Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CompMod / System / ComponentModel / Design / Serialization / InstanceDescriptor.cs / 1305376 / InstanceDescriptor.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Security.Permissions; ////// EventArgs for the ResolveNameEventHandler. This event is used /// by the serialization process to match a name to an object /// instance. /// [HostProtection(SharedState = true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class InstanceDescriptor { private MemberInfo member; private ICollection arguments; private bool isComplete; ////// Creates a new InstanceDescriptor. /// public InstanceDescriptor(MemberInfo member, ICollection arguments) : this(member, arguments, true) { } ////// Creates a new InstanceDescriptor. /// public InstanceDescriptor(MemberInfo member, ICollection arguments, bool isComplete) { this.member = member; this.isComplete = isComplete; if (arguments == null) { this.arguments = new object[0]; } else { object[] args = new object[arguments.Count]; arguments.CopyTo(args, 0); this.arguments = args; } if (member is FieldInfo) { FieldInfo fi = (FieldInfo)member; if (!fi.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeStatic)); } if (this.arguments.Count != 0) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorLengthMismatch)); } } else if (member is ConstructorInfo) { ConstructorInfo ci = (ConstructorInfo)member; if (ci.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorCannotBeStatic)); } if (this.arguments.Count != ci.GetParameters().Length) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorLengthMismatch)); } } else if (member is MethodInfo) { MethodInfo mi = (MethodInfo)member; if (!mi.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeStatic)); } if (this.arguments.Count != mi.GetParameters().Length) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorLengthMismatch)); } } else if (member is PropertyInfo) { PropertyInfo pi = (PropertyInfo)member; if (!pi.CanRead) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeReadable)); } MethodInfo mi = pi.GetGetMethod(); if (mi != null && !mi.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeStatic)); } } } ////// The collection of arguments that should be passed to /// MemberInfo in order to create an instance. /// public ICollection Arguments { get { return arguments; } } ////// Determines if the contents of this instance descriptor completely identify the instance. /// This will normally be the case, but some objects may be too complex for a single method /// or constructor to represent. IsComplete can be used to identify these objects and take /// additional steps to further describe their state. /// public bool IsComplete { get { return isComplete; } } ////// The MemberInfo object that was passed into the constructor /// of this InstanceDescriptor. /// public MemberInfo MemberInfo { get { return member; } } ////// Invokes this instance descriptor, returning the object /// the descriptor describes. /// public object Invoke() { object[] translatedArguments = new object[arguments.Count]; arguments.CopyTo(translatedArguments, 0); // Instance descriptors can contain other instance // descriptors. Translate them if necessary. // for(int i = 0; i < translatedArguments.Length; i++) { if (translatedArguments[i] is InstanceDescriptor) { translatedArguments[i] = ((InstanceDescriptor)translatedArguments[i]).Invoke(); } } if (member is ConstructorInfo) { return ((ConstructorInfo)member).Invoke(translatedArguments); } else if (member is MethodInfo) { return ((MethodInfo)member).Invoke(null, translatedArguments); } else if (member is PropertyInfo) { return ((PropertyInfo)member).GetValue(null, translatedArguments); } else if (member is FieldInfo) { return ((FieldInfo)member).GetValue(null); } else { Debug.Fail("Unrecognized reflection type in instance descriptor: " + member.GetType().Name); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.ComponentModel.Design.Serialization { using System; using System.Collections; using System.Diagnostics; using System.Reflection; using System.Security.Permissions; ////// EventArgs for the ResolveNameEventHandler. This event is used /// by the serialization process to match a name to an object /// instance. /// [HostProtection(SharedState = true)] [System.Security.Permissions.PermissionSetAttribute(System.Security.Permissions.SecurityAction.LinkDemand, Name = "FullTrust")] public sealed class InstanceDescriptor { private MemberInfo member; private ICollection arguments; private bool isComplete; ////// Creates a new InstanceDescriptor. /// public InstanceDescriptor(MemberInfo member, ICollection arguments) : this(member, arguments, true) { } ////// Creates a new InstanceDescriptor. /// public InstanceDescriptor(MemberInfo member, ICollection arguments, bool isComplete) { this.member = member; this.isComplete = isComplete; if (arguments == null) { this.arguments = new object[0]; } else { object[] args = new object[arguments.Count]; arguments.CopyTo(args, 0); this.arguments = args; } if (member is FieldInfo) { FieldInfo fi = (FieldInfo)member; if (!fi.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeStatic)); } if (this.arguments.Count != 0) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorLengthMismatch)); } } else if (member is ConstructorInfo) { ConstructorInfo ci = (ConstructorInfo)member; if (ci.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorCannotBeStatic)); } if (this.arguments.Count != ci.GetParameters().Length) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorLengthMismatch)); } } else if (member is MethodInfo) { MethodInfo mi = (MethodInfo)member; if (!mi.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeStatic)); } if (this.arguments.Count != mi.GetParameters().Length) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorLengthMismatch)); } } else if (member is PropertyInfo) { PropertyInfo pi = (PropertyInfo)member; if (!pi.CanRead) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeReadable)); } MethodInfo mi = pi.GetGetMethod(); if (mi != null && !mi.IsStatic) { throw new ArgumentException(SR.GetString(SR.InstanceDescriptorMustBeStatic)); } } } ////// The collection of arguments that should be passed to /// MemberInfo in order to create an instance. /// public ICollection Arguments { get { return arguments; } } ////// Determines if the contents of this instance descriptor completely identify the instance. /// This will normally be the case, but some objects may be too complex for a single method /// or constructor to represent. IsComplete can be used to identify these objects and take /// additional steps to further describe their state. /// public bool IsComplete { get { return isComplete; } } ////// The MemberInfo object that was passed into the constructor /// of this InstanceDescriptor. /// public MemberInfo MemberInfo { get { return member; } } ////// Invokes this instance descriptor, returning the object /// the descriptor describes. /// public object Invoke() { object[] translatedArguments = new object[arguments.Count]; arguments.CopyTo(translatedArguments, 0); // Instance descriptors can contain other instance // descriptors. Translate them if necessary. // for(int i = 0; i < translatedArguments.Length; i++) { if (translatedArguments[i] is InstanceDescriptor) { translatedArguments[i] = ((InstanceDescriptor)translatedArguments[i]).Invoke(); } } if (member is ConstructorInfo) { return ((ConstructorInfo)member).Invoke(translatedArguments); } else if (member is MethodInfo) { return ((MethodInfo)member).Invoke(null, translatedArguments); } else if (member is PropertyInfo) { return ((PropertyInfo)member).GetValue(null, translatedArguments); } else if (member is FieldInfo) { return ((FieldInfo)member).GetValue(null); } else { Debug.Fail("Unrecognized reflection type in instance descriptor: " + member.GetType().Name); } return null; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
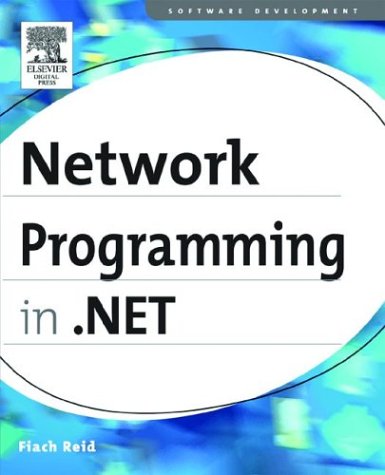
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeConstructor.cs
- ViewCellSlot.cs
- BuilderInfo.cs
- SqlCommand.cs
- SafeProcessHandle.cs
- MaskDesignerDialog.cs
- Thread.cs
- LogoValidationException.cs
- MetadataFile.cs
- DbModificationClause.cs
- InvokePatternIdentifiers.cs
- DummyDataSource.cs
- ReadOnlyCollection.cs
- DataGridViewDataConnection.cs
- CommunicationException.cs
- ScrollBarRenderer.cs
- ReflectionPermission.cs
- StateElement.cs
- FastEncoderWindow.cs
- ColumnHeader.cs
- HttpCachePolicy.cs
- AccessText.cs
- MethodExpression.cs
- NamespaceEmitter.cs
- BitmapImage.cs
- TemplateComponentConnector.cs
- FrameworkReadOnlyPropertyMetadata.cs
- ProfileModule.cs
- DataObject.cs
- MetaType.cs
- SecurityException.cs
- SecurityVersion.cs
- HierarchicalDataSourceConverter.cs
- XmlILIndex.cs
- WeakHashtable.cs
- KeyGestureValueSerializer.cs
- ReadOnlyObservableCollection.cs
- TemplateXamlParser.cs
- BamlLocalizableResource.cs
- VirtualDirectoryMapping.cs
- XmlQueryTypeFactory.cs
- FileRecordSequence.cs
- SqlFormatter.cs
- EventItfInfo.cs
- tooltip.cs
- XmlReflectionImporter.cs
- ValueQuery.cs
- SpeechSynthesizer.cs
- XPathExpr.cs
- OdbcTransaction.cs
- ProtocolElementCollection.cs
- XmlSchemaInfo.cs
- DetailsViewUpdatedEventArgs.cs
- SqlDataRecord.cs
- ApplicationBuildProvider.cs
- StorageComplexPropertyMapping.cs
- TextContainerHelper.cs
- LoginCancelEventArgs.cs
- SrgsToken.cs
- SqlBinder.cs
- StaticResourceExtension.cs
- TreeIterators.cs
- ToolStripContentPanel.cs
- FormatConvertedBitmap.cs
- CompiledQueryCacheEntry.cs
- DataGridViewSortCompareEventArgs.cs
- XamlPointCollectionSerializer.cs
- ProtectedConfiguration.cs
- RepeatInfo.cs
- TreeViewItem.cs
- AbstractExpressions.cs
- ListViewInsertedEventArgs.cs
- ServiceNameCollection.cs
- XmlSerializerAssemblyAttribute.cs
- ImpersonationContext.cs
- FilterQuery.cs
- CryptoStream.cs
- CommandField.cs
- _HeaderInfoTable.cs
- ThousandthOfEmRealDoubles.cs
- WmpBitmapEncoder.cs
- COAUTHIDENTITY.cs
- Renderer.cs
- AlphabeticalEnumConverter.cs
- ReturnEventArgs.cs
- NetworkInformationPermission.cs
- BindingList.cs
- IDataContractSurrogate.cs
- PolicyException.cs
- AppDomainUnloadedException.cs
- LongTypeConverter.cs
- DisplayInformation.cs
- Simplifier.cs
- XmlSchemaImporter.cs
- HttpModulesSection.cs
- MenuAdapter.cs
- InvalidPrinterException.cs
- wgx_sdk_version.cs
- DESCryptoServiceProvider.cs
- Label.cs