Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / clr / src / BCL / System / Security / Policy / UnionCodeGroup.cs / 1305376 / UnionCodeGroup.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // UnionCodeGroup.cs // //[....] // // Representation for code groups used for the policy mechanism // namespace System.Security.Policy { using System; using System.Security.Util; using System.Security; using System.Collections; using System.Diagnostics.Contracts; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [Obsolete("This type is obsolete and will be removed in a future release of the .NET Framework. See http://go.microsoft.com/fwlink/?LinkID=155570 for more information.")] sealed public class UnionCodeGroup : CodeGroup, IUnionSemanticCodeGroup { internal UnionCodeGroup() : base() { } internal UnionCodeGroup( IMembershipCondition membershipCondition, PermissionSet permSet ) : base( membershipCondition, permSet ) { } public UnionCodeGroup( IMembershipCondition membershipCondition, PolicyStatement policy ) : base( membershipCondition, policy ) { } [System.Security.SecuritySafeCritical] // auto-generated public override PolicyStatement Resolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); Contract.EndContractBlock(); object usedEvidence = null; if (PolicyManager.CheckMembershipCondition(MembershipCondition, evidence, out usedEvidence)) { PolicyStatement thisPolicy = PolicyStatement; // PolicyStatement getter makes a copy for us // If any delay-evidence was used to generate this grant set, then we need to keep track of // that for potentially later forcing it to be verified. IDelayEvaluatedEvidence delayEvidence = usedEvidence as IDelayEvaluatedEvidence; bool delayEvidenceNeedsVerification = delayEvidence != null && !delayEvidence.IsVerified; if (delayEvidenceNeedsVerification) { thisPolicy.AddDependentEvidence(delayEvidence); } bool foundExclusiveChild = false; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext() && !foundExclusiveChild) { PolicyStatement childPolicy = PolicyManager.ResolveCodeGroup(enumerator.Current as CodeGroup, evidence); if (childPolicy != null) { thisPolicy.InplaceUnion(childPolicy); if ((childPolicy.Attributes & PolicyStatementAttribute.Exclusive) == PolicyStatementAttribute.Exclusive) { foundExclusiveChild = true; } } } return thisPolicy; } else { return null; } } ///PolicyStatement IUnionSemanticCodeGroup.InternalResolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); Contract.EndContractBlock(); if (this.MembershipCondition.Check( evidence )) { return this.PolicyStatement; } else { return null; } } [System.Security.SecuritySafeCritical] // auto-generated public override CodeGroup ResolveMatchingCodeGroups( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); Contract.EndContractBlock(); if (this.MembershipCondition.Check( evidence )) { CodeGroup retGroup = this.Copy(); retGroup.Children = new ArrayList(); IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { CodeGroup matchingGroups = ((CodeGroup)enumerator.Current).ResolveMatchingCodeGroups( evidence ); // If the child has a policy, we are done. if (matchingGroups != null) { retGroup.AddChild( matchingGroups ); } } return retGroup; } else { return null; } } [System.Security.SecuritySafeCritical] // auto-generated public override CodeGroup Copy() { UnionCodeGroup group = new UnionCodeGroup(); group.MembershipCondition = this.MembershipCondition; group.PolicyStatement = this.PolicyStatement; group.Name = this.Name; group.Description = this.Description; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { group.AddChild( (CodeGroup)enumerator.Current ); } return group; } public override String MergeLogic { get { return Environment.GetResourceString( "MergeLogic_Union" ); } } internal override String GetTypeName() { return "System.Security.Policy.UnionCodeGroup"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // UnionCodeGroup.cs // // [....] // // Representation for code groups used for the policy mechanism // namespace System.Security.Policy { using System; using System.Security.Util; using System.Security; using System.Collections; using System.Diagnostics.Contracts; [Serializable] [System.Runtime.InteropServices.ComVisible(true)] [Obsolete("This type is obsolete and will be removed in a future release of the .NET Framework. See http://go.microsoft.com/fwlink/?LinkID=155570 for more information.")] sealed public class UnionCodeGroup : CodeGroup, IUnionSemanticCodeGroup { internal UnionCodeGroup() : base() { } internal UnionCodeGroup( IMembershipCondition membershipCondition, PermissionSet permSet ) : base( membershipCondition, permSet ) { } public UnionCodeGroup( IMembershipCondition membershipCondition, PolicyStatement policy ) : base( membershipCondition, policy ) { } [System.Security.SecuritySafeCritical] // auto-generated public override PolicyStatement Resolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); Contract.EndContractBlock(); object usedEvidence = null; if (PolicyManager.CheckMembershipCondition(MembershipCondition, evidence, out usedEvidence)) { PolicyStatement thisPolicy = PolicyStatement; // PolicyStatement getter makes a copy for us // If any delay-evidence was used to generate this grant set, then we need to keep track of // that for potentially later forcing it to be verified. IDelayEvaluatedEvidence delayEvidence = usedEvidence as IDelayEvaluatedEvidence; bool delayEvidenceNeedsVerification = delayEvidence != null && !delayEvidence.IsVerified; if (delayEvidenceNeedsVerification) { thisPolicy.AddDependentEvidence(delayEvidence); } bool foundExclusiveChild = false; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext() && !foundExclusiveChild) { PolicyStatement childPolicy = PolicyManager.ResolveCodeGroup(enumerator.Current as CodeGroup, evidence); if (childPolicy != null) { thisPolicy.InplaceUnion(childPolicy); if ((childPolicy.Attributes & PolicyStatementAttribute.Exclusive) == PolicyStatementAttribute.Exclusive) { foundExclusiveChild = true; } } } return thisPolicy; } else { return null; } } ///PolicyStatement IUnionSemanticCodeGroup.InternalResolve( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); Contract.EndContractBlock(); if (this.MembershipCondition.Check( evidence )) { return this.PolicyStatement; } else { return null; } } [System.Security.SecuritySafeCritical] // auto-generated public override CodeGroup ResolveMatchingCodeGroups( Evidence evidence ) { if (evidence == null) throw new ArgumentNullException("evidence"); Contract.EndContractBlock(); if (this.MembershipCondition.Check( evidence )) { CodeGroup retGroup = this.Copy(); retGroup.Children = new ArrayList(); IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { CodeGroup matchingGroups = ((CodeGroup)enumerator.Current).ResolveMatchingCodeGroups( evidence ); // If the child has a policy, we are done. if (matchingGroups != null) { retGroup.AddChild( matchingGroups ); } } return retGroup; } else { return null; } } [System.Security.SecuritySafeCritical] // auto-generated public override CodeGroup Copy() { UnionCodeGroup group = new UnionCodeGroup(); group.MembershipCondition = this.MembershipCondition; group.PolicyStatement = this.PolicyStatement; group.Name = this.Name; group.Description = this.Description; IEnumerator enumerator = this.Children.GetEnumerator(); while (enumerator.MoveNext()) { group.AddChild( (CodeGroup)enumerator.Current ); } return group; } public override String MergeLogic { get { return Environment.GetResourceString( "MergeLogic_Union" ); } } internal override String GetTypeName() { return "System.Security.Policy.UnionCodeGroup"; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
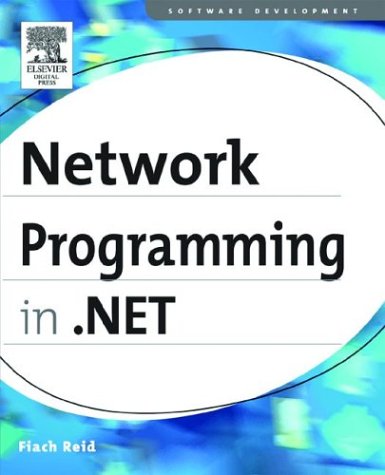
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LookupNode.cs
- BindingMemberInfo.cs
- DataGridViewAutoSizeModeEventArgs.cs
- RightsManagementEncryptedStream.cs
- DataRecordInternal.cs
- Debug.cs
- Constants.cs
- BevelBitmapEffect.cs
- DetailsView.cs
- DataGridViewLinkColumn.cs
- CompositeActivityTypeDescriptor.cs
- DES.cs
- StringAnimationUsingKeyFrames.cs
- DesignTimeData.cs
- SafeFileMappingHandle.cs
- SpotLight.cs
- EdmType.cs
- HttpConfigurationSystem.cs
- Socket.cs
- ObjectStateEntryBaseUpdatableDataRecord.cs
- CaseCqlBlock.cs
- SqlConnection.cs
- GeometryModel3D.cs
- OdbcCommandBuilder.cs
- SafeNativeMethods.cs
- StorageFunctionMapping.cs
- Stackframe.cs
- AxisAngleRotation3D.cs
- HostProtectionException.cs
- CalendarSelectionChangedEventArgs.cs
- ClientBuildManager.cs
- XPathNavigatorKeyComparer.cs
- LongValidatorAttribute.cs
- TreeViewAutomationPeer.cs
- FreezableOperations.cs
- CheckedPointers.cs
- DataGridViewHitTestInfo.cs
- GridViewDeletedEventArgs.cs
- recordstatescratchpad.cs
- PkcsUtils.cs
- SerializationFieldInfo.cs
- MetadataImporterQuotas.cs
- DataBoundControlAdapter.cs
- WindowsListViewScroll.cs
- InheritablePropertyChangeInfo.cs
- Byte.cs
- TrackingProfileDeserializationException.cs
- HostSecurityManager.cs
- ItemsControl.cs
- Exceptions.cs
- selecteditemcollection.cs
- XPathNavigator.cs
- NativeDirectoryServicesQueryAPIs.cs
- Tracking.cs
- HeaderedContentControl.cs
- MarginCollapsingState.cs
- DebugController.cs
- StrokeNodeData.cs
- TimeManager.cs
- SafeNativeMethodsOther.cs
- DataTable.cs
- WebServiceHandlerFactory.cs
- BevelBitmapEffect.cs
- XamlPointCollectionSerializer.cs
- CorrelationToken.cs
- FixedSOMPageConstructor.cs
- FixedSOMTable.cs
- CapabilitiesUse.cs
- ErrorHandler.cs
- BezierSegment.cs
- PermissionRequestEvidence.cs
- ContextProperty.cs
- BaseResourcesBuildProvider.cs
- InputDevice.cs
- SerializationException.cs
- SoapSchemaImporter.cs
- TraceEventCache.cs
- RelatedPropertyManager.cs
- JoinGraph.cs
- EventListenerClientSide.cs
- Propagator.JoinPropagator.JoinPredicateVisitor.cs
- x509utils.cs
- HierarchicalDataSourceControl.cs
- SQLBoolean.cs
- ConstructorNeedsTagAttribute.cs
- ListViewDesigner.cs
- ImageSource.cs
- CLSCompliantAttribute.cs
- ProcessStartInfo.cs
- infer.cs
- _CommandStream.cs
- TableRow.cs
- BitArray.cs
- NaturalLanguageHyphenator.cs
- BuildResultCache.cs
- WindowsPrincipal.cs
- ItemsChangedEventArgs.cs
- oledbconnectionstring.cs
- DelayLoadType.cs
- ButtonBaseAutomationPeer.cs