Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Metadata / Edm / CollectionType.cs / 1305376 / CollectionType.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Data.Common; using System.Text; namespace System.Data.Metadata.Edm { ////// Represents the Edm Collection Type /// public sealed class CollectionType : EdmType { #region Constructors ////// The constructor for constructing a CollectionType object with the element type it contains /// /// The element type that this collection type contains ///Thrown if the argument elementType is null internal CollectionType(EdmType elementType) : this(TypeUsage.Create(elementType)) { this.DataSpace = elementType.DataSpace; } ////// The constructor for constructing a CollectionType object with the element type (as a TypeUsage) it contains /// /// The element type that this collection type contains ///Thrown if the argument elementType is null internal CollectionType(TypeUsage elementType) : base(GetIdentity(EntityUtil.GenericCheckArgumentNull(elementType, "elementType")), EdmConstants.TransientNamespace, elementType.EdmType.DataSpace) { _typeUsage = elementType; SetReadOnly(); } #endregion #region Fields private readonly TypeUsage _typeUsage; #endregion #region Properties ////// Returns the kind of the type /// public override BuiltInTypeKind BuiltInTypeKind { get { return BuiltInTypeKind.CollectionType; } } ////// The type of the element that this collection type contains /// [MetadataProperty(BuiltInTypeKind.TypeUsage, false)] public TypeUsage TypeUsage { get { return _typeUsage; } } #endregion #region Methods ////// Constructs the name of the collection type /// /// The typeusage for the element type that this collection type refers to ///The identity of the resulting collection type private static string GetIdentity(TypeUsage typeUsage) { StringBuilder builder = new StringBuilder(50); builder.Append("collection["); typeUsage.BuildIdentity(builder); builder.Append("]"); return builder.ToString(); } ////// Override EdmEquals to support value comparison of TypeUsage property /// /// ///internal override bool EdmEquals(MetadataItem item) { // short-circuit if this and other are reference equivalent if (Object.ReferenceEquals(this, item)) { return true; } // check type of item if (null == item || BuiltInTypeKind.CollectionType != item.BuiltInTypeKind) { return false; } CollectionType other = (CollectionType)item; // compare type usage return this.TypeUsage.EdmEquals(other.TypeUsage); } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
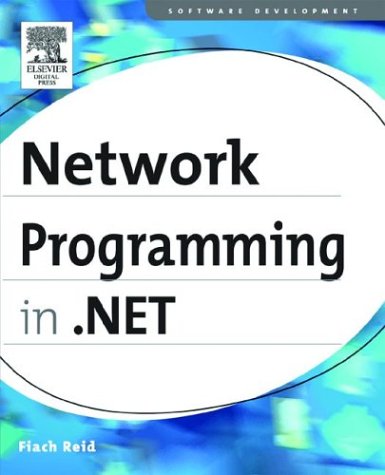
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DropDownButton.cs
- DiscoveryVersion.cs
- SemanticBasicElement.cs
- XomlDesignerLoader.cs
- DataBoundControlHelper.cs
- ApplicationFileParser.cs
- SafeArrayTypeMismatchException.cs
- QueryCacheEntry.cs
- ClientSideQueueItem.cs
- MenuCommand.cs
- TabControl.cs
- ValidationErrorCollection.cs
- Rect3D.cs
- WizardStepBase.cs
- FixedSOMLineCollection.cs
- NullableIntSumAggregationOperator.cs
- MexTcpBindingElement.cs
- SystemIPv6InterfaceProperties.cs
- SqlProviderManifest.cs
- DesignerActionVerbItem.cs
- TreeNodeCollection.cs
- ReservationCollection.cs
- QilName.cs
- CollectionType.cs
- DataControlFieldCollection.cs
- DataServiceQueryProvider.cs
- XmlDownloadManager.cs
- ZipIOExtraFieldZip64Element.cs
- DataGridViewCellStateChangedEventArgs.cs
- MetaTableHelper.cs
- SessionStateModule.cs
- TextEndOfParagraph.cs
- RegexWorker.cs
- WsatTransactionInfo.cs
- QilXmlReader.cs
- ContentElementAutomationPeer.cs
- RoutedEventArgs.cs
- _ProxyChain.cs
- DrawItemEvent.cs
- GeometryConverter.cs
- WebPartTransformerAttribute.cs
- SqlEnums.cs
- PackageProperties.cs
- CodeIdentifiers.cs
- ComponentSerializationService.cs
- DeviceContext2.cs
- OSFeature.cs
- TraceEventCache.cs
- InputLanguageSource.cs
- DataGridViewLinkCell.cs
- HandlerWithFactory.cs
- DBSchemaRow.cs
- SecurityDescriptor.cs
- GiveFeedbackEvent.cs
- SapiInterop.cs
- EventSetter.cs
- ReadonlyMessageFilter.cs
- FunctionMappingTranslator.cs
- CursorEditor.cs
- Authorization.cs
- TokenDescriptor.cs
- ImageKeyConverter.cs
- ProgressBarAutomationPeer.cs
- FormatterConverter.cs
- SHA256Cng.cs
- MarshalByValueComponent.cs
- ErrorActivity.cs
- Roles.cs
- Margins.cs
- AppDomainUnloadedException.cs
- MouseActionValueSerializer.cs
- Partitioner.cs
- PageWrapper.cs
- DocumentSequence.cs
- TlsnegoTokenAuthenticator.cs
- PartialClassGenerationTask.cs
- ADConnectionHelper.cs
- BamlLocalizationDictionary.cs
- MetabaseSettings.cs
- TypeExtensionSerializer.cs
- DescriptionAttribute.cs
- PriorityBindingExpression.cs
- MailAddress.cs
- LinkArea.cs
- WindowsStatusBar.cs
- DbConnectionPoolIdentity.cs
- FocusChangedEventArgs.cs
- UpdateManifestForBrowserApplication.cs
- PropertyDescriptorCollection.cs
- CompiledXpathExpr.cs
- NullReferenceException.cs
- DataService.cs
- StyleBamlTreeBuilder.cs
- HttpModulesSection.cs
- TextMetrics.cs
- BaseValidator.cs
- SqlNotificationEventArgs.cs
- NonVisualControlAttribute.cs
- SecureEnvironment.cs
- ExpressionConverter.cs