Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / WebServiceAttribute.cs / 1305376 / WebServiceAttribute.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services { using System; using System.Web.Services.Protocols; ////// /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface)] public sealed class WebServiceAttribute : Attribute { string description; string ns = DefaultNamespace; string name; ///The WebService attribute may be used to add additional information to a /// Web Service, such as a string describing its functionality. The attribute is not required for a Web Service to /// be published and executed. ////// /// Initializes a new instance of the public WebServiceAttribute() { } ///class. /// /// /// A descriptive message for the Web Service. The message /// is used when generating description documents for the Web Service, such as the /// Sevice Contract and the Service Description page. /// public string Description { get { return (description == null) ? string.Empty : description; } set { description = value; } } ////// /// The default XML namespace to use for the web service. /// public string Namespace { get { return ns; } set { ns = value; } } ////// /// The name to use for the web service in the service description. /// public string Name { get { return name == null ? string.Empty : name; } set { name = value; } } ////// /// The default value for the XmlNamespace property. /// public const string DefaultNamespace = "http://tempuri.org/"; } internal class WebServiceReflector { private WebServiceReflector() {} internal static WebServiceAttribute GetAttribute(Type type) { object[] attrs = type.GetCustomAttributes(typeof(WebServiceAttribute), false); if (attrs.Length == 0) return new WebServiceAttribute(); return (WebServiceAttribute)attrs[0]; } internal static WebServiceAttribute GetAttribute(LogicalMethodInfo[] methodInfos) { if (methodInfos.Length == 0) return new WebServiceAttribute(); Type mostDerived = GetMostDerivedType(methodInfos); return GetAttribute(mostDerived); } internal static Type GetMostDerivedType(LogicalMethodInfo[] methodInfos) { if (methodInfos.Length == 0) return null; Type mostDerived = methodInfos[0].DeclaringType; for (int i = 1; i < methodInfos.Length; i++) { Type derived = methodInfos[i].DeclaringType; if (derived.IsSubclassOf(mostDerived)) { mostDerived = derived; } } return mostDerived; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services { using System; using System.Web.Services.Protocols; ////// /// [AttributeUsage(AttributeTargets.Class | AttributeTargets.Interface)] public sealed class WebServiceAttribute : Attribute { string description; string ns = DefaultNamespace; string name; ///The WebService attribute may be used to add additional information to a /// Web Service, such as a string describing its functionality. The attribute is not required for a Web Service to /// be published and executed. ////// /// Initializes a new instance of the public WebServiceAttribute() { } ///class. /// /// /// A descriptive message for the Web Service. The message /// is used when generating description documents for the Web Service, such as the /// Sevice Contract and the Service Description page. /// public string Description { get { return (description == null) ? string.Empty : description; } set { description = value; } } ////// /// The default XML namespace to use for the web service. /// public string Namespace { get { return ns; } set { ns = value; } } ////// /// The name to use for the web service in the service description. /// public string Name { get { return name == null ? string.Empty : name; } set { name = value; } } ////// /// The default value for the XmlNamespace property. /// public const string DefaultNamespace = "http://tempuri.org/"; } internal class WebServiceReflector { private WebServiceReflector() {} internal static WebServiceAttribute GetAttribute(Type type) { object[] attrs = type.GetCustomAttributes(typeof(WebServiceAttribute), false); if (attrs.Length == 0) return new WebServiceAttribute(); return (WebServiceAttribute)attrs[0]; } internal static WebServiceAttribute GetAttribute(LogicalMethodInfo[] methodInfos) { if (methodInfos.Length == 0) return new WebServiceAttribute(); Type mostDerived = GetMostDerivedType(methodInfos); return GetAttribute(mostDerived); } internal static Type GetMostDerivedType(LogicalMethodInfo[] methodInfos) { if (methodInfos.Length == 0) return null; Type mostDerived = methodInfos[0].DeclaringType; for (int i = 1; i < methodInfos.Length; i++) { Type derived = methodInfos[i].DeclaringType; if (derived.IsSubclassOf(mostDerived)) { mostDerived = derived; } } return mostDerived; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
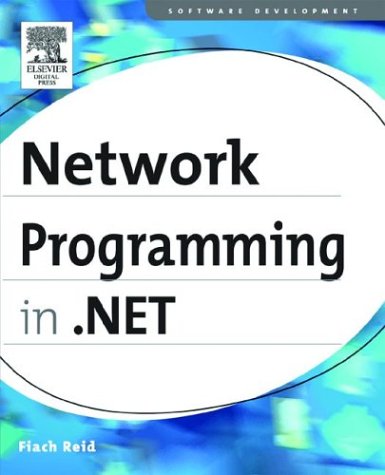
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ValueQuery.cs
- ContainerParagraph.cs
- ScopeCollection.cs
- ClaimComparer.cs
- UntypedNullExpression.cs
- SuspendDesigner.cs
- CodeExporter.cs
- BevelBitmapEffect.cs
- FormView.cs
- SqlMethodAttribute.cs
- NativeMethodsOther.cs
- CompiledAction.cs
- SignatureConfirmationElement.cs
- CodeDirectoryCompiler.cs
- MemoryPressure.cs
- RectangleGeometry.cs
- ForEachAction.cs
- ContentPresenter.cs
- QueryOperator.cs
- XamlToRtfParser.cs
- ISFTagAndGuidCache.cs
- FormsAuthenticationEventArgs.cs
- InternalBufferManager.cs
- CombinedTcpChannel.cs
- ReflectionPermission.cs
- Emitter.cs
- EntityContainerEntitySet.cs
- LinqExpressionNormalizer.cs
- EdmProviderManifest.cs
- TimeSpanMinutesConverter.cs
- CallbackValidator.cs
- Enum.cs
- DataListDesigner.cs
- CodeDirectionExpression.cs
- KeyTimeConverter.cs
- OrderingQueryOperator.cs
- StringValueConverter.cs
- RemotingServices.cs
- ConfigXmlWhitespace.cs
- InternalBufferManager.cs
- PeerResolverSettings.cs
- TextDecorations.cs
- AlphabetConverter.cs
- UICuesEvent.cs
- PropertyToken.cs
- GridItemPatternIdentifiers.cs
- FixedSOMFixedBlock.cs
- Stroke2.cs
- NetworkInterface.cs
- SqlUnionizer.cs
- GPRECT.cs
- TypeUsage.cs
- CodeDOMUtility.cs
- GridViewSortEventArgs.cs
- ControlTemplate.cs
- ContractBase.cs
- CompressedStack.cs
- RuntimeConfig.cs
- PreProcessor.cs
- MinimizableAttributeTypeConverter.cs
- ReadContentAsBinaryHelper.cs
- AndCondition.cs
- MsmqIntegrationBinding.cs
- ManagementPath.cs
- ValueQuery.cs
- SafeIUnknown.cs
- DataGridViewCellParsingEventArgs.cs
- IndexExpression.cs
- EventLogPropertySelector.cs
- ParallelEnumerable.cs
- ScheduleChanges.cs
- TableCell.cs
- TriState.cs
- ContractNamespaceAttribute.cs
- GeneralTransform2DTo3DTo2D.cs
- BitmapFrame.cs
- AsymmetricSignatureFormatter.cs
- RealizationDrawingContextWalker.cs
- ListViewInsertionMark.cs
- ExeConfigurationFileMap.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- SchemaElementDecl.cs
- _NetworkingPerfCounters.cs
- DocumentGridPage.cs
- ClassDataContract.cs
- PreservationFileWriter.cs
- ProviderConnectionPoint.cs
- SelectionEditingBehavior.cs
- TypeDescriptor.cs
- IPAddressCollection.cs
- SurrogateSelector.cs
- SymbolUsageManager.cs
- DataSourceXmlSerializer.cs
- WorkflowRuntimeServiceElementCollection.cs
- VisualStyleRenderer.cs
- CompiledQuery.cs
- WizardPanel.cs
- DataGridCellsPanel.cs
- GeometryGroup.cs
- ClientConvert.cs