Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Controls / BorderGapMaskConverter.cs / 1305600 / BorderGapMaskConverter.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: Used to create a Gap in the Border for GroupBox style // //--------------------------------------------------------------------------- using System.Globalization; using System.Windows.Controls; using System.Windows.Data; using System.Windows.Media; using System.Windows.Shapes; namespace System.Windows.Controls { ////// BorderGapMaskConverter class /// public class BorderGapMaskConverter : IMultiValueConverter { ////// Convert a value. /// /// values as produced by source binding /// target type /// converter parameter /// culture information ////// Converted value. /// Visual Brush that is used as the opacity mask for the Border /// in the style for GroupBox. /// public object Convert(object[] values, Type targetType, object parameter, CultureInfo culture) { // // Parameter Validation // Type doubleType = typeof(double); if (parameter == null || values == null || values.Length != 3 || values[0] == null || values[1] == null || values[2] == null || !doubleType.IsAssignableFrom(values[0].GetType()) || !doubleType.IsAssignableFrom(values[1].GetType()) || !doubleType.IsAssignableFrom(values[2].GetType()) ) { return DependencyProperty.UnsetValue; } Type paramType = parameter.GetType(); if (!(doubleType.IsAssignableFrom(paramType) || typeof(string).IsAssignableFrom(paramType))) { return DependencyProperty.UnsetValue; } // // Conversion // double headerWidth = (double)values[0]; double borderWidth = (double)values[1]; double borderHeight = (double)values[2]; // Doesn't make sense to have a Grid // with 0 as width or height if (borderWidth == 0 || borderHeight == 0) { return null; } // Width of the line to the left of the header // to be used to set the width of the first column of the Grid double lineWidth; if (parameter is string) { lineWidth = Double.Parse(((string)parameter), NumberFormatInfo.InvariantInfo); } else { lineWidth = (double)parameter; } Grid grid = new Grid(); grid.Width = borderWidth; grid.Height = borderHeight; ColumnDefinition colDef1 = new ColumnDefinition(); ColumnDefinition colDef2 = new ColumnDefinition(); ColumnDefinition colDef3 = new ColumnDefinition(); colDef1.Width = new GridLength(lineWidth); colDef2.Width = new GridLength(headerWidth); colDef3.Width = new GridLength(1, GridUnitType.Star); grid.ColumnDefinitions.Add(colDef1); grid.ColumnDefinitions.Add(colDef2); grid.ColumnDefinitions.Add(colDef3); RowDefinition rowDef1 = new RowDefinition(); RowDefinition rowDef2 = new RowDefinition(); rowDef1.Height = new GridLength(borderHeight / 2); rowDef2.Height = new GridLength(1, GridUnitType.Star); grid.RowDefinitions.Add(rowDef1); grid.RowDefinitions.Add(rowDef2); Rectangle rectColumn1 = new Rectangle(); Rectangle rectColumn2 = new Rectangle(); Rectangle rectColumn3 = new Rectangle(); rectColumn1.Fill = Brushes.Black; rectColumn2.Fill = Brushes.Black; rectColumn3.Fill = Brushes.Black; Grid.SetRowSpan(rectColumn1, 2); Grid.SetRow(rectColumn1, 0); Grid.SetColumn(rectColumn1, 0); Grid.SetRow(rectColumn2, 1); Grid.SetColumn(rectColumn2, 1); Grid.SetRowSpan(rectColumn3, 2); Grid.SetRow(rectColumn3, 0); Grid.SetColumn(rectColumn3, 2); grid.Children.Add(rectColumn1); grid.Children.Add(rectColumn2); grid.Children.Add(rectColumn3); return (new VisualBrush(grid)); } ////// Not Supported /// /// value, as produced by target /// target types /// converter parameter /// culture information ///Nothing public object[] ConvertBack(object value, Type[] targetTypes, object parameter, CultureInfo culture) { return new object[] { Binding.DoNothing }; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
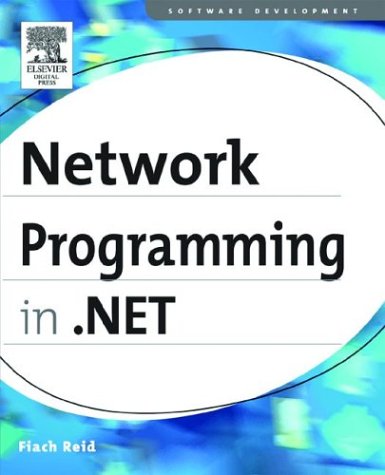
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- CodeDomSerializerException.cs
- SapiRecognizer.cs
- InsufficientMemoryException.cs
- Int32Converter.cs
- ScrollBar.cs
- PolyBezierSegmentFigureLogic.cs
- RootProfilePropertySettingsCollection.cs
- RectValueSerializer.cs
- ClonableStack.cs
- RadioButtonFlatAdapter.cs
- QueryOutputWriter.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- DataGridViewTextBoxColumn.cs
- TemplateBindingExpressionConverter.cs
- ImagingCache.cs
- PanelDesigner.cs
- FormatConvertedBitmap.cs
- Msec.cs
- ObjectDataSource.cs
- MetaModel.cs
- UniqueConstraint.cs
- ToolStripItemRenderEventArgs.cs
- SelectionEditor.cs
- MenuAdapter.cs
- MimeWriter.cs
- SatelliteContractVersionAttribute.cs
- AdCreatedEventArgs.cs
- EntityDataSourceQueryBuilder.cs
- ListBase.cs
- XmlSchemaElement.cs
- ControlCollection.cs
- WindowsScroll.cs
- ActivityTypeResolver.xaml.cs
- SmtpReplyReader.cs
- DesignTimeVisibleAttribute.cs
- ConfigurationSettings.cs
- ThicknessKeyFrameCollection.cs
- TablePatternIdentifiers.cs
- InputBindingCollection.cs
- ScrollPattern.cs
- ServiceHostFactory.cs
- Label.cs
- RichTextBox.cs
- RadioButtonPopupAdapter.cs
- XmlLinkedNode.cs
- GridLength.cs
- ScrollBar.cs
- DataMemberListEditor.cs
- Model3DGroup.cs
- ReaderOutput.cs
- BaseCAMarshaler.cs
- AsymmetricKeyExchangeFormatter.cs
- CategoryAttribute.cs
- CatalogPartCollection.cs
- ACL.cs
- ProcessInputEventArgs.cs
- SqlClientFactory.cs
- PeekCompletedEventArgs.cs
- SelectionProviderWrapper.cs
- Authorization.cs
- CollectionViewGroupRoot.cs
- PrefixHandle.cs
- AnnotationResourceChangedEventArgs.cs
- PlanCompilerUtil.cs
- EventMappingSettingsCollection.cs
- MailSettingsSection.cs
- _PooledStream.cs
- EndEvent.cs
- X509Utils.cs
- Html32TextWriter.cs
- SafeEventLogWriteHandle.cs
- ThumbAutomationPeer.cs
- SHA512.cs
- IndependentAnimationStorage.cs
- ToolStripDropDownMenu.cs
- ClassImporter.cs
- ImageField.cs
- RadioButtonAutomationPeer.cs
- JulianCalendar.cs
- Wizard.cs
- PerformanceCounterPermissionEntry.cs
- ActivityDelegate.cs
- DragDropHelper.cs
- DataGridRowHeaderAutomationPeer.cs
- ActiveXHost.cs
- TextRange.cs
- ForEachAction.cs
- UrlUtility.cs
- RectAnimationUsingKeyFrames.cs
- CompatibleComparer.cs
- DataRowCollection.cs
- LongAverageAggregationOperator.cs
- ImageSourceValueSerializer.cs
- XmlElement.cs
- ClickablePoint.cs
- TreeViewImageGenerator.cs
- RMEnrollmentPage3.cs
- FaultPropagationQuery.cs
- ControlIdConverter.cs
- WebPartActionVerb.cs