Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Linq / Parallel / Scheduling / CancellationState.cs / 1305376 / CancellationState.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // CancellationState.cs // //[....] // // A bag of cancellation-related items that are passed around as a group. // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System; using System.Collections.Generic; using System.Threading; namespace System.Linq.Parallel { internal class CancellationState { // a cancellation signal that can be set internally to prompt early query termination. internal CancellationTokenSource InternalCancellationTokenSource; // the external cancellationToken that the user sets to ask for the query to terminate early. // this has to be tracked explicitly so that an OCE(externalToken) can be thrown as the query // execution unravels. internal CancellationToken ExternalCancellationToken; // A combined token Source for internal/external cancellation, defining the total cancellation state. internal CancellationTokenSource MergedCancellationTokenSource; // A combined token for internal/external cancellation, defining the total cancellation state. internal CancellationToken MergedCancellationToken { get { if( MergedCancellationTokenSource != null) return MergedCancellationTokenSource.Token; else return new CancellationToken(false); } } // A shared boolean flag to track whether a query-opening-enumerator dispose has occured. internal SharedTopLevelDisposedFlag; internal CancellationState(CancellationToken externalCancellationToken) { ExternalCancellationToken = externalCancellationToken; TopLevelDisposedFlag = new Shared (false); //it would always be initialised to false, so no harm doing it here and avoid #if around constructors. } /// /// Poll frequency (number of loops per cancellation check) for situations where per-1-loop testing is too high an overhead. /// internal const int POLL_INTERVAL = 63; //must be of the form (2^n)-1. // The two main situations requiring POLL_INTERVAL are: // 1. inner loops of sorting/merging operations // 2. tight loops that perform very little work per MoveNext call. // Testing has shown both situations have similar requirements and can share the same constant for polling interval. // // Because the poll checks are per-N loops, if there are delays in user code, they may affect cancellation timeliness. // Guidance is that all user-delegates should perform cancellation checks at least every 1ms. // // Inner loop code should poll once per n loop, typically via: // if ((i++ & CancellationState.POLL_INTERVAL) == 0) // CancellationState.ThrowIfCanceled(m_cancellationToken); // (Note, this only behaves as expected if FREQ is of the form (2^n)-1 ////// Throws an OCE if the merged token has been canceled. /// /// A token to check for cancelation. internal static void ThrowIfCanceled(CancellationToken token) { if (token.IsCancellationRequested) throw new OperationCanceledException(token); } // Test if external cancellation was requested and occured, and if so throw a standardize OCE with standardized message internal static void ThrowWithStandardMessageIfCanceled(CancellationToken externalCancellationToken) { if (externalCancellationToken.IsCancellationRequested) { string oceMessage = SR.GetString(SR.PLINQ_ExternalCancellationRequested); throw new OperationCanceledException(oceMessage, externalCancellationToken); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== // =+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+=+ // // CancellationState.cs // //[....] // // A bag of cancellation-related items that are passed around as a group. // // =-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=-=- using System; using System.Collections.Generic; using System.Threading; namespace System.Linq.Parallel { internal class CancellationState { // a cancellation signal that can be set internally to prompt early query termination. internal CancellationTokenSource InternalCancellationTokenSource; // the external cancellationToken that the user sets to ask for the query to terminate early. // this has to be tracked explicitly so that an OCE(externalToken) can be thrown as the query // execution unravels. internal CancellationToken ExternalCancellationToken; // A combined token Source for internal/external cancellation, defining the total cancellation state. internal CancellationTokenSource MergedCancellationTokenSource; // A combined token for internal/external cancellation, defining the total cancellation state. internal CancellationToken MergedCancellationToken { get { if( MergedCancellationTokenSource != null) return MergedCancellationTokenSource.Token; else return new CancellationToken(false); } } // A shared boolean flag to track whether a query-opening-enumerator dispose has occured. internal SharedTopLevelDisposedFlag; internal CancellationState(CancellationToken externalCancellationToken) { ExternalCancellationToken = externalCancellationToken; TopLevelDisposedFlag = new Shared (false); //it would always be initialised to false, so no harm doing it here and avoid #if around constructors. } /// /// Poll frequency (number of loops per cancellation check) for situations where per-1-loop testing is too high an overhead. /// internal const int POLL_INTERVAL = 63; //must be of the form (2^n)-1. // The two main situations requiring POLL_INTERVAL are: // 1. inner loops of sorting/merging operations // 2. tight loops that perform very little work per MoveNext call. // Testing has shown both situations have similar requirements and can share the same constant for polling interval. // // Because the poll checks are per-N loops, if there are delays in user code, they may affect cancellation timeliness. // Guidance is that all user-delegates should perform cancellation checks at least every 1ms. // // Inner loop code should poll once per n loop, typically via: // if ((i++ & CancellationState.POLL_INTERVAL) == 0) // CancellationState.ThrowIfCanceled(m_cancellationToken); // (Note, this only behaves as expected if FREQ is of the form (2^n)-1 ////// Throws an OCE if the merged token has been canceled. /// /// A token to check for cancelation. internal static void ThrowIfCanceled(CancellationToken token) { if (token.IsCancellationRequested) throw new OperationCanceledException(token); } // Test if external cancellation was requested and occured, and if so throw a standardize OCE with standardized message internal static void ThrowWithStandardMessageIfCanceled(CancellationToken externalCancellationToken) { if (externalCancellationToken.IsCancellationRequested) { string oceMessage = SR.GetString(SR.PLINQ_ExternalCancellationRequested); throw new OperationCanceledException(oceMessage, externalCancellationToken); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
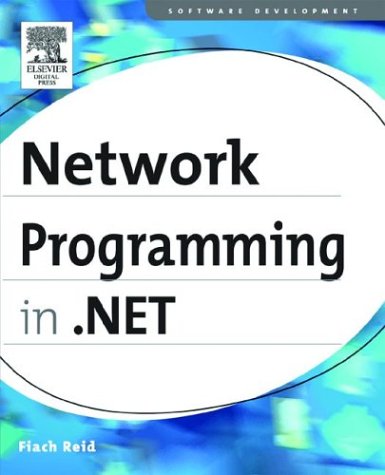
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FragmentQueryProcessor.cs
- ToolStripItemBehavior.cs
- Roles.cs
- PerspectiveCamera.cs
- XslCompiledTransform.cs
- RSACryptoServiceProvider.cs
- RedistVersionInfo.cs
- XmlBuffer.cs
- AnnotationMap.cs
- CatalogZone.cs
- FixedSOMTableRow.cs
- SmiContextFactory.cs
- ImageDrawing.cs
- RegistrationServices.cs
- QilStrConcat.cs
- DataBindingCollection.cs
- BaseUriHelper.cs
- FormViewUpdateEventArgs.cs
- HandlerFactoryWrapper.cs
- ModelTreeEnumerator.cs
- BorderGapMaskConverter.cs
- SqlCharStream.cs
- ValueProviderWrapper.cs
- SqlXml.cs
- NamespaceMapping.cs
- StrongTypingException.cs
- DataBoundControlDesigner.cs
- DetailsViewInsertedEventArgs.cs
- PointConverter.cs
- PathSegment.cs
- TransformerInfo.cs
- XmlWriterSettings.cs
- RegexRunner.cs
- ImageAnimator.cs
- MetricEntry.cs
- EntityProxyFactory.cs
- X509AsymmetricSecurityKey.cs
- EdmValidator.cs
- WorkflowTransactionOptions.cs
- ToolStripSettings.cs
- UIElement3D.cs
- ObjectNotFoundException.cs
- SqlFacetAttribute.cs
- Pair.cs
- ISFTagAndGuidCache.cs
- ReadOnlyObservableCollection.cs
- DataGridLinkButton.cs
- CompilerGlobalScopeAttribute.cs
- StyleCollection.cs
- XmlDataSourceDesigner.cs
- __Filters.cs
- MetafileHeader.cs
- SafeProcessHandle.cs
- ProcessModelInfo.cs
- CapabilitiesRule.cs
- XmlSchemaSimpleType.cs
- WebPartConnectionsCancelEventArgs.cs
- OdbcPermission.cs
- ConstNode.cs
- sqlcontext.cs
- ImportContext.cs
- CompositeControl.cs
- WebCategoryAttribute.cs
- TreeNodeSelectionProcessor.cs
- MonthCalendar.cs
- SqlDuplicator.cs
- WebPartVerbCollection.cs
- iisPickupDirectory.cs
- SQLString.cs
- ExpressionWriter.cs
- TimeoutValidationAttribute.cs
- MsdtcClusterUtils.cs
- LinearGradientBrush.cs
- EntityDataSourceReferenceGroup.cs
- HandleCollector.cs
- RelationshipEnd.cs
- DetailsViewRow.cs
- RouteData.cs
- SqlDataSource.cs
- MultiView.cs
- BevelBitmapEffect.cs
- RadialGradientBrush.cs
- MemberDescriptor.cs
- HyperLinkColumn.cs
- WizardForm.cs
- SymbolDocumentGenerator.cs
- StdRegProviderWrapper.cs
- Bits.cs
- TreeView.cs
- ClusterRegistryConfigurationProvider.cs
- SingleAnimationBase.cs
- DataRecordInternal.cs
- SqlTypeSystemProvider.cs
- ScriptMethodAttribute.cs
- ZoneButton.cs
- NativeObjectSecurity.cs
- DataServiceQueryException.cs
- OleDbPropertySetGuid.cs
- DecimalStorage.cs
- TextWriterTraceListener.cs