subTreeNodesThatNeedBackLinkPatching = new List (); this.modelItems = new List (); UpdateInstance(instance); } public override event NotifyCollectionChangedEventHandler CollectionChanged; public override event PropertyChangedEventHandler PropertyChanged; public override AttributeCollection Attributes { get { Fx.Assert(this.itemType != null, "item type cannot be null"); return TypeDescriptor.GetAttributes(this.itemType); } } public override ModelProperty Content { get { if (this.contentProperty == null) { Fx.Assert(this.instance != null, "instance cannot be null"); ContentPropertyAttribute contentAttribute = TypeDescriptor.GetAttributes(this.instance)[typeof(ContentPropertyAttribute)] as ContentPropertyAttribute; if (contentAttribute != null && !String.IsNullOrEmpty(contentAttribute.Name)) { this.contentProperty = this.Properties.Find(contentAttribute.Name); } } return contentProperty; } } public override int Count { get { Fx.Assert(instance != null, "instance cannot be null"); if (instance != null) { return ((ICollection)instance).Count; } return 0; } } public override bool IsReadOnly { get { IList instanceList = instance as IList; Fx.Assert(instanceList != null, "instance should be IList"); return instanceList.IsReadOnly; } } public override Type ItemType { get { return this.itemType; } } public override string Name { get { string name = null; if ((this.NameProperty != null) && (this.NameProperty.Value != null)) { name = (string)this.NameProperty.Value.GetCurrentValue(); } return name; } set { this.NameProperty.SetValue(value); } } public override ModelItem Parent { get { return (this.Parents.Count() > 0) ? this.Parents.First() : null; } } public override ModelPropertyCollection Properties { get { if (this.properties == null) { properties = new ModelPropertyCollectionImpl(this); } return properties; } } public override ModelItem Root { get { return this.modelTreeManager.Root; } } public override ModelProperty Source { get { return (this.sources.Count > 0) ? this.sources.First() : null; } } public override DependencyObject View { get { return this.view; } } public override IEnumerable Parents { get { return this.parents.Concat( from source in this.sources select source.Parent); } } public override IEnumerable Sources { get { return this.sources; } } internal List Items { get { return modelItems; } } internal Dictionary ModelPropertyStore { get { return modelPropertyStore; } } protected ModelProperty NameProperty { get { if (this.nameProperty == null) { Fx.Assert(this.instance != null, "instance cannot be null"); RuntimeNamePropertyAttribute runtimeNamePropertyAttribute = TypeDescriptor.GetAttributes(this.instance)[typeof(RuntimeNamePropertyAttribute)] as RuntimeNamePropertyAttribute; if (runtimeNamePropertyAttribute != null && !String.IsNullOrEmpty(runtimeNamePropertyAttribute.Name)) { this.nameProperty = this.Properties.Find(runtimeNamePropertyAttribute.Name); } } return nameProperty; } } ModelItem IModelTreeItem.ModelItem { get { return this; } } Dictionary IModelTreeItem.ModelPropertyStore { get { return modelPropertyStore; } } ModelTreeManager IModelTreeItem.ModelTreeManager { get { return modelTreeManager; } } public override ModelItem this[int index] { get { return this.modelItems[index]; } set { this.Insert(index, value); } } public void SetCurrentView(DependencyObject view) { this.view = view; } public override ModelItem Add(object value) { ModelItem item = value as ModelItem; if (item == null) { item = this.modelTreeManager.WrapAsModelItem(this, value); } if (item == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("value")); } Add(item); return item; } public override void Add(ModelItem item) { if (item == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("item")); } this.modelTreeManager.CollectionAdd(this, item); } public override ModelEditingScope BeginEdit(string description) { return this.modelTreeManager.CreateEditingScope(description); } public override ModelEditingScope BeginEdit() { return this.BeginEdit(null); } public override void Clear() { if (!this.IsReadOnly && (this.modelItems.Count > 0)) { this.modelTreeManager.CollectionClear(this); } } public override bool Contains(object value) { ModelItem item = value as ModelItem; if (item == null) { return ((IList)instance).Contains(value); } return Contains(item); } public override bool Contains(ModelItem item) { return this.Items.Contains(item); } public override void CopyTo(ModelItem[] array, int arrayIndex) { if (array == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("array")); } if (this.Items != null) { this.Items.CopyTo(array, arrayIndex); } } public override object GetCurrentValue() { return this.instance; } public override IEnumerator GetEnumerator() { foreach (ModelItem modelItem in modelItems) { yield return modelItem; } } void IModelTreeItem.OnPropertyChanged(string propertyName) { if (PropertyChanged != null) { PropertyChanged(this, new PropertyChangedEventArgs(propertyName)); } } void IModelTreeItem.SetParent(ModelItem dataModelItem) { if (!this.parents.Contains(dataModelItem)) { this.parents.Add(dataModelItem); } } void IModelTreeItem.SetSource(ModelProperty property) { if (!this.sources.Contains(property)) { // also check if the same parent.property is in the list as a different instance of oldModelProperty ModelProperty foundProperty = this.sources.Find((modelProperty) => modelProperty.Name.Equals(property.Name) && property.Parent == modelProperty.Parent); if (foundProperty == null) { this.sources.Add(property); } } } IList IModelTreeItem.SubNodesThatNeedBackLinkUpdate { get { return this.subTreeNodesThatNeedBackLinkPatching; } } public override int IndexOf(ModelItem item) { if (item == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("item")); } return this.modelItems.IndexOf(item); } public override ModelItem Insert(int index, object value) { ModelItem item = value as ModelItem; if (item == null) { item = this.modelTreeManager.WrapAsModelItem(this, value); } if (item == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("value")); } Insert(index, item); return item; } public override void Insert(int index, ModelItem item) { if (item == null) { throw FxTrace.Exception.AsError(new ArgumentNullException("item")); } this.modelTreeManager.CollectionInsert(this, index, item); } public override void Move(int fromIndex, int toIndex) { if (fromIndex > this.Items.Count) { throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException("fromIndex")); } if (toIndex > this.Items.Count) { throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException("toIndex")); } ModelItem movingItem = this.Items[fromIndex]; this.RemoveAt(fromIndex); this.Insert(toIndex, movingItem); } public override bool Remove(object value) { ModelItem item = value as ModelItem; if (item == null) { Fx.Assert(this.Items != null, "Items collection is null when trying to iterate over it"); foreach (ModelItem childItem in this.Items) { if (childItem.GetCurrentValue() == value) { item = childItem; break; } } if (item == null) { return false; } } return Remove(item); } public override bool Remove(ModelItem item) { this.modelTreeManager.CollectionRemove(this, item); return true; } public override void RemoveAt(int index) { if (index >= this.Count) { throw FxTrace.Exception.AsError(new ArgumentOutOfRangeException("index")); } this.modelTreeManager.CollectionRemoveAt(this, index); } internal void AddCore(ModelItem item) { Fx.Assert(instance is IList, "instance should be IList"); Fx.Assert(instance != null, "instance should not be null"); IList instanceList = (IList)instance; instanceList.Add(item.GetCurrentValue()); ((IModelTreeItem)item).SetParent(this); this.modelItems.Add(item); if (this.PropertyChanged != null) { this.PropertyChanged(this, new PropertyChangedEventArgs("Count")); } if (this.CollectionChanged != null) { this.CollectionChanged(this, new System.Collections.Specialized.NotifyCollectionChangedEventArgs(System.Collections.Specialized.NotifyCollectionChangedAction.Add, item, this.Count - 1)); } } internal void ClearCore() { Fx.Assert(instance is IList, " Instance needs to be Ilist for clear to work"); Fx.Assert(instance != null, "Instance should not be null"); this.modelItems.Clear(); IList instanceList = (IList)instance; instanceList.Clear(); if (this.PropertyChanged != null) { this.PropertyChanged(this, new PropertyChangedEventArgs("Count")); } } internal void InsertCore(int index, ModelItem item) { Fx.Assert(instance is IList, "instance needs to be IList"); Fx.Assert(instance != null, "instance should not be null"); IList instanceList = (IList)instance; instanceList.Insert(index, item.GetCurrentValue()); ((IModelTreeItem)item).SetParent(this); this.modelItems.Insert(index, item); if (this.PropertyChanged != null) { this.PropertyChanged(this, new PropertyChangedEventArgs("Count")); } if (this.CollectionChanged != null) { this.CollectionChanged(this, new System.Collections.Specialized.NotifyCollectionChangedEventArgs(System.Collections.Specialized.NotifyCollectionChangedAction.Add, item, index)); } } internal void RemoveCore(ModelItem item) { Fx.Assert(instance is IList, "Instance needs to be IList for remove to work"); Fx.Assert(instance != null, "instance should not be null"); IList instanceList = (IList)instance; int index = instanceList.IndexOf(item.GetCurrentValue()); instanceList.Remove(item.GetCurrentValue()); this.modelItems.Remove(item); if (this.PropertyChanged != null) { this.PropertyChanged(this, new PropertyChangedEventArgs("Count")); } if (this.CollectionChanged != null) { this.CollectionChanged(this, new System.Collections.Specialized.NotifyCollectionChangedEventArgs(System.Collections.Specialized.NotifyCollectionChangedAction.Remove, item, index)); } } internal void RemoveAtCore(int index) { Fx.Assert(instance is IList, "Instance needs to be IList for remove to work"); Fx.Assert(instance != null, "instance should not be null"); IList instanceList = (IList)instance; ModelItem item = this.modelItems[index]; instanceList.RemoveAt(index); this.modelItems.RemoveAt(index); if (this.PropertyChanged != null) { this.PropertyChanged(this, new PropertyChangedEventArgs("Count")); } if (this.CollectionChanged != null) { this.CollectionChanged(this, new System.Collections.Specialized.NotifyCollectionChangedEventArgs(System.Collections.Specialized.NotifyCollectionChangedAction.Remove, item, index)); } } void UpdateInstance(object instance) { this.instance = instance; IEnumerable instanceCollection = this.instance as IEnumerable; if (instanceCollection != null) { foreach (object item in instanceCollection) { ModelItem modelItem = this.modelTreeManager.WrapAsModelItem(this, item); this.modelItems.Add(modelItem); } } } void IModelTreeItem.RemoveParent(ModelItem oldParent) { if (this.parents.Contains(oldParent)) { this.parents.Remove(oldParent); } } void IModelTreeItem.RemoveSource(ModelProperty oldModelProperty) { if (this.sources.Contains(oldModelProperty)) { this.sources.Remove(oldModelProperty); } else { // also check if the same parent.property is in the list as a different instance of oldModelProperty ModelProperty foundProperty = this.sources.Find((modelProperty) => modelProperty.Name.Equals(oldModelProperty.Name) && modelProperty.Parent == oldModelProperty.Parent); if (foundProperty != null) { this.sources.Remove(foundProperty); } else { Fx.Assert("Trying to call RemoveSource() on modelItem that is not parented by oldModelProperty"); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
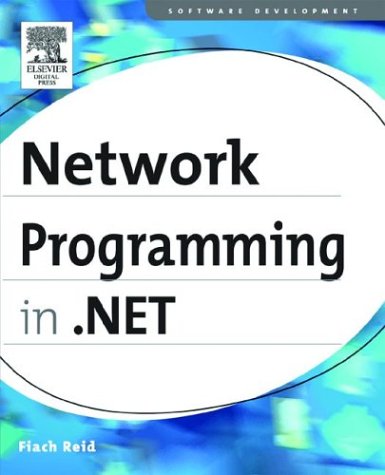
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakReference.cs
- RequiredFieldValidator.cs
- AddInDeploymentState.cs
- MethodImplAttribute.cs
- ListControl.cs
- InputScope.cs
- CheckBoxField.cs
- TemplateXamlTreeBuilder.cs
- WebPartHelpVerb.cs
- HebrewNumber.cs
- HMACSHA256.cs
- TransactionsSectionGroup.cs
- ImageCollectionCodeDomSerializer.cs
- Vertex.cs
- DebugInfoGenerator.cs
- WebPartChrome.cs
- PrinterResolution.cs
- ClaimTypeRequirement.cs
- DecoderBestFitFallback.cs
- SiteMapDataSourceView.cs
- BuildProviderUtils.cs
- IFlowDocumentViewer.cs
- ApplicationInfo.cs
- Vector.cs
- TemplateColumn.cs
- RequestDescription.cs
- XXXInfos.cs
- XmlSerializerAssemblyAttribute.cs
- Match.cs
- BStrWrapper.cs
- DbConnectionStringBuilder.cs
- XmlProcessingInstruction.cs
- _FtpDataStream.cs
- MenuCommandsChangedEventArgs.cs
- XmlRootAttribute.cs
- Clock.cs
- MetadataItem_Static.cs
- NodeFunctions.cs
- IBuiltInEvidence.cs
- AsyncPostBackErrorEventArgs.cs
- OdbcHandle.cs
- UInt16Converter.cs
- SqlTypeConverter.cs
- HttpHeaderCollection.cs
- HierarchicalDataBoundControl.cs
- XmlValidatingReader.cs
- QueryComponents.cs
- Geometry3D.cs
- SimpleType.cs
- Point.cs
- TableDetailsRow.cs
- RegexCharClass.cs
- BindingBase.cs
- updatecommandorderer.cs
- StructuralComparisons.cs
- DataContractSerializer.cs
- LayoutInformation.cs
- ExponentialEase.cs
- RelatedPropertyManager.cs
- SafeLibraryHandle.cs
- shaper.cs
- ClientFormsIdentity.cs
- MaskInputRejectedEventArgs.cs
- CollectionType.cs
- Stylesheet.cs
- SqlDataSourceQueryEditor.cs
- RpcCryptoRequest.cs
- WebControlParameterProxy.cs
- ToolStripItemCollection.cs
- SelectionUIHandler.cs
- VerificationAttribute.cs
- SafeReversePInvokeHandle.cs
- WindowsComboBox.cs
- SapiAttributeParser.cs
- ExceptionUtil.cs
- DebugView.cs
- StrokeCollection2.cs
- QilInvoke.cs
- HttpDictionary.cs
- DbProviderFactory.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- UnmanagedMemoryStreamWrapper.cs
- ITreeGenerator.cs
- DataGridViewLinkCell.cs
- ApplicationActivator.cs
- XmlReflectionImporter.cs
- DocumentViewerBase.cs
- TreeNodeBindingDepthConverter.cs
- StructuralCache.cs
- BookmarkUndoUnit.cs
- BindingEntityInfo.cs
- PassportPrincipal.cs
- ContentValidator.cs
- SqlBooleanizer.cs
- ApplicationServiceHelper.cs
- OleDbWrapper.cs
- AtlasWeb.Designer.cs
- FloaterParaClient.cs
- EventItfInfo.cs
- IPAddress.cs