Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / AccessControlList.cs / 1305376 / AccessControlList.cs
using System; using System.Security.Permissions; using System.Security; using System.Collections; using System.Runtime.InteropServices; using System.ComponentModel; using System.Text; using System.Messaging.Interop; namespace System.Messaging { ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1039:ListsAreStronglyTyped")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public class AccessControlList : CollectionBase { internal static readonly int UnknownEnvironment = 0; internal static readonly int W2kEnvironment = 1; internal static readonly int NtEnvironment = 2; internal static readonly int NonNtEnvironment = 3; private static int environment = UnknownEnvironment; private static object staticLock = new object(); ///[To be supplied.] ///public AccessControlList() { } internal static int CurrentEnvironment { get { if (environment == UnknownEnvironment) { lock (AccessControlList.staticLock) { if (environment == UnknownEnvironment) { //SECREVIEW: [....]- need to assert Environment permissions here // the environment check is not exposed as a public // method EnvironmentPermission environmentPermission = new EnvironmentPermission(PermissionState.Unrestricted); environmentPermission.Assert(); try { if (Environment.OSVersion.Platform == PlatformID.Win32NT) { if (Environment.OSVersion.Version.Major >= 5) environment = W2kEnvironment; else environment = NtEnvironment; } else environment = NonNtEnvironment; } finally { EnvironmentPermission.RevertAssert(); } } } } return environment; } } /// /// /// public int Add(AccessControlEntry entry) { return List.Add(entry); } ///[To be supplied.] ////// /// public void Insert(int index, AccessControlEntry entry) { List.Insert(index, entry); } ///[To be supplied.] ////// /// public int IndexOf(AccessControlEntry entry) { return List.IndexOf(entry); } internal static void CheckEnvironment() { if (CurrentEnvironment == NonNtEnvironment) throw new PlatformNotSupportedException(Res.GetString(Res.WinNTRequired)); } ///[To be supplied.] ////// /// public bool Contains(AccessControlEntry entry) { return List.Contains(entry); } ///[To be supplied.] ////// /// public void Remove(AccessControlEntry entry) { List.Remove(entry); } ///[To be supplied.] ////// /// public void CopyTo(AccessControlEntry[] array, int index) { List.CopyTo(array, index); } internal IntPtr MakeAcl(IntPtr oldAcl) { CheckEnvironment(); int ACECount = List.Count; IntPtr newAcl; NativeMethods.ExplicitAccess[] entries = new NativeMethods.ExplicitAccess[ACECount]; GCHandle mem = GCHandle.Alloc(entries, GCHandleType.Pinned); try { for (int i = 0; i < ACECount; i++) { int sidSize = 0; int sidtype; int domainSize = 0; AccessControlEntry ace = (AccessControlEntry)List[i]; if (ace.Trustee == null) throw new InvalidOperationException(Res.GetString(Res.InvalidTrustee)); string name = ace.Trustee.Name; if (name == null) throw new InvalidOperationException(Res.GetString(Res.InvalidTrusteeName)); if ((ace.Trustee.TrusteeType == TrusteeType.Computer) && !name.EndsWith("$")) name += "$"; if (!UnsafeNativeMethods.LookupAccountName(ace.Trustee.SystemName, name, (IntPtr)0, ref sidSize, null, ref domainSize, out sidtype)) { int errval = Marshal.GetLastWin32Error(); if (errval != 122) throw new InvalidOperationException(Res.GetString(Res.CouldntResolve ,ace.Trustee.Name, errval)); } entries[i].data = (IntPtr)Marshal.AllocHGlobal(sidSize); StringBuilder domainName = new StringBuilder(domainSize); if (!UnsafeNativeMethods.LookupAccountName(ace.Trustee.SystemName, name, entries[i].data, ref sidSize, domainName, ref domainSize, out sidtype)) throw new InvalidOperationException(Res.GetString(Res.CouldntResolveName, ace.Trustee.Name)); entries[i].grfAccessPermissions = ace.accessFlags; entries[i].grfAccessMode = (int)ace.EntryType; entries[i].grfInheritance = 0; entries[i].pMultipleTrustees = (IntPtr)0; entries[i].MultipleTrusteeOperation = NativeMethods.NO_MULTIPLE_TRUSTEE; entries[i].TrusteeForm = NativeMethods.TRUSTEE_IS_SID; entries[i].TrusteeType = (int)ace.Trustee.TrusteeType; } int err = SafeNativeMethods.SetEntriesInAclW(ACECount, (IntPtr)mem.AddrOfPinnedObject(), oldAcl, out newAcl); if (err != NativeMethods.ERROR_SUCCESS) throw new Win32Exception(err); } finally { mem.Free(); for (int i = 0; i < ACECount; i++) if (entries[i].data != (IntPtr)0) Marshal.FreeHGlobal(entries[i].data); } return newAcl; } internal static void FreeAcl(IntPtr acl) { SafeNativeMethods.LocalFree(acl); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Security.Permissions; using System.Security; using System.Collections; using System.Runtime.InteropServices; using System.ComponentModel; using System.Text; using System.Messaging.Interop; namespace System.Messaging { ///[To be supplied.] ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1039:ListsAreStronglyTyped")] [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1710:IdentifiersShouldHaveCorrectSuffix")] public class AccessControlList : CollectionBase { internal static readonly int UnknownEnvironment = 0; internal static readonly int W2kEnvironment = 1; internal static readonly int NtEnvironment = 2; internal static readonly int NonNtEnvironment = 3; private static int environment = UnknownEnvironment; private static object staticLock = new object(); ///[To be supplied.] ///public AccessControlList() { } internal static int CurrentEnvironment { get { if (environment == UnknownEnvironment) { lock (AccessControlList.staticLock) { if (environment == UnknownEnvironment) { //SECREVIEW: [....]- need to assert Environment permissions here // the environment check is not exposed as a public // method EnvironmentPermission environmentPermission = new EnvironmentPermission(PermissionState.Unrestricted); environmentPermission.Assert(); try { if (Environment.OSVersion.Platform == PlatformID.Win32NT) { if (Environment.OSVersion.Version.Major >= 5) environment = W2kEnvironment; else environment = NtEnvironment; } else environment = NonNtEnvironment; } finally { EnvironmentPermission.RevertAssert(); } } } } return environment; } } /// /// /// public int Add(AccessControlEntry entry) { return List.Add(entry); } ///[To be supplied.] ////// /// public void Insert(int index, AccessControlEntry entry) { List.Insert(index, entry); } ///[To be supplied.] ////// /// public int IndexOf(AccessControlEntry entry) { return List.IndexOf(entry); } internal static void CheckEnvironment() { if (CurrentEnvironment == NonNtEnvironment) throw new PlatformNotSupportedException(Res.GetString(Res.WinNTRequired)); } ///[To be supplied.] ////// /// public bool Contains(AccessControlEntry entry) { return List.Contains(entry); } ///[To be supplied.] ////// /// public void Remove(AccessControlEntry entry) { List.Remove(entry); } ///[To be supplied.] ////// /// public void CopyTo(AccessControlEntry[] array, int index) { List.CopyTo(array, index); } internal IntPtr MakeAcl(IntPtr oldAcl) { CheckEnvironment(); int ACECount = List.Count; IntPtr newAcl; NativeMethods.ExplicitAccess[] entries = new NativeMethods.ExplicitAccess[ACECount]; GCHandle mem = GCHandle.Alloc(entries, GCHandleType.Pinned); try { for (int i = 0; i < ACECount; i++) { int sidSize = 0; int sidtype; int domainSize = 0; AccessControlEntry ace = (AccessControlEntry)List[i]; if (ace.Trustee == null) throw new InvalidOperationException(Res.GetString(Res.InvalidTrustee)); string name = ace.Trustee.Name; if (name == null) throw new InvalidOperationException(Res.GetString(Res.InvalidTrusteeName)); if ((ace.Trustee.TrusteeType == TrusteeType.Computer) && !name.EndsWith("$")) name += "$"; if (!UnsafeNativeMethods.LookupAccountName(ace.Trustee.SystemName, name, (IntPtr)0, ref sidSize, null, ref domainSize, out sidtype)) { int errval = Marshal.GetLastWin32Error(); if (errval != 122) throw new InvalidOperationException(Res.GetString(Res.CouldntResolve ,ace.Trustee.Name, errval)); } entries[i].data = (IntPtr)Marshal.AllocHGlobal(sidSize); StringBuilder domainName = new StringBuilder(domainSize); if (!UnsafeNativeMethods.LookupAccountName(ace.Trustee.SystemName, name, entries[i].data, ref sidSize, domainName, ref domainSize, out sidtype)) throw new InvalidOperationException(Res.GetString(Res.CouldntResolveName, ace.Trustee.Name)); entries[i].grfAccessPermissions = ace.accessFlags; entries[i].grfAccessMode = (int)ace.EntryType; entries[i].grfInheritance = 0; entries[i].pMultipleTrustees = (IntPtr)0; entries[i].MultipleTrusteeOperation = NativeMethods.NO_MULTIPLE_TRUSTEE; entries[i].TrusteeForm = NativeMethods.TRUSTEE_IS_SID; entries[i].TrusteeType = (int)ace.Trustee.TrusteeType; } int err = SafeNativeMethods.SetEntriesInAclW(ACECount, (IntPtr)mem.AddrOfPinnedObject(), oldAcl, out newAcl); if (err != NativeMethods.ERROR_SUCCESS) throw new Win32Exception(err); } finally { mem.Free(); for (int i = 0; i < ACECount; i++) if (entries[i].data != (IntPtr)0) Marshal.FreeHGlobal(entries[i].data); } return newAcl; } internal static void FreeAcl(IntPtr acl) { SafeNativeMethods.LocalFree(acl); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.[To be supplied.] ///
Link Menu
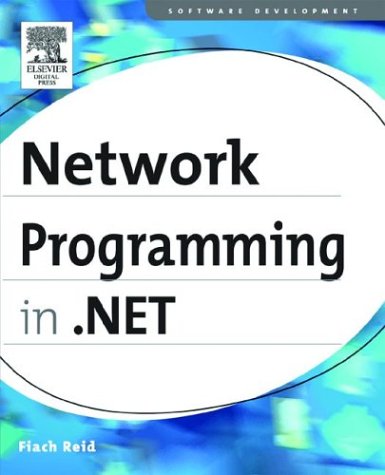
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RestHandler.cs
- BCryptHashAlgorithm.cs
- RecognizeCompletedEventArgs.cs
- RSACryptoServiceProvider.cs
- InputProcessorProfilesLoader.cs
- SystemIPv6InterfaceProperties.cs
- TextMetrics.cs
- ProxyWebPart.cs
- HitTestParameters3D.cs
- MsmqElementBase.cs
- Delegate.cs
- Baml2006ReaderFrame.cs
- FormsAuthenticationEventArgs.cs
- CompilerInfo.cs
- DbModificationClause.cs
- ButtonBase.cs
- PrimitiveList.cs
- TypeDescriptorFilterService.cs
- DataBoundControl.cs
- PropertyEntry.cs
- UserControl.cs
- DesignerTextViewAdapter.cs
- Comparer.cs
- SynchronizationScope.cs
- InvalidPrinterException.cs
- HttpClientCertificate.cs
- HyperlinkAutomationPeer.cs
- base64Transforms.cs
- DiscoveryDocumentSearchPattern.cs
- BasicCommandTreeVisitor.cs
- PromptBuilder.cs
- List.cs
- XmlDocumentFieldSchema.cs
- IsolatedStorageException.cs
- StylusPointProperties.cs
- _HeaderInfo.cs
- MimeTypePropertyAttribute.cs
- PeerDefaultCustomResolverClient.cs
- ReliabilityContractAttribute.cs
- SourceChangedEventArgs.cs
- NamedPipeAppDomainProtocolHandler.cs
- BindingOperations.cs
- Signature.cs
- ColorTransformHelper.cs
- EntityDataSourceStatementEditorForm.cs
- TemplatePropertyEntry.cs
- NameTable.cs
- PartialClassGenerationTaskInternal.cs
- CannotUnloadAppDomainException.cs
- DataTableClearEvent.cs
- DataGrid.cs
- DesignerView.cs
- ProcessMonitor.cs
- FileDataSourceCache.cs
- TextTreeDeleteContentUndoUnit.cs
- LockCookie.cs
- TypeDependencyAttribute.cs
- DefaultValidator.cs
- AlignmentXValidation.cs
- WindowsMenu.cs
- BindToObject.cs
- BaseTreeIterator.cs
- DiscriminatorMap.cs
- ValidationContext.cs
- ILGenerator.cs
- TakeQueryOptionExpression.cs
- _NegoStream.cs
- WeakReferenceEnumerator.cs
- SqlExpressionNullability.cs
- GetMemberBinder.cs
- DayRenderEvent.cs
- StylusPointPropertyUnit.cs
- CheckedListBox.cs
- ProfessionalColorTable.cs
- ListenerElementsCollection.cs
- HttpAsyncResult.cs
- AttachedPropertyBrowsableWhenAttributePresentAttribute.cs
- ValueTypeFixupInfo.cs
- Evidence.cs
- OverrideMode.cs
- TreeNodeCollectionEditorDialog.cs
- StorageSetMapping.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- GlyphRunDrawing.cs
- CompositeCollection.cs
- LockCookie.cs
- ListMarkerLine.cs
- SpellCheck.cs
- UserControlBuildProvider.cs
- MasterPage.cs
- SwitchAttribute.cs
- MaterialCollection.cs
- FocusWithinProperty.cs
- RelativeSource.cs
- HandleRef.cs
- OleDbSchemaGuid.cs
- TextDecorationCollection.cs
- EarlyBoundInfo.cs
- MetadataUtilsSmi.cs
- NativeRightsManagementAPIsStructures.cs