Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / QueryCache / CompiledQueryCacheEntry.cs / 1305376 / CompiledQueryCacheEntry.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Data.Objects.Internal; using System.Diagnostics; using System.Threading; ////// Represents a compiled LINQ ObjectQuery cache entry /// internal sealed class CompiledQueryCacheEntry : QueryCacheEntry { ////// The merge option that was inferred during expression conversion. /// public readonly MergeOption? PropagatedMergeOption; ////// The execution plan to use for the 'AppendOnly' merge option. /// private ObjectQueryExecutionPlan _appendOnlyPlan; ////// The execution plan to use for the 'NoTracking' merge option. /// private ObjectQueryExecutionPlan _noTrackingPlan; ////// The execution plan to use for the 'OverwriteChanges' merge option. /// private ObjectQueryExecutionPlan _overwriteChangesPlan; ////// The execution plan to use for the 'PreserveChanges' merge option. /// private ObjectQueryExecutionPlan _preserveChangesPlan; #region Constructors ////// constructor /// /// The cache key that targets this cache entry ///The inferred merge option that applies to this cached query internal CompiledQueryCacheEntry(QueryCacheKey queryCacheKey, MergeOption? mergeOption) : base(queryCacheKey, null) { this.PropagatedMergeOption = mergeOption; } #endregion #region Methods/Properties ////// Retrieves the execution plan for the specified merge option. May return null if the plan for that merge option has yet to be populated. /// /// The merge option for which an execution plan is required. ///The corresponding execution plan, if it exists; otherwise internal ObjectQueryExecutionPlan GetExecutionPlan(MergeOption mergeOption) { switch(mergeOption) { case MergeOption.AppendOnly: return this._appendOnlyPlan; case MergeOption.NoTracking: return this._noTrackingPlan; case MergeOption.OverwriteChanges: return this._overwriteChangesPlan; case MergeOption.PreserveChanges: return this._preserveChangesPlan; default: throw EntityUtil.ArgumentOutOfRange("mergeOption"); } } ///null ./// Attempts to set the execution plan for /// The new execution plan to add to this cache entry. ///'s merge option on this cache entry to . /// If a plan already exists for that merge option, the current value is not changed but is returned to the caller. /// Otherwise is returned to the caller. /// The execution plan that corresponds to internal ObjectQueryExecutionPlan SetExecutionPlan(ObjectQueryExecutionPlan newPlan) { Debug.Assert(newPlan != null, "New plan cannot be null"); ObjectQueryExecutionPlan currentPlan; switch(newPlan.MergeOption) { case MergeOption.AppendOnly: currentPlan = Interlocked.CompareExchange(ref _appendOnlyPlan, newPlan, null); break; case MergeOption.NoTracking: currentPlan = Interlocked.CompareExchange(ref _noTrackingPlan, newPlan, null); break; case MergeOption.OverwriteChanges: currentPlan = Interlocked.CompareExchange(ref _overwriteChangesPlan, newPlan, null); break; case MergeOption.PreserveChanges: currentPlan = Interlocked.CompareExchange(ref _preserveChangesPlan, newPlan, null); break; default: throw EntityUtil.ArgumentOutOfRange("newPlan.MergeOption"); } return (currentPlan ?? newPlan); } ///'s merge option, which may be or may be a previously added execution plan. /// Convenience method to retrieve the result type from the first non-null execution plan found on this cache entry. /// /// The result type of any execution plan that is or could be added to this cache entry ///internal bool TryGetResultType(out TypeUsage resultType) { // AppendOnly - the default ObjectQueryExecutionPlan plan = this._appendOnlyPlan; if (plan != null) { resultType = plan.ResultType; return true; } // NoTracking plan = this._noTrackingPlan; if (plan != null) { resultType = plan.ResultType; return true; } // OverwriteChanges plan = this._overwriteChangesPlan; if (plan != null) { resultType = plan.ResultType; return true; } // PreserveChanges plan = this._preserveChangesPlan; if (plan != null) { resultType = plan.ResultType; return true; } // No plan is present resultType = null; return false; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // true if at least one execution plan was present and a result type could be retrieved; otherwisefalse // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //----------------------------------------------------------------------------- namespace System.Data.Common.QueryCache { using System; using System.Data.Metadata.Edm; using System.Data.Objects; using System.Data.Objects.Internal; using System.Diagnostics; using System.Threading; ////// Represents a compiled LINQ ObjectQuery cache entry /// internal sealed class CompiledQueryCacheEntry : QueryCacheEntry { ////// The merge option that was inferred during expression conversion. /// public readonly MergeOption? PropagatedMergeOption; ////// The execution plan to use for the 'AppendOnly' merge option. /// private ObjectQueryExecutionPlan _appendOnlyPlan; ////// The execution plan to use for the 'NoTracking' merge option. /// private ObjectQueryExecutionPlan _noTrackingPlan; ////// The execution plan to use for the 'OverwriteChanges' merge option. /// private ObjectQueryExecutionPlan _overwriteChangesPlan; ////// The execution plan to use for the 'PreserveChanges' merge option. /// private ObjectQueryExecutionPlan _preserveChangesPlan; #region Constructors ////// constructor /// /// The cache key that targets this cache entry ///The inferred merge option that applies to this cached query internal CompiledQueryCacheEntry(QueryCacheKey queryCacheKey, MergeOption? mergeOption) : base(queryCacheKey, null) { this.PropagatedMergeOption = mergeOption; } #endregion #region Methods/Properties ////// Retrieves the execution plan for the specified merge option. May return null if the plan for that merge option has yet to be populated. /// /// The merge option for which an execution plan is required. ///The corresponding execution plan, if it exists; otherwise internal ObjectQueryExecutionPlan GetExecutionPlan(MergeOption mergeOption) { switch(mergeOption) { case MergeOption.AppendOnly: return this._appendOnlyPlan; case MergeOption.NoTracking: return this._noTrackingPlan; case MergeOption.OverwriteChanges: return this._overwriteChangesPlan; case MergeOption.PreserveChanges: return this._preserveChangesPlan; default: throw EntityUtil.ArgumentOutOfRange("mergeOption"); } } ///null ./// Attempts to set the execution plan for /// The new execution plan to add to this cache entry. ///'s merge option on this cache entry to . /// If a plan already exists for that merge option, the current value is not changed but is returned to the caller. /// Otherwise is returned to the caller. /// The execution plan that corresponds to internal ObjectQueryExecutionPlan SetExecutionPlan(ObjectQueryExecutionPlan newPlan) { Debug.Assert(newPlan != null, "New plan cannot be null"); ObjectQueryExecutionPlan currentPlan; switch(newPlan.MergeOption) { case MergeOption.AppendOnly: currentPlan = Interlocked.CompareExchange(ref _appendOnlyPlan, newPlan, null); break; case MergeOption.NoTracking: currentPlan = Interlocked.CompareExchange(ref _noTrackingPlan, newPlan, null); break; case MergeOption.OverwriteChanges: currentPlan = Interlocked.CompareExchange(ref _overwriteChangesPlan, newPlan, null); break; case MergeOption.PreserveChanges: currentPlan = Interlocked.CompareExchange(ref _preserveChangesPlan, newPlan, null); break; default: throw EntityUtil.ArgumentOutOfRange("newPlan.MergeOption"); } return (currentPlan ?? newPlan); } ///'s merge option, which may be or may be a previously added execution plan. /// Convenience method to retrieve the result type from the first non-null execution plan found on this cache entry. /// /// The result type of any execution plan that is or could be added to this cache entry ///internal bool TryGetResultType(out TypeUsage resultType) { // AppendOnly - the default ObjectQueryExecutionPlan plan = this._appendOnlyPlan; if (plan != null) { resultType = plan.ResultType; return true; } // NoTracking plan = this._noTrackingPlan; if (plan != null) { resultType = plan.ResultType; return true; } // OverwriteChanges plan = this._overwriteChangesPlan; if (plan != null) { resultType = plan.ResultType; return true; } // PreserveChanges plan = this._preserveChangesPlan; if (plan != null) { resultType = plan.ResultType; return true; } // No plan is present resultType = null; return false; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. true if at least one execution plan was present and a result type could be retrieved; otherwisefalse
Link Menu
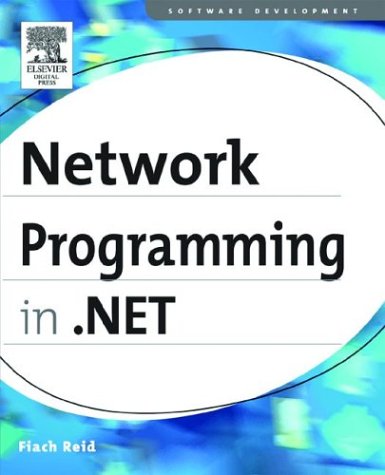
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- WeakHashtable.cs
- PropertyAccessVisitor.cs
- LineVisual.cs
- SamlAssertion.cs
- PriorityQueue.cs
- FrameworkTextComposition.cs
- AddDataControlFieldDialog.cs
- DocumentXPathNavigator.cs
- Converter.cs
- ComboBoxRenderer.cs
- BaseHashHelper.cs
- EditorPartDesigner.cs
- Int64Storage.cs
- FontUnit.cs
- DesignTimeVisibleAttribute.cs
- HoistedLocals.cs
- TabRenderer.cs
- SignatureGenerator.cs
- StringFunctions.cs
- SqlWorkflowPersistenceService.cs
- Label.cs
- ExpressionNode.cs
- PreloadedPackages.cs
- FixedLineResult.cs
- NamedPermissionSet.cs
- Rijndael.cs
- PageSettings.cs
- cookiecontainer.cs
- DocumentViewerConstants.cs
- HashCryptoHandle.cs
- CapabilitiesState.cs
- ConstrainedDataObject.cs
- ParameterReplacerVisitor.cs
- SymmetricSecurityProtocol.cs
- SynchronizedDispatch.cs
- SqlGatherConsumedAliases.cs
- CharAnimationUsingKeyFrames.cs
- AppearanceEditorPart.cs
- SByte.cs
- FileDialogCustomPlace.cs
- UdpTransportSettingsElement.cs
- DataGridRowAutomationPeer.cs
- CroppedBitmap.cs
- ThreadPool.cs
- MarshalByRefObject.cs
- Thread.cs
- FullTextLine.cs
- DirectoryGroupQuery.cs
- localization.cs
- CommandHelper.cs
- NodeCounter.cs
- ViewManager.cs
- KeyedPriorityQueue.cs
- DayRenderEvent.cs
- TimeoutException.cs
- UnknownWrapper.cs
- MissingMemberException.cs
- InputLanguageCollection.cs
- Rotation3DAnimationUsingKeyFrames.cs
- ValidatingReaderNodeData.cs
- GridViewPageEventArgs.cs
- LoginUtil.cs
- InvalidOperationException.cs
- AppDomainAttributes.cs
- PointCollection.cs
- ImageButton.cs
- EventProxy.cs
- RegionInfo.cs
- Convert.cs
- HttpHandlerAction.cs
- RectangleGeometry.cs
- CompiledQueryCacheKey.cs
- Vector3DAnimationUsingKeyFrames.cs
- SafePipeHandle.cs
- X509RecipientCertificateClientElement.cs
- DiffuseMaterial.cs
- DiscoveryDocumentSerializer.cs
- RelatedView.cs
- ExtendedPropertyCollection.cs
- ClientRoleProvider.cs
- TypefaceMap.cs
- DefaultParameterValueAttribute.cs
- TypeKeyValue.cs
- Int64Converter.cs
- TypeUnloadedException.cs
- DataGridTablesFactory.cs
- StringKeyFrameCollection.cs
- sitestring.cs
- LineSegment.cs
- SrgsItemList.cs
- PropertySourceInfo.cs
- NativeMethods.cs
- XPathScanner.cs
- XmlDataContract.cs
- UnregisterInfo.cs
- NeutralResourcesLanguageAttribute.cs
- HtmlWindow.cs
- SoapFault.cs
- BitmapFrameDecode.cs
- rsa.cs