Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / HatchBrush.cs / 1305376 / HatchBrush.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Drawing.Internal; using System.Runtime.Versioning; /** * Represent a HatchBrush brush object */ ////// /// Defines a rectangular brush with a hatch /// style, a foreground color, and a background color. /// public sealed class HatchBrush : Brush { /** * Create a new hatch brush object */ ////// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public HatchBrush(HatchStyle hatchstyle, Color foreColor) : this(hatchstyle, foreColor, Color.FromArgb( (int) unchecked( (int) 0xff000000) ) ) { } ////// Initializes a new instance of the ///class with the specified and foreground color. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public HatchBrush(HatchStyle hatchstyle, Color foreColor, Color backColor) { IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateHatchBrush((int) hatchstyle, foreColor.ToArgb(), backColor.ToArgb(), out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Initializes a new instance of the ///class with the specified , /// foreground color, and background color. /// /// Constructor to initialize this object from a GDI+ native reference. /// internal HatchBrush(IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrushInternal( nativeBrush ); } ////// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new HatchBrush(cloneBrush); } /** * Get hatch brush object attributes */ ///. /// /// /// Gets the hatch style of this public HatchStyle HatchStyle { get { int hatchStyle = 0; int status = SafeNativeMethods.Gdip.GdipGetHatchStyle(new HandleRef(this, this.NativeBrush), out hatchStyle); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return (HatchStyle) hatchStyle; } } ///. /// /// /// Gets the color of hatch lines drawn by this /// public Color ForegroundColor { get { int forecol; int status = SafeNativeMethods.Gdip.GdipGetHatchForegroundColor(new HandleRef(this, this.NativeBrush), out forecol); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return Color.FromArgb(forecol); } } ///. /// /// /// public Color BackgroundColor { get { int backcol; int status = SafeNativeMethods.Gdip.GdipGetHatchBackgroundColor(new HandleRef(this, this.NativeBrush), out backcol); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return Color.FromArgb(backcol); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Gets the color of spaces between the hatch /// lines drawn by this ///. /// // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Drawing2D { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.Drawing; using System.ComponentModel; using Microsoft.Win32; using System.Drawing.Internal; using System.Runtime.Versioning; /** * Represent a HatchBrush brush object */ ////// /// Defines a rectangular brush with a hatch /// style, a foreground color, and a background color. /// public sealed class HatchBrush : Brush { /** * Create a new hatch brush object */ ////// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public HatchBrush(HatchStyle hatchstyle, Color foreColor) : this(hatchstyle, foreColor, Color.FromArgb( (int) unchecked( (int) 0xff000000) ) ) { } ////// Initializes a new instance of the ///class with the specified and foreground color. /// /// /// [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public HatchBrush(HatchStyle hatchstyle, Color foreColor, Color backColor) { IntPtr brush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCreateHatchBrush((int) hatchstyle, foreColor.ToArgb(), backColor.ToArgb(), out brush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); SetNativeBrushInternal(brush); } ////// Initializes a new instance of the ///class with the specified , /// foreground color, and background color. /// /// Constructor to initialize this object from a GDI+ native reference. /// internal HatchBrush(IntPtr nativeBrush ) { Debug.Assert( nativeBrush != IntPtr.Zero, "Initializing native brush with null." ); SetNativeBrushInternal( nativeBrush ); } ////// /// Creates an exact copy of this [ResourceExposure(ResourceScope.Process)] [ResourceConsumption(ResourceScope.Process)] public override object Clone() { IntPtr cloneBrush = IntPtr.Zero; int status = SafeNativeMethods.Gdip.GdipCloneBrush(new HandleRef(this, this.NativeBrush), out cloneBrush); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return new HatchBrush(cloneBrush); } /** * Get hatch brush object attributes */ ///. /// /// /// Gets the hatch style of this public HatchStyle HatchStyle { get { int hatchStyle = 0; int status = SafeNativeMethods.Gdip.GdipGetHatchStyle(new HandleRef(this, this.NativeBrush), out hatchStyle); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return (HatchStyle) hatchStyle; } } ///. /// /// /// Gets the color of hatch lines drawn by this /// public Color ForegroundColor { get { int forecol; int status = SafeNativeMethods.Gdip.GdipGetHatchForegroundColor(new HandleRef(this, this.NativeBrush), out forecol); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return Color.FromArgb(forecol); } } ///. /// /// /// public Color BackgroundColor { get { int backcol; int status = SafeNativeMethods.Gdip.GdipGetHatchBackgroundColor(new HandleRef(this, this.NativeBrush), out backcol); if (status != SafeNativeMethods.Gdip.Ok) throw SafeNativeMethods.Gdip.StatusException(status); return Color.FromArgb(backcol); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Gets the color of spaces between the hatch /// lines drawn by this ///. ///
Link Menu
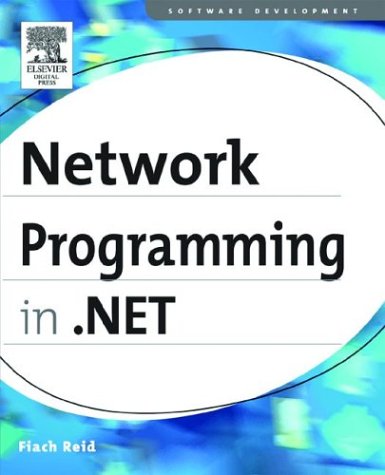
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UpdateRecord.cs
- CodeCastExpression.cs
- AnnotationResourceChangedEventArgs.cs
- FontUnitConverter.cs
- UriWriter.cs
- SerializationSectionGroup.cs
- RectangleGeometry.cs
- FontDifferentiator.cs
- QueryFunctions.cs
- RequestTimeoutManager.cs
- AccessorTable.cs
- FontConverter.cs
- FormsAuthentication.cs
- IteratorFilter.cs
- BindingSource.cs
- ZoneLinkButton.cs
- MessageBox.cs
- FaultCallbackWrapper.cs
- TableLayoutSettings.cs
- DataRowView.cs
- RegisteredHiddenField.cs
- Interlocked.cs
- RepeaterDesigner.cs
- _PooledStream.cs
- Accessible.cs
- SmiRequestExecutor.cs
- MethodExpr.cs
- Missing.cs
- LinearGradientBrush.cs
- LogExtentCollection.cs
- DecimalKeyFrameCollection.cs
- ResourceDescriptionAttribute.cs
- SourceInterpreter.cs
- WebControlParameterProxy.cs
- BuildProvider.cs
- ArgumentsParser.cs
- CustomAttributeBuilder.cs
- WorkflowServiceNamespace.cs
- ParentUndoUnit.cs
- WebPartMinimizeVerb.cs
- FormViewUpdateEventArgs.cs
- SeverityFilter.cs
- ConnectionProviderAttribute.cs
- XmlSchemaSequence.cs
- UntrustedRecipientException.cs
- _LoggingObject.cs
- DeobfuscatingStream.cs
- odbcmetadatacolumnnames.cs
- ViewGenResults.cs
- HttpCacheVaryByContentEncodings.cs
- SignerInfo.cs
- WsrmFault.cs
- DefaultEventAttribute.cs
- TableColumn.cs
- TypeLibConverter.cs
- SQLUtility.cs
- CompoundFileIOPermission.cs
- HybridDictionary.cs
- RegexRunner.cs
- WindowClosedEventArgs.cs
- xsdvalidator.cs
- _PooledStream.cs
- WindowsAuthenticationEventArgs.cs
- GroupBoxRenderer.cs
- ScriptComponentDescriptor.cs
- WebPartEditorOkVerb.cs
- PkcsUtils.cs
- SimpleRecyclingCache.cs
- SiteMapNode.cs
- LockCookie.cs
- SystemInformation.cs
- PersonalizationStateInfo.cs
- InstanceContext.cs
- IgnorePropertiesAttribute.cs
- ConfigurationException.cs
- DESCryptoServiceProvider.cs
- QilDataSource.cs
- ShapeTypeface.cs
- ComplexBindingPropertiesAttribute.cs
- DoubleCollectionValueSerializer.cs
- ItemCollection.cs
- ToolStripPanelDesigner.cs
- XmlSerializerNamespaces.cs
- BackStopAuthenticationModule.cs
- ToolStripRenderEventArgs.cs
- GridViewUpdateEventArgs.cs
- DependencyPropertyConverter.cs
- SessionStateSection.cs
- TextProviderWrapper.cs
- SourceLocation.cs
- ListItemCollection.cs
- SourceLineInfo.cs
- IApplicationTrustManager.cs
- SafeNativeMethodsOther.cs
- CodeDefaultValueExpression.cs
- AuthorizationSection.cs
- HttpRequestCacheValidator.cs
- GlyphRunDrawing.cs
- TabControl.cs
- KeyPullup.cs